Master Java Exception Handling: 10 Proven Patterns for Robust Applications
As a best-selling author, I invite you to explore my books on Amazon. Don't forget to follow me on Medium and show your support. Thank you! Your support means the world! Java exception handling is something I've worked with extensively throughout my career as a developer. Creating resilient applications requires thoughtful error management strategies. Let's explore the most effective approaches to handling exceptions in Java applications. Understanding Java Exception Handling Exceptions in Java represent abnormal conditions that disrupt the normal flow of program execution. The Java Exception hierarchy starts with the Throwable class, which branches into Error and Exception. While Errors typically represent unrecoverable conditions, Exceptions are meant to be caught and handled. try { // Code that might throw an exception int result = 10 / 0; } catch (ArithmeticException e) { // Exception handling code System.err.println("Division by zero: " + e.getMessage()); } finally { // Code that always executes System.out.println("This always runs"); } This basic structure forms the foundation for more sophisticated exception handling patterns. Creating Custom Exception Hierarchies One of the most effective patterns I've implemented is designing domain-specific exception hierarchies. This approach makes error classification more semantic and helps organize exceptions in a way that mirrors your business domain. Instead of generic exceptions, I create specific ones that clearly communicate what went wrong: // Base exception for all payment-related issues public class PaymentException extends RuntimeException { private final String transactionId; public PaymentException(String message, String transactionId) { super(message); this.transactionId = transactionId; } public String getTransactionId() { return transactionId; } } // More specific payment exceptions public class InsufficientFundsException extends PaymentException { private final BigDecimal available; private final BigDecimal required; public InsufficientFundsException(String message, String transactionId, BigDecimal available, BigDecimal required) { super(message, transactionId); this.available = available; this.required = required; } // Getters for additional fields } public class PaymentGatewayException extends PaymentException { private final String gatewayErrorCode; public PaymentGatewayException(String message, String transactionId, String gatewayErrorCode) { super(message, transactionId); this.gatewayErrorCode = gatewayErrorCode; } public String getGatewayErrorCode() { return gatewayErrorCode; } } This hierarchy allows for precise error handling based on exception type: try { paymentService.processPayment(payment); } catch (InsufficientFundsException e) { // Handle insufficient funds specifically notifyUserAboutFundsIssue(e.getAvailable(), e.getRequired()); } catch (PaymentGatewayException e) { // Handle gateway errors differently logGatewayError(e.getGatewayErrorCode()); retryOrNotifyAdmin(e); } catch (PaymentException e) { // Generic payment error handling logPaymentError(e.getTransactionId()); } Exception Translation In multi-layered applications, I've found that exception translation significantly improves maintainability. This pattern involves catching low-level exceptions and rethrowing them as higher-level exceptions that make sense in the current context. For example, when accessing a database repository: public Customer findCustomerById(Long id) { try { return customerRepository.findById(id) .orElseThrow(() -> new CustomerNotFoundException("Customer not found: " + id)); } catch (DataAccessException e) { throw new DatabaseException("Database error while finding customer: " + id, e); } catch (Exception e) { throw new ServiceException("Unexpected error finding customer: " + id, e); } } This approach: Preserves the original exception as the cause Provides context-appropriate error messages Abstracts implementation details from higher layers Implementing Selective Retry Patterns For operations that might experience transient failures (like network calls), I implement retry mechanisms with backoff strategies: public T executeWithRetry(Supplier operation, int maxAttempts, long initialDelayMs) { int attempts = 0; long delay = initialDelayMs; while (true) { try { attempts++; return operation.get(); } catch (Exception e) { if (isRetryable(e) && attempts customerService.getCustomer(customerId), 3, // max 3 attempts 1000 // starting with 1 second delay ); This pattern works especially well with functio
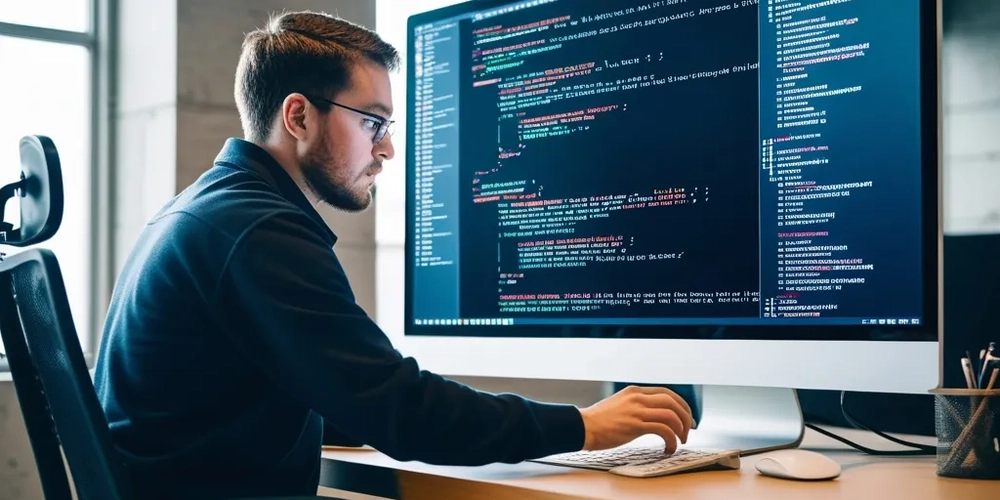
As a best-selling author, I invite you to explore my books on Amazon. Don't forget to follow me on Medium and show your support. Thank you! Your support means the world!
Java exception handling is something I've worked with extensively throughout my career as a developer. Creating resilient applications requires thoughtful error management strategies. Let's explore the most effective approaches to handling exceptions in Java applications.
Understanding Java Exception Handling
Exceptions in Java represent abnormal conditions that disrupt the normal flow of program execution. The Java Exception hierarchy starts with the Throwable class, which branches into Error and Exception. While Errors typically represent unrecoverable conditions, Exceptions are meant to be caught and handled.
try {
// Code that might throw an exception
int result = 10 / 0;
} catch (ArithmeticException e) {
// Exception handling code
System.err.println("Division by zero: " + e.getMessage());
} finally {
// Code that always executes
System.out.println("This always runs");
}
This basic structure forms the foundation for more sophisticated exception handling patterns.
Creating Custom Exception Hierarchies
One of the most effective patterns I've implemented is designing domain-specific exception hierarchies. This approach makes error classification more semantic and helps organize exceptions in a way that mirrors your business domain.
Instead of generic exceptions, I create specific ones that clearly communicate what went wrong:
// Base exception for all payment-related issues
public class PaymentException extends RuntimeException {
private final String transactionId;
public PaymentException(String message, String transactionId) {
super(message);
this.transactionId = transactionId;
}
public String getTransactionId() {
return transactionId;
}
}
// More specific payment exceptions
public class InsufficientFundsException extends PaymentException {
private final BigDecimal available;
private final BigDecimal required;
public InsufficientFundsException(String message, String transactionId,
BigDecimal available, BigDecimal required) {
super(message, transactionId);
this.available = available;
this.required = required;
}
// Getters for additional fields
}
public class PaymentGatewayException extends PaymentException {
private final String gatewayErrorCode;
public PaymentGatewayException(String message, String transactionId, String gatewayErrorCode) {
super(message, transactionId);
this.gatewayErrorCode = gatewayErrorCode;
}
public String getGatewayErrorCode() {
return gatewayErrorCode;
}
}
This hierarchy allows for precise error handling based on exception type:
try {
paymentService.processPayment(payment);
} catch (InsufficientFundsException e) {
// Handle insufficient funds specifically
notifyUserAboutFundsIssue(e.getAvailable(), e.getRequired());
} catch (PaymentGatewayException e) {
// Handle gateway errors differently
logGatewayError(e.getGatewayErrorCode());
retryOrNotifyAdmin(e);
} catch (PaymentException e) {
// Generic payment error handling
logPaymentError(e.getTransactionId());
}
Exception Translation
In multi-layered applications, I've found that exception translation significantly improves maintainability. This pattern involves catching low-level exceptions and rethrowing them as higher-level exceptions that make sense in the current context.
For example, when accessing a database repository:
public Customer findCustomerById(Long id) {
try {
return customerRepository.findById(id)
.orElseThrow(() -> new CustomerNotFoundException("Customer not found: " + id));
} catch (DataAccessException e) {
throw new DatabaseException("Database error while finding customer: " + id, e);
} catch (Exception e) {
throw new ServiceException("Unexpected error finding customer: " + id, e);
}
}
This approach:
- Preserves the original exception as the cause
- Provides context-appropriate error messages
- Abstracts implementation details from higher layers
Implementing Selective Retry Patterns
For operations that might experience transient failures (like network calls), I implement retry mechanisms with backoff strategies:
public <T> T executeWithRetry(Supplier<T> operation, int maxAttempts, long initialDelayMs) {
int attempts = 0;
long delay = initialDelayMs;
while (true) {
try {
attempts++;
return operation.get();
} catch (Exception e) {
if (isRetryable(e) && attempts < maxAttempts) {
try {
Thread.sleep(delay);
// Exponential backoff
delay *= 2;
} catch (InterruptedException ie) {
Thread.currentThread().interrupt();
throw new ServiceException("Retry interrupted", ie);
}
} else {
throw e;
}
}
}
}
private boolean isRetryable(Exception e) {
return e instanceof TimeoutException ||
e instanceof ConnectionException ||
(e instanceof SQLException && isTransientSqlError((SQLException) e));
}
// Example usage
Customer customer = executeWithRetry(
() -> customerService.getCustomer(customerId),
3, // max 3 attempts
1000 // starting with 1 second delay
);
This pattern works especially well with functional interfaces in Java 8+, allowing for clean, reusable retry logic.
Failure Isolation with Bulkhead Patterns
To prevent failures in one component from cascading throughout an application, I employ bulkhead patterns. This approach compartmentalizes operations so that failures remain isolated:
public class DashboardService {
private final CriticalDataService criticalDataService;
private final OptionalDataService optionalDataService;
public DashboardData getDashboardData(String userId) {
DashboardData dashboard = new DashboardData();
// Critical path - failure here should fail the whole operation
dashboard.setCoreData(criticalDataService.getCoreUserData(userId));
// Non-critical paths - we can continue even if these fail
try {
dashboard.setRecommendations(optionalDataService.getRecommendations(userId));
} catch (Exception e) {
log.warn("Failed to load recommendations for user {}: {}", userId, e.getMessage());
dashboard.setRecommendations(Collections.emptyList());
}
try {
dashboard.setNotifications(optionalDataService.getNotifications(userId));
} catch (Exception e) {
log.warn("Failed to load notifications for user {}: {}", userId, e.getMessage());
dashboard.setNotifications(Collections.emptyList());
}
return dashboard;
}
}
For more sophisticated isolation, I sometimes use Circuit Breaker patterns or thread isolation with libraries like Resilience4j or Hystrix.
Structured Logging for Exception Diagnostics
When exceptions occur, capturing the right context is crucial. I implement structured logging to make diagnostic information readily available:
try {
orderService.processOrder(order);
} catch (OrderProcessingException e) {
Map<String, Object> errorContext = new HashMap<>();
errorContext.put("orderId", order.getId());
errorContext.put("customerId", order.getCustomerId());
errorContext.put("orderAmount", order.getTotalAmount());
errorContext.put("paymentMethod", order.getPaymentMethod());
errorContext.put("errorCode", e.getErrorCode());
log.error("Order processing failed: {}", e.getMessage(), errorContext, e);
// Handle the exception appropriately
}
With a structured logging framework like SLF4J with Logback and a JSON encoder, these entries become easily searchable in log aggregation tools.
Try-with-Resources for Resource Management
To ensure resources are properly closed even when exceptions occur, I always use try-with-resources for AutoCloseable resources:
public List<Customer> loadCustomersFromFile(Path path) {
List<Customer> customers = new ArrayList<>();
try (BufferedReader reader = Files.newBufferedReader(path, StandardCharsets.UTF_8)) {
String line;
while ((line = reader.readLine()) != null) {
customers.add(parseCustomer(line));
}
} catch (IOException e) {
throw new DataImportException("Failed to load customers from " + path, e);
}
return customers;
}
This pattern eliminates resource leaks and makes the code more maintainable.
Validating Early to Prevent Exceptions
I find that preventing exceptions is often better than handling them. Using validation early in methods can avoid many common exceptions:
public Order createOrder(OrderRequest request) {
// Validate input early
if (request == null) {
throw new IllegalArgumentException("Order request cannot be null");
}
if (request.getCustomerId() == null) {
throw new ValidationException("Customer ID is required");
}
if (request.getItems() == null || request.getItems().isEmpty()) {
throw new ValidationException("Order must contain at least one item");
}
// Continue with valid data...
}
For more complex validation, I sometimes use specification patterns or validation frameworks like Hibernate Validator.
Exception Handling in Asynchronous Code
With the increasing use of CompletableFuture and reactive patterns, exception handling becomes more complex. I handle exceptions in async code like this:
CompletableFuture<Order> orderFuture = CompletableFuture
.supplyAsync(() -> orderService.fetchOrder(orderId))
.thenApply(order -> enrichOrder(order))
.exceptionally(ex -> {
if (ex instanceof OrderNotFoundException) {
log.warn("Order not found: {}", orderId);
return Order.createEmptyOrder(orderId);
}
log.error("Error processing order {}", orderId, ex);
throw new CompletionException(
new ServiceException("Failed to process order: " + orderId, ex));
});
// For reactive code using Project Reactor
Mono<Order> orderMono = orderService.fetchOrderReactive(orderId)
.flatMap(this::enrichOrderReactive)
.onErrorResume(OrderNotFoundException.class, ex -> {
log.warn("Order not found: {}", orderId);
return Mono.just(Order.createEmptyOrder(orderId));
})
.onErrorMap(ex -> new ServiceException("Failed to process order: " + orderId, ex));
Global Exception Handling
In web applications, I implement global exception handlers to ensure consistent error responses:
@ControllerAdvice
public class GlobalExceptionHandler {
@ExceptionHandler(ResourceNotFoundException.class)
public ResponseEntity<ErrorResponse> handleResourceNotFound(ResourceNotFoundException ex) {
ErrorResponse error = new ErrorResponse("NOT_FOUND", ex.getMessage());
return new ResponseEntity<>(error, HttpStatus.NOT_FOUND);
}
@ExceptionHandler(ValidationException.class)
public ResponseEntity<ErrorResponse> handleValidation(ValidationException ex) {
ErrorResponse error = new ErrorResponse("VALIDATION_FAILED", ex.getMessage());
return new ResponseEntity<>(error, HttpStatus.BAD_REQUEST);
}
@ExceptionHandler(Exception.class)
public ResponseEntity<ErrorResponse> handleGeneric(Exception ex) {
ErrorResponse error = new ErrorResponse("INTERNAL_ERROR", "An unexpected error occurred");
return new ResponseEntity<>(error, HttpStatus.INTERNAL_SERVER_ERROR);
}
}
This ensures that all exceptions are converted to appropriate HTTP responses with consistent formats.
Using Optional to Avoid NullPointerExceptions
I leverage Java's Optional type to handle potential null values gracefully:
public CustomerDto findCustomer(Long id) {
return customerRepository.findById(id)
.map(this::convertToDto)
.orElseThrow(() -> new CustomerNotFoundException("Customer not found: " + id));
}
// Or for when a default is acceptable
public CustomerDto findCustomerOrDefault(Long id) {
return customerRepository.findById(id)
.map(this::convertToDto)
.orElse(CustomerDto.createDefaultCustomer());
}
Testing Exception Handling
I always include tests for exception scenarios to ensure my error handling works as expected:
@Test
void shouldThrowExceptionWhenCustomerNotFound() {
// Given
when(customerRepository.findById(anyLong())).thenReturn(Optional.empty());
// When, Then
CustomerNotFoundException exception = assertThrows(
CustomerNotFoundException.class,
() -> customerService.getCustomerById(1L)
);
assertEquals("Customer not found: 1", exception.getMessage());
}
@Test
void shouldRetryAndEventuallySucceed() {
// Given
when(paymentGateway.processPayment(any()))
.thenThrow(new TimeoutException("Gateway timeout"))
.thenThrow(new ConnectionException("Connection lost"))
.thenReturn(PaymentResult.success("TX123"));
// When
PaymentResult result = paymentService.processPayment(new Payment(100.0, "USD"));
// Then
assertTrue(result.isSuccessful());
verify(paymentGateway, times(3)).processPayment(any());
}
Performance Considerations
Exception handling in Java has performance implications. I follow these principles:
- Don't use exceptions for normal flow control
- Catch exceptions at the right level - not too early, not too late
- Be cautious with exception wrapping that can create deep stack traces
- Use exception pooling for high-frequency exceptions in performance-critical code
// Instead of this:
try {
return map.get(key);
} catch (NullPointerException e) {
return defaultValue;
}
// Do this:
if (map.containsKey(key)) {
return map.get(key);
} else {
return defaultValue;
}
Conclusion
Effective exception handling is crucial for building robust Java applications. By implementing these patterns - custom exception hierarchies, exception translation, retry mechanisms, failure isolation, and structured logging - I've been able to create systems that fail gracefully and provide clear diagnostic information.
The most important lesson I've learned is that exception handling should be designed thoughtfully as part of the overall application architecture, not added as an afterthought. When done right, it makes applications more resilient, maintainable, and user-friendly, even when things go wrong.
101 Books
101 Books is an AI-driven publishing company co-founded by author Aarav Joshi. By leveraging advanced AI technology, we keep our publishing costs incredibly low—some books are priced as low as $4—making quality knowledge accessible to everyone.
Check out our book Golang Clean Code available on Amazon.
Stay tuned for updates and exciting news. When shopping for books, search for Aarav Joshi to find more of our titles. Use the provided link to enjoy special discounts!
Our Creations
Be sure to check out our creations:
Investor Central | Investor Central Spanish | Investor Central German | Smart Living | Epochs & Echoes | Puzzling Mysteries | Hindutva | Elite Dev | JS Schools
We are on Medium
Tech Koala Insights | Epochs & Echoes World | Investor Central Medium | Puzzling Mysteries Medium | Science & Epochs Medium | Modern Hindutva