What is bash? How to use it ?
What is Bash? Bash (Bourne Again Shell) is a command-line shell and scripting language used primarily in Unix-based operating systems like Linux and macOS. It is the default shell for many Linux distributions and macOS. Bash allows users to interact with the operating system by typing commands, running scripts, and automating tasks. Key Features of Bash 1. Command Execution: Run commands to interact with the operating system, manage files, and execute programs. 2.Scripting: Write scripts to automate repetitive tasks or complex workflows. 3.Environment Variables: Store and manipulate system and user-defined variables. 4.Pipelines and Redirection: Combine commands using pipes (|) and redirect input/output ( >, >). 5.Job Control: Manage background processes, suspend jobs, and bring them to the foreground. 6.Customization: Configure the shell environment using configuration files like .bashrc and .bash_profile. How to Use Bash 1. Accessing Bash On Linux or macOS, open the Terminal application. On Windows, you can use Git Bash, Windows Subsystem for Linux (WSL), or a terminal emulator like Cygwin. 2. Basic Commands Here are some common Bash commands: Example: ls -l # List files in long format cd /home/user/Documents # Change to the Documents directory pwd # Print the current directory 3. Environment Variables View all environment variables: printenv Set a variable: export MY_VAR="Hello, World!" Access a variable: echo $MY_VAR 4. Input/Output Redirection Redirect output to a file: echo "Hello" > output.txt Append to a file: echo "World" >> output.txt Redirect input from a file: cat < input.txt 5. Pipelines Combine commands using pipes (|): ls -l | grep ".txt" # List files and filter for .txt files 6. Scripting Bash scripts are text files containing a series of commands. To create a script: Create a file with a .sh extension: nano myscript.sh Add the following to the file: #!/bin/bash echo "Hello, World!" Make the script executable: chmod +x myscript.sh Run the script: ./myscript.sh 7. Conditionals and Loops If Statement: if [ "$VAR" == "value" ]; then echo "Match found!" else echo "No match." fi For Loop: for i in {1..5}; do echo "Iteration $i" done While Loop: count=1 while [ $count -le 5 ]; do echo "Count: $count" count=$((count + 1)) done 8. Functions Define and use functions in Bash: greet() { echo "Hello, $1!" } greet "Alice" 9. Job Control Run a command in the background: sleep 10 & List background jobs: jobs Bring a job to the foreground: fg %1 10. Customizing Bash Edit the .bashrc or .bash_profile file in your home directory to customize your shell environment. Example: nano ~/.bashrc Add aliases or environment variables: alias ll='ls -la' export PATH=$PATH:/custom/path Example Bash Script Here’s a simple script that lists files, checks for a specific file, and prints a message: #!/bin/bash # List files echo "Files in the current directory:" ls # Check if a file exists if [ -f "example.txt" ]; then echo "example.txt exists!" else echo "example.txt does not exist." fi Summary Bash is a powerful tool for interacting with Unix-based systems. You can use it to: Run commands and manage files. Write scripts to automate tasks. Customize your shell environment. To get started, open a terminal and experiment with basic commands. As you become more comfortable, explore scripting and advanced features like pipelines, conditionals, and loops. For more details, refer to the Bash manual (man bash) or online resources.
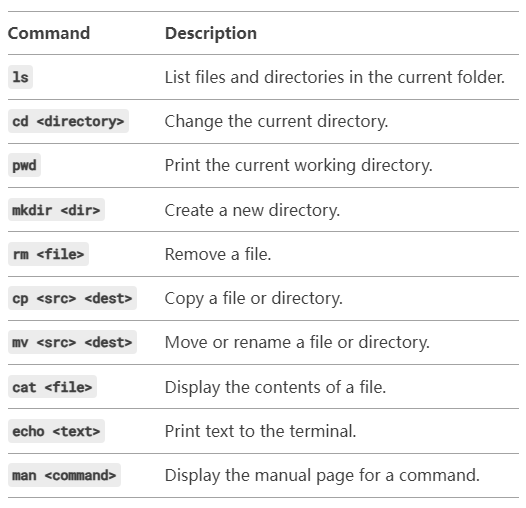
What is Bash?
Bash (Bourne Again Shell) is a command-line shell and scripting language used primarily in Unix-based operating systems like Linux and macOS. It is the default shell for many Linux distributions and macOS. Bash allows users to interact with the operating system by typing commands, running scripts, and automating tasks.
Key Features of Bash
1. Command Execution:
- Run commands to interact with the operating system, manage files, and execute programs.
2.Scripting:
- Write scripts to automate repetitive tasks or complex workflows.
3.Environment Variables:
- Store and manipulate system and user-defined variables.
4.Pipelines and Redirection:
- Combine commands using pipes (
|
) and redirect input/output (>
,<
,>>
).
5.Job Control:
- Manage background processes, suspend jobs, and bring them to the foreground.
6.Customization:
- Configure the shell environment using configuration files like
.bashrc
and.bash_profile
.
How to Use Bash
1. Accessing Bash
- On Linux or macOS, open the Terminal application.
- On Windows, you can use Git Bash, Windows Subsystem for Linux (WSL), or a terminal emulator like Cygwin.
2. Basic Commands
Here are some common Bash commands:
Example:
ls -l # List files in long format
cd /home/user/Documents # Change to the Documents directory
pwd # Print the current directory
3. Environment Variables
- View all environment variables:
printenv
- Set a variable:
export MY_VAR="Hello, World!"
- Access a variable:
echo $MY_VAR
4. Input/Output Redirection
- Redirect output to a file:
echo "Hello" > output.txt
- Append to a file:
echo "World" >> output.txt
- Redirect input from a file:
cat < input.txt
5. Pipelines
- Combine commands using pipes (
|
):
ls -l | grep ".txt" # List files and filter for .txt files
6. Scripting
Bash scripts are text files containing a series of commands. To create a script:
- Create a file with a
.sh
extension:
nano myscript.sh
- Add the following to the file:
#!/bin/bash
echo "Hello, World!"
- Make the script executable:
chmod +x myscript.sh
- Run the script:
./myscript.sh
7. Conditionals and Loops
- If Statement:
if [ "$VAR" == "value" ]; then
echo "Match found!"
else
echo "No match."
fi
- For Loop:
for i in {1..5}; do
echo "Iteration $i"
done
- While Loop:
count=1
while [ $count -le 5 ]; do
echo "Count: $count"
count=$((count + 1))
done
8. Functions
Define and use functions in Bash:
greet() {
echo "Hello, $1!"
}
greet "Alice"
9. Job Control
- Run a command in the background:
sleep 10 &
- List background jobs:
jobs
- Bring a job to the foreground:
fg %1
10. Customizing Bash
Edit the
.bashrc
or.bash_profile
file in your home directory to customize your shell environment.Example:
nano ~/.bashrc
Add aliases or environment variables:
alias ll='ls -la'
export PATH=$PATH:/custom/path
Example Bash Script
Here’s a simple script that lists files, checks for a specific file, and prints a message:
#!/bin/bash
# List files
echo "Files in the current directory:"
ls
# Check if a file exists
if [ -f "example.txt" ]; then
echo "example.txt exists!"
else
echo "example.txt does not exist."
fi
Summary
Bash is a powerful tool for interacting with Unix-based systems. You can use it to:
- Run commands and manage files.
- Write scripts to automate tasks.
- Customize your shell environment.
To get started, open a terminal and experiment with basic commands. As you become more comfortable, explore scripting and advanced features like pipelines, conditionals, and loops. For more details, refer to the Bash manual (man bash
) or online resources.