JavaScript var vs let vs const: Understanding the Differences and Best Practices
In JavaScript, variables are fundamental building blocks. With the introduction of ES6, developers gained more options - let and const - alongside the traditional var. Understanding the distinctions between these declarations is crucial for writing clean, efficient, and bug-free code. Regardless of whether you are new to programming or not, knowing how var, let, and const operate can help you avoid a lot of common mistakes and write better JavaScript. This complete guide covers the differences in detail, provides real world examples, best practices, and answers to frequently asked questions. The Evolution of Variable Declarations in JavaScript The Era of var Initially, JavaScript offered only the var keyword for variable declarations. While functional, var has characteristics that can lead to unintended behaviors, especially in larger codebases. For instance, its function-scoped nature and support for re-declarations make it less predictable. Former to ES6, developers tended to rely on patterns and conventions to mimic block scoping or immutability, such as wrapping variables in IIFEs (Immediately Invoked Function Expressions). These types of solutions demonstrated the need for an improvement in variable declaration methods. The Advent of let and const To solve some of the challenges that could be faced with var, ES6 (ECMAScript 2015) added let and const, which provide developers better control of variable scope and mutability. The addition of let and const fundamentally changed the way that JavaScript handles variables, and they are regarded as a best practice. In-Depth Look at var Characteristics of var: Function Scope: Variables declared with var are confined to the function in which they're declared, not the block. javascript function example() { if (true) { var x = 10; } console.log(x); // Outputs: 10 } example(); Hoisting: var declarations are hoisted to the top of their scope and initialized with undefined. This can lead to bugs if the developer assumes the variable is available only after the declaration line. javascript console.log(y); // Outputs: undefined var y = 5; Re-declaration: Variables declared with var can be re-declared within the same scope without errors. javascript var z = 1; var z = 2; console.log(z); // Outputs: 2 Exploring let Characteristics of let: Block Scope: let confines variables to the block in which they're declared, reducing the risk of accidental overwrites and scope leakage. javascript function test() { if (true) { let a = 20; console.log(a); // Outputs: 20 } console.log(a); // ReferenceError: a is not defined } test(); Temporal Dead Zone (TDZ): Variables declared with let are hoisted but not initialized until their definition is evaluated, making it easier to catch bugs at runtime. javascript console.log(b); // ReferenceError: Cannot access 'b' before initialization let b = 15; No Re-declaration: let does not allow re-declaration within the same scope, enforcing better coding practices. javascript let c = 3; let c = 4; // SyntaxError: Identifier 'c' has already been declared Ideal for Loops: let is often preferred in loop constructs because it provides block scope. javascript for (let i = 0; i < 5; i++) { setTimeout(() => console.log(i), 100); } Understanding const Characteristics of const: Block Scope: Similar to let, const is block-scoped. Immutability: Variables declared with const cannot be reassigned after their initial assignment. This doesn't mean the value itself is immutable—objects and arrays declared with const can still be mutated. javascript const PI = 3.14159; PI = 3.14; // TypeError: Assignment to constant variable. Mutable Contents: The contents of a const object can be modified. javascript const arr = [1, 2, 3]; arr.push(4); // Works fine console.log(arr); // Outputs: [1, 2, 3, 4] You can even escape and unescape JSON data stored in const objects dynamically. Use Cases: const is ideal for values that should never change, like configuration settings, API endpoints, or constant references. 5. var vs let vs const: A Comparative Overview Scope var is function-scoped. python jsCopyEditfunction test() { var x = 10; } console.log(x); // Error: x is not defined let and const are block-scoped. python jsCopyEdit{ let y = 20; const z = 30; } console.log(y); // Error console.log(z); // Error Hoisting var is hoisted and initialized as undefined. python jsCopyEditconsole.log(a); // undefined var a = 5; let and const are hoisted but not initialized — accessing them before declaration throws an error (Temporal Dead Zone). python jsCopyEditconsole.log(b); // ReferenceError let b = 10; Re-declaration var allows re-declaration. python jsCopyEditvar a = 1; var a = 2; // No error let and const don’t allow it. pyth
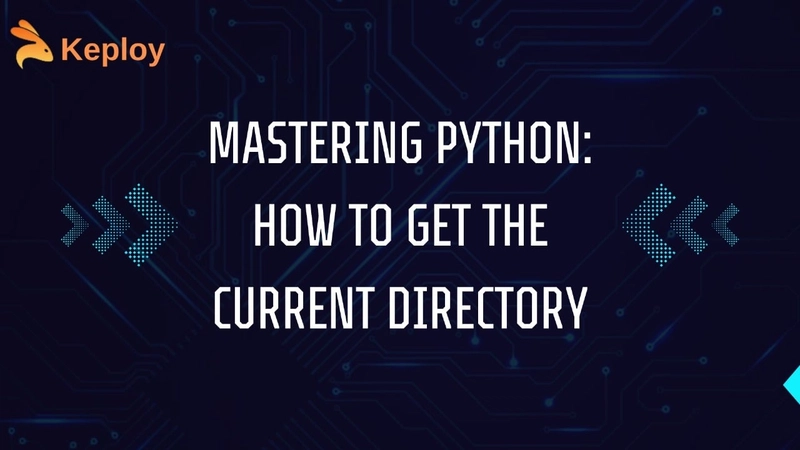
In JavaScript, variables are fundamental building blocks. With the introduction of ES6, developers gained more options - let
and const
- alongside the traditional var
. Understanding the distinctions between these declarations is crucial for writing clean, efficient, and bug-free code.
Regardless of whether you are new to programming or not, knowing how var
, let
, and const
operate can help you avoid a lot of common mistakes and write better JavaScript. This complete guide covers the differences in detail, provides real world examples, best practices, and answers to frequently asked questions. The Evolution of Variable Declarations in JavaScript
The Era of var
Initially, JavaScript offered only the var
keyword for variable declarations. While functional, var
has characteristics that can lead to unintended behaviors, especially in larger codebases. For instance, its function-scoped nature and support for re-declarations make it less predictable.
Former to ES6, developers tended to rely on patterns and conventions to mimic block scoping or immutability, such as wrapping variables in IIFEs (Immediately Invoked Function Expressions). These types of solutions demonstrated the need for an improvement in variable declaration methods.
The Advent of let
and const
To solve some of the challenges that could be faced with var, ES6 (ECMAScript 2015) added let and const, which provide developers better control of variable scope and mutability. The addition of let and const fundamentally changed the way that JavaScript handles variables, and they are regarded as a best practice.
In-Depth Look at var
Characteristics of var
:
-
Function Scope: Variables declared with
var
are confined to the function in which they're declared, not the block.
javascript function example() { if (true) { var x = 10; } console.log(x); // Outputs: 10 } example();
-
Hoisting:
var
declarations are hoisted to the top of their scope and initialized withundefined
. This can lead to bugs if the developer assumes the variable is available only after the declaration line.
javascript console.log(y); // Outputs: undefined var y = 5;
-
Re-declaration: Variables declared with
var
can be re-declared within the same scope without errors.
javascript var z = 1; var z = 2; console.log(z); // Outputs: 2
Exploring let
Characteristics of let
:
-
Block Scope:
let
confines variables to the block in which they're declared, reducing the risk of accidental overwrites and scope leakage.
javascript function test() { if (true) { let a = 20; console.log(a); // Outputs: 20 } console.log(a); // ReferenceError: a is not defined } test();
-
Temporal Dead Zone (TDZ): Variables declared with
let
are hoisted but not initialized until their definition is evaluated, making it easier to catch bugs at runtime.
javascript console.log(b); // ReferenceError: Cannot access 'b' before initialization let b = 15;
-
No Re-declaration:
let
does not allow re-declaration within the same scope, enforcing better coding practices.
javascript let c = 3; let c = 4; // SyntaxError: Identifier 'c' has already been declared
-
Ideal for Loops:
let
is often preferred in loop constructs because it provides block scope.
javascript for (let i = 0; i < 5; i++) { setTimeout(() => console.log(i), 100); }
Understanding
const
Characteristics of const
:
Block Scope: Similar to
let
,const
is block-scoped.-
Immutability: Variables declared with
const
cannot be reassigned after their initial assignment. This doesn't mean the value itself is immutable—objects and arrays declared withconst
can still be mutated.
javascript const PI = 3.14159; PI = 3.14; // TypeError: Assignment to constant variable.
-
Mutable Contents: The contents of a
const
object can be modified.
javascript const arr = [1, 2, 3]; arr.push(4); // Works fine console.log(arr); // Outputs: [1, 2, 3, 4]
You can even escape and unescape JSON data stored in
const
objects dynamically.Use Cases:
const
is ideal for values that should never change, like configuration settings, API endpoints, or constant references.
5. var
vs let
vs const
: A Comparative Overview
Scope
-
var
is function-scoped.
python jsCopyEditfunction test() { var x = 10; } console.log(x); // Error: x is not defined
-
let
andconst
are block-scoped.
python jsCopyEdit{ let y = 20; const z = 30; } console.log(y); // Error console.log(z); // Error
Hoisting
-
var
is hoisted and initialized asundefined
.
python jsCopyEditconsole.log(a); // undefined var a = 5;
-
let
andconst
are hoisted but not initialized — accessing them before declaration throws an error (Temporal Dead Zone).
python jsCopyEditconsole.log(b); // ReferenceError let b = 10;
Re-declaration
-
var
allows re-declaration.
python jsCopyEditvar a = 1; var a = 2; // No error
-
let
andconst
don’t allow it.
python jsCopyEditlet b = 1; let b = 2; // Error
Re-assignment
-
var
andlet
allow reassignment.
python jsCopyEditvar a = 1; a = 2; // ✅ let b = 3; b = 4; // ✅
-
const
does not.
python jsCopyEditconst c = 5; c = 6; // ❌ TypeError
Immutability
-
const
prevents reassignment, but not object mutation.
python jsCopyEditconst obj = { a: 1 }; obj.a = 2; // ✅ Allowed
Best Practices for Variable Declarations
Default to
const
: Useconst
for variables that shouldn't be reassigned. This makes the intent clear and helps prevent accidental reassignments.Use
let
for Mutability: When a variable's value needs to change, opt forlet
. For example, in afor
loop or when tracking user interactions.Avoid
var
: Given its function-scoped nature and hoisting behavior,var
can lead to unexpected bugs. Modern JavaScript development favorslet
andconst
for their predictable scoping.Use Linters: Tools like ESLint can help enforce the use of
let
andconst
, flagging potential misuse ofvar
and helping enforce consistency. Also, when testing your codebase, make sure you effectively write Java Unit Tests to catch issues early.Refactor Legacy Code: When working with older codebases that use
var
, consider refactoring tolet
andconst
where appropriate for better maintainability.
Common Pitfalls and How to Avoid Them
Accidental Global Variables:
Declaring a variable without var
, let
, or const
automatically makes it global, which can lead to unintended side effects. To verify if your logic is sound, you can learn how to check if a key exists in a JS object without errors.
javascript
function example() {
x = 10; // Implicit global variable
}
example();
console.log(x); // Outputs: 10
Avoidance Tip: Always declare variables using let
, const
, or var
to prevent polluting the global namespace.
Confusion with Hoisting:
Misunderstanding hoisting can lead to bugs, especially when using var
. If you're testing backend logic with var
, see how to master Node.js backend testing with Mocha and Chai.
javascript
console.log(d); // Outputs: undefined
var d = 5;
Avoidance Tip: Familiarize yourself with hoisting behaviors and prefer let
or const
to mitigate related issues.
Temporal Dead Zone (TDZ):
Accessing variables declared with let
or const
before their declaration results in a ReferenceError due to the TDZ.
javascript
console.log(e); // ReferenceError
let e = 10;
Avoidance Tip: Always declare variables at the beginning of their scope to avoid the TDZ.
Real-World Use Cases
When to Use var
Legacy codebases that predate ES6.
When working within older frameworks or libraries that expect it.
When to Use let
Iterating over data in loops. Learn how to generate random numbers in JavaScript for dynamic iterations.
Tracking values that change over time.
Managing mutable state within a function or block.
When to Use const
Defining configuration objects.
Assigning fixed references (like API keys, constants).
Declaring functions and arrow functions.
Conclusion
Understanding the differences between var
, let
, and const
is essential for writing efficient and error-free JavaScript code. While var
served well in the early days, the arrival of let
and const
has improved how developers handle scope and immutability. Use const
by default, let
when necessary, and avoid var
unless there's a specific reason.
By following modern best practices and staying consistent with variable declarations, you'll not only write better code but also ensure it's easier to debug, refactor, and maintain.
Keywords covered naturally: var vs let
, javascript let vs var
, var vs let vs const
, javascript let vs var
.
FAQs
Q1: Can I change the value of a const
variable?
No, once assigned, a const
variable cannot be reassigned. However, if it's an object or array, you can modify its properties or elements.
Q2: Should I always use const
?
Use const
by default and switch to let
only when you know the value needs to change.
Q3: Is var
still used in modern JavaScript?
While var
still works, modern JavaScript practices encourage the use of let
and const
for cleaner, more predictable code.
Q4: What is the Temporal Dead Zone (TDZ)?
The TDZ is the period between entering the scope and the variable's declaration where accessing the variable will result in a ReferenceError.
Q5: Why does var
allow access before declaration?
Because var
is hoisted and initialized to undefined
, you can access it before the actual line of declaration, though it's usually not a good practice.
Q6: Can I use const
with arrow functions?
Yes, in fact, it’s a common pattern to declare arrow functions using const
to avoid accidental reassignment.
javascript
const greet = () => console.log('Hello!');