JAVA - CONSTRUCTOR & METHOD CHAINING
Constructor and Method Chaining in Java Constructor Chaining Constructor chaining is the process of calling one constructor from another constructor within the same class or from the parent class. Types of Constructor Chaining Within the same class (using this()) From parent class (using super()) 1. Same Class Constructor Chaining (this()) public class Student { private String name; private int age; private String university; // Default constructor public Student() { this("Unknown", 0); // Calls 2-arg constructor } // 2-arg constructor public Student(String name, int age) { this(name, age, "MIT"); // Calls 3-arg constructor } // 3-arg constructor (main constructor) public Student(String name, int age, String university) { this.name = name; this.age = age; this.university = university; } } Key Points: this() must be the first statement in the constructor Used to avoid code duplication Helps in providing multiple ways to initialize objects 2. Parent Class Constructor Chaining (super()) class Person { private String name; public Person(String name) { this.name = name; } } class Employee extends Person { private int empId; public Employee(String name, int empId) { super(name); // Calls parent class constructor this.empId = empId; } } Key Points: super() must be the first statement in the constructor If not written explicitly, compiler inserts super() (calling no-arg parent constructor) If parent doesn't have no-arg constructor and child doesn't call any super constructor explicitly, compilation error occurs Method Chaining Method chaining is a technique where multiple methods are called in a single statement by having each method return an object (often this). Example of Method Chaining public class StringBuilderExample { private StringBuilder sb = new StringBuilder(); public StringBuilderExample append(String str) { sb.append(str); return this; // returns current object } public StringBuilderExample appendLine(String str) { sb.append(str).append("\n"); return this; } public String build() { return sb.toString(); } } // Usage: String result = new StringBuilderExample() .append("Hello") .appendLine(" World!") .append("Java") .build(); Built-in Examples of Method Chaining StringBuilder/StringBuffer String str = new StringBuilder() .append("Hello") .append(" ") .append("World") .toString(); Java 8 Streams List filtered = list.stream() .filter(s -> s.startsWith("A")) .map(String::toUpperCase) .collect(Collectors.toList()); Constructor vs Method Chaining Feature Constructor Chaining Method Chaining Purpose Initialize object state Perform multiple operations Keyword used this(), super() Return this from methods Order dependency Must be first statement No strict order requirement Return type No return type Returns object (often this) Inheritance role Crucial for parent-child relation Typically within same class Best Practices Constructor Chaining: Keep chain simple (avoid long chains) Put the most complete constructor at the end of the chain Document which constructor is the "main" one Method Chaining: Ensure each method in chain maintains object in valid state Consider making the class immutable if appropriate Clearly document if methods modify state or return new instances Common Pitfalls: Forgetting that this() or super() must be first statement Creating infinite loops in constructor chains Method chaining that makes debugging harder (combine with proper logging)
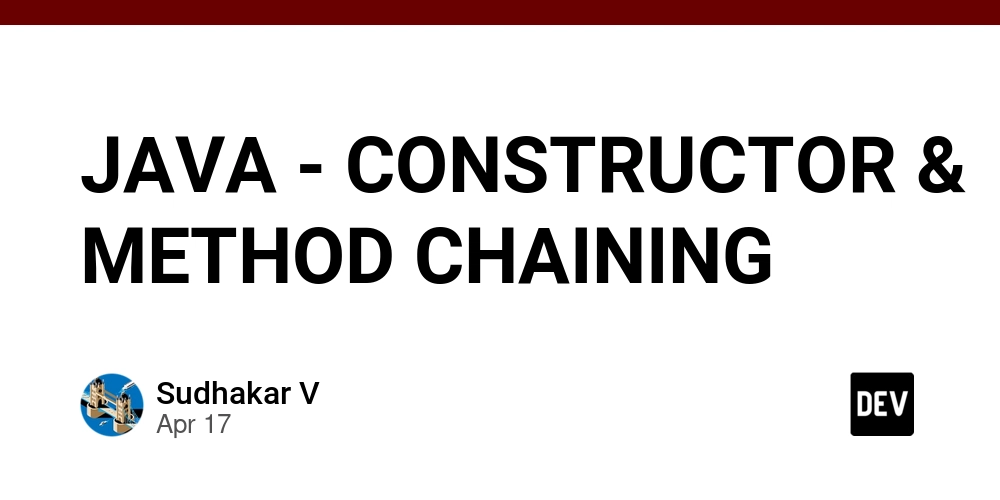
Constructor and Method Chaining in Java
Constructor Chaining
Constructor chaining is the process of calling one constructor from another constructor within the same class or from the parent class.
Types of Constructor Chaining
- Within the same class (using
this()
) - From parent class (using
super()
)
1. Same Class Constructor Chaining (this()
)
public class Student {
private String name;
private int age;
private String university;
// Default constructor
public Student() {
this("Unknown", 0); // Calls 2-arg constructor
}
// 2-arg constructor
public Student(String name, int age) {
this(name, age, "MIT"); // Calls 3-arg constructor
}
// 3-arg constructor (main constructor)
public Student(String name, int age, String university) {
this.name = name;
this.age = age;
this.university = university;
}
}
Key Points:
-
this()
must be the first statement in the constructor - Used to avoid code duplication
- Helps in providing multiple ways to initialize objects
2. Parent Class Constructor Chaining (super()
)
class Person {
private String name;
public Person(String name) {
this.name = name;
}
}
class Employee extends Person {
private int empId;
public Employee(String name, int empId) {
super(name); // Calls parent class constructor
this.empId = empId;
}
}
Key Points:
-
super()
must be the first statement in the constructor - If not written explicitly, compiler inserts
super()
(calling no-arg parent constructor) - If parent doesn't have no-arg constructor and child doesn't call any super constructor explicitly, compilation error occurs
Method Chaining
Method chaining is a technique where multiple methods are called in a single statement by having each method return an object (often this
).
Example of Method Chaining
public class StringBuilderExample {
private StringBuilder sb = new StringBuilder();
public StringBuilderExample append(String str) {
sb.append(str);
return this; // returns current object
}
public StringBuilderExample appendLine(String str) {
sb.append(str).append("\n");
return this;
}
public String build() {
return sb.toString();
}
}
// Usage:
String result = new StringBuilderExample()
.append("Hello")
.appendLine(" World!")
.append("Java")
.build();
Built-in Examples of Method Chaining
- StringBuilder/StringBuffer
String str = new StringBuilder()
.append("Hello")
.append(" ")
.append("World")
.toString();
- Java 8 Streams
List<String> filtered = list.stream()
.filter(s -> s.startsWith("A"))
.map(String::toUpperCase)
.collect(Collectors.toList());
Constructor vs Method Chaining
Feature | Constructor Chaining | Method Chaining |
---|---|---|
Purpose | Initialize object state | Perform multiple operations |
Keyword used |
this() , super()
|
Return this from methods |
Order dependency | Must be first statement | No strict order requirement |
Return type | No return type | Returns object (often this ) |
Inheritance role | Crucial for parent-child relation | Typically within same class |
Best Practices
-
Constructor Chaining:
- Keep chain simple (avoid long chains)
- Put the most complete constructor at the end of the chain
- Document which constructor is the "main" one
-
Method Chaining:
- Ensure each method in chain maintains object in valid state
- Consider making the class immutable if appropriate
- Clearly document if methods modify state or return new instances
-
Common Pitfalls:
- Forgetting that
this()
orsuper()
must be first statement - Creating infinite loops in constructor chains
- Method chaining that makes debugging harder (combine with proper logging)
- Forgetting that