Applying MVC Architecture in Game Development
This post is an excerpt from the book Learning Game Architecture with Unity. Check my other books at Amazon. Selecting the right architecture for a game project is crucial for its success and maintainability. Depending on the project's scope, complexity, and goals, several architectural approaches can be considered, mentioned as follows: No architecture: For simpler projects or prototypes, a formal architecture might be overkill. In these cases, keeping things straightforward without imposing a structured framework can be practical. This approach allows for rapid development and iteration, ideal for small-scale projects where complexity is minimal, and requirements are likely to change frequently. Custom architecture: As projects grow in size and complexity, a custom architecture might be designed to address specific needs. This approach allows developers to create a tailored solution that fits the unique requirements of the project. However, designing a custom architecture requires careful planning and consideration to ensure it effectively manages the complexity and scales with the project. Proven architectures: For larger and more complex projects, leveraging established architectural patterns can be highly beneficial. Proven architectures like MVC, MVP, and MVVM provide well-defined frameworks that promote separation of concerns, modularity, and maintainability. These architectures are tested and refined, offering a solid foundation for managing intricate game mechanics and interactions. MVC is one of the most widely adopted architectural patterns, known for its effective separation of concerns and flexibility. By exploring MVC, we will understand how it organizes game components into distinct layers, Model, View, and Controller, and how this separation enhances code maintainability and scalability. Understanding MVC/MVCS architecture The MVC architecture is a classic design pattern used to separate concerns in software development, making it easier to manage and scale applications. Let us break down the key areas of concern in MVC and its application in game development: Model: The Model represents the data and the business logic of the application. It manages the state of the game, handles data storage, and processes game logic. In a game, the Model could be responsible for managing player statistics, game state, and other core data. View: The View is responsible for rendering the user interface and presenting the data from the Model to the user. It displays information such as game graphics, user interfaces, and other visual elements. In Unity, the View typically corresponds to MonoBehaviours that handle visual aspects and render updates based on the data provided by the Model. Controller: The Controller handles the input from the user, processes it, and updates the Model accordingly. It acts as an intermediary between the View and the Model, ensuring that user interactions are translated into changes in the game state. In classic MVC, the Controller directly handles user input and updates the Model. MVC in Unity In Unity, the roles of View and Controller are slightly adapted due to the engine's architecture and design. Here is how Unity handles these components: View: In Unity, MonoBehaviours are often used for handling user input and rendering. The View is responsible for capturing user interactions, such as button clicks or movement inputs, and updating the game’s visual representation accordingly. This approach leverages Unity’s component-based architecture to manage game visuals and inputs directly. Controller: Instead of handling input directly, the Controller in Unity processes the input captured by the View. The Controller takes the user input from the View and translates it into actions that modify the Model. This separation allows for a more modular design where input handling and game logic are distinctly managed. From Classic MVC to MVCS Modern games often communicate with servers and external services to handle additional data and functionalities. To accommodate these needs, the classic MVC architecture is extended to MVCS, where an additional layer, Services, is introduced. Here is how MVCS enhances MVC: Services layer: This layer interacts with backend services, external APIs, or databases to manage external data and server communication. It handles tasks such as fetching game data from a server, managing user accounts, or processing in-game transactions. Integration with MVC: In the MVCS architecture, the Services layer works alongside the Model, View, and Controller. The Controller interacts with the Services layer to retrieve or send data, which then updates the Model. The Model reflects these changes in the View, ensuring that the game remains synchronized with external data sources. The new architecture with the Service layer added is shown below: It is essential to highlight that in an MVC archit
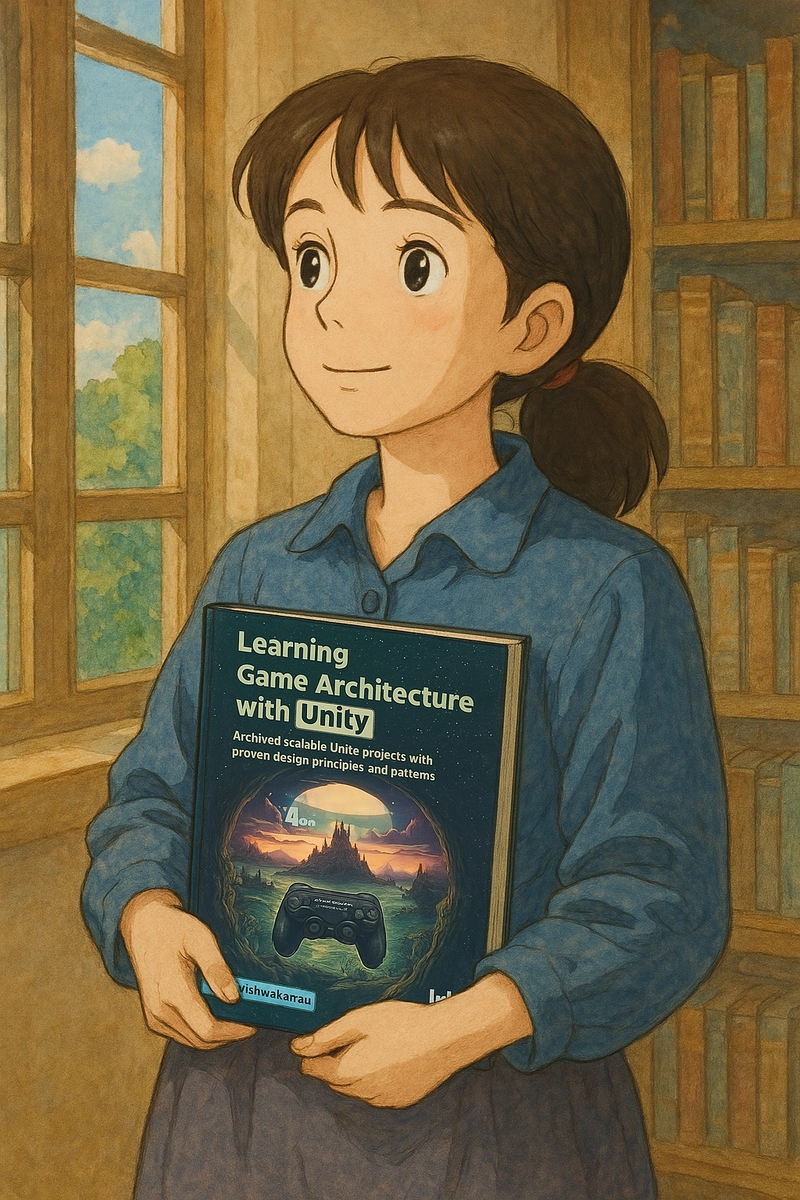
This post is an excerpt from the book Learning Game Architecture with Unity. Check my other books at Amazon.
Selecting the right architecture for a game project is crucial for its success and maintainability. Depending on the project's scope, complexity, and goals, several architectural approaches can be considered, mentioned as follows:
- No architecture: For simpler projects or prototypes, a formal architecture might be overkill. In these cases, keeping things straightforward without imposing a structured framework can be practical. This approach allows for rapid development and iteration, ideal for small-scale projects where complexity is minimal, and requirements are likely to change frequently.
- Custom architecture: As projects grow in size and complexity, a custom architecture might be designed to address specific needs. This approach allows developers to create a tailored solution that fits the unique requirements of the project. However, designing a custom architecture requires careful planning and consideration to ensure it effectively manages the complexity and scales with the project.
- Proven architectures: For larger and more complex projects, leveraging established architectural patterns can be highly beneficial. Proven architectures like MVC, MVP, and MVVM provide well-defined frameworks that promote separation of concerns, modularity, and maintainability. These architectures are tested and refined, offering a solid foundation for managing intricate game mechanics and interactions.
MVC is one of the most widely adopted architectural patterns, known for its effective separation of concerns and flexibility. By exploring MVC, we will understand how it organizes game components into distinct layers, Model, View, and Controller, and how this separation enhances code maintainability and scalability.
Understanding MVC/MVCS architecture
The MVC architecture is a classic design pattern used to separate concerns in software development, making it easier to manage and scale applications. Let us break down the key areas of concern in MVC and its application in game development:
- Model: The Model represents the data and the business logic of the application. It manages the state of the game, handles data storage, and processes game logic. In a game, the Model could be responsible for managing player statistics, game state, and other core data.
- View: The View is responsible for rendering the user interface and presenting the data from the Model to the user. It displays information such as game graphics, user interfaces, and other visual elements. In Unity, the View typically corresponds to MonoBehaviours that handle visual aspects and render updates based on the data provided by the Model.
- Controller: The Controller handles the input from the user, processes it, and updates the Model accordingly. It acts as an intermediary between the View and the Model, ensuring that user interactions are translated into changes in the game state. In classic MVC, the Controller directly handles user input and updates the Model.
MVC in Unity
In Unity, the roles of View and Controller are slightly adapted due to the engine's architecture and design. Here is how Unity handles these components:
- View: In Unity, MonoBehaviours are often used for handling user input and rendering. The View is responsible for capturing user interactions, such as button clicks or movement inputs, and updating the game’s visual representation accordingly. This approach leverages Unity’s component-based architecture to manage game visuals and inputs directly.
- Controller: Instead of handling input directly, the Controller in Unity processes the input captured by the View. The Controller takes the user input from the View and translates it into actions that modify the Model. This separation allows for a more modular design where input handling and game logic are distinctly managed.
From Classic MVC to MVCS
Modern games often communicate with servers and external services to handle additional data and functionalities. To accommodate these needs, the classic MVC architecture is extended to MVCS, where an additional layer, Services, is introduced. Here is how MVCS enhances MVC:
- Services layer: This layer interacts with backend services, external APIs, or databases to manage external data and server communication. It handles tasks such as fetching game data from a server, managing user accounts, or processing in-game transactions.
- Integration with MVC: In the MVCS architecture, the Services layer works alongside the Model, View, and Controller. The Controller interacts with the Services layer to retrieve or send data, which then updates the Model. The Model reflects these changes in the View, ensuring that the game remains synchronized with external data sources.
The new architecture with the Service layer added is shown below:
It is essential to highlight that in an MVC architecture within Unity, there can be multiple Models, Views, and Controllers. This flexibility allows each component to handle different aspects of the game separately. For instance, you might have a PlayerModel, EnemyModel, and WeaponModel, each with corresponding Views and Controllers. This modularity not only keeps your codebase clean and organized but also enables you to expand or modify individual components without affecting the entire system. This setup is particularly beneficial in complex games where different systems need to interact while remaining decoupled. Refer to the following figure:
This separation not only made the codebase more manageable but also allowed for easier testing and future modifications. Each component could be developed and tested independently, reducing the risk of introducing bugs when making changes. For example, the developer could easily adjust the firing logic in the Controller without affecting the weapon's visual representation in the view.
Application of MVC architecture has been covered with great details in the book: Learning Game Architecture with Unity, which is available @ Amazon, Amazon India, BPB International, BPB India.