Handling class specific behavior: polymorphism vs instanceof
I'm designing a system with different types of components, and I'm trying to decide whether to use polymorphism or instanceof checks for handling type-specific behavior. I see two possible approaches: Polymorphism Approach – Define all possible methods in a base class (even if only some subclasses override them). instanceof Approach – Only define relevant methods in specific subclasses and check types before calling them. Example with polymorphism abstract class Component { // Default implementations (may do nothing) void specialAction() {} } class GeneralComponent extends Component { // Doesn't need to override specialAction() } class SpecialComponent extends Component { @Override void specialAction() { System.out.println("Special action executed!"); } } // Usage void handleComponent(Component component) { component.specialAction(); // Will only do something if overridden } Example with instanceof In this approach, methods exist only in the relevant subclasses, and we check the type before calling them. abstract class Component {} class GeneralComponent extends Component {} class SpecialComponent extends Component { void specialAction() { System.out.println("Special action executed!"); } } // Usage void handleComponent(Component component) { if (component instanceof SpecialComponent) { ((SpecialComponent) component).specialAction(); } } I understand that excessive use of instanceof is generally discouraged, but adding methods to the base class that aren't shared among all the subclasses doesn't seems to be a good design choice either.
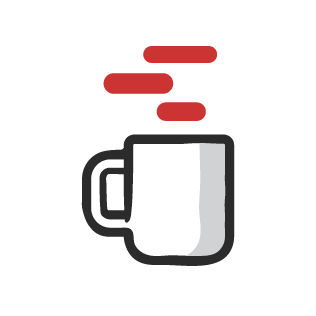
I'm designing a system with different types of components, and I'm trying to decide whether to use polymorphism or instanceof checks for handling type-specific behavior.
I see two possible approaches:
- Polymorphism Approach – Define all possible methods in a base class (even if only some subclasses override them).
- instanceof Approach – Only define relevant methods in specific subclasses and check types before calling them.
Example with polymorphism
abstract class Component {
// Default implementations (may do nothing)
void specialAction() {}
}
class GeneralComponent extends Component {
// Doesn't need to override specialAction()
}
class SpecialComponent extends Component {
@Override
void specialAction() {
System.out.println("Special action executed!");
}
}
// Usage
void handleComponent(Component component) {
component.specialAction(); // Will only do something if overridden
}
Example with instanceof
In this approach, methods exist only in the relevant subclasses, and we check the type before calling them.
abstract class Component {}
class GeneralComponent extends Component {}
class SpecialComponent extends Component {
void specialAction() {
System.out.println("Special action executed!");
}
}
// Usage
void handleComponent(Component component) {
if (component instanceof SpecialComponent) {
((SpecialComponent) component).specialAction();
}
}
I understand that excessive use of instanceof is generally discouraged, but adding methods to the base class that aren't shared among all the subclasses doesn't seems to be a good design choice either.