How to Integrate AI into Your Web App in 2025 (Without Losing Your Mind)
AI is everywhere in 2025 — chatbots, smart replies, content generators. But did you know it’s actually super easy to add AI to your own web app? In this post, I’ll show you how to use OpenAI’s GPT model in a web app to summarize text — like blog posts, notes, or articles. No need to be an AI expert. Just some basic web dev skills and an API key! What We’re Building A simple app where users can: Paste some text Click a button Get a smart summary from AI What You’ll Need • Python (we’ll use Flask for the backend) • OpenAI API key (you can get it from platform.openai.com) # app.py from flask import Flask, request, jsonify import openai import os app = Flask(__name__) openai.api_key = os.getenv("OPENAI_API_KEY") @app.route('/summarize', methods=['POST']) def summarize(): data = request.get_json() text = data.get("text", "") if not text: return jsonify({"error": "No text provided"}), 400 response = openai.ChatCompletion.create( model="gpt-4", messages=[ {"role": "system", "content": "You are a helpful assistant that summarizes text."}, {"role": "user", "content": text} ] ) summary = response['choices'][0]['message']['content'] return jsonify({"summary": summary}) if __name__ == '__main__': app.run(debug=True) Make sure to set your OpenAI key: export OPENAI_API_KEY=your_key_here That’s It! You now have a basic web app that uses AI to summarize text.
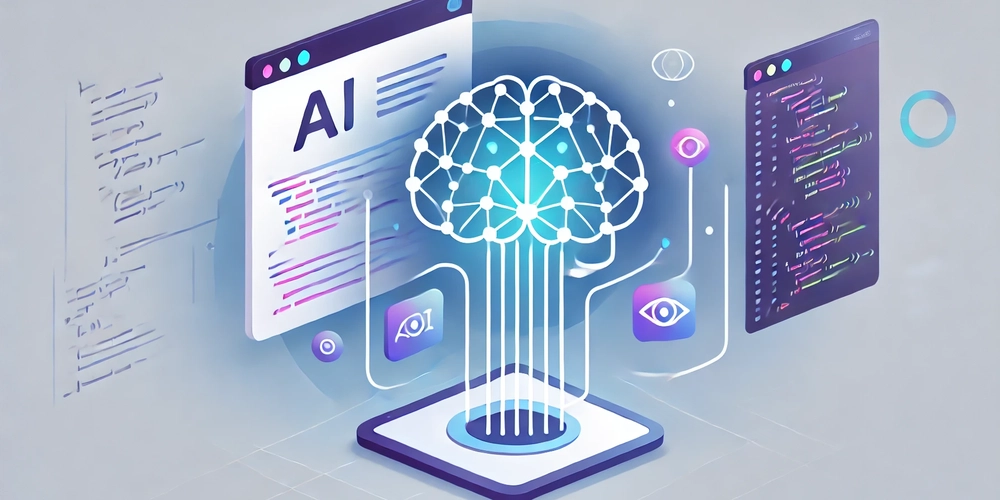
AI is everywhere in 2025 — chatbots, smart replies, content generators. But did you know it’s actually super easy to add AI to your own web app?
In this post, I’ll show you how to use OpenAI’s GPT model in a web app to summarize text — like blog posts, notes, or articles. No need to be an AI expert. Just some basic web dev skills and an API key!
What We’re Building
A simple app where users can:
- Paste some text
- Click a button
- Get a smart summary from AI
What You’ll Need
• Python (we’ll use Flask for the backend)
• OpenAI API key (you can get it from platform.openai.com)
# app.py
from flask import Flask, request, jsonify
import openai
import os
app = Flask(__name__)
openai.api_key = os.getenv("OPENAI_API_KEY")
@app.route('/summarize', methods=['POST'])
def summarize():
data = request.get_json()
text = data.get("text", "")
if not text:
return jsonify({"error": "No text provided"}), 400
response = openai.ChatCompletion.create(
model="gpt-4",
messages=[
{"role": "system", "content": "You are a helpful assistant that summarizes text."},
{"role": "user", "content": text}
]
)
summary = response['choices'][0]['message']['content']
return jsonify({"summary": summary})
if __name__ == '__main__':
app.run(debug=True)
Make sure to set your OpenAI key:
export OPENAI_API_KEY=your_key_here
That’s It!
You now have a basic web app that uses AI to summarize text.