day-31: this, super and inner class in java
The "this" Keyword in Java The this keyword in Java is a reference variable that refers to the current object of a class. It has several important uses: Main Uses of this: 1). To refer to current class instance variables (to resolve ambiguity between instance variables and parameters) public class Student { String name; public Student(String name) { this.name = name; // 'this.name' refers to instance variable } } 2). To invoke current class constructor (constructor chaining) public class Rectangle { int width, height; public Rectangle() { this(10, 10); // calls parameterized constructor } public Rectangle(int width, int height) { this.width = width; this.height = height; } } Important Notes a). this cannot be used in a static context (static methods or static blocks) b). this is automatically added by the compiler in many cases (like when calling instance methods) c). It helps make code more readable and resolves naming conflicts d). In event handling or anonymous classes, this can refer to different objects, so you might need to qualify it with the class name (e.g., ClassName.this) Understanding this is fundamental to proper object-oriented programming in Java. The "super" Keyword in Java: The super keyword in Java is a reference variable that refers to the immediate parent class object. It's used to access parent class members (fields, methods, and constructors) from a subclass. Main Uses of super 1). Accessing Parent Class Variables Used when child and parent classes have fields with the same name: class Parent { String color = "white"; } class Child extends Parent { String color = "black"; void printColor() { System.out.println(super.color); // prints "white" (parent) System.out.println(color); // prints "black" (child) } } 2). Calling Parent Class Methods Used to call overridden methods from the parent class: class Parent { void display() { System.out.println("Parent method"); } } class Child extends Parent { void display() { super.display(); // calls parent's display() System.out.println("Child method"); } } Calling Parent Class Constructors Used to invoke parent class constructor (must be the first statement in child constructor): class Parent { Parent() { System.out.println("Parent constructor"); } } class Child extends Parent { Child() { super(); // calls parent constructor (implicit if omitted) System.out.println("Child constructor"); } } Important Notes About super super() must be the first statement in a constructor if used If you don't write super(), the compiler automatically inserts a call to the no-arg parent constructor You can pass parameters to super() to call specific parent constructors: super(parameter1, parameter2); super cannot be used in static contexts (static methods or blocks) Unlike this, super is not a reference to an object - it's a keyword for special compiler handling super vs this Feature super this Reference Immediate parent class Current class instance Constructor Calls parent constructor Calls current class constructor Variables Accesses parent class variables Accesses current class variables Methods Calls overridden parent methods Calls current class methods Understanding super is essential for proper inheritance and method overriding in Java. Inner Class in Java - Simple Explanation An inner class is a class defined inside another class. It helps organize related classes together and can access all members (even private) of the outer class. Why Use Inner Classes? Better Encapsulation: Hide helper classes inside another class. Access Outer Class Private Members: Inner classes can access private fields/methods of the outer class. Cleaner Code: Group related classes logically. Key Points ✔ Inner classes can be private, protected, or public. ✔ Non-static inner classes cannot have static members (except static final constants). ✔ Static nested classes can have static members. Example in Real Life Think of a Car (Outer Class) having an Engine (Inner Class). The Engine is part of the Car and can access its private components, but it doesn’t make sense to use the Engine outside the Car. class Car { private String model = "Tesla"; class Engine { void start() { System.out.println(model + "'s engine started!"); // Can access private model } } } Conclusion Inner classes help in organizing code logically and provide better encapsulation. Use them when a class is only meaningful inside another class!
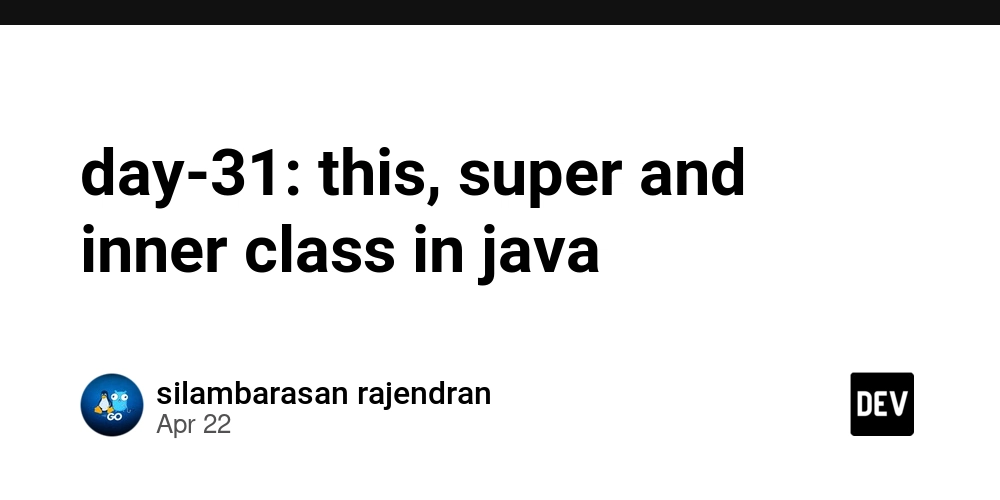
The "this" Keyword in Java
The this keyword in Java is a reference variable that refers to the current object of a class.
It has several important uses:
Main Uses of this:
1). To refer to current class instance variables (to resolve ambiguity between instance variables and parameters)
public class Student {
String name;
public Student(String name) {
this.name = name; // 'this.name' refers to instance variable
}
}
2). To invoke current class constructor (constructor chaining)
public class Rectangle {
int width, height;
public Rectangle() {
this(10, 10); // calls parameterized constructor
}
public Rectangle(int width, int height) {
this.width = width;
this.height = height;
}
}
Important Notes
a). this cannot be used in a static context (static methods or static blocks)
b). this is automatically added by the compiler in many cases (like when calling instance methods)
c). It helps make code more readable and resolves naming conflicts
d). In event handling or anonymous classes, this can refer to different objects, so you might need to qualify it with the class name (e.g., ClassName.this)
Understanding this is fundamental to proper object-oriented programming in Java.
The "super" Keyword in Java:
The super keyword in Java is a reference variable that refers to the immediate parent class object. It's used to access parent class members (fields, methods, and constructors) from a subclass.
Main Uses of super
1). Accessing Parent Class Variables
Used when child and parent classes have fields with the same name:
class Parent {
String color = "white";
}
class Child extends Parent {
String color = "black";
void printColor() {
System.out.println(super.color); // prints "white" (parent)
System.out.println(color); // prints "black" (child)
}
}
2). Calling Parent Class Methods
Used to call overridden methods from the parent class:
class Parent {
void display() {
System.out.println("Parent method");
}
}
class Child extends Parent {
void display() {
super.display(); // calls parent's display()
System.out.println("Child method");
}
}
- Calling Parent Class Constructors
Used to invoke parent class constructor (must be the first statement in child constructor):
class Parent {
Parent() {
System.out.println("Parent constructor");
}
}
class Child extends Parent {
Child() {
super(); // calls parent constructor (implicit if omitted)
System.out.println("Child constructor");
}
}
Important Notes About super
super() must be the first statement in a constructor if used
If you don't write super(), the compiler automatically inserts a call to the no-arg parent constructor
You can pass parameters to super() to call specific parent constructors:
super(parameter1, parameter2);
super cannot be used in static contexts (static methods or blocks)
Unlike this, super is not a reference to an object - it's a keyword for special compiler handling
super vs this
Feature super this
Reference Immediate parent class Current class instance
Constructor Calls parent constructor Calls current class constructor
Variables Accesses parent class variables Accesses current class variables
Methods Calls overridden parent methods Calls current class methods
Understanding super is essential for proper inheritance and method overriding in Java.
Inner Class in Java - Simple Explanation
An inner class is a class defined inside another class. It helps organize related classes together and can access all members (even private) of the outer class.
Why Use Inner Classes?
Better Encapsulation: Hide helper classes inside another class.
Access Outer Class Private Members: Inner classes can access private fields/methods of the outer class.
Cleaner Code: Group related classes logically.
Key Points
✔ Inner classes can be private, protected, or public.
✔ Non-static inner classes cannot have static members (except static final constants).
✔ Static nested classes can have static members.
Example in Real Life
Think of a Car (Outer Class) having an Engine (Inner Class). The Engine is part of the Car and can access its private components, but it doesn’t make sense to use the Engine outside the Car.
class Car {
private String model = "Tesla";
class Engine {
void start() {
System.out.println(model + "'s engine started!"); // Can access private model
}
}
}
Conclusion
Inner classes help in organizing code logically and provide better encapsulation. Use them when a class is only meaningful inside another class!