15 Must-Know Hoisting Questions to Crush Your Next Interview
(** to Catapult You to Senior JavaScript Developer **) ✅1.What is hoisting in JavaScript, and how does it affect variable and function declarations? ✅2.How does hoisting differ between var, let, and const? ✅3.Why does this code log undefined instead of throwing an error? ➡️Code: console.log(x); var x = 42; ✅4.What happens when you try to access a let variable before its declaration? ✅5.Explain why this function call works before the function is defined. ➡️Code: greet(); // "Hello!" function greet() { console.log("Hello!"); } ✅6.Why does this function expression throw an error? ➡️Code: callMe(); // TypeError var callMe = function() { console.log("Hey!"); }; ✅7.What’s the output of this code involving variable shadowing? ➡️Code: var name = "Alice"; function greet() { console.log(name); // undefined var name = "Bob"; } greet(); ✅8.Why does this code prioritize the function over the variable? ➡️Code: console.log(myFunc); // [Function: myFunc] var myFunc = "I’m a string!"; function myFunc() { console.log("I’m a function!"); } ✅9.What happens when you redeclare a variable with var in the same scope? ✅10.Why does this loop with var cause unexpected output? ➡️Code: for (var i = 0; i < 3; i++) { setTimeout(() => console.log(i), 1000); } // Outputs: 3, 3, 3 ✅11.How does hoisting behave in a function’s block scope? ✅12.What’s the output of this code with multiple declarations? ➡️Code: var x = 1; function x() {} console.log(x); // 1 ✅13.Why does this IIFE behave unexpectedly with var? ➡️Code: var x = 10; (function() { console.log(x); // undefined var x = 20; })(); ✅14.How does hoisting affect arrow functions? ✅15.How can you avoid hoisting-related bugs in production code? "Let’s dive into the answers to these 15 hoisting questions, packed with examples to boost your JavaScript mastery!
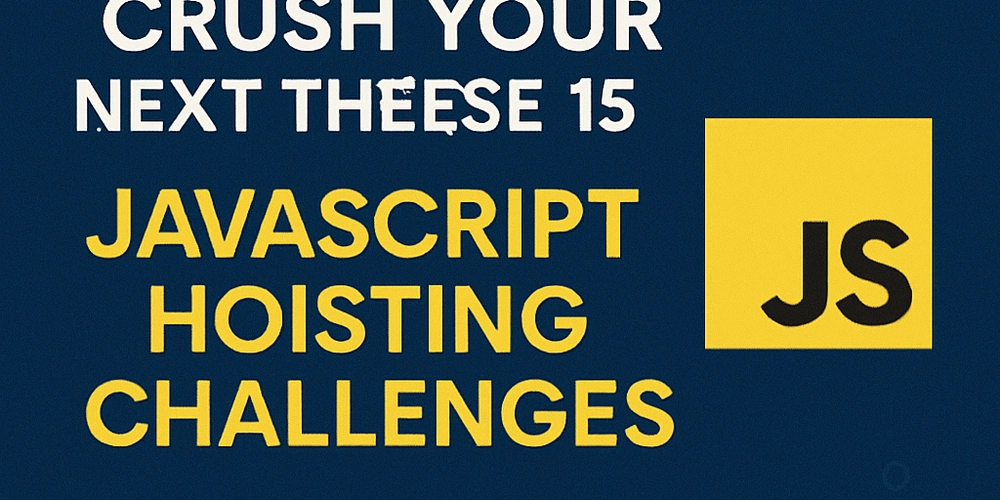
(** to Catapult You to Senior JavaScript Developer **)
✅1.What is hoisting in JavaScript, and how does it affect variable and function declarations?
✅2.How does hoisting differ between var, let, and const?
✅3.Why does this code log undefined instead of throwing an error?
➡️Code:
console.log(x);
var x = 42;
✅4.What happens when you try to access a let variable before its declaration?
✅5.Explain why this function call works before the function is defined.
➡️Code:
greet(); // "Hello!"
function greet() {
console.log("Hello!");
}
✅6.Why does this function expression throw an error?
➡️Code:
callMe(); // TypeError
var callMe = function() {
console.log("Hey!");
};
✅7.What’s the output of this code involving variable shadowing?
➡️Code:
var name = "Alice";
function greet() {
console.log(name); // undefined
var name = "Bob";
}
greet();
✅8.Why does this code prioritize the function over the variable?
➡️Code:
console.log(myFunc); // [Function: myFunc]
var myFunc = "I’m a string!";
function myFunc() {
console.log("I’m a function!");
}
✅9.What happens when you redeclare a variable with var in the same scope?
✅10.Why does this loop with var cause unexpected output?
➡️Code:
for (var i = 0; i < 3; i++) {
setTimeout(() => console.log(i), 1000);
}
// Outputs: 3, 3, 3
✅11.How does hoisting behave in a function’s block scope?
✅12.What’s the output of this code with multiple declarations?
➡️Code:
var x = 1;
function x() {}
console.log(x); // 1
✅13.Why does this IIFE behave unexpectedly with var?
➡️Code:
var x = 10;
(function() {
console.log(x); // undefined
var x = 20;
})();
✅14.How does hoisting affect arrow functions?
✅15.How can you avoid hoisting-related bugs in production code?
"Let’s dive into the answers to these 15 hoisting questions, packed with examples to boost your JavaScript mastery!