How to create page in Next.js
page.tsx File-based routing is introduced in Next.js from v13. That means the file's name is the route name. If we create a page.tsx into the app directory, the page.tsx will create the default route.This is known as the home route. If you visit http://localhost:3000/ you can see the Home component there. File structure: └── app/ └── page.tsx Again if we create any directory under app directory,this will create a new route. The name of the route is the of the file itself. Like we want to create a new route about. User can see the about component or page if he/she navigate to http://localhost:3000/about └── app/ ├── about/ │ └── page.tsx └── page.tsx import React from 'react'; const AboutPage: React.FC = () => { return ( About Us Welcome to our website! We are a team of passionate individuals dedicated to creating amazing things. Our mission is to provide high-quality services and products that meet the needs of our customers. We believe in innovation, collaboration, and continuous improvement. ); }; export default AboutPage; Now user see the AboutPage component or page if he/she navigate to http://localhost:3000/about NB: Naming Convention: Use all lowercase letters for directory names to follow best practices. To create a route we need a folder. The folder name is the route. Inside that folder, add a special file named page.tsx, page.jsx, or page.js. This file contains the component that will render when the route is accessed. Now we are able to create any route for level one. But what about http://localhost:3000/products/mobile/samsung Nested Routes Imagine the situation, where the user can navigate to the home page, and clicking on a product will navigate to the products page. Clicking on a product from the products page, the user will navigate to the specific product (mobile) route. Further, if the user wants to see product(mobile) details user will be navigated to another route(samsung). How can we do this? It is very simple in Next.js. You should think simply. Step 01: We know to create a route we need a directory. The directory name must be matched with the route name. Step 02: Into app directory there is a page.tsx file that will create the home route. http//:localhost:3000/ Step 03: Nested Routes For every nested routes, we also need a nested directory. In our case, our route is .../products/mobile/samsung. This means need a directory in app directory named products. Then create another directory in products directory named mobile. In the same way, create another directory named samsung into mobile directory. └── app/ ├── about/ │ └── page.tsx ├── page.tsx └── products/ └── mobile/ └── samsung/ We just created the routes http://localhost:3000/products/mobile/samsung. Step 04: page.tsx However, the user can not access the routes. If any user tries to access the routes he/she will be directed to 404 | Not Found page. To make it publically accessible we need a special file page.tsx for every nested route. Into the page.tsx file you can render your desired component. └── app/ ├── about/ │ └── page.tsx ├── page.tsx └── products/ ├── mobile/ │ ├── page.tsx │ └── samsung/ │ └── page.tsx └── page.tsx Now users can get access to the routes. If the user navigates http://localhost:3000/products user can see products page. By navigating http://localhost:3000/products/mobile users have access to this routes. Finally use have access for http://localhost:3000/products/mobile/samsung routes.
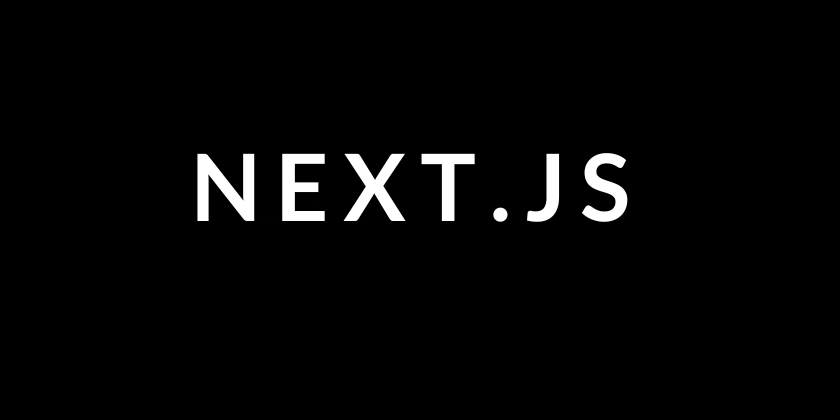
page.tsx
File-based routing is introduced in Next.js from v13. That means the file's name is the route name.
If we create a page.tsx into the app directory, the page.tsx will create the default route.This is known as the home route. If you visit http://localhost:3000/ you can see the Home component there.
File structure:
└── app/
└── page.tsx
Again if we create any directory under app directory,this will create a new route. The name of the route is the of the file itself. Like we want to create a new route about. User can see the about component or page if he/she navigate to http://localhost:3000/about
└── app/
├── about/
│ └── page.tsx
└── page.tsx
import React from 'react';
const AboutPage: React.FC = () => {
return (
About Us
Welcome to our website! We are a team of passionate individuals dedicated to creating amazing things.
Our mission is to provide high-quality services and products that meet the needs of our customers.
We believe in innovation, collaboration, and continuous improvement.
);
};
export default AboutPage;
Now user see the AboutPage component or page if he/she navigate to http://localhost:3000/about
NB: Naming Convention: Use all lowercase letters for directory names to follow best practices.
To create a route we need a folder. The folder name is the route. Inside that folder, add a special file named page.tsx, page.jsx, or page.js. This file contains the component that will render when the route is accessed.
Now we are able to create any route for level one. But what about http://localhost:3000/products/mobile/samsung
Nested Routes
Imagine the situation, where the user can navigate to the home page, and clicking on a product will navigate to the products page. Clicking on a product from the products page, the user will navigate to the specific product (mobile) route. Further, if the user wants to see product(mobile) details user will be navigated to another route(samsung). How can we do this?
It is very simple in Next.js. You should think simply.
Step 01: We know to create a route we need a directory. The directory name must be matched with the route name.
Step 02: Into app
directory there is a page.tsx
file that will create the home route. http//:localhost:3000/
Step 03: Nested Routes
For every nested routes, we also need a nested directory. In our case, our route is .../products/mobile/samsung. This means need a directory in app
directory named products
. Then create another directory in products
directory named mobile
. In the same way, create another directory named samsung
into mobile
directory.
└── app/
├── about/
│ └── page.tsx
├── page.tsx
└── products/
└── mobile/
└── samsung/
We just created the routes http://localhost:3000/products/mobile/samsung.
Step 04: page.tsx
However, the user can not access the routes. If any user tries to access the routes he/she will be directed to 404 | Not Found page. To make it publically accessible we need a special file page.tsx
for every nested route. Into the page.tsx
file you can render your desired component.
└── app/
├── about/
│ └── page.tsx
├── page.tsx
└── products/
├── mobile/
│ ├── page.tsx
│ └── samsung/
│ └── page.tsx
└── page.tsx
Now users can get access to the routes.
If the user navigates http://localhost:3000/products user can see products
page.
By navigating http://localhost:3000/products/mobile users have access to this routes. Finally use have access for http://localhost:3000/products/mobile/samsung routes.