Rust and OpenGL: Building Safe, High-Performance Graphics Applications
As a best-selling author, I invite you to explore my books on Amazon. Don't forget to follow me on Medium and show your support. Thank you! Your support means the world! Graphics programming has always fascinated me. The intersection of technical precision and visual creativity creates a unique programming domain. When I discovered Rust's capabilities for OpenGL development, I found a compelling match between language design and graphics requirements. Rust's safety guarantees elegantly address many challenges in graphics programming while maintaining the performance crucial for real-time rendering. Let me share what I've learned about this powerful combination. Rust and OpenGL: A Natural Partnership Graphics programming demands careful resource management, precise memory handling, and optimal performance—areas where Rust excels. OpenGL, as a mature and widely supported graphics API, pairs naturally with Rust's systems programming capabilities. The relationship between Rust and OpenGL creates a programming environment where performance-critical code can run safely without the typical trade-offs found in other languages. This is particularly valuable in graphics applications where bugs can be difficult to trace and diagnose. Setting Up Rust for OpenGL Development Starting with OpenGL in Rust requires several foundational crates. The main binding libraries include: // Cargo.toml dependencies [dependencies] gl = "0.14.0" // Raw OpenGL bindings glutin = "0.28.0" // OpenGL context creation nalgebra = "0.31.0" // Math library for graphics image = "0.24.5" // Texture loading The windowing and context setup typically follows this pattern: use glutin::{event_loop::{EventLoop, ControlFlow}, window::WindowBuilder, ContextBuilder}; fn main() { let event_loop = EventLoop::new(); let window_builder = WindowBuilder::new() .with_title("My Rust OpenGL App") .with_inner_size(glutin::dpi::LogicalSize::new(800.0, 600.0)); let windowed_context = ContextBuilder::new() .with_vsync(true) .build_windowed(window_builder, &event_loop) .expect("Failed to create OpenGL context"); let windowed_context = unsafe { windowed_context.make_current().expect("Failed to make context current") }; // Load OpenGL function pointers gl::load_with(|s| windowed_context.get_proc_address(s) as *const _); // OpenGL setup code here... // Main event loop event_loop.run(move |event, _, control_flow| { // Handle events and rendering... }); } This setup creates a window, establishes an OpenGL context, and loads the OpenGL functions—all essential steps before any rendering can occur. Memory Safety in Graphics Programming Graphics programming traditionally suffers from numerous memory-related pitfalls. Rust's ownership system helps prevent these issues through compile-time guarantees. For example, when managing OpenGL buffer objects: struct VertexBuffer { id: gl::types::GLuint, } impl VertexBuffer { fn new(vertices: &[f32]) -> Self { let mut vbo = 0; unsafe { gl::GenBuffers(1, &mut vbo); gl::BindBuffer(gl::ARRAY_BUFFER, vbo); gl::BufferData( gl::ARRAY_BUFFER, (vertices.len() * std::mem::size_of::()) as gl::types::GLsizeiptr, vertices.as_ptr() as *const _, gl::STATIC_DRAW ); } VertexBuffer { id: vbo } } fn bind(&self) { unsafe { gl::BindBuffer(gl::ARRAY_BUFFER, self.id); } } } impl Drop for VertexBuffer { fn drop(&mut self) { unsafe { gl::DeleteBuffers(1, &self.id); } } } Here, Rust's Drop trait ensures GPU resources are freed when the buffer goes out of scope. This prevents both leaks and use-after-free errors that plague graphics code in other languages. Shader Management in Rust Shader programs form the core of modern OpenGL applications. Rust helps make shader management more robust: struct ShaderProgram { id: gl::types::GLuint, } impl ShaderProgram { fn new(vertex_src: &str, fragment_src: &str) -> Result { let vertex_shader = compile_shader(vertex_src, gl::VERTEX_SHADER)?; let fragment_shader = compile_shader(fragment_src, gl::FRAGMENT_SHADER)?; let program_id = unsafe { gl::CreateProgram() }; unsafe { gl::AttachShader(program_id, vertex_shader); gl::AttachShader(program_id, fragment_shader); gl::LinkProgram(program_id); // Check for linking errors let mut success = 0; gl::GetProgramiv(program_id, gl::LINK_STATUS, &mut success); if success == 0 { let mut log_length = 0; gl::GetProgramiv(program_id, gl::INFO_LOG_LENGTH, &mut log_length); let mut lo
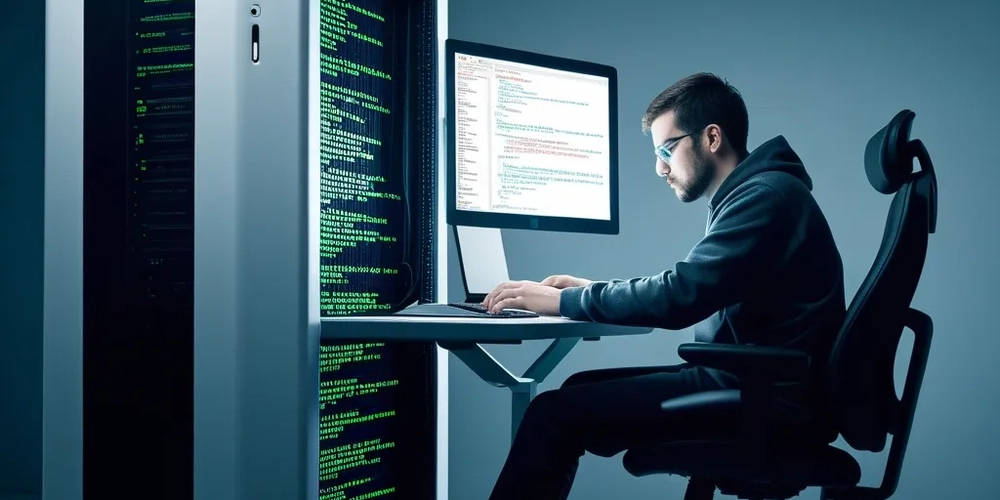
As a best-selling author, I invite you to explore my books on Amazon. Don't forget to follow me on Medium and show your support. Thank you! Your support means the world!
Graphics programming has always fascinated me. The intersection of technical precision and visual creativity creates a unique programming domain. When I discovered Rust's capabilities for OpenGL development, I found a compelling match between language design and graphics requirements.
Rust's safety guarantees elegantly address many challenges in graphics programming while maintaining the performance crucial for real-time rendering. Let me share what I've learned about this powerful combination.
Rust and OpenGL: A Natural Partnership
Graphics programming demands careful resource management, precise memory handling, and optimal performance—areas where Rust excels. OpenGL, as a mature and widely supported graphics API, pairs naturally with Rust's systems programming capabilities.
The relationship between Rust and OpenGL creates a programming environment where performance-critical code can run safely without the typical trade-offs found in other languages. This is particularly valuable in graphics applications where bugs can be difficult to trace and diagnose.
Setting Up Rust for OpenGL Development
Starting with OpenGL in Rust requires several foundational crates. The main binding libraries include:
// Cargo.toml dependencies
[dependencies]
gl = "0.14.0" // Raw OpenGL bindings
glutin = "0.28.0" // OpenGL context creation
nalgebra = "0.31.0" // Math library for graphics
image = "0.24.5" // Texture loading
The windowing and context setup typically follows this pattern:
use glutin::{event_loop::{EventLoop, ControlFlow}, window::WindowBuilder, ContextBuilder};
fn main() {
let event_loop = EventLoop::new();
let window_builder = WindowBuilder::new()
.with_title("My Rust OpenGL App")
.with_inner_size(glutin::dpi::LogicalSize::new(800.0, 600.0));
let windowed_context = ContextBuilder::new()
.with_vsync(true)
.build_windowed(window_builder, &event_loop)
.expect("Failed to create OpenGL context");
let windowed_context = unsafe {
windowed_context.make_current().expect("Failed to make context current")
};
// Load OpenGL function pointers
gl::load_with(|s| windowed_context.get_proc_address(s) as *const _);
// OpenGL setup code here...
// Main event loop
event_loop.run(move |event, _, control_flow| {
// Handle events and rendering...
});
}
This setup creates a window, establishes an OpenGL context, and loads the OpenGL functions—all essential steps before any rendering can occur.
Memory Safety in Graphics Programming
Graphics programming traditionally suffers from numerous memory-related pitfalls. Rust's ownership system helps prevent these issues through compile-time guarantees.
For example, when managing OpenGL buffer objects:
struct VertexBuffer {
id: gl::types::GLuint,
}
impl VertexBuffer {
fn new(vertices: &[f32]) -> Self {
let mut vbo = 0;
unsafe {
gl::GenBuffers(1, &mut vbo);
gl::BindBuffer(gl::ARRAY_BUFFER, vbo);
gl::BufferData(
gl::ARRAY_BUFFER,
(vertices.len() * std::mem::size_of::<f32>()) as gl::types::GLsizeiptr,
vertices.as_ptr() as *const _,
gl::STATIC_DRAW
);
}
VertexBuffer { id: vbo }
}
fn bind(&self) {
unsafe {
gl::BindBuffer(gl::ARRAY_BUFFER, self.id);
}
}
}
impl Drop for VertexBuffer {
fn drop(&mut self) {
unsafe {
gl::DeleteBuffers(1, &self.id);
}
}
}
Here, Rust's Drop
trait ensures GPU resources are freed when the buffer goes out of scope. This prevents both leaks and use-after-free errors that plague graphics code in other languages.
Shader Management in Rust
Shader programs form the core of modern OpenGL applications. Rust helps make shader management more robust:
struct ShaderProgram {
id: gl::types::GLuint,
}
impl ShaderProgram {
fn new(vertex_src: &str, fragment_src: &str) -> Result<Self, String> {
let vertex_shader = compile_shader(vertex_src, gl::VERTEX_SHADER)?;
let fragment_shader = compile_shader(fragment_src, gl::FRAGMENT_SHADER)?;
let program_id = unsafe { gl::CreateProgram() };
unsafe {
gl::AttachShader(program_id, vertex_shader);
gl::AttachShader(program_id, fragment_shader);
gl::LinkProgram(program_id);
// Check for linking errors
let mut success = 0;
gl::GetProgramiv(program_id, gl::LINK_STATUS, &mut success);
if success == 0 {
let mut log_length = 0;
gl::GetProgramiv(program_id, gl::INFO_LOG_LENGTH, &mut log_length);
let mut log = vec![0u8; log_length as usize];
gl::GetProgramInfoLog(
program_id,
log_length,
std::ptr::null_mut(),
log.as_mut_ptr() as *mut gl::types::GLchar
);
let error_message = String::from_utf8_lossy(&log).to_string();
gl::DeleteProgram(program_id);
gl::DeleteShader(vertex_shader);
gl::DeleteShader(fragment_shader);
return Err(error_message);
}
// Clean up shader objects
gl::DeleteShader(vertex_shader);
gl::DeleteShader(fragment_shader);
}
Ok(ShaderProgram { id: program_id })
}
fn use_program(&self) {
unsafe {
gl::UseProgram(self.id);
}
}
fn set_uniform_4f(&self, name: &str, v0: f32, v1: f32, v2: f32, v3: f32) {
let c_name = std::ffi::CString::new(name).unwrap();
unsafe {
let location = gl::GetUniformLocation(self.id, c_name.as_ptr());
gl::Uniform4f(location, v0, v1, v2, v3);
}
}
}
impl Drop for ShaderProgram {
fn drop(&mut self) {
unsafe {
gl::DeleteProgram(self.id);
}
}
}
fn compile_shader(source: &str, shader_type: gl::types::GLenum) -> Result<gl::types::GLuint, String> {
let shader = unsafe { gl::CreateShader(shader_type) };
let c_source = std::ffi::CString::new(source).unwrap();
unsafe {
gl::ShaderSource(shader, 1, &c_source.as_ptr(), std::ptr::null());
gl::CompileShader(shader);
let mut success = 0;
gl::GetShaderiv(shader, gl::COMPILE_STATUS, &mut success);
if success == 0 {
let mut log_length = 0;
gl::GetShaderiv(shader, gl::INFO_LOG_LENGTH, &mut log_length);
let mut log = vec![0u8; log_length as usize];
gl::GetShaderInfoLog(
shader,
log_length,
std::ptr::null_mut(),
log.as_mut_ptr() as *mut gl::types::GLchar
);
let error_message = String::from_utf8_lossy(&log).to_string();
gl::DeleteShader(shader);
return Err(error_message);
}
}
Ok(shader)
}
This approach returns explicit Result
types for compilation and linking errors, making error handling clear and mandatory. The shader program's resources are automatically cleaned up when it goes out of scope.
Complete Rendering Pipeline Example
Let's examine a more complete example that demonstrates texture loading and rendering a textured quad:
fn main() {
// Window setup code (as shown earlier)...
// Vertex data for a textured quad (positions, texture coordinates)
let vertices: [f32; 20] = [
// positions // texture coords
0.5, 0.5, 0.0, 1.0, 1.0, // top right
0.5, -0.5, 0.0, 1.0, 0.0, // bottom right
-0.5, -0.5, 0.0, 0.0, 0.0, // bottom left
-0.5, 0.5, 0.0, 0.0, 1.0 // top left
];
let indices: [u32; 6] = [
0, 1, 3, // first triangle
1, 2, 3 // second triangle
];
// Vertex array object
let mut vao = 0;
// Vertex buffer object
let mut vbo = 0;
// Element buffer object
let mut ebo = 0;
unsafe {
gl::GenVertexArrays(1, &mut vao);
gl::GenBuffers(1, &mut vbo);
gl::GenBuffers(1, &mut ebo);
gl::BindVertexArray(vao);
gl::BindBuffer(gl::ARRAY_BUFFER, vbo);
gl::BufferData(
gl::ARRAY_BUFFER,
(vertices.len() * std::mem::size_of::<f32>()) as gl::types::GLsizeiptr,
vertices.as_ptr() as *const _,
gl::STATIC_DRAW
);
gl::BindBuffer(gl::ELEMENT_ARRAY_BUFFER, ebo);
gl::BufferData(
gl::ELEMENT_ARRAY_BUFFER,
(indices.len() * std::mem::size_of::<u32>()) as gl::types::GLsizeiptr,
indices.as_ptr() as *const _,
gl::STATIC_DRAW
);
// Position attribute
gl::VertexAttribPointer(
0, 3, gl::FLOAT, gl::FALSE,
5 * std::mem::size_of::<f32>() as gl::types::GLsizei,
std::ptr::null()
);
gl::EnableVertexAttribArray(0);
// Texture coordinate attribute
gl::VertexAttribPointer(
1, 2, gl::FLOAT, gl::FALSE,
5 * std::mem::size_of::<f32>() as gl::types::GLsizei,
(3 * std::mem::size_of::<f32>()) as *const _
);
gl::EnableVertexAttribArray(1);
}
// Load and create texture
let texture = load_texture("texture.jpg");
// Shader creation
let vertex_shader_src = r#"
#version 330 core
layout (location = 0) in vec3 aPos;
layout (location = 1) in vec2 aTexCoord;
out vec2 TexCoord;
void main() {
gl_Position = vec4(aPos, 1.0);
TexCoord = aTexCoord;
}
"#;
let fragment_shader_src = r#"
#version 330 core
out vec4 FragColor;
in vec2 TexCoord;
uniform sampler2D texture1;
void main() {
FragColor = texture(texture1, TexCoord);
}
"#;
let shader_program = ShaderProgram::new(vertex_shader_src, fragment_shader_src)
.expect("Failed to create shader program");
// Main render loop
event_loop.run(move |event, _, control_flow| {
match event {
glutin::event::Event::WindowEvent { event, .. } => match event {
glutin::event::WindowEvent::CloseRequested => {
*control_flow = ControlFlow::Exit
},
_ => {}
},
glutin::event::Event::RedrawRequested(_) => {
// Render
unsafe {
gl::ClearColor(0.2, 0.3, 0.3, 1.0);
gl::Clear(gl::COLOR_BUFFER_BIT);
// Bind texture
gl::ActiveTexture(gl::TEXTURE0);
gl::BindTexture(gl::TEXTURE_2D, texture);
// Render container
shader_program.use_program();
gl::BindVertexArray(vao);
gl::DrawElements(gl::TRIANGLES, 6, gl::UNSIGNED_INT, std::ptr::null());
}
windowed_context.swap_buffers().unwrap();
},
_ => {}
}
});
}
fn load_texture(path: &str) -> gl::types::GLuint {
let mut texture = 0;
// Load image with the image crate
let img = image::open(path).expect("Failed to load texture").flipv();
let data = img.as_bytes();
let format = match img.color() {
image::ColorType::Rgb8 => gl::RGB,
image::ColorType::Rgba8 => gl::RGBA,
_ => panic!("Unsupported image format"),
};
unsafe {
gl::GenTextures(1, &mut texture);
gl::BindTexture(gl::TEXTURE_2D, texture);
// Set texture parameters
gl::TexParameteri(gl::TEXTURE_2D, gl::TEXTURE_WRAP_S, gl::REPEAT as i32);
gl::TexParameteri(gl::TEXTURE_2D, gl::TEXTURE_WRAP_T, gl::REPEAT as i32);
gl::TexParameteri(gl::TEXTURE_2D, gl::TEXTURE_MIN_FILTER, gl::LINEAR as i32);
gl::TexParameteri(gl::TEXTURE_2D, gl::TEXTURE_MAG_FILTER, gl::LINEAR as i32);
// Upload texture data
gl::TexImage2D(
gl::TEXTURE_2D,
0,
format as i32,
img.width() as i32,
img.height() as i32,
0,
format,
gl::UNSIGNED_BYTE,
data.as_ptr() as *const _
);
gl::GenerateMipmap(gl::TEXTURE_2D);
}
texture
}
This example demonstrates handling vertex data, index buffers, texture loading, and shader management in a cohesive application.
Performance Considerations
Graphics applications often need to process large amounts of data quickly. Rust provides several tools for optimizing OpenGL performance:
- Zero-cost abstractions let you build safe wrappers without runtime overhead
- Fine-grained control over memory layout for cache-friendly data structures
- SIMD optimization through libraries like
glam
for vector math - Efficient buffer mapping with minimal overhead
For instance, when updating buffer data frequently:
// Efficient dynamic buffer update
let mut positions = Vec::with_capacity(1000);
// Fill positions...
unsafe {
gl::BindBuffer(gl::ARRAY_BUFFER, vbo);
// Map buffer for writing
let ptr = gl::MapBuffer(gl::ARRAY_BUFFER, gl::WRITE_ONLY) as *mut f32;
// Direct memory access - very efficient
for (i, &pos) in positions.iter().enumerate() {
*ptr.add(i) = pos;
}
// Unmap buffer
gl::UnmapBuffer(gl::ARRAY_BUFFER);
}
This approach minimizes data copying, allowing direct access to GPU memory through Rust's pointer manipulation capabilities.
Advanced Rendering Techniques
Rust's expressiveness enables clean implementations of complex rendering techniques:
Deferred Rendering
// G-buffer setup
struct GBuffer {
fbo: gl::types::GLuint,
position_texture: gl::types::GLuint,
normal_texture: gl::types::GLuint,
albedo_spec_texture: gl::types::GLuint,
depth_rbo: gl::types::GLuint,
}
impl GBuffer {
fn new(width: i32, height: i32) -> Self {
let mut g_buffer = GBuffer {
fbo: 0,
position_texture: 0,
normal_texture: 0,
albedo_spec_texture: 0,
depth_rbo: 0,
};
unsafe {
// Create framebuffer
gl::GenFramebuffers(1, &mut g_buffer.fbo);
gl::BindFramebuffer(gl::FRAMEBUFFER, g_buffer.fbo);
// Position buffer
gl::GenTextures(1, &mut g_buffer.position_texture);
gl::BindTexture(gl::TEXTURE_2D, g_buffer.position_texture);
gl::TexImage2D(gl::TEXTURE_2D, 0, gl::RGBA16F as i32, width, height, 0, gl::RGBA, gl::FLOAT, std::ptr::null());
gl::TexParameteri(gl::TEXTURE_2D, gl::TEXTURE_MIN_FILTER, gl::NEAREST as i32);
gl::TexParameteri(gl::TEXTURE_2D, gl::TEXTURE_MAG_FILTER, gl::NEAREST as i32);
gl::FramebufferTexture2D(gl::FRAMEBUFFER, gl::COLOR_ATTACHMENT0, gl::TEXTURE_2D, g_buffer.position_texture, 0);
// Normal buffer
gl::GenTextures(1, &mut g_buffer.normal_texture);
gl::BindTexture(gl::TEXTURE_2D, g_buffer.normal_texture);
gl::TexImage2D(gl::TEXTURE_2D, 0, gl::RGBA16F as i32, width, height, 0, gl::RGBA, gl::FLOAT, std::ptr::null());
gl::TexParameteri(gl::TEXTURE_2D, gl::TEXTURE_MIN_FILTER, gl::NEAREST as i32);
gl::TexParameteri(gl::TEXTURE_2D, gl::TEXTURE_MAG_FILTER, gl::NEAREST as i32);
gl::FramebufferTexture2D(gl::FRAMEBUFFER, gl::COLOR_ATTACHMENT1, gl::TEXTURE_2D, g_buffer.normal_texture, 0);
// Albedo + specular buffer
gl::GenTextures(1, &mut g_buffer.albedo_spec_texture);
gl::BindTexture(gl::TEXTURE_2D, g_buffer.albedo_spec_texture);
gl::TexImage2D(gl::TEXTURE_2D, 0, gl::RGBA as i32, width, height, 0, gl::RGBA, gl::UNSIGNED_BYTE, std::ptr::null());
gl::TexParameteri(gl::TEXTURE_2D, gl::TEXTURE_MIN_FILTER, gl::NEAREST as i32);
gl::TexParameteri(gl::TEXTURE_2D, gl::TEXTURE_MAG_FILTER, gl::NEAREST as i32);
gl::FramebufferTexture2D(gl::FRAMEBUFFER, gl::COLOR_ATTACHMENT2, gl::TEXTURE_2D, g_buffer.albedo_spec_texture, 0);
// Tell OpenGL which color attachments to use for rendering
let attachments = [gl::COLOR_ATTACHMENT0, gl::COLOR_ATTACHMENT1, gl::COLOR_ATTACHMENT2];
gl::DrawBuffers(3, attachments.as_ptr());
// Create depth buffer
gl::GenRenderbuffers(1, &mut g_buffer.depth_rbo);
gl::BindRenderbuffer(gl::RENDERBUFFER, g_buffer.depth_rbo);
gl::RenderbufferStorage(gl::RENDERBUFFER, gl::DEPTH_COMPONENT, width, height);
gl::FramebufferRenderbuffer(gl::FRAMEBUFFER, gl::DEPTH_ATTACHMENT, gl::RENDERBUFFER, g_buffer.depth_rbo);
// Check if framebuffer is complete
if gl::CheckFramebufferStatus(gl::FRAMEBUFFER) != gl::FRAMEBUFFER_COMPLETE {
panic!("Framebuffer not complete!");
}
gl::BindFramebuffer(gl::FRAMEBUFFER, 0);
}
g_buffer
}
}
impl Drop for GBuffer {
fn drop(&mut self) {
unsafe {
gl::DeleteFramebuffers(1, &self.fbo);
gl::DeleteTextures(1, &self.position_texture);
gl::DeleteTextures(1, &self.normal_texture);
gl::DeleteTextures(1, &self.albedo_spec_texture);
gl::DeleteRenderbuffers(1, &self.depth_rbo);
}
}
}
Instanced Rendering
// Efficient instanced rendering
fn render_instanced(positions: &[nalgebra::Vector3<f32>]) {
// Generate instance buffer
let mut instance_buffer = 0;
unsafe {
gl::GenBuffers(1, &mut instance_buffer);
gl::BindBuffer(gl::ARRAY_BUFFER, instance_buffer);
gl::BufferData(
gl::ARRAY_BUFFER,
(positions.len() * std::mem::size_of::<nalgebra::Vector3<f32>>()) as gl::types::GLsizeiptr,
positions.as_ptr() as *const _,
gl::STATIC_DRAW
);
gl::BindVertexArray(vao);
// Configure instance data
gl::EnableVertexAttribArray(2);
gl::VertexAttribPointer(
2, 3, gl::FLOAT, gl::FALSE,
std::mem::size_of::<nalgebra::Vector3<f32>>() as gl::types::GLsizei,
std::ptr::null()
);
gl::VertexAttribDivisor(2, 1); // Tell OpenGL this is per-instance data
// Render all instances
gl::DrawElementsInstanced(
gl::TRIANGLES,
indices_count,
gl::UNSIGNED_INT,
std::ptr::null(),
positions.len() as gl::types::GLsizei
);
gl::DeleteBuffers(1, &instance_buffer);
}
}
Higher-Level Abstractions
While the examples above use mostly raw OpenGL bindings, several higher-level libraries simplify Rust OpenGL development:
-
glow
provides a common API across WebGL and OpenGL -
luminance
offers a safer, higher-level graphics framework -
wgpu
provides a modern graphics API abstraction supporting WebGPU, Vulkan, Metal, and D3D12 along with a fallback OpenGL backend
These libraries build on Rust's safety features to create more ergonomic APIs without sacrificing performance.
Personal Experience
I've found that the combination of Rust and OpenGL transformed how I think about graphics code. When I first started with OpenGL in C++, I spent countless hours tracking down buffer management bugs and memory leaks. After switching to Rust, these issues largely disappeared.
For one recent project, I created a procedural terrain renderer. Rust's ownership model elegantly handled the complex relationships between the terrain chunks, their mesh data, and textures. The borrow checker prevented me from accidentally using meshes that were being updated, leading to significantly fewer bugs.
The safety guarantees also gave me confidence to aggressively optimize the renderer. I could safely implement complex caching strategies and buffer reuse patterns without worrying about introducing memory corruption.
Conclusion
Rust provides an excellent foundation for OpenGL development. The language's focus on memory safety, zero-cost abstractions, and performance aligns perfectly with the needs of graphics programming.
By encapsulating OpenGL objects in Rust types with proper lifetime management, you can eliminate entire categories of common graphics bugs. The type system helps express relationships between graphics resources clearly, and the borrow checker ensures those relationships remain valid.
Whether you're creating a game engine, a data visualization tool, or a creative coding project, the combination of Rust and OpenGL offers a powerful, productive, and reliable platform for graphics development.
101 Books
101 Books is an AI-driven publishing company co-founded by author Aarav Joshi. By leveraging advanced AI technology, we keep our publishing costs incredibly low—some books are priced as low as $4—making quality knowledge accessible to everyone.
Check out our book Golang Clean Code available on Amazon.
Stay tuned for updates and exciting news. When shopping for books, search for Aarav Joshi to find more of our titles. Use the provided link to enjoy special discounts!
Our Creations
Be sure to check out our creations:
Investor Central | Investor Central Spanish | Investor Central German | Smart Living | Epochs & Echoes | Puzzling Mysteries | Hindutva | Elite Dev | JS Schools
We are on Medium
Tech Koala Insights | Epochs & Echoes World | Investor Central Medium | Puzzling Mysteries Medium | Science & Epochs Medium | Modern Hindutva