Why React Rocks: A Friendly Guide
If you’ve ever worked with plain JavaScript, you know the drill: whenever you want to update the page, you reach out, grab elements from the DOM, and fiddle with them directly. With React, it’s a whole different—and way more delightful—approach. Here’s why React is so special: Don’t Touch the DOM — I’ll Handle It: You never manually touch the DOM. Instead, you write “React components” that declare what you want the page to look like for a given state. Build Websites Like LEGO Blocks: Imagine you’re playing with LEGO bricks. You have small pieces (4×2 bricks, tiny tiles) that snap together to build bigger structures (houses, castles). React works the same way: you build small, reusable components and compose them into larger ones. Because each piece is self‑contained—handling its own state, styles, and rendering logic—you can reuse it anywhere. Unidirectional Data Flow: Top → Down, Easy to Debug: With jQuery or older frameworks, it was easy to get tangled in “who updated what and when.” Data might change in one place, trigger an event somewhere else, and suddenly your page is out of sync, React enforces a unidirectional data flow: Parent components pass props (data) down to child components. If a child wants to change something—say, a button click triggers an update—it calls a function (prop) passed down from the parent. That parent function updates its state, React re-renders the parent and its children, and the new data flows down again. Visually, it looks like a single arrow: Parent → Child → Child → … When something breaks, you know exactly where to look: follow the arrow upstream to see where the data originated, and downstream to see where it ended up. I’m Just the UI—The Rest Is Up to You: Think of a full-fledged framework (like Angular) as a complete kitchen: it comes with the stove, oven, fridge, sink, cabinets—everything you need in one place. You open the door and say, “Alright, let’s make dinner,” and most of the tools are already there. React, on the other hand, is more like a quality stove. It’s fantastic at one job—cooking (i.e., rendering user interfaces). But if you want to store ingredients (data fetching, state management), wash dishes (routing, form validation), or preserve leftovers (server‑side rendering, build tooling), you’ll need to bring in your own fridge, sink, and cabinets—that is, other libraries: Data-fetching & state management: You might choose React Query or Axios + useState/useEffect, or pick Redux if you want a global store with strict rules. Routing: Use React Router to handle page URLs. Form management: Reach for Formik or React Hook Form. Styling: Try styled-components, Emotion, or plain CSS Modules. Because React only focuses on “how to render a component tree”, it’s extremely flexible—and this approach is often called “cross‑platform” because the same virtual DOM blueprint can power web apps, mobile apps (with React Native), VR experiences (with React 360), and more. You swap out the “renderer” (e.g., React DOM vs. React Native) and keep your component logic the same. React’s magic comes down to four big ideas: Declarative paradigm: Tell React what you want, not how to manipulate the DOM step by step. Component-based design: Build UIs like LEGO—small, reusable blocks that fit together. Unidirectional data flow: Data moves predictably from parents to children, making debugging a breeze. UI library, not full framework: React is a powerful “stove,” and you’re free to choose which “fridge,” “sink,” and “cabinets” you pair it with—fueling cross‑platform experiences from the same core components.
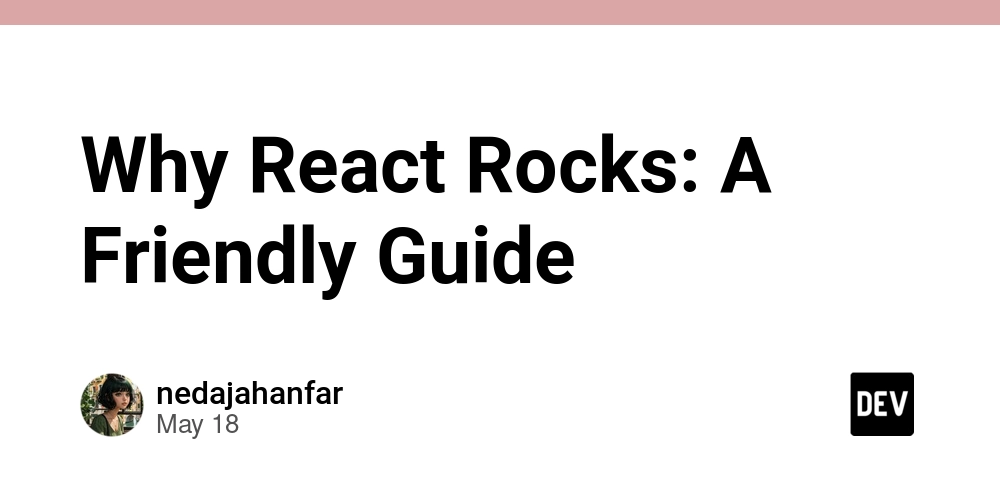
If you’ve ever worked with plain JavaScript, you know the drill: whenever you want to update the page, you reach out, grab elements from the DOM, and fiddle with them directly. With React, it’s a whole different—and way more delightful—approach. Here’s why React is so special:
- Don’t Touch the DOM — I’ll Handle It: You never manually touch the DOM. Instead, you write “React components” that declare what you want the page to look like for a given state.
- Build Websites Like LEGO Blocks: Imagine you’re playing with LEGO bricks. You have small pieces (4×2 bricks, tiny tiles) that snap together to build bigger structures (houses, castles). React works the same way: you build small, reusable components and compose them into larger ones. Because each piece is self‑contained—handling its own state, styles, and rendering logic—you can reuse it anywhere.
- Unidirectional Data Flow: Top → Down, Easy to Debug: With jQuery or older frameworks, it was easy to get tangled in “who updated what and when.” Data might change in one place, trigger an event somewhere else, and suddenly your page is out of sync, React enforces a unidirectional data flow:
Parent components pass props (data) down to child components.
If a child wants to change something—say, a button click triggers an update—it calls a function (prop) passed down from the parent.
That parent function updates its state, React re-renders the parent and its children, and the new data flows down again.
Visually, it looks like a single arrow: Parent → Child → Child → … When something breaks, you know exactly where to look: follow the arrow upstream to see where the data originated, and downstream to see where it ended up.
- I’m Just the UI—The Rest Is Up to You: Think of a full-fledged framework (like Angular) as a complete kitchen: it comes with the stove, oven, fridge, sink, cabinets—everything you need in one place. You open the door and say, “Alright, let’s make dinner,” and most of the tools are already there. React, on the other hand, is more like a quality stove. It’s fantastic at one job—cooking (i.e., rendering user interfaces). But if you want to store ingredients (data fetching, state management), wash dishes (routing, form validation), or preserve leftovers (server‑side rendering, build tooling), you’ll need to bring in your own fridge, sink, and cabinets—that is, other libraries:
Data-fetching & state management: You might choose React Query or Axios + useState/useEffect, or pick Redux if you want a global store with strict rules.
Routing: Use React Router to handle page URLs.
Form management: Reach for Formik or React Hook Form.
Styling: Try styled-components, Emotion, or plain CSS Modules.
Because React only focuses on “how to render a component tree”, it’s extremely flexible—and this approach is often called “cross‑platform” because the same virtual DOM blueprint can power web apps, mobile apps (with React Native), VR experiences (with React 360), and more. You swap out the “renderer” (e.g., React DOM vs. React Native) and keep your component logic the same.
React’s magic comes down to four big ideas:
Declarative paradigm: Tell React what you want, not how to manipulate the DOM step by step.
Component-based design: Build UIs like LEGO—small, reusable blocks that fit together.
Unidirectional data flow: Data moves predictably from parents to children, making debugging a breeze.
UI library, not full framework: React is a powerful “stove,” and you’re free to choose which “fridge,” “sink,” and “cabinets” you pair it with—fueling cross‑platform experiences from the same core components.