Building an AI Chatbot with Google AI Studio & Flask. And how you can create one yourself.
Hello there, nerds! Arsey here. I've always wanted to build and integrate AI into my projects, but the lack of resources made it quite a hassle. However, as the saying goes, "your imagination is the limit!" So, I took up the challenge and successfully built an AI-powered chatbot using Flask, HTML, CSS, and JavaScript—and guess what? I did it using only my smartphone! Impressive, right? Well, this was made possible thanks to Google AI Studio, a platform that allows developers to easily build and integrate Google’s state-of-the-art generative model, Gemini. In this tutorial, I'll guide you step by step on how I built this chatbot so you can do it too. Let’s get coding! Prerequisites Before we begin, I assume you have some basic knowledge of: ✅ Python (especially Flask) ✅ JavaScript, HTML, and CSS If you're comfortable with these, you're good to go! You can also check out the source code for this project on my GitHub. Part 1: Setting Up the Environment Install Python First, ensure you have Python installed. If you haven't installed it yet, download it here and follow the installation steps. Install Required Libraries Now, let's install the necessary dependencies. Run the following command: pip install flask markdown google-generativeai Get Your Google AI Studio API Key Open Google AI Studio in your browser. Sign up or log in. Navigate to the side panel. Click on "Get API Key" and follow the steps to create a new API key. Testing the API Key Let's verify that our API key works by running this simple script: import google.generativeai as genai genai.configure(api_key="YOUR_GEMINI_API_KEY") model = genai.GenerativeModel("gemini-2.0-flash") response = model.generate_content("Explain how AI works") print(response.text) Breaking it Down: ✅ We import the google-generativeai library. ✅ We configure the AI model using genai.configure(api_key="YOUR_GEMINI_API_KEY"). ✅ We choose Gemini 2.0 Flash as our model. ✅ We generate a response using model.generate_content("Explain how AI works"). ✅ Finally, we print the response to see Gemini's answer. If everything is set up correctly, you should see an AI-generated explanation of how AI works!
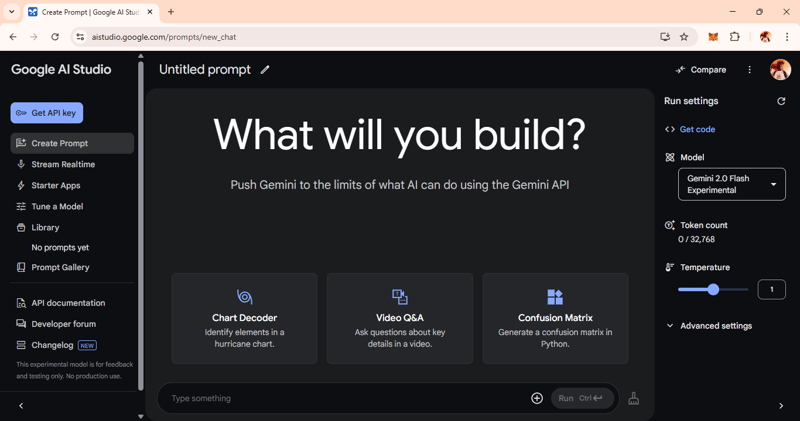
Hello there, nerds! Arsey here.
I've always wanted to build and integrate AI into my projects, but the lack of resources made it quite a hassle. However, as the saying goes, "your imagination is the limit!"
So, I took up the challenge and successfully built an AI-powered chatbot using Flask, HTML, CSS, and JavaScript—and guess what? I did it using only my smartphone! Impressive, right? Well, this was made possible thanks to Google AI Studio, a platform that allows developers to easily build and integrate Google’s state-of-the-art generative model, Gemini.
In this tutorial, I'll guide you step by step on how I built this chatbot so you can do it too. Let’s get coding!
Prerequisites
Before we begin, I assume you have some basic knowledge of:
✅ Python (especially Flask)
✅ JavaScript, HTML, and CSS
If you're comfortable with these, you're good to go! You can also check out the source code for this project on my GitHub.
Part 1: Setting Up the Environment
Install Python
First, ensure you have Python installed. If you haven't installed it yet, download it here and follow the installation steps.Install Required Libraries
Now, let's install the necessary dependencies. Run the following command:
pip install flask markdown google-generativeai
- Get Your Google AI Studio API Key Open Google AI Studio in your browser. Sign up or log in.
Navigate to the side panel.
Click on "Get API Key" and follow the steps to create a new API key.
Testing the API Key
Let's verify that our API key works by running this simple script:
import google.generativeai as genai
genai.configure(api_key="YOUR_GEMINI_API_KEY")
model = genai.GenerativeModel("gemini-2.0-flash")
response = model.generate_content("Explain how AI works")
print(response.text)
Breaking it Down:
✅ We import the google-generativeai library.
✅ We configure the AI model using genai.configure(api_key="YOUR_GEMINI_API_KEY")
.
✅ We choose Gemini 2.0 Flash as our model.
✅ We generate a response using model.generate_content("Explain how AI works")
.
✅ Finally, we print the response to see Gemini's answer.
If everything is set up correctly, you should see an AI-generated explanation of how AI works!