From Idea to 1,000+ Stars: How Laravel Workflow Took Off
I was working at a fintech company, wrestling with long-running processes that felt like a constant headache. We were using queued jobs that polled to check if an earlier step had completed, requeueing themselves if it hadn’t. It worked, but it was noisy, hard to track, and, honestly, felt wrong. I knew there had to be a better way. So, I went hunting for a solution. That’s when I stumbled across Temporal. Their PHP SDK was a breath of fresh air. You could write workflows like regular PHP code, yield async steps, and the system would magically resume where it left off. It was elegant, and the developer experience felt right. I was sold. Then I pitched it to the DevOps team. And hit a brick wall. They stared at me like I’d suggested launching a spaceship to run PHP jobs. Temporal required a Kubernetes cluster or a subscription to their cloud service. “Why would we add all this complexity just to replace Laravel queues?” they asked. I didn’t have a great answer. Deep down, I knew they had a point. We already had Laravel queues. We had a database. Couldn’t we build something similar without the ceremony? So, I rolled up my sleeves and got to work. Inspired by Temporal’s PHP SDK, I kept the core idea of using yield as a coroutine checkpoint but threw out the heavy stuff, no server, no cluster, no cloud. Now a workflow is just a queued job that runs until it hits a yield. When the yielded job completes, it requeues the workflow, rebuilds the state, and picks up where it left off. Here’s a quick example of how it looks in practice: class PaymentWorkflow extends Workflow { public function execute($orderId) { $order = yield ActivityStub::make(ReserveOrder::class, $orderId); $payment = yield ActivityStub::make(ProcessPayment::class, $order->id); yield ActivityStub::make(NotifyCustomer::class, $payment->id); return $payment; } } This workflow reserves an order, processes a payment, and notifies the customer, with each step running asynchronously. If any step fails or needs retrying, the workflow resumes seamlessly. No polling, no mess. That was the proof of concept. It worked. We shipped a basic version to production, and it handled our workflows like a charm. But I couldn’t stop tinkering. It felt like a puzzle I had to solve. I added support for side effects, sagas, and child workflows, making it more powerful while keeping it dead simple. I shared the private repo with an engineer I’d befriended at Temporal (after pestering him with way too many questions). He took a look and said, “Wow, this is really cool. You should release it.” So, I did. I wrote docs, opened the repo, and tagged a Composer release. I called it Laravel Workflow. Then something clicked with the community: Temporal isn’t built for PHP devs like us. Don’t get me wrong. Temporal’s fantastic for massive, distributed systems at enterprise scale, like powering Netflix or Uber. But their PHP SDK, while solid, isn’t their priority. It even requires Roadrunner, an extra runtime on top of their server. That’s friction most Laravel devs don’t need. Meanwhile, Laravel Workflow is all about simplicity: No external services. No custom runtimes. Just Laravel queues, a database, and yield. Fast forward, and Laravel Workflow has quietly become the default for long-running orchestration in the Laravel community. It’s racked up over 1,000 stars on GitHub, climbed to the top of Google for terms like “Laravel workflow orchestration,” and even covers my domain fees through GitHub Sponsors. Temporal’s PHP SDK still has 4-5x more daily installs, but they’ve got $350 million in funding and a massive marketing machine. I’m just a guy with a repo and a passion for making PHP dev feel right. The only “downside”? I don’t have a shiny SaaS to sell. There’s no cloud product, no upsell. Laravel Workflow is just a tool that solves a problem, and I’m okay with that. But I’m not done yet. I’m dreaming up new features, exploring deeper Laravel integrations, and loving the community that’s rallying around this project. If you’re a Laravel dev struggling with long-running processes, give Laravel Workflow a try. Check out the GitHub repo, kick the tires, or share your own workflow war stories. Let’s keep building tools that make development fun, simple, and powerful.
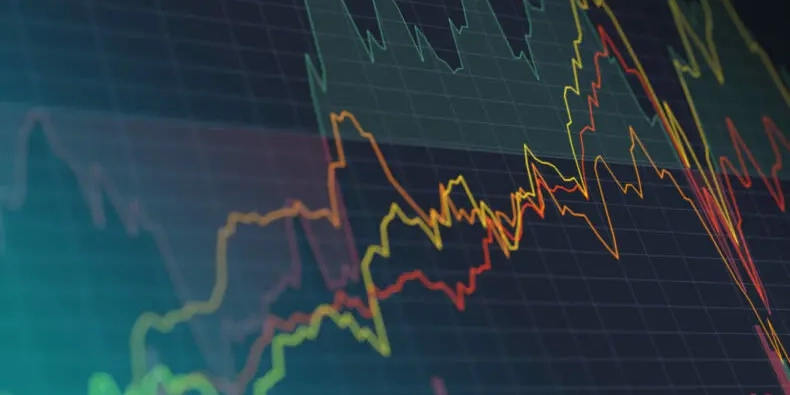
I was working at a fintech company, wrestling with long-running processes that felt like a constant headache. We were using queued jobs that polled to check if an earlier step had completed, requeueing themselves if it hadn’t. It worked, but it was noisy, hard to track, and, honestly, felt wrong. I knew there had to be a better way.
So, I went hunting for a solution.
That’s when I stumbled across Temporal. Their PHP SDK was a breath of fresh air. You could write workflows like regular PHP code, yield async steps, and the system would magically resume where it left off. It was elegant, and the developer experience felt right. I was sold.
Then I pitched it to the DevOps team.
And hit a brick wall.
They stared at me like I’d suggested launching a spaceship to run PHP jobs. Temporal required a Kubernetes cluster or a subscription to their cloud service. “Why would we add all this complexity just to replace Laravel queues?” they asked. I didn’t have a great answer. Deep down, I knew they had a point.
We already had Laravel queues. We had a database. Couldn’t we build something similar without the ceremony?
So, I rolled up my sleeves and got to work.
Inspired by Temporal’s PHP SDK, I kept the core idea of using yield as a coroutine checkpoint but threw out the heavy stuff, no server, no cluster, no cloud.
Now a workflow is just a queued job that runs until it hits a yield. When the yielded job completes, it requeues the workflow, rebuilds the state, and picks up where it left off.
Here’s a quick example of how it looks in practice:
class PaymentWorkflow extends Workflow
{
public function execute($orderId)
{
$order = yield ActivityStub::make(ReserveOrder::class, $orderId);
$payment = yield ActivityStub::make(ProcessPayment::class, $order->id);
yield ActivityStub::make(NotifyCustomer::class, $payment->id);
return $payment;
}
}
This workflow reserves an order, processes a payment, and notifies the customer, with each step running asynchronously. If any step fails or needs retrying, the workflow resumes seamlessly. No polling, no mess.
That was the proof of concept. It worked. We shipped a basic version to production, and it handled our workflows like a charm.
But I couldn’t stop tinkering.
It felt like a puzzle I had to solve. I added support for side effects, sagas, and child workflows, making it more powerful while keeping it dead simple.
I shared the private repo with an engineer I’d befriended at Temporal (after pestering him with way too many questions). He took a look and said, “Wow, this is really cool. You should release it.”
So, I did. I wrote docs, opened the repo, and tagged a Composer release. I called it Laravel Workflow.
Then something clicked with the community: Temporal isn’t built for PHP devs like us.
Don’t get me wrong. Temporal’s fantastic for massive, distributed systems at enterprise scale, like powering Netflix or Uber. But their PHP SDK, while solid, isn’t their priority. It even requires Roadrunner, an extra runtime on top of their server. That’s friction most Laravel devs don’t need.
Meanwhile, Laravel Workflow is all about simplicity:
No external services.
No custom runtimes.
Just Laravel queues, a database, and yield.
Fast forward, and Laravel Workflow has quietly become the default for long-running orchestration in the Laravel community. It’s racked up over 1,000 stars on GitHub, climbed to the top of Google for terms like “Laravel workflow orchestration,” and even covers my domain fees through GitHub Sponsors.
Temporal’s PHP SDK still has 4-5x more daily installs, but they’ve got $350 million in funding and a massive marketing machine. I’m just a guy with a repo and a passion for making PHP dev feel right.
The only “downside”? I don’t have a shiny SaaS to sell. There’s no cloud product, no upsell. Laravel Workflow is just a tool that solves a problem, and I’m okay with that.
But I’m not done yet. I’m dreaming up new features, exploring deeper Laravel integrations, and loving the community that’s rallying around this project.
If you’re a Laravel dev struggling with long-running processes, give Laravel Workflow a try. Check out the GitHub repo, kick the tires, or share your own workflow war stories. Let’s keep building tools that make development fun, simple, and powerful.