JavaScript Best Practices for Beginners
JavaScript Best Practices for Beginners JavaScript is one of the most popular programming languages in the world, powering over 98% of all websites as a client-side language. Whether you're building interactive web applications, dynamic user interfaces, or server-side applications with Node.js, following best practices will help you write cleaner, more efficient, and maintainable code. If you're looking to make money with your web programming skills, consider checking out MillionFormula, a platform that helps developers monetize their expertise. Now, let’s dive into essential JavaScript best practices for beginners. 1. Use const and let Instead of var ES6 introduced const and let, which provide block-scoping and help avoid common pitfalls associated with var. const: Use for variables that won’t be reassigned. let: Use for variables that will change. javascriptCopyDownload// Bad var name = "John"; var age = 25; // Good const name = "John"; let age = 25; Avoid var because it is function-scoped, which can lead to unintended behavior in loops and conditionals. 2. Follow Consistent Naming Conventions Meaningful variable and function names improve readability. Use camelCase for variables and functions. Use PascalCase for constructor functions and classes. Use UPPER_SNAKE_CASE for constants. javascriptCopyDownload// Bad let firstname = "John"; function getuser() {} // Good let firstName = "John"; function getUser() {} // Constants const MAX_USERS = 100; 3. Avoid Global Variables Global variables can cause naming collisions and make debugging difficult. Instead, use modules or closures to encapsulate logic. javascriptCopyDownload// Bad (pollutes global scope) let counter = 0; // Better (encapsulated) (function() { let counter = 0; function increment() { counter++; } })(); Modern JavaScript projects use ES6 modules for better organization: javascriptCopyDownload// utils.js export function formatDate(date) { return new Date(date).toLocaleString(); } // app.js import { formatDate } from './utils.js'; 4. Use Strict Mode ('use strict') Strict mode helps catch common mistakes by throwing errors for unsafe actions like: Assigning to undeclared variables. Using deprecated features. javascriptCopyDownload'use strict'; x = 10; // Throws an error (x is not defined) Enable it at the top of your scripts or functions. 5. Always Use === Instead of == The strict equality operator (===) avoids type coercion, leading to more predictable comparisons. javascriptCopyDownload// Bad (type coercion) if (5 == "5") { console.log("True"); // Outputs True } // Good (strict comparison) if (5 === "5") { console.log("False"); // Not executed } 6. Handle Errors with try-catch Unhandled errors can crash your application. Use try-catch for error-prone operations like API calls. javascriptCopyDownloadasync function fetchData() { try { const response = await fetch('https://api.example.com/data'); const data = await response.json(); console.log(data); } catch (error) { console.error("Fetch failed:", error); } } For frontend logging, consider tools like Sentry. 7. Avoid Callback Hell with Promises & Async/Await Nested callbacks lead to "callback hell". Instead, use: Promises Async/Await javascriptCopyDownload// Callback Hell (Bad) getUser(userId, (user) => { getPosts(user.id, (posts) => { getComments(posts[0].id, (comments) => { console.log(comments); }); }); }); // Async/Await (Good) async function loadData() { const user = await getUser(userId); const posts = await getPosts(user.id); const comments = await getComments(posts[0].id); console.log(comments); } 8. Optimize Loops for Performance Avoid heavy computations inside loops. Cache array lengths and use efficient loops like for...of or Array methods. javascriptCopyDownloadconst items = [1, 2, 3, 4, 5]; // Bad (recalculates length each iteration) for (let i = 0; i Hello, ${name}; // Destructuring const { id, username } = user; // Spread Operator const merged = [...arr1, ...arr2]; 10. Use ESLint & Prettier for Code Quality Automate code formatting and error-checking with: ESLint (Catches errors) Prettier (Auto-formats code) Install them via npm: bashCopyDownloadnpm install eslint prettier --save-dev Configure them in your project for consistent style. Final Thoughts Following these best practices will help you write cleaner, more maintainable, and bug-free JavaScript. As you improve, consider exploring advanced topics like design patterns, performance optimization, and testing. And if you're looking to turn your coding skills into income, check out MillionFormula for opportunities to monetize your expertise. Happy coding!
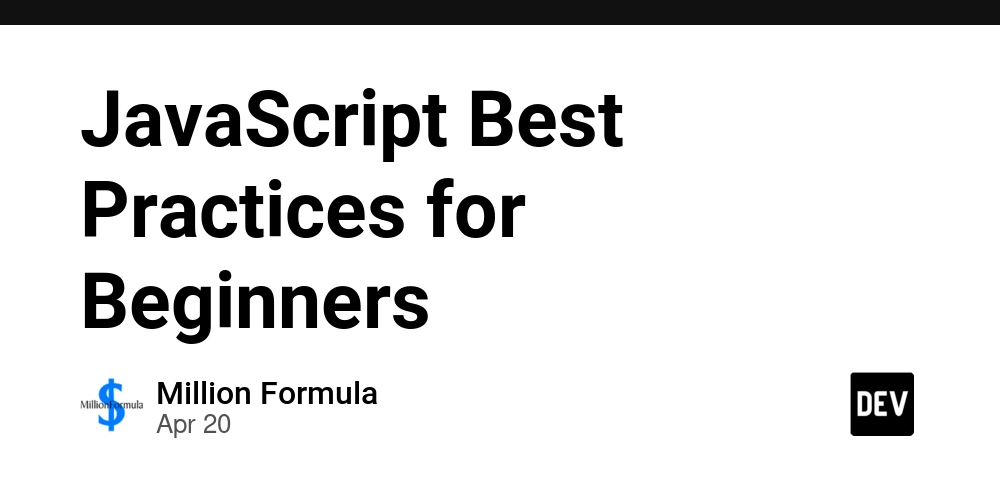
JavaScript Best Practices for Beginners
JavaScript is one of the most popular programming languages in the world, powering over 98% of all websites as a client-side language. Whether you're building interactive web applications, dynamic user interfaces, or server-side applications with Node.js, following best practices will help you write cleaner, more efficient, and maintainable code.
If you're looking to make money with your web programming skills, consider checking out MillionFormula, a platform that helps developers monetize their expertise.
Now, let’s dive into essential JavaScript best practices for beginners.
1. Use const
and let
Instead of var
ES6 introduced const
and let
, which provide block-scoping and help avoid common pitfalls associated with var
.
const
: Use for variables that won’t be reassigned.let
: Use for variables that will change.
// Bad
var name = "John";
var age = 25;// Good
const name = "John";
let age = 25;
Avoid var
because it is function-scoped, which can lead to unintended behavior in loops and conditionals.
2. Follow Consistent Naming Conventions
Meaningful variable and function names improve readability.
Use camelCase for variables and functions.
Use PascalCase for constructor functions and classes.
Use UPPER_SNAKE_CASE for constants.
// Bad
let firstname = "John";
function getuser() {}// Good
let firstName = "John";
function getUser() {}// Constants
const MAX_USERS = 100;
3. Avoid Global Variables
Global variables can cause naming collisions and make debugging difficult. Instead, use modules or closures to encapsulate logic. javascriptCopyDownload
// Bad (pollutes global scope)
let counter = 0;// Better (encapsulated)
(function() {
let counter = 0;
function increment() {
counter++;
}
})();
Modern JavaScript projects use ES6 modules for better organization: javascriptCopyDownload
// utils.js
export function formatDate(date) {
return new Date(date).toLocaleString();
}// app.js
import { formatDate } from './utils.js';
4. Use Strict Mode ('use strict'
)
Strict mode helps catch common mistakes by throwing errors for unsafe actions like:
Assigning to undeclared variables.
Using deprecated features.
'use strict';x = 10; // Throws an error (x is not defined)
Enable it at the top of your scripts or functions.
5. Always Use ===
Instead of ==
The strict equality operator (===
) avoids type coercion, leading to more predictable comparisons.
javascriptCopyDownload
// Bad (type coercion)
if (5 == "5") {
console.log("True"); // Outputs True
}// Good (strict comparison)
if (5 === "5") {
console.log("False"); // Not executed
}
6. Handle Errors with try-catch
Unhandled errors can crash your application. Use try-catch
for error-prone operations like API calls.
javascriptCopyDownload
async function fetchData() {
try {
const response = await fetch('https://api.example.com/data');
const data = await response.json();
console.log(data);
} catch (error) {
console.error("Fetch failed:", error);
}
}
For frontend logging, consider tools like Sentry.
7. Avoid Callback Hell with Promises & Async/Await
Nested callbacks lead to "callback hell". Instead, use:
Promises
Async/Await
// Callback Hell (Bad)
getUser(userId, (user) => {
getPosts(user.id, (posts) => {
getComments(posts[0].id, (comments) => {
console.log(comments);
});
});
});// Async/Await (Good)
async function loadData() {
const user = await getUser(userId);
const posts = await getPosts(user.id);
const comments = await getComments(posts[0].id);
console.log(comments);
}
8. Optimize Loops for Performance
Avoid heavy computations inside loops. Cache array lengths and use efficient loops like for...of
or Array methods
.
javascriptCopyDownload
const items = [1, 2, 3, 4, 5];// Bad (recalculates length each iteration)
for (let i = 0; i < items.length; i++) {
console.log(items[i]);
}// Good (cached length)
for (let i = 0, len = items.length; i < len; i++) {
console.log(items[i]);
}// Best (modern JS)
for (const item of items) {
console.log(item);
}// Functional approach (map, filter, reduce)
items.map(item => console.log(item));
9. Leverage Modern JavaScript Features
Use ES6+ features for cleaner code:
Arrow Functions
Template Literals
Destructuring
Spread/Rest Operators
// Arrow Functions
const greet = (name) =>Hello, ${name};
// Destructuring
const { id, username } = user;// Spread Operator
const merged = [...arr1, ...arr2];
10. Use ESLint & Prettier for Code Quality
Automate code formatting and error-checking with:
Install them via npm: bashCopyDownload
npm install eslint prettier --save-dev
Configure them in your project for consistent style.
Final Thoughts
Following these best practices will help you write cleaner, more maintainable, and bug-free JavaScript. As you improve, consider exploring advanced topics like design patterns, performance optimization, and testing.
And if you're looking to turn your coding skills into income, check out MillionFormula for opportunities to monetize your expertise.
Happy coding!