Must getters return values as is?
We have entities with numeric properties, they are of boxed types (e.g. Integer). Typically, each of those properties has a pair of getters: getInt(), which returns zero on null values, and getIntNullable(), which returns values as is. // we have a bazillion entities like this one public class SomeEntity { public Integer someInt; /** * @deprecated Maps nulls to zero */ public int getSomeInt() { return someInt == null ? 0 : someInt; } public Integer getSomeIntNullable() { return someInt; } } getInt() type of methods are marked @Deprecated. I guess it's because there were some bugs related to that in the past. It's a mature project. But getInt() methods come in handy in some cases: for example, when we need to pass zero for newly persisted entities. It makes no apparent sense to call getIntNullable() and then map it to zero if we can get zero directly from the instance right away (for example, using Optional.ofNullable(nullableInt).orElse(0)). Besides, replacing getInt() with getIntNullable() may cause (and did cause) NPEs on unboxing. Those kinds of issues can sometimes be tricky to spot and may not immediately emerge. But any time we use those deprecated methods, we get shamed by the IDE. The only reason I see for those methods to be deprecated is getters, I believe, are supposed to return values as is. Including any mapping logic in them feels kind of wrong. That argument may be a bit too theoretical, though. One solution is to rename getIntNullable() to getInt() and to rename getInt() to getIntOrZero(), removing deprecation. It's going to be a ton of work (on the other hand, it's going to be a ton of work either way, if we are to get it right). Also, may be a bit confusing to those used to old naming patterns. What's your advice? Can getters perform that sort of mapping — or should it be a taboo in software engineering?
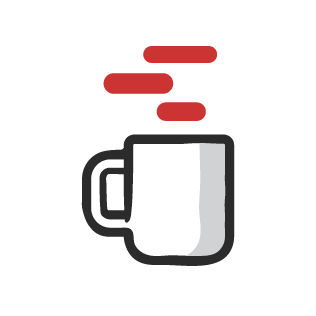
We have entities with numeric properties, they are of boxed types (e.g. Integer
). Typically, each of those properties has a pair of getters: getInt()
, which returns zero on null
values, and getIntNullable()
, which returns values as is.
// we have a bazillion entities like this one
public class SomeEntity {
public Integer someInt;
/**
* @deprecated Maps nulls to zero
*/
public int getSomeInt() {
return someInt == null ? 0 : someInt;
}
public Integer getSomeIntNullable() {
return someInt;
}
}
getInt()
type of methods are marked @Deprecated
. I guess it's because there were some bugs related to that in the past. It's a mature project. But getInt()
methods come in handy in some cases: for example, when we need to pass zero for newly persisted entities. It makes no apparent sense to call getIntNullable()
and then map it to zero if we can get zero directly from the instance right away (for example, using Optional.ofNullable(nullableInt).orElse(0)
). Besides, replacing getInt()
with getIntNullable()
may cause (and did cause) NPEs on unboxing. Those kinds of issues can sometimes be tricky to spot and may not immediately emerge. But any time we use those deprecated methods, we get shamed by the IDE.
The only reason I see for those methods to be deprecated is getters, I believe, are supposed to return values as is. Including any mapping logic in them feels kind of wrong. That argument may be a bit too theoretical, though.
One solution is to rename getIntNullable()
to getInt()
and to rename getInt()
to getIntOrZero()
, removing deprecation. It's going to be a ton of work (on the other hand, it's going to be a ton of work either way, if we are to get it right). Also, may be a bit confusing to those used to old naming patterns.
What's your advice? Can getters perform that sort of mapping — or should it be a taboo in software engineering?