Stringable toInteger method
I just read a toInteger article. And it feels like a lazy contribution for me. My solution is; /** * 0x or 0X indicates heximal * 0 indicates octal * 0b or 0B indicates binary */ public function prefixToInteger() { return intval($this->value, 0); } public function heximalToInteger() { return intval($this->value, 16); } public function decimalToInteger() { return intval($this->value, 10); } public function octalToInteger() { return intval($this->value, 8); } public function binaryToInteger() { return intval($this->value, 2); } When you take the effort to wrap a native function, I go all the way to make it as readable as possible. In the example this example is given. $data = 'Sensor: reading=0x4B, status=0x03'; $reading = Str::of($data) ->between('reading=', ',') // Extract "0x4B" ->replaceFirst('0x', '') // Remove "0x" prefix ->toInteger(16); I would clean that up with my solution to $reading = Str::of($data) ->between('reading=', ',') // Extract "0x4B" ->prefixToInteger(); What do you think? Am I going overboard?
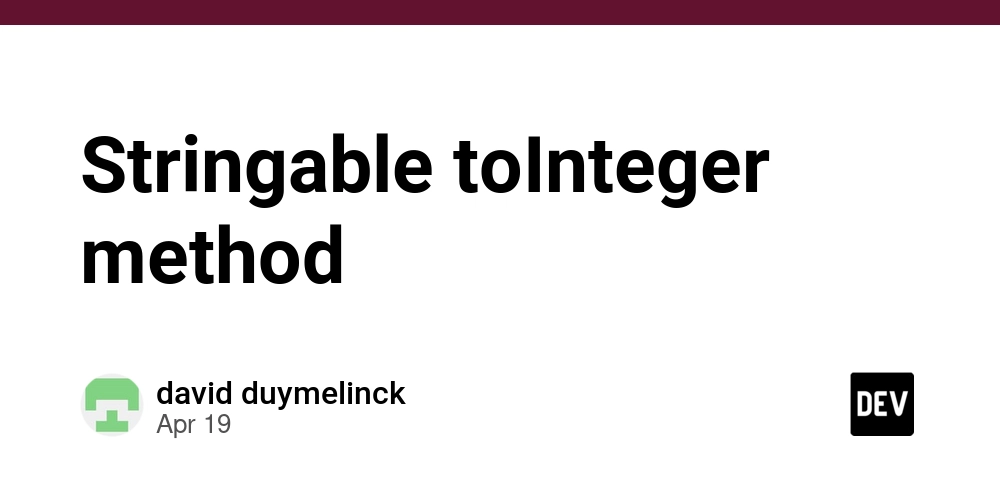
I just read a toInteger article. And it feels like a lazy contribution for me.
My solution is;
/**
* 0x or 0X indicates heximal
* 0 indicates octal
* 0b or 0B indicates binary
*/
public function prefixToInteger()
{
return intval($this->value, 0);
}
public function heximalToInteger()
{
return intval($this->value, 16);
}
public function decimalToInteger()
{
return intval($this->value, 10);
}
public function octalToInteger()
{
return intval($this->value, 8);
}
public function binaryToInteger()
{
return intval($this->value, 2);
}
When you take the effort to wrap a native function, I go all the way to make it as readable as possible.
In the example this example is given.
$data = 'Sensor: reading=0x4B, status=0x03';
$reading = Str::of($data)
->between('reading=', ',') // Extract "0x4B"
->replaceFirst('0x', '') // Remove "0x" prefix
->toInteger(16);
I would clean that up with my solution to
$reading = Str::of($data)
->between('reading=', ',') // Extract "0x4B"
->prefixToInteger();
What do you think? Am I going overboard?