JavaScript’s New structuredClone() Function – Say Goodbye to Deep Cloning Headaches!
Deep cloning in JavaScript has always been a bit of a struggle. We’ve tried everything from using JSON.parse(JSON.stringify())—which fails with functions and circular references—to relying on libraries like lodash’s cloneDeep(). But now, there’s a native solution: structuredClone(). What is structuredClone()? structuredClone() is a built-in deep-cloning function that accurately copies most types of data. Unlike JSON.stringify(), it handles many data types correctly: Dates, Maps, Sets, and TypedArrays: Clones these without losing their type. Circular References: Easily clones objects that refer to themselves. Browser & Node.js Support: Available in modern browsers and Node.js (v17+). Old vs. New Deep Cloning The Old Way (Using JSON.stringify()) Consider this example: const obj = { date: new Date(), func: () => "Hello" }; const cloned = JSON.parse(JSON.stringify(obj)); console.log(cloned.date instanceof Date); // false — Date becomes a string! console.log(cloned.func); // undefined — Functions are lost! The New Way (Using structuredClone()) Now, see how structuredClone() improves the situation: const obj = { date: new Date(), func: () => "Hello" }; const cloned = structuredClone(obj); console.log(cloned.date instanceof Date); // true — Date is preserved! // Note: Functions are not cloned because they aren't serializable. Important: While structuredClone() correctly handles most data types and circular references, it does not clone functions or prototype chains. Why structuredClone() is a Game-Changer No More Workarounds: Forget about third-party libraries or manual deep cloning methods. Handles Complex Structures: Clones objects with circular references without crashing. Native Performance: Leverages optimized browser internals for better performance. Cloning Circular References Old method using JSON.stringify() would throw an error: const obj = {}; obj.self = obj; JSON.parse(JSON.stringify(obj)); // Throws an error: Circular structure! Using structuredClone(): const obj = {}; obj.self = obj; const cloned = structuredClone(obj); console.log(cloned.self === cloned); // true — Works flawlessly! Final Thoughts If you’ve struggled with deep cloning in JavaScript, it’s time to embrace structuredClone(). This native function makes cloning safer and more reliable—no more battling with JSON quirks or extra libraries! I'll be back with something new soon!ta-ta
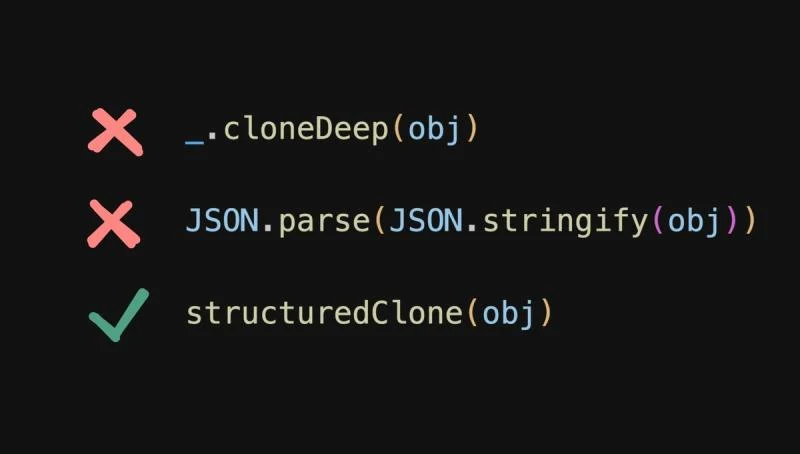
Deep cloning in JavaScript has always been a bit of a struggle. We’ve tried everything from using JSON.parse(JSON.stringify())
—which fails with functions and circular references—to relying on libraries like lodash’s cloneDeep()
. But now, there’s a native solution: structuredClone()
.
What is structuredClone()
?
structuredClone()
is a built-in deep-cloning function that accurately copies most types of data. Unlike JSON.stringify()
, it handles many data types correctly:
- Dates, Maps, Sets, and TypedArrays: Clones these without losing their type.
- Circular References: Easily clones objects that refer to themselves.
- Browser & Node.js Support: Available in modern browsers and Node.js (v17+).
Old vs. New Deep Cloning
The Old Way (Using JSON.stringify()
)
Consider this example:
const obj = {
date: new Date(),
func: () => "Hello"
};
const cloned = JSON.parse(JSON.stringify(obj));
console.log(cloned.date instanceof Date); // false — Date becomes a string!
console.log(cloned.func); // undefined — Functions are lost!
The New Way (Using structuredClone()
)
Now, see how structuredClone()
improves the situation:
const obj = {
date: new Date(),
func: () => "Hello"
};
const cloned = structuredClone(obj);
console.log(cloned.date instanceof Date); // true — Date is preserved!
// Note: Functions are not cloned because they aren't serializable.
Important: While structuredClone()
correctly handles most data types and circular references, it does not clone functions or prototype chains.
Why structuredClone()
is a Game-Changer
- No More Workarounds: Forget about third-party libraries or manual deep cloning methods.
- Handles Complex Structures: Clones objects with circular references without crashing.
- Native Performance: Leverages optimized browser internals for better performance.
Cloning Circular References
Old method using JSON.stringify()
would throw an error:
const obj = {};
obj.self = obj;
JSON.parse(JSON.stringify(obj)); // Throws an error: Circular structure!
Using structuredClone()
:
const obj = {};
obj.self = obj;
const cloned = structuredClone(obj);
console.log(cloned.self === cloned); // true — Works flawlessly!
Final Thoughts
If you’ve struggled with deep cloning in JavaScript, it’s time to embrace structuredClone()
. This native function makes cloning safer and more reliable—no more battling with JSON quirks or extra libraries!
I'll be back with something new soon!ta-ta