Building an HR Team-Matching Agent With MongoDB Vector Search, Voyage AI, & Vercel AI SDK
Picture this: You're an HR manager at a growing tech company. It's Monday morning, and the VP of Product has just dropped a request in your inbox: "We need a team for a new strategic project. Must have experience with React, MongoDB, authentication systems, and cloud deployment. They need to work well together, and we need them to start by Friday." Sound familiar? If you've worked in HR or talent management, you know this scenario all too well. Building the right team is often more art than science. The traditional approach involves scanning resumes for keywords, checking availability spreadsheets, and relying heavily on your memory of who works well with whom. It's manual, time-consuming, and prone to overlooking the perfect candidate whose resume doesn't explicitly list "React" but has extensive "frontend JavaScript framework" experience. This is precisely the kind of problem where traditional workflow-based AI falls short. If you tried to map out every possible decision tree for team building, you'd need to account for: Thousands of possible skill combinations. Varying levels of experience and proficiency. Complex interpersonal dynamics. Project-specific requirements. Changing availability. Career development goals. No wonder traditional HR systems struggle with this complexity! Enter agentic AI with Vercel and MongoDB What if you could simply describe your project needs in plain language and have an AI assistant that: Understands the semantic meaning behind your requirements? Searches your talent database using intelligent matching? Reasons through complex team compositions? Provides recommendations with clear justifications? Saves teams to a database for tracking and management? This is where the combination of Vercel AI SDK's agentic capabilities and MongoDB's Vector Search becomes transformative. Unlike traditional AI approaches that follow rigid, predefined paths, agentic AI can break down complex problems into manageable steps and solve them iteratively—much like an experienced HR professional would. The Vercel AI SDK makes this easy with its maxSteps parameter, allowing the AI to make a series of intelligent decisions as it builds the perfect team. Meanwhile, MongoDB's vector search capabilities solve the keyword matching problem by understanding the semantic relationships between skills. "React developer," "JSX experience," and "frontend JavaScript framework specialist" are no longer separate search terms—they're related concepts in a semantic space. In this article, we'll build an HR team-matching agent that combines these powerful technologies to transform how organizations assemble project teams. You'll learn how to: Represent employee skills as vector embeddings using Voyage AI. Create powerful search tools with MongoDB Atlas Vector Search. Implement agentic patterns with Vercel AI SDK. Build a complete database-backed system for team recommendations. Create a management interface for tracking and approving teams. Let's dive in! Understanding vector embeddings for skills Before we dive into the implementation, let's discuss why vector embeddings are so powerful for representing employee skills. Traditional keyword matching has significant limitations in HR contexts: Synonym problems: Does your database recognize that "ML" and "Machine Learning" are the same skill? Contextual understanding: Is "Python" being used for data science, web development, or automation? Skill relatedness: How do you capture that someone with React experience might ramp up quickly on Vue? Expertise levels: How do you differentiate between "familiar with Python" and "Python expert"? Vector embeddings solve these challenges by representing skills in a high-dimensional space where semantic similarity corresponds to vector proximity. This allows us to capture nuanced relationships between skills that keyword matching simply cannot. Generating skill embeddings with Voyage AI For our implementation, we'll use Voyage AI to generate high-quality embeddings. Voyage offers state-of-the-art embedding models that capture the semantic relationships between different skills and technical concepts. Recently, Voyage AI joined MongoDB. With Voyage AI, MongoDB's roadmap is to have AI-powered search and retrieval native to the database. Instead of implementing workarounds or managing separate systems, developers can generate high-quality embeddings from real-time operational data, store vectors, perform semantic search, and refine results—all within MongoDB. First, let's create a utility function to generate embeddings (GitHub): import { voyage } from 'voyage-ai-provider'; import { embedMany } from 'ai'; const embeddingModel = voyage.textEmbeddingModel('voyage-3'); export const generateEmbeddings = async ( value: string, ): Promise => { // Generate chunks from the input value const chunks = value.split('\n'); const { embeddings } = await embedMany({ model: embed
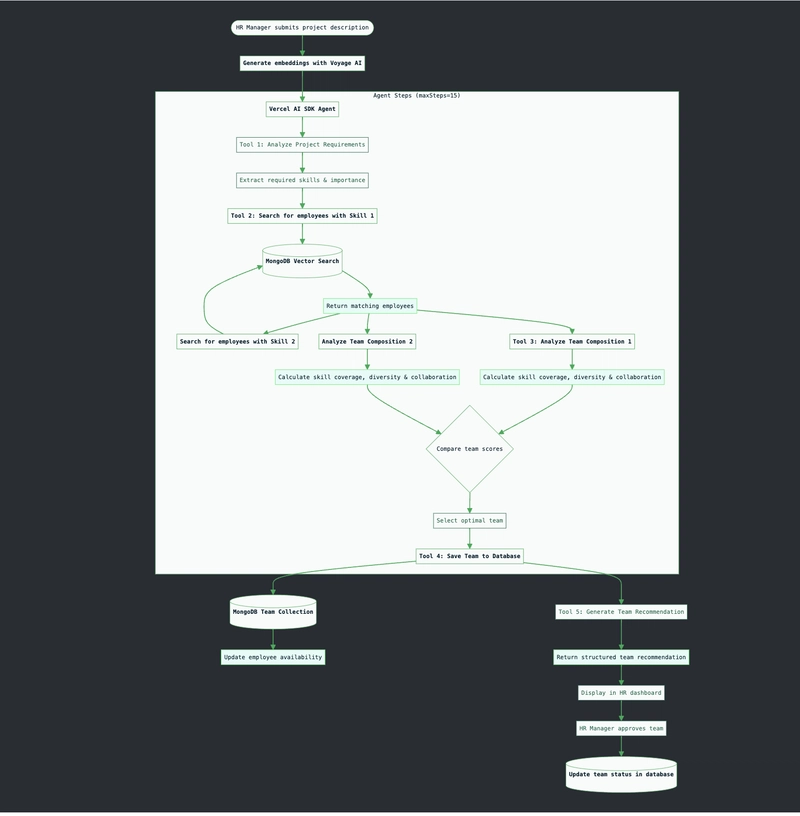
Picture this: You're an HR manager at a growing tech company. It's Monday morning, and the VP of Product has just dropped a request in your inbox: "We need a team for a new strategic project. Must have experience with React, MongoDB, authentication systems, and cloud deployment. They need to work well together, and we need them to start by Friday."
Sound familiar? If you've worked in HR or talent management, you know this scenario all too well.
Building the right team is often more art than science. The traditional approach involves scanning resumes for keywords, checking availability spreadsheets, and relying heavily on your memory of who works well with whom. It's manual, time-consuming, and prone to overlooking the perfect candidate whose resume doesn't explicitly list "React" but has extensive "frontend JavaScript framework" experience.
This is precisely the kind of problem where traditional workflow-based AI falls short. If you tried to map out every possible decision tree for team building, you'd need to account for:
- Thousands of possible skill combinations.
- Varying levels of experience and proficiency.
- Complex interpersonal dynamics.
- Project-specific requirements.
- Changing availability.
- Career development goals.
No wonder traditional HR systems struggle with this complexity!
Enter agentic AI with Vercel and MongoDB
What if you could simply describe your project needs in plain language and have an AI assistant that:
- Understands the semantic meaning behind your requirements?
- Searches your talent database using intelligent matching?
- Reasons through complex team compositions?
- Provides recommendations with clear justifications?
- Saves teams to a database for tracking and management?
This is where the combination of Vercel AI SDK's agentic capabilities and MongoDB's Vector Search becomes transformative.
Unlike traditional AI approaches that follow rigid, predefined paths, agentic AI can break down complex problems into manageable steps and solve them iteratively—much like an experienced HR professional would. The Vercel AI SDK makes this easy with its maxSteps
parameter, allowing the AI to make a series of intelligent decisions as it builds the perfect team.
Meanwhile, MongoDB's vector search capabilities solve the keyword matching problem by understanding the semantic relationships between skills. "React developer," "JSX experience," and "frontend JavaScript framework specialist" are no longer separate search terms—they're related concepts in a semantic space.
In this article, we'll build an HR team-matching agent that combines these powerful technologies to transform how organizations assemble project teams. You'll learn how to:
- Represent employee skills as vector embeddings using Voyage AI.
- Create powerful search tools with MongoDB Atlas Vector Search.
- Implement agentic patterns with Vercel AI SDK.
- Build a complete database-backed system for team recommendations.
- Create a management interface for tracking and approving teams.
Let's dive in!
Understanding vector embeddings for skills
Before we dive into the implementation, let's discuss why vector embeddings are so powerful for representing employee skills.
Traditional keyword matching has significant limitations in HR contexts:
- Synonym problems: Does your database recognize that "ML" and "Machine Learning" are the same skill?
- Contextual understanding: Is "Python" being used for data science, web development, or automation?
- Skill relatedness: How do you capture that someone with React experience might ramp up quickly on Vue?
- Expertise levels: How do you differentiate between "familiar with Python" and "Python expert"?
Vector embeddings solve these challenges by representing skills in a high-dimensional space where semantic similarity corresponds to vector proximity. This allows us to capture nuanced relationships between skills that keyword matching simply cannot.
Generating skill embeddings with Voyage AI
For our implementation, we'll use Voyage AI to generate high-quality embeddings. Voyage offers state-of-the-art embedding models that capture the semantic relationships between different skills and technical concepts. Recently, Voyage AI joined MongoDB. With Voyage AI, MongoDB's roadmap is to have AI-powered search and retrieval native to the database. Instead of implementing workarounds or managing separate systems, developers can generate high-quality embeddings from real-time operational data, store vectors, perform semantic search, and refine results—all within MongoDB.
First, let's create a utility function to generate embeddings (GitHub):
import { voyage } from 'voyage-ai-provider';
import { embedMany } from 'ai';
const embeddingModel = voyage.textEmbeddingModel('voyage-3');
export const generateEmbeddings = async (
value: string,
): Promise<Array<{ embedding: number[]; content: string }>> => {
// Generate chunks from the input value
const chunks = value.split('\n');
const { embeddings } = await embedMany({
model: embeddingModel,
values: chunks,
});
return embeddings.map((e, i) => ({ content: chunks[i], embedding: e }));
};
When creating or updating employee profiles, we'll generate embeddings for their skills that capture not just the skill name but also proficiency, experience, and contexts:
async function generateSkillEmbeddings(employees: Array<Omit<Employee, '_id'>>) {
for (const employee of employees) {
try {
// Create combined skill description using | as separator
const skillDescriptions = employee.skills.map(skill =>
`${skill.name}: ${skill.proficiency}/5 proficiency with ${skill.yearsExperience} years experience in contexts like ${skill.contexts.join(', ')}`
);
const combinedSkillDescription = skillDescriptions.join(' | ');
// Generate embedding for all skills combined
const embeddingResults = await generateEmbeddings(combinedSkillDescription);
// Add the embedding to the employee document at the top level
if (embeddingResults.length > 0) {
(employee as any).embedding = embeddingResults[0].embedding;
console.log(`✅ Generated top-level embedding for ${employee.name}'s combined skills`);
} else {
console.warn(`⚠️ No embeddings generated for ${employee.name}'s combined skills`);
(employee as any).embedding = [];
}
} catch (error) {
console.error(`❌ Error generating embedding for ${employee.name}'s skills:`, error);
// Set an empty embedding as fallback
(employee as any).embedding = [];
}
}
return employees;
}
async function updateEmployeeSkillEmbeddings(employee) {
const employeesWithEmbeddings = await generateSkillEmbeddings(employee);
// Update employee record in MongoDB
await db.employees.updateOne(
{ _id: employee._id },
{ $set: { embedding: employeesWithEmbeddings.embedding } }
);
}
MongoDB data models and vector search setup
Before we dive into the implementation details, let's define our MongoDB schema and set up vector search. (GitHub)
Database schemas
// types.ts - Database schema definitions
export interface Employee {
_id?: ObjectId;
name: string;
title: string;
department: string;
email: string;
hireDate: Date;
availability: number; // percentage available for new projects
skills: Skill[];
performance: {
rating: number; // 1-5 scale
teamworkScore: number; // 1-5 scale
lastReviewDate: Date;
};
pastProjects: {
projectId: ObjectId;
role: string;
contribution: string;
}[];
teamHistory: ObjectId[]; // IDs of employees they've worked with
embedding: number[]; // Combined skills embedding at the top level
}
export interface Team {
_id?: ObjectId;
projectTitle: string;
projectDescription: string;
createdAt: Date;
members: {
name: string;
role: string;
keySkills: string[];
justification: string;
}[];
skillCoverage: number;
overallRationale: string;
risks: string[];
mitigationStrategies: string[];
status: 'proposed' | 'approved' | 'active' | 'completed';
}
Setting up MongoDB Atlas Vector Search
First, we need to create a vector search index in MongoDB Atlas on the "employees" collection:
// Vector search index configuration via atlas UI called `skill_vector_index`
{
"fields": [
{
"type": "vector",
"path": "embedding",
"numDimensions": 1024,
"similarity": "cosine"
}
]
}
Once our index is set up, we can perform vector searches to find employees with specific skills:
const embedding = await generateEmbeddings(query)
// Perform vector search with additional filters
const matchingEmployees = await employeesCollection.aggregate([
{
$vectorSearch: {
index: "skills_vector_index",
path: "embedding",
queryVector: embedding,
numCandidates: 100,
limit: 20
}
},
// Filter by proficiency and availability
{
$match: {
"skills.proficiency": { $gte: minProficiency },
"availability": { $gte: minAvailability }
}
},
// Project relevant fields
{
$project: {
_id: 1,
name: 1,
title: 1,
department: 1,
availability: 1,
hireDate: 1,
performance: 1,
relevantSkills: {
$filter: {
input: "$skills",
as: "skill",
cond: { $gte: ["$$skill.proficiency", minProficiency] }
}
},
pastProjects: 1,
teamHistory: 1,
similarity: { $meta: "vectorSearchScore" }
}
},
// Sort by similarity score
{
$sort: {
similarity: -1
}
}
]).toArray();
return matchingEmployees;
This query will return employees whose skills are semantically similar to the requested skill, not just those who have an exact keyword match.
Building agentic HR tools with Vercel AI SDK
What makes Vercel AI SDK so powerful for HR applications is its agentic capabilities. Unlike traditional AI implementations that follow a fixed workflow, an agentic approach allows the AI to break down complex problems, make decisions, and iteratively work toward a solution.
This is particularly valuable for HR challenges like team building, where the solution path isn't always clear at the outset.
Understanding Vercel's agentic pattern
At the heart of Vercel's agentic implementation is the maxSteps
parameter. Here's how it works:
- The HR manager submits a project description.
- The AI analyzes the request and determines what information it needs.
- The AI calls a tool (like searching for employees with a particular skill).
- The AI receives the result and decides what to do next.
- Steps 3-4 repeat until the AI has gathered enough information to make a recommendation.
- The AI delivers a final, structured recommendation.
This iterative process mirrors how an experienced HR professional might approach team building—gathering information, testing hypotheses, and refining recommendations based on what they learn.
Implementing team analysis logic
Before we create our tools, let's implement the core logic for analyzing team compositions (GitHub):
calculateSkillCoverage
calculateTeamDiversity
analyzeCollaborationHistory
calculateTeamScore
Defining our HR agent tools
Now, let's create the specialized tools our HR agent will need. These are the available endpoints for the large language model to produce the needed context or results (GitHub):
analyzeProjectRequirements
searchEmployeesBySkill
analyzeTeamComposition
saveTeamToDatabase
-
generateTeamRecommendation
import { tool } from 'ai';
import { z } from 'zod';
import { MongoClient, ObjectId } from 'mongodb';
import { voyage } from 'voyage-ai-provider';
import { embedMany } from 'ai';
import {
calculateSkillCoverage,
calculateTeamDiversity,
analyzeCollaborationHistory,
calculateTeamScore,
generateTeamAnalysis
} from './teamAnalysis';
import { Employee, Team } from './types';
import { generateEmbeddings } from './embeddings';
// Initialize MongoDB connection
const mongoClient = new MongoClient(process.env.MONGODB_URI!);
const db = mongoClient.db('hr_database');
const employeesCollection = db.collection<Employee>('employees');
const teamsCollection = db.collection<Team>('teams');
// Tool 1: Project Requirements Analysis
// Tool 2: Skill Search with MongoDB Vector Search
export const searchEmployeesBySkill = tool({
description: 'Searches for employees with specific skills using semantic matching.',
parameters: z.object({
skillDescription: z.string().describe('Description of the required skill'),
minProficiency: z.number().describe('Minimum proficiency level (1-5)'),
minAvailability: z.number().describe('Minimum availability percentage')
}),
execute: async ({ skillDescription, minProficiency = 3, minAvailability = 20 }) => {
try {
// Generate embedding for the skill description using Voyage AI
const embeddingResults = await generateEmbeddings(skillDescription);
if (embeddingResults.length === 0) {
return { error: "Failed to generate embedding for skill description" };
}
const embedding = embeddingResults[0].embedding;
// Perform vector search with additional filters
const matchingEmployees = await employeesCollection.aggregate([
{
$vectorSearch: {
index: "skills_vector_index",
path: "embedding",
queryVector: embedding,
numCandidates: 100,
limit: 20
}
},
// Filter by proficiency and availability
{
$match: {
"skills.proficiency": { $gte: minProficiency },
"availability": { $gte: minAvailability }
}
},
// Project relevant fields
{
$project: {
_id: 1,
name: 1,
title: 1,
department: 1,
availability: 1,
hireDate: 1,
performance: 1,
relevantSkills: {
$filter: {
input: "$skills",
as: "skill",
cond: { $gte: ["$$skill.proficiency", minProficiency] }
}
},
pastProjects: 1,
teamHistory: 1,
similarity: { $meta: "vectorSearchScore" }
}
}
]).toArray();
return matchingEmployees;
} catch (error) {
console.error("Error searching for employees:", error);
return { error: "Failed to search for employees" };
}
},
});
// Tool 3: Team Composition Analysis
// Tool 4: Database Integration - Save Team
// Tool 5: Team Recommendation (structured output)
Integrating tools with Vercel AI SDK
Now, let's implement the main agent function that orchestrates these tools under api/build-team/route.ts
(GitHub):
import { NextRequest, NextResponse } from 'next/server';
import { openai } from '@ai-sdk/openai';
import { generateText, tool } from 'ai';
import { z } from 'zod';
import {
analyzeProjectRequirements,
searchEmployeesBySkill,
analyzeTeamComposition,
saveTeamToDatabase,
generateTeamRecommendation
} from '../../../utils/tools';
export async function POST(req: NextRequest) {
try {
const { projectDescription } = await req.json();
if (!projectDescription) {
return NextResponse.json({ error: 'Project description is required' }, { status: 400 });
}
const result = await buildTeam(projectDescription);
return NextResponse.json(result);
} catch (error) {
console.error('Error building team:', error);
return NextResponse.json({ error: 'Failed to build team' }, { status: 500 });
}
}
async function buildTeam(projectDescription: string) {
const { steps, toolCalls } = await generateText({
model: openai('o3-mini', { structuredOutputs: true }),
tools: {
analyzeProjectRequirements,
searchEmployeesBySkill,
analyzeTeamComposition,
saveTeamToDatabase,
generateTeamRecommendation,
},
toolChoice: 'auto', // Force structured output using the generateTeamRecommendation tool
maxSteps: 15, // This is the key to enabling agentic behavior!
system: `You are an expert HR assistant that builds optimal teams for projects.
Given a project description follow this set of steps:
1. Analyze the requirements to identify needed skills (analyzeProjectRequirements)
2. Search for employees with matching skills (searchEmployeesBySkill)
3. Evaluate possible team compositions (analyzeTeamComposition)
4. MUST Save the recommended team to the database (saveTeamToDatabase)
5. Generate a final recommendation with justification (generateTeamRecommendation)
Consider skill coverage, team diversity, availability, and past collaboration success.
Be thorough in your analysis but aim to build the smallest effective team possible. If unable to complete a team, please provide a reason why but do place people with potential.
After your final recommendation is complete, save the team to the database for record-keeping.`,
prompt: projectDescription,
});
console.log(JSON.stringify(steps.map(step => step.toolCalls), null, 2))
// Find the last generateTeamRecommendation call for the final recommendation
const recommendationCall = toolCalls.find(
call => call.toolName === 'generateTeamRecommendation'
);
// Find the database save call to get the team ID
const databaseCall = toolCalls.find(
call => call.toolName === 'saveTeamToDatabase'
);
// Combine the recommendation with the database result
if (recommendationCall && databaseCall) {
return {
recommendation: recommendationCall.args,
databaseResult: databaseCall.args
};
}
// Fallback to just the recommendation if database save failed
if (recommendationCall) {
return { recommendation: recommendationCall.args };
}
return { error: "Failed to generate team recommendation" };
}
The maxSteps
parameter is what enables our agent to make multiple tool calls in sequence, iteratively building its solution. It allows the agent to:
- Analyze project requirements.
- Search for employees with specific skills.
- Evaluate different team compositions.
- Save the chosen team to the database.
- Generate a structured recommendation.
Without maxSteps
, we would need to manually code each step of this process, making our implementation much more rigid and less adaptable to different kinds of projects.
The complete HR team-building workflow
Let's visualize the entire workflow to understand how the different components work together:
The workflow starts when the HR manager submits a project description. This kicks off a series of steps:
- Project Analysis: The agent analyzes the project description to extract required skills and their importance.
- Skill Matching: For each required skill, the agent performs a vector search to find matching employees.
- Team Composition: The agent evaluates different combinations of employees to form potential teams.
- Team Analysis: Each potential team is analyzed for skill coverage, diversity, and past collaboration.
- Team Selection: The agent selects the optimal team based on the analysis.
- Database Storage: The selected team is saved to the database for future reference.
- Recommendation Generation: A structured recommendation with justification is returned to the HR manager.
- Team Approval: The HR manager can review and approve the recommended team.
- Status Tracking: The team's status is updated as it moves through the project lifecycle.
This end-to-end process creates a seamless experience for HR managers, from initial request to team tracking.
Bringing it all together: A practical example
Let's see our HR team-building agent in action with a real-world scenario. Imagine you're an HR manager at a fintech company, and you receive the following project request:
"We need to build a new customer portal for our wealth management platform. The portal needs to have a modern React front end with sophisticated data visualization components, connect to our MongoDB database, and implement OAuth 2.0 for secure authentication. We also need someone familiar with financial regulations and compliance. The project timeline is tight—we need to launch in 2 months. This is a high-visibility project for our top clients."
Step 1: Analyze project requirements
First, the agent calls analyzeProjectRequirements
to extract structured information:
// Agent's first tool call
{
"tool": "analyzeProjectRequirements",
"parameters": {
"projectDescription": "We need to build a new customer portal for our wealth management platform. The portal needs to have a modern React frontend with sophisticated data visualization components, connect to our MongoDB database, and implement OAuth 2.0 for secure authentication. We also need someone familiar with financial regulations and compliance. The project timeline is tight—we need to launch in 2 months. This is a high-visibility project for our top clients."
}
}
The tool returns:
{
"requiredSkills": [
{ "skill": "React", "importance": 5 },
{ "skill": "Data Visualization", "importance": 4 },
{ "skill": "MongoDB", "importance": 4 },
{ "skill": "OAuth 2.0", "importance": 4 },
{ "skill": "Financial Regulations", "importance": 3 },
{ "skill": "UI/UX for Financial Applications", "importance": 3 }
],
"estimatedTeamSize": 4,
"timeline": "2 months",
"specialRequirements": [
"High visibility for top clients",
"Financial compliance expertise",
"Tight deadline"
]
}
Step 2: Search for key skills
Based on the requirements analysis, the agent starts searching for employees with the necessary skills:
// Agent's second tool call
{
"tool": "searchEmployeesBySkill",
"parameters": {
"skillDescription": "Modern React development with data visualization experience",
"minProficiency": 4,
"minAvailability": 40
}
}
MongoDB Vector Search returns several candidates with relevant frontend skills:
[
{
"_id": "60a2c1e04f5bc12345678901",
"name": "Alex Chen",
"title": "Senior Frontend Developer",
"department": "Engineering",
"availability": 75,
"relevantSkills": [
{
"name": "React",
"proficiency": 5,
"yearsExperience": 4
},
{
"name": "D3.js",
"proficiency": 4,
"yearsExperience": 3
}
],
"similarity": 0.92
},
// ... more results
]
The agent continues searching for other required skills:
// Searching for MongoDB expertise
{
"tool": "searchEmployeesBySkill",
"parameters": {
"skillDescription": "MongoDB database experience for financial applications",
"minProficiency": 3,
"minAvailability": 30
}
}
// Searching for authentication expertise
{
"tool": "searchEmployeesBySkill",
"parameters": {
"skillDescription": "OAuth 2.0 implementation and security protocols",
"minProficiency": 4,
"minAvailability": 30
}
}
// Searching for financial compliance expertise
{
"tool": "searchEmployeesBySkill",
"parameters": {
"skillDescription": "Financial regulations and compliance for wealth management",
"minProficiency": 3,
"minAvailability": 20
}
}
Step 3: Evaluate team compositions
After gathering potential candidates for each skill area, the agent begins evaluating possible team compositions:
// Agent evaluates a potential team
{
"tool": "analyzeTeamComposition",
"parameters": {
"teamMembers": [
"60a2c1e04f5bc12345678901", // Alex - React/D3.js
"60a2c1e04f5bc12345678903", // Sam - MongoDB
"60a2c1e04f5bc12345678905", // Riley - Security/OAuth
"60a2c1e04f5bc12345678907" // Jordan - Financial compliance
],
"requiredSkills": [
{ "skill": "React", "importance": 5 },
{ "skill": "Data Visualization", "importance": 4 },
{ "skill": "MongoDB", "importance": 4 },
{ "skill": "OAuth 2.0", "importance": 4 },
{ "skill": "Financial Regulations", "importance": 3 },
{ "skill": "UI/UX for Financial Applications", "importance": 3 }
]
}
}
The tool returns an analysis:
{
"skillCoverage": 91,
"diversityScore": 76,
"collaborationHistory": {
"previousCollaborations": 2,
"averageSuccessScore": 4.0
},
"overallScore": 85,
"analysis": "This team of 4 members has excellent skill coverage. The team includes 3 high-performing members with strong track records. The team spans 3 different departments, which brings diverse perspectives but may require additional coordination. This team composition is highly recommended."
}
The agent might try a few different team compositions, adding or removing team members to balance skill coverage, collaboration history, and team size.
Step 4: Save team to database
After evaluating different options, the agent saves the chosen team to the database:
// Agent's database tool call
{
"tool": "saveTeamToDatabase",
"parameters": {
"projectTitle": "Wealth Management Customer Portal",
"projectDescription": "We need to build a new customer portal for our wealth management platform...",
"teamMembers": [
{
"id": "60a2c1e04f5bc12345678901",
"name": "Alex Chen",
"role": "Frontend Lead",
"keySkills": ["React", "D3.js", "Frontend Architecture"],
"justification": "Alex brings 4 years of React experience and strong data visualization skills with D3.js, which are critical for the financial dashboard components."
},
// ... other team members
],
"skillCoverage": 91,
"overallRationale": "This team combines strong technical skills in all required areas with crucial domain expertise in financial regulations. The inclusion of Morgan with both design and compliance knowledge allows us to keep the team size efficient while ensuring the interface meets regulatory requirements.",
"risks": [
"Tight 2-month timeline for a complex financial application",
"High visibility increases pressure for a polished product"
],
"mitigationStrategies": [
"Start with compliance and security architecture to avoid late-stage rework",
"Implement phased delivery with core features first",
"Schedule weekly demos with key stakeholders for early feedback"
]
}
}
The database operation returns:
{
"success": true,
"teamId": "60a2c1e04f5bc12345678999",
"message": "Team \"Wealth Management Customer Portal\" has been saved to the database with 4 members.",
"status": "proposed"
}
Step 5: Generate final recommendation
Finally, the agent provides a structured recommendation:
// Agent's final tool call
{
"tool": "generateTeamRecommendation",
"parameters": {
"projectTitle": "Wealth Management Customer Portal",
"projectDescription": "We need to build a new customer portal for our wealth management platform...",
"teamMembers": [
{
"id": "60a2c1e04f5bc12345678901",
"name": "Alex Chen",
"role": "Frontend Lead",
"keySkills": ["React", "D3.js", "Frontend Architecture"],
"justification": "Alex brings 4 years of React experience and strong data visualization skills with D3.js, which are critical for the financial dashboard components. Previous leadership experience on similar projects."
},
{
"id": "60a2c1e04f5bc12345678903",
"name": "Sam Patel",
"role": "Backend Developer",
"keySkills": ["MongoDB", "Node.js", "API Design"],
"justification": "Sam has extensive experience with MongoDB in financial contexts and has worked with Alex before, which will streamline the frontend-backend integration."
},
{
"id": "60a2c1e04f5bc12345678905",
"name": "Riley Johnson",
"role": "Security Specialist",
"keySkills": ["OAuth 2.0", "Security Protocols", "Compliance"],
"justification": "Riley's expertise in OAuth 2.0 and security is essential for protecting sensitive financial data. Has implemented similar authentication systems for other financial products."
},
{
"id": "60a2c1e04f5bc12345678909",
"name": "Morgan Lee",
"role": "UI/UX Designer & Financial Compliance",
"keySkills": ["UI/UX Design", "Financial Regulations", "User Research"],
"justification": "Morgan brings the dual expertise of UI/UX design specifically for financial applications and knowledge of financial regulations. This combination is rare and valuable for ensuring the portal is both compliant and user-friendly."
}
],
"overallRationale": "This team combines strong technical skills in all required areas with crucial domain expertise in financial regulations. The inclusion of Morgan with both design and compliance knowledge allows us to keep the team size efficient while ensuring the interface meets regulatory requirements. Alex and Sam's previous successful collaboration will help meet the tight timeline.",
"skillCoverage": 94,
"risks": [
"Tight 2-month timeline for a complex financial application",
"High visibility increases pressure for a polished product"
],
"mitigationStrategies": [
"Start with compliance and security architecture to avoid late-stage rework",
"Implement phased delivery with core features first",
"Schedule weekly demos with key stakeholders for early feedback",
"Consider adding a QA specialist for the final testing phase"
]
}
}
The user interface
To make this technology accessible to HR professionals, we've created a simple web interface:
https://github.com/mongodb-developer/GenAI-Showcase/tree/main/apps/vercel_sdk_hr_agent/app
Conclusion
The HR team-building agent we've developed demonstrates the significant advantages of integrating MongoDB Vector Search with Vercel AI SDK's agentic capabilities and Voyage AI embeddings. By representing skills as nuanced vectors within a semantic space, this solution empowers HR professionals to navigate the complex, multi-dimensional challenge of building effective teams.
This approach marks a transformative shift from rigid, keyword-based HR systems toward dynamic, adaptive tools capable of iterative reasoning and context-sensitive decision-making. To maximize the effectiveness of this solution in your organization, consider the following implementation tips:
- Start with quality data: The success of vector search hinges on detailed, context-rich skill descriptions. Enrich employee profiles with information on how skills were practically applied in previous projects.
- Consider skill contexts: Generate embeddings that encapsulate not just skill names but also proficiency levels, experience, and relevant usage contexts, creating richer and more accurate vector representations.
- Optimize MongoDB indexes: For optimal performance in large-scale deployments, fine-tune vector search indexes by experimenting with parameters such as
numCandidates
and similarity thresholds. - Balance tool complexity: Design agent tools that maintain a balance between specificity and flexibility, enabling AI-driven agents to adapt effectively while preserving utility.
- Incorporate feedback loops: Establish mechanisms that allow HR professionals to provide feedback on recommendations. Continuous learning from such feedback ensures progressively improved and refined team-building suggestions.
Ultimately, the true strength of this HR team-building agent lies in its ability to complement and amplify human expertise rather than replace it. The fusion of AI's semantic understanding and computational precision with the emotional intelligence and strategic insights of HR professionals creates an innovative, collaborative environment for more insightful team formation and effective project management.
Try MongoDB Atlas today, Happy team building!