Mastering System Design: 24 Key Concepts for Beginners
System design is crucial for software engineers, especially as you grow from a junior to a senior developer. Understanding the fundamental concepts in system design helps you build scalable applications and perform well in technical interviews. In this blog, I’ll walk you through 24 key concepts that are essential for system design, using simple examples to make them easy to grasp. 1. Client-Server Architecture In every web application, there's a client and a server. The client (like a web browser or mobile app) sends requests, and the server responds with the necessary data. Example: When you use Facebook, your phone is the client, and Facebook’s server processes your requests, like fetching new posts or sending messages. 2. DNS (Domain Name System) DNS acts like the phonebook of the internet, translating easy-to-remember domain names (like google.com) into IP addresses, so the client knows where to find the server. Example: When you type google.com, your browser asks a DNS server for the corresponding IP address, then connects to Google’s server. 3. Proxies and Reverse Proxies A proxy server sits between the client and the internet, hiding the client’s real IP address. A reverse proxy directs client requests to the appropriate backend server. Example: If you access a website through a proxy server, the website sees the proxy’s IP, not yours. A reverse proxy like Nginx might send your request to different backend servers, depending on the load. 4. Latency and Geographically Distributed Servers Latency is the delay caused by the distance between the client and the server. To reduce latency, servers can be located closer to users. Example: If you're in India and accessing a website hosted in the US, the data has to travel halfway around the world, which causes a delay. To reduce this, the website might host servers in India for faster responses. 5. HTTP vs. HTTPS HTTP is a protocol used to send data over the web, but it's not secure. HTTPS, on the other hand, encrypts the data to protect it from hackers. Example: When you visit https://bank.com, your data (like login credentials) is encrypted, making it safer than visiting an HTTP website. 6. APIs (Application Programming Interfaces) APIs allow different software systems to communicate with each other. Think of an API like a waiter who takes your order and brings your food. Example: When you use a weather app, it likely uses an API to fetch weather data from a weather server. The app sends a request, and the server sends back the data. 7. REST vs. GraphQL REST and GraphQL are two different ways of handling API requests. REST uses predefined endpoints to fetch data, while GraphQL allows you to request only the data you need. Example: With REST, if you want both a user’s profile and their posts, you might need to make two separate requests. With GraphQL, you can ask for both the profile and posts in a single request. 8. SQL vs. NoSQL Databases SQL databases store data in structured tables, while NoSQL databases handle unstructured data and are more flexible. Example: SQL: A banking system with accounts and transactions uses SQL to store data in a structured way. NoSQL: A social media app with user-generated content like posts, comments, and likes can store the data in NoSQL, as the structure might change frequently. 9. Vertical vs. Horizontal Scaling When your app grows, you need to scale it. Vertical scaling involves upgrading the existing server (e.g., adding more RAM), while horizontal scaling adds more servers to share the load. Example: Vertical Scaling: If your app’s server is slow, you add more RAM to make it faster. Horizontal Scaling: If your app’s traffic increases, you add more servers to handle the extra load. 10. Load Balancers A load balancer distributes traffic evenly across multiple servers to prevent any one server from getting overloaded. Example: Imagine a restaurant with multiple chefs. A waiter (load balancer) decides which chef (server) should take the next order to keep all the chefs busy without overloading one. 11. Database Indexing Indexing makes it faster to search for data in a database, much like using an index in a book to quickly find the information you need. Example: If you have a database of users, indexing by their email addresses will make searching for a user’s email much faster than scanning through every entry. 12. Database Replication Replication creates copies of a database to improve performance and availability. The primary database handles writes, while copies (replicas) handle reads. Example: Imagine a popular website. It stores data in a central database, but for faster access, there are multiple copies of the database spread across different locations for users to access. 13. Sharding Sharding split
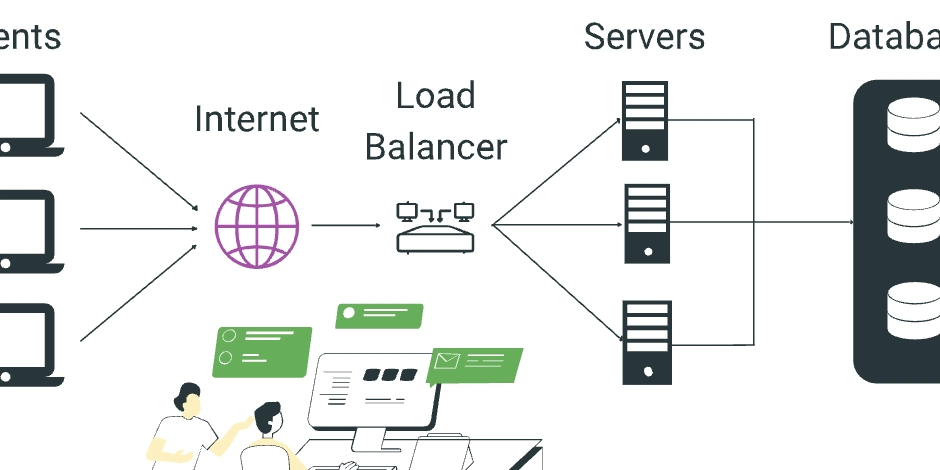
System design is crucial for software engineers, especially as you grow from a junior to a senior developer. Understanding the fundamental concepts in system design helps you build scalable applications and perform well in technical interviews.
In this blog, I’ll walk you through 24 key concepts that are essential for system design, using simple examples to make them easy to grasp.
1. Client-Server Architecture
In every web application, there's a client and a server. The client (like a web browser or mobile app) sends requests, and the server responds with the necessary data.
Example: When you use Facebook, your phone is the client, and Facebook’s server processes your requests, like fetching new posts or sending messages.
2. DNS (Domain Name System)
DNS acts like the phonebook of the internet, translating easy-to-remember domain names (like google.com
) into IP addresses, so the client knows where to find the server.
Example: When you type google.com
, your browser asks a DNS server for the corresponding IP address, then connects to Google’s server.
3. Proxies and Reverse Proxies
A proxy server sits between the client and the internet, hiding the client’s real IP address. A reverse proxy directs client requests to the appropriate backend server.
Example: If you access a website through a proxy server, the website sees the proxy’s IP, not yours. A reverse proxy like Nginx might send your request to different backend servers, depending on the load.
4. Latency and Geographically Distributed Servers
Latency is the delay caused by the distance between the client and the server. To reduce latency, servers can be located closer to users.
Example: If you're in India and accessing a website hosted in the US, the data has to travel halfway around the world, which causes a delay. To reduce this, the website might host servers in India for faster responses.
5. HTTP vs. HTTPS
HTTP is a protocol used to send data over the web, but it's not secure. HTTPS, on the other hand, encrypts the data to protect it from hackers.
Example: When you visit https://bank.com
, your data (like login credentials) is encrypted, making it safer than visiting an HTTP website.
6. APIs (Application Programming Interfaces)
APIs allow different software systems to communicate with each other. Think of an API like a waiter who takes your order and brings your food.
Example: When you use a weather app, it likely uses an API to fetch weather data from a weather server. The app sends a request, and the server sends back the data.
7. REST vs. GraphQL
REST and GraphQL are two different ways of handling API requests. REST uses predefined endpoints to fetch data, while GraphQL allows you to request only the data you need.
Example:
- With REST, if you want both a user’s profile and their posts, you might need to make two separate requests.
- With GraphQL, you can ask for both the profile and posts in a single request.
8. SQL vs. NoSQL Databases
SQL databases store data in structured tables, while NoSQL databases handle unstructured data and are more flexible.
Example:
- SQL: A banking system with accounts and transactions uses SQL to store data in a structured way.
- NoSQL: A social media app with user-generated content like posts, comments, and likes can store the data in NoSQL, as the structure might change frequently.
9. Vertical vs. Horizontal Scaling
When your app grows, you need to scale it. Vertical scaling involves upgrading the existing server (e.g., adding more RAM), while horizontal scaling adds more servers to share the load.
Example:
- Vertical Scaling: If your app’s server is slow, you add more RAM to make it faster.
- Horizontal Scaling: If your app’s traffic increases, you add more servers to handle the extra load.
10. Load Balancers
A load balancer distributes traffic evenly across multiple servers to prevent any one server from getting overloaded.
Example: Imagine a restaurant with multiple chefs. A waiter (load balancer) decides which chef (server) should take the next order to keep all the chefs busy without overloading one.
11. Database Indexing
Indexing makes it faster to search for data in a database, much like using an index in a book to quickly find the information you need.
Example: If you have a database of users, indexing by their email addresses will make searching for a user’s email much faster than scanning through every entry.
12. Database Replication
Replication creates copies of a database to improve performance and availability. The primary database handles writes, while copies (replicas) handle reads.
Example: Imagine a popular website. It stores data in a central database, but for faster access, there are multiple copies of the database spread across different locations for users to access.
13. Sharding
Sharding splits a large database into smaller pieces (called shards) to make it easier to manage and scale.
Example: If you have a huge database of users, you could split it by user IDs: Users with IDs 1–100,000 go to one shard, and users with IDs 100,001–200,000 go to another shard.
14. Caching
Caching stores frequently used data in memory so it can be quickly accessed without needing to fetch it from a database.
Example: When you check the weather on your phone, it might show the cached result for your location if you recently checked, reducing the time it takes to show the data.
15. Denormalization
Denormalization combines related data into a single table to make queries faster, even if it means some data is repeated.
Example: In a shopping website, instead of storing product details in one table and order details in another, you might store both together to quickly retrieve them when a customer makes a purchase.
16. The CAP Theorem
The CAP Theorem explains that in a distributed system, you can’t guarantee all three: Consistency, Availability, and Partition Tolerance.
Example: In a distributed shopping cart system, if a network partition occurs between servers, you may have to choose whether to show the latest cart data (consistency) or ensure the cart is available (availability).
17. Blob Storage and CDN
Blob storage is used for storing large files like images and videos. A Content Delivery Network (CDN) ensures these files load quickly by caching them on servers near the user.
Example: When you watch a video on YouTube, the video file might be stored in blob storage and delivered to you via a CDN, so the video loads quickly no matter where you are.
18. WebSockets for Real-Time Communication
WebSockets allow two-way communication between a client and a server in real time. Once the connection is open, data can flow freely in both directions.
Example: Online multiplayer games use WebSockets to send and receive real-time player movements and game updates without delays.
19. Webhooks
Webhooks allow one server to notify another when something happens, instead of constantly checking for updates.
Example: When a payment is made on your e-commerce site, the payment provider sends a webhook to your server to confirm the transaction.
20. Microservices Architecture
Microservices divide a large application into smaller, independent services, each handling a specific task.
Example: In an online store, one microservice could handle user authentication, another handles payments, and another manages inventory.
21. Message Queues
Message queues allow services to communicate asynchronously, which means they can send messages without waiting for a response.
Example: When a user submits a form, instead of waiting for the form to be processed, the system sends a message to a queue, where it can be processed later without slowing down the rest of the system.
22. Rate Limiting
Rate limiting restricts the number of requests a user can make to an API in a given period, preventing abuse.
Example: If you use a public API to get weather data, rate limiting would prevent you from making too many requests in a short period, which could overload the server.
23. API Gateways
An API Gateway acts as a central point for managing all API requests. It handles tasks like authentication, rate limiting, and routing requests to the appropriate microservice.
Example: If you have a microservices-based app for a movie streaming service, an API Gateway can route the user’s request to the correct service, whether it’s for recommendations, streaming, or user management.
24. Idempotency
Idempotency ensures that multiple identical requests have the same effect as a single request, which is important for preventing duplicate transactions.
Example: If you accidentally click the "Buy Now" button twice, idempotency ensures that you are only charged once for the item.
These 24 concepts are the building blocks of system design. By understanding and mastering them, you’ll be well on your way to designing scalable, efficient, and reliable systems!
Happy learning and designing!