How to Correctly Read a Bash Array from a File
Introduction In this guide, we'll tackle the challenge of reading an array from a file in Bash, addressing a common issue that many users face. You're not alone in feeling like this should be a simple task. Many people struggle with getting their array elements parsed correctly when reading from a file instead of declaring them directly in the script. We aim to clarify this process and help you resolve any issues you're experiencing. Understanding the Issue The core problem here arises from how Bash handles whitespace when reading from files and processing strings. When you attempt to read elements into an array using methods like command substitution or xargs, Bash separates values based on whitespace. This behavior can lead to unexpected results when your elements contain spaces, ultimately resulting in elements getting split improperly. Solution: Reading Array Elements Correctly To set your array correctly from a file while maintaining the intended structure, you can utilize quoting techniques combined with proper splitting commands. Step 1: Create Your Input File First, we need to create the input file that contains your array elements. The contents of this file must ensure that all elements appear on a single line, which you've already indicated is a requirement. In your code snippet, you were on the right track with using a here-document (EOF). Here's how you can adapt it correctly: # Create input file with array elements cat $tmpFile 'Debian Linux' 'Redhat Linux' 'Ubuntu Linux' EOF Step 2: Reading from the File into an Array Now, to read the contents of the file into an array, you can use a combination of mapfile or readarray, depending on your version of Bash. This method ensures that the elements are stored as whole pieces, preserving the intended values. Here’s the command: # Reading from the file into an array readarray -d ' ' -t ARRAY_2 < $tmpFile Here the -d ' ' option specifies a delimiter. However, since we need to preserve spaces in the strings, you'll be better served using the following method, which treats each line as a whole: # Correctly creating an array from file contents while IFS= read -r line; do ARRAY_2+=("$line") done < $tmpFile Step 3: Printing the Array Elements After populating the array successfully, let's echo the elements to verify that everything works as expected: # Verify ARRAY_2 contents echo ARRAY_2: ${ARRAY_2[@]} NUM_ELEMENTS=${#ARRAY_2[@]} for (( i=0; i < NUM_ELEMENTS; i++ )); do echo ${ARRAY_2[i]} done Complete Example Here’s a complete, corrected example that integrates all the above: #!/bin/bash # Declare array with 4 elements directly ARRAY_1=( 'Debian Linux' 'Redhat Linux' 'Ubuntu Linux' ) # Contents of ARRAY_1 echo ARRAY_1: ${ARRAY_1[@]} # Get number of elements in the array NUM_ELEMENTS=${#ARRAY_1[@]} # echo each element in the array for (( i=0; i < NUM_ELEMENTS; i++ )); do echo ${ARRAY_1[i]} done # Prepare to read from a file echo echo Now try with reading from a file. echo The goal is to behave like ARRAY_1 tmpFile=/tmp/TEST_ELEMENTS.$$ cat $tmpFile 'Debian Linux' 'Redhat Linux' 'Ubuntu Linux' EOF # Read from file into an array while IFS= read -r line; do ARRAY_2+=("$line") done < $tmpFile # Verify the results echo ARRAY_2: ${ARRAY_2[@]} NUM_ELEMENTS=${#ARRAY_2[@]} for (( i=0; i < NUM_ELEMENTS; i++ )); do echo ${ARRAY_2[i]} done # Clean up m $tmpFile Conclusion Now you've successfully created a Bash script that reads array elements from a file without splitting any strings unintentionally. Utilizing proper techniques ensures your arrays maintain fidelity, making your Bash scripting much more robust. Frequently Asked Questions 1. What is the difference between readarray and while read? readarray is a specialized command to read lines into an array but works best for line-based input. The while read approach gives you more control over processing, especially with complex data. 2. Why is my array splitting elements? This occurs when whitespace is used as a separator in command substitutions or when reading without quoting strings correctly. Always use double quotes when dealing with variables that might contain spaces. 3. Can I read multiple lines into a single array element? Yes, by reading and appending each line properly, you can preserve the multiple lines in single array entries as shown in the example above.
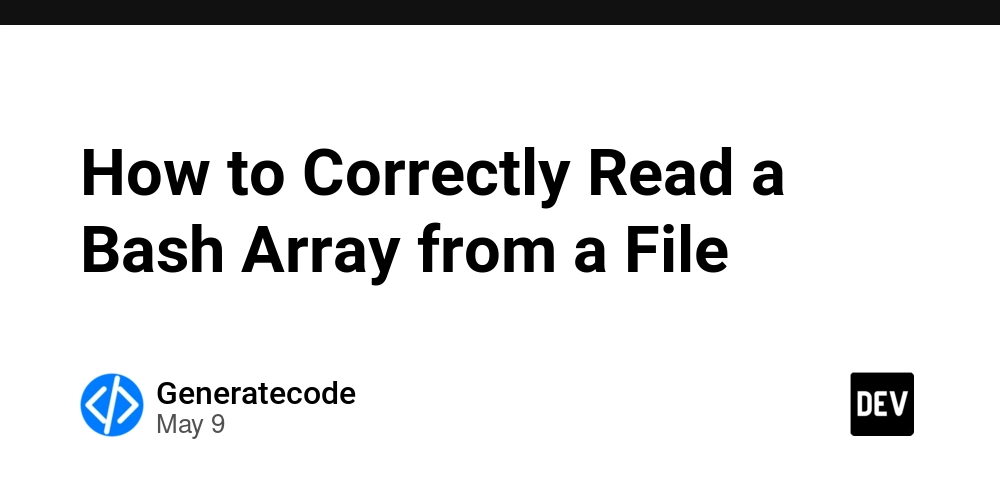
Introduction
In this guide, we'll tackle the challenge of reading an array from a file in Bash, addressing a common issue that many users face. You're not alone in feeling like this should be a simple task. Many people struggle with getting their array elements parsed correctly when reading from a file instead of declaring them directly in the script. We aim to clarify this process and help you resolve any issues you're experiencing.
Understanding the Issue
The core problem here arises from how Bash handles whitespace when reading from files and processing strings. When you attempt to read elements into an array using methods like command substitution or xargs, Bash separates values based on whitespace. This behavior can lead to unexpected results when your elements contain spaces, ultimately resulting in elements getting split improperly.
Solution: Reading Array Elements Correctly
To set your array correctly from a file while maintaining the intended structure, you can utilize quoting techniques combined with proper splitting commands.
Step 1: Create Your Input File
First, we need to create the input file that contains your array elements. The contents of this file must ensure that all elements appear on a single line, which you've already indicated is a requirement.
In your code snippet, you were on the right track with using a here-document (EOF). Here's how you can adapt it correctly:
# Create input file with array elements
cat << EOF > $tmpFile
'Debian Linux' 'Redhat Linux' 'Ubuntu Linux'
EOF
Step 2: Reading from the File into an Array
Now, to read the contents of the file into an array, you can use a combination of mapfile
or readarray
, depending on your version of Bash. This method ensures that the elements are stored as whole pieces, preserving the intended values. Here’s the command:
# Reading from the file into an array
readarray -d ' ' -t ARRAY_2 < $tmpFile
Here the -d ' '
option specifies a delimiter. However, since we need to preserve spaces in the strings, you'll be better served using the following method, which treats each line as a whole:
# Correctly creating an array from file contents
while IFS= read -r line; do
ARRAY_2+=("$line")
done < $tmpFile
Step 3: Printing the Array Elements
After populating the array successfully, let's echo the elements to verify that everything works as expected:
# Verify ARRAY_2 contents
echo ARRAY_2: ${ARRAY_2[@]}
NUM_ELEMENTS=${#ARRAY_2[@]}
for (( i=0; i < NUM_ELEMENTS; i++ )); do
echo ${ARRAY_2[i]}
done
Complete Example
Here’s a complete, corrected example that integrates all the above:
#!/bin/bash
# Declare array with 4 elements directly
ARRAY_1=( 'Debian Linux' 'Redhat Linux' 'Ubuntu Linux' )
# Contents of ARRAY_1
echo ARRAY_1: ${ARRAY_1[@]}
# Get number of elements in the array
NUM_ELEMENTS=${#ARRAY_1[@]}
# echo each element in the array
for (( i=0; i < NUM_ELEMENTS; i++ )); do
echo ${ARRAY_1[i]}
done
# Prepare to read from a file
echo
echo Now try with reading from a file.
echo The goal is to behave like ARRAY_1
tmpFile=/tmp/TEST_ELEMENTS.$$
cat << EOF > $tmpFile
'Debian Linux' 'Redhat Linux' 'Ubuntu Linux'
EOF
# Read from file into an array
while IFS= read -r line; do
ARRAY_2+=("$line")
done < $tmpFile
# Verify the results
echo ARRAY_2: ${ARRAY_2[@]}
NUM_ELEMENTS=${#ARRAY_2[@]}
for (( i=0; i < NUM_ELEMENTS; i++ )); do
echo ${ARRAY_2[i]}
done
# Clean up
m $tmpFile
Conclusion
Now you've successfully created a Bash script that reads array elements from a file without splitting any strings unintentionally. Utilizing proper techniques ensures your arrays maintain fidelity, making your Bash scripting much more robust.
Frequently Asked Questions
1. What is the difference between readarray
and while read
?
readarray
is a specialized command to read lines into an array but works best for line-based input. The while read
approach gives you more control over processing, especially with complex data.
2. Why is my array splitting elements?
This occurs when whitespace is used as a separator in command substitutions or when reading without quoting strings correctly. Always use double quotes when dealing with variables that might contain spaces.
3. Can I read multiple lines into a single array element?
Yes, by reading and appending each line properly, you can preserve the multiple lines in single array entries as shown in the example above.