HarmonyOS NEXT Development Case: Converting Numbers to Chinese Numerals
// Import necessary modules import { promptAction } from '@kit.ArkUI'; // For displaying toast messages import { pasteboard } from '@kit.BasicServicesKit'; // Clipboard operations import { toChineseNumber } from '@nutpi/chinese-finance-number'; // Convert numbers to Chinese financial uppercase import { toChineseWithUnits, // Convert numbers to Chinese with units toUpperCase, // Convert Chinese lowercase to uppercase } from '@nutpi/chinese-number-format'; @Entry // Mark as entry component @Component // Define component struct NumberToChineseConverter { @State private exampleNumber: number = 88.8; // Default example number @State private textColor: string = "#2e2e2e"; // Primary text color @State private lineColor: string = "#d5d5d5"; // Divider color @State private basePadding: number = 30; // Base padding value @State private chineseLowercase: string = ""; // Lowercase Chinese result @State private chineseUppercase: string = ""; // Uppercase Chinese result @State private chineseUppercaseAmount: string = ""; // Financial uppercase result @State @Watch('inputChanged') private inputText: string = ""; // Watched input field // Handle input changes inputChanged() { this.chineseLowercase = toChineseWithUnits(Number(this.inputText), 'zh-CN'); this.chineseUppercase = toUpperCase(this.chineseLowercase, 'zh-CN'); this.chineseUppercaseAmount = toChineseNumber(Number(this.inputText)); } // Copy text to clipboard private copyToClipboard(text: string): void { const pasteboardData = pasteboard.createData(pasteboard.MIMETYPE_TEXT_PLAIN, text); const systemPasteboard = pasteboard.getSystemPasteboard(); systemPasteboard.setData(pasteboardData); promptAction.showToast({ message: 'Copied' }); } // UI construction build() { Column() { // Header section Text('Number to Chinese Converter') .fontColor(this.textColor) .fontSize(18) .width('100%') .height(50) .textAlign(TextAlign.Center) .backgroundColor(Color.White) .shadow({ radius: 2, color: this.lineColor, offsetX: 0, offsetY: 5 }); Scroll() { Column() { // Introduction section Column() { Text('Tool Introduction').fontSize(20).fontWeight(600).fontColor(this.textColor); Text('Convert numbers to Chinese formats for financial documents, contracts, and reports. Supports conversion from "cent" to "trillion".') .textAlign(TextAlign.JUSTIFY) .fontSize(18).fontColor(this.textColor).margin({ top: `${this.basePadding / 2}lpx` }); } .alignItems(HorizontalAlign.Start) .width('650lpx') .padding(`${this.basePadding}lpx`) .margin({ top: `${this.basePadding}lpx` }) .borderRadius(10) .backgroundColor(Color.White) .shadow({ radius: 10, color: this.lineColor }); // Input section Column() { Row() { Text('Example') .fontColor("#5871ce") .fontSize(18) .padding(`${this.basePadding / 2}lpx`) .backgroundColor("#f2f1fd") .borderRadius(5) .clickEffect({ level: ClickEffectLevel.LIGHT, scale: 0.8 }) .onClick(() => { this.inputText = `${this.exampleNumber}`; }); Blank(); Text('Clear') .fontColor("#e48742") .fontSize(18) .padding(`${this.basePadding / 2}lpx`) .clickEffect({ level: ClickEffectLevel.LIGHT, scale: 0.8 }) .backgroundColor("#ffefe6") .borderRadius(5) .onClick(() => { this.inputText = ""; }); }.height(45).justifyContent(FlexAlign.SpaceBetween); Divider().margin({ top: 5, bottom: 5 }); TextInput({ text: $$this.inputText, placeholder: `Enter number, e.g.: ${this.exampleNumber}` }) .width('100%') .fontSize(18) .caretColor(this.textColor) .margin({ top: `${this.basePadding}lpx` }) .type(InputType.NUMBER_DECIMAL); } .width('650lpx') .padding(`${this.basePadding}lpx`) .margin({ top: `${this.basePadding}lpx` }) .backgroundColor(Color.White) .shadow({ radius: 10, color: this.lineColor }); // Result section Column() { // ... (similar structure for result rows with copy buttons) } .width('650lpx') .padding(`${this.basePadding}lpx`) .margin({ top: `${this.basePadding}lpx` }) .backgroundColor(Color.White) .shadow({ radius: 10, color: this.lineColor }); } }.scrollBar(BarState.Off); } .height('100%') .width('100%') .backgroundColor("#f4f8fb"); } } Technical Implement
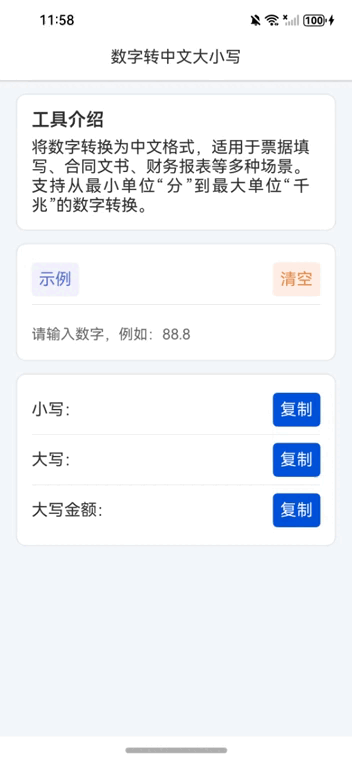
// Import necessary modules
import { promptAction } from '@kit.ArkUI'; // For displaying toast messages
import { pasteboard } from '@kit.BasicServicesKit'; // Clipboard operations
import { toChineseNumber } from '@nutpi/chinese-finance-number'; // Convert numbers to Chinese financial uppercase
import {
toChineseWithUnits, // Convert numbers to Chinese with units
toUpperCase, // Convert Chinese lowercase to uppercase
} from '@nutpi/chinese-number-format';
@Entry // Mark as entry component
@Component // Define component
struct NumberToChineseConverter {
@State private exampleNumber: number = 88.8; // Default example number
@State private textColor: string = "#2e2e2e"; // Primary text color
@State private lineColor: string = "#d5d5d5"; // Divider color
@State private basePadding: number = 30; // Base padding value
@State private chineseLowercase: string = ""; // Lowercase Chinese result
@State private chineseUppercase: string = ""; // Uppercase Chinese result
@State private chineseUppercaseAmount: string = ""; // Financial uppercase result
@State @Watch('inputChanged') private inputText: string = ""; // Watched input field
// Handle input changes
inputChanged() {
this.chineseLowercase = toChineseWithUnits(Number(this.inputText), 'zh-CN');
this.chineseUppercase = toUpperCase(this.chineseLowercase, 'zh-CN');
this.chineseUppercaseAmount = toChineseNumber(Number(this.inputText));
}
// Copy text to clipboard
private copyToClipboard(text: string): void {
const pasteboardData = pasteboard.createData(pasteboard.MIMETYPE_TEXT_PLAIN, text);
const systemPasteboard = pasteboard.getSystemPasteboard();
systemPasteboard.setData(pasteboardData);
promptAction.showToast({ message: 'Copied' });
}
// UI construction
build() {
Column() {
// Header section
Text('Number to Chinese Converter')
.fontColor(this.textColor)
.fontSize(18)
.width('100%')
.height(50)
.textAlign(TextAlign.Center)
.backgroundColor(Color.White)
.shadow({
radius: 2,
color: this.lineColor,
offsetX: 0,
offsetY: 5
});
Scroll() {
Column() {
// Introduction section
Column() {
Text('Tool Introduction').fontSize(20).fontWeight(600).fontColor(this.textColor);
Text('Convert numbers to Chinese formats for financial documents, contracts, and reports. Supports conversion from "cent" to "trillion".')
.textAlign(TextAlign.JUSTIFY)
.fontSize(18).fontColor(this.textColor).margin({ top: `${this.basePadding / 2}lpx` });
}
.alignItems(HorizontalAlign.Start)
.width('650lpx')
.padding(`${this.basePadding}lpx`)
.margin({ top: `${this.basePadding}lpx` })
.borderRadius(10)
.backgroundColor(Color.White)
.shadow({ radius: 10, color: this.lineColor });
// Input section
Column() {
Row() {
Text('Example')
.fontColor("#5871ce")
.fontSize(18)
.padding(`${this.basePadding / 2}lpx`)
.backgroundColor("#f2f1fd")
.borderRadius(5)
.clickEffect({ level: ClickEffectLevel.LIGHT, scale: 0.8 })
.onClick(() => { this.inputText = `${this.exampleNumber}`; });
Blank();
Text('Clear')
.fontColor("#e48742")
.fontSize(18)
.padding(`${this.basePadding / 2}lpx`)
.clickEffect({ level: ClickEffectLevel.LIGHT, scale: 0.8 })
.backgroundColor("#ffefe6")
.borderRadius(5)
.onClick(() => { this.inputText = ""; });
}.height(45).justifyContent(FlexAlign.SpaceBetween);
Divider().margin({ top: 5, bottom: 5 });
TextInput({ text: $$this.inputText, placeholder: `Enter number, e.g.: ${this.exampleNumber}` })
.width('100%')
.fontSize(18)
.caretColor(this.textColor)
.margin({ top: `${this.basePadding}lpx` })
.type(InputType.NUMBER_DECIMAL);
}
.width('650lpx')
.padding(`${this.basePadding}lpx`)
.margin({ top: `${this.basePadding}lpx` })
.backgroundColor(Color.White)
.shadow({ radius: 10, color: this.lineColor });
// Result section
Column() {
// ... (similar structure for result rows with copy buttons)
}
.width('650lpx')
.padding(`${this.basePadding}lpx`)
.margin({ top: `${this.basePadding}lpx` })
.backgroundColor(Color.White)
.shadow({ radius: 10, color: this.lineColor });
}
}.scrollBar(BarState.Off);
}
.height('100%')
.width('100%')
.backgroundColor("#f4f8fb");
}
}
Technical Implementation Overview
1. Core Conversion Logic
- toChineseWithUnits: Converts numbers to colloquial Chinese with units (e.g., 88.8 → 八十八点八)
- toUpperCase: Transforms lowercase Chinese numbers to formal uppercase (e.g., 八 → 捌)
- toChineseNumber: Specialized financial conversion following official accounting standards
2. State Management
-
@State
decorators manage UI-reactive variables -
@Watch
observes input changes to trigger conversions - Three conversion results maintain independent states for optimal rendering
3. UI Features
- Responsive Layout: Adaptive design for multiple screen sizes
-
Visual Feedback:
- Click effects with scaling animation
- Toast notifications for copy operations
- Shadow effects for depth perception
-
Input Validation:
- Restricted to decimal numbers
- Automatic conversion handling
4. Clipboard Integration
- System pasteboard API usage
- MIME type handling for text data
- Error-safe data packaging
Usage Scenarios
- Financial document preparation
- Legal contract writing
- Invoice processing systems
- Educational tools for Chinese numerals
- Cross-cultural business applications
This implementation demonstrates HarmonyOS NEXT's capabilities in handling:
- Reactive UI development
- Native service integration
- Complex number formatting
- Cross-cultural localization requirements
Developers can extend this pattern to implement other regional number formatting requirements while maintaining the core architecture.