Interface Segregation Principle (ISP) in MuleSoft: Specific Interfaces for Specific Needs
What is ISP? The Interface Segregation Principle (ISP) states that no client should be forced to depend on methods or interfaces it does not use. In other words, it is better to have several small and specific interfaces than one large and generic interface. In the context of MuleSoft, ISP can be applied by designing APIs and flows that segregate responsibilities into different layers or endpoints, ensuring that each consumer uses only what they truly need. Why Is ISP Important for MuleSoft? Imagine our order system has a monolithic API with multiple endpoints related to orders, inventory, and payments. If an API consumer only needs data for a specific order, they would still be forced to deal with endpoints or parameters related to inventory or payments—violating ISP. This approach can lead to: Unnecessary complexity: Consumers need to understand parts of the API that are irrelevant to them. Low reusability: Generic APIs become difficult to adapt for new consumers. High maintenance costs: Changes in one part of the API affect all consumers. By applying ISP, we can design APIs and flows that are more specific and tailored to consumers' needs. How to Apply ISP in the Order System? 1. Divide APIs into Layers Using API-Led Connectivity MuleSoft promotes the use of the API-Led Connectivity pattern, which divides APIs into three main layers: System APIs: Interfaces that expose raw data directly from underlying systems (e.g., ERP, CRM). Process APIs: Interfaces that aggregate and transform data between systems. Experience APIs: Interfaces formatted for end consumers (e.g., mobile or web applications). This approach ensures that each consumer uses only the relevant interfaces for their needs. Practical Example – API Layers: Layer Purpose Example Endpoint System API Expose raw ERP data /erp/orders Process API Aggregate order and inventory data /process/orders-with-stock Experience API Formatted data for applications /mobile/orders 2. Create APIs Specific to Consumers Instead of creating a single generic API for all consumers, divide endpoints based on specific needs. Practical Example – Before (violating ISP): #%RAML 1.0 title: Order API version: v1 /resources: /orders: get: description: Returns orders with inventory and payment information. responses: 200: body: application/json: type: object properties: orderId: string items: array stockAvailability: boolean paymentStatus: string In this case, all API consumers are forced to deal with inventory and payment information even if they only need basic order details. Practical Example – After (applying ISP): #%RAML 1.0 title: Order API version: v1 /resources: /orders: get: description: Returns only basic order details. responses: 200: body: application/json: type: object properties: orderId: string items: array /orders/stock: get: description: Returns stock availability for order items. responses: 200: body: application/json: type: object properties: orderId: string stockAvailability: boolean /orders/payment-status: get: description: Returns payment status for an order. responses: 200: body: application/json: type: object properties: orderId: string paymentStatus: string Now, each consumer can access only the relevant endpoints for their needs. 3. Modularize Flows with Dedicated Subflows Similar to SRP, modularizing flows into dedicated subflows also helps apply ISP. Each subflow can be designed to handle a specific responsibility. Practical Example – Modularized Flow by Responsibility Benefits of ISP in the Order System Simplicity: Consumers access only the information relevant to their needs. Reusability: Specific APIs are easier to adapt for new use cases. Simplified Maintenance: Changes in one part of the API do not affect all consumers. Checklist for Applying ISP in MuleSoft [ ] Have APIs been divided into layers (System, Process, Experience)? [ ] Are endpoints specific to different types of consumers? [ ] Have flows been modularized by responsibility? Next Steps Now that we’ve covered ISP, let’s move on to the final principle in the SOLID series: the Dependency Inversion Principle (DIP) — designing flows that depend on abstractions rather than implementations. ← Previous Principle: LSP | → Next Principle: DIP
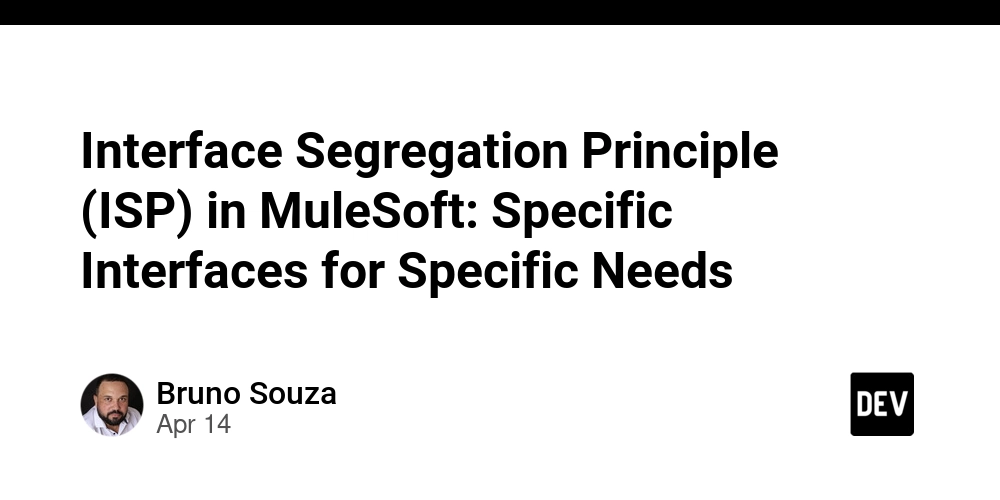
What is ISP?
The Interface Segregation Principle (ISP) states that no client should be forced to depend on methods or interfaces it does not use. In other words, it is better to have several small and specific interfaces than one large and generic interface.
In the context of MuleSoft, ISP can be applied by designing APIs and flows that segregate responsibilities into different layers or endpoints, ensuring that each consumer uses only what they truly need.
Why Is ISP Important for MuleSoft?
Imagine our order system has a monolithic API with multiple endpoints related to orders, inventory, and payments. If an API consumer only needs data for a specific order, they would still be forced to deal with endpoints or parameters related to inventory or payments—violating ISP.
This approach can lead to:
- Unnecessary complexity: Consumers need to understand parts of the API that are irrelevant to them.
- Low reusability: Generic APIs become difficult to adapt for new consumers.
- High maintenance costs: Changes in one part of the API affect all consumers.
By applying ISP, we can design APIs and flows that are more specific and tailored to consumers' needs.
How to Apply ISP in the Order System?
1. Divide APIs into Layers Using API-Led Connectivity
MuleSoft promotes the use of the API-Led Connectivity pattern, which divides APIs into three main layers:
- System APIs: Interfaces that expose raw data directly from underlying systems (e.g., ERP, CRM).
- Process APIs: Interfaces that aggregate and transform data between systems.
- Experience APIs: Interfaces formatted for end consumers (e.g., mobile or web applications).
This approach ensures that each consumer uses only the relevant interfaces for their needs.
Practical Example – API Layers:
Layer | Purpose | Example Endpoint |
---|---|---|
System API | Expose raw ERP data | /erp/orders |
Process API | Aggregate order and inventory data | /process/orders-with-stock |
Experience API | Formatted data for applications | /mobile/orders |
2. Create APIs Specific to Consumers
Instead of creating a single generic API for all consumers, divide endpoints based on specific needs.
Practical Example – Before (violating ISP):
#%RAML 1.0
title: Order API
version: v1
/resources:
/orders:
get:
description: Returns orders with inventory and payment information.
responses:
200:
body:
application/json:
type: object
properties:
orderId: string
items: array
stockAvailability: boolean
paymentStatus: string
In this case, all API consumers are forced to deal with inventory and payment information even if they only need basic order details.
Practical Example – After (applying ISP):
#%RAML 1.0
title: Order API
version: v1
/resources:
/orders:
get:
description: Returns only basic order details.
responses:
200:
body:
application/json:
type: object
properties:
orderId: string
items: array
/orders/stock:
get:
description: Returns stock availability for order items.
responses:
200:
body:
application/json:
type: object
properties:
orderId: string
stockAvailability: boolean
/orders/payment-status:
get:
description: Returns payment status for an order.
responses:
200:
body:
application/json:
type: object
properties:
orderId: string
paymentStatus: string
Now, each consumer can access only the relevant endpoints for their needs.
3. Modularize Flows with Dedicated Subflows
Similar to SRP, modularizing flows into dedicated subflows also helps apply ISP. Each subflow can be designed to handle a specific responsibility.
Practical Example – Modularized Flow by Responsibility
name="processOrder">
path="/orders"/>
name="validateOrder"/>
name="transformOrder"/>
name="validateOrder">
name="transformOrder">
Benefits of ISP in the Order System
- Simplicity: Consumers access only the information relevant to their needs.
- Reusability: Specific APIs are easier to adapt for new use cases.
- Simplified Maintenance: Changes in one part of the API do not affect all consumers.
Checklist for Applying ISP in MuleSoft
- [ ] Have APIs been divided into layers (System, Process, Experience)?
- [ ] Are endpoints specific to different types of consumers?
- [ ] Have flows been modularized by responsibility?
Next Steps
Now that we’ve covered ISP, let’s move on to the final principle in the SOLID series: the Dependency Inversion Principle (DIP) — designing flows that depend on abstractions rather than implementations.
← Previous Principle: LSP | → Next Principle: DIP