Building a React CMS: Fonts, SCSS Resets, and Layout Implementation
In our previous post, we completed the initial setup of our project, including the configuration of all necessary packages, and now, we're diving into the next phase of our application development journey. This entails creating the first route for our login and signup pages and configuring the entire routing process. Additionally, we'll establish some fundamental app configurations such as resetting styles, setting up fonts, and configuring the main layout. Let's outline the plan of action for this crucial stage of our project. Setting Up Fonts in Our React Application Configuring SCSS Reset for Our New React Project Building and Structuring the Main Layout Page in Our CMS Now we have a plan that we need to work on, and it is not small. Let's make a new cup of coffee and back to work. 1. Setting Up Fonts in Our React Application It's one of the preparation steps, that need to be done. I will use the Google Fonts service, we can use CDN links but I like to have those fonts directly in a project that is why I will download a list of fonts. I want to use the neutral font "Roboto", I think this type of font will fantastically represent our app with its functionality. I will download them and take only a few of them: regular, bold, light, and medium types. Btw, if you want to get more about implementing fonts into React projects you can check another tutorial: "How to Add Custom Fonts to Your React App?" Okay, create an assets folder in our src project folder, The assets folder will store styles, fonts, icons, and some images. Inside the assets folder create a new fonts folder and copy into this folder our fonts. Next, create a styles folder inside the assets and index.scss file which will store styles for the whole app. SCSS allows to import of other style files, and it's convenient because you can separate different modules. Also, we need to import our main styles.scss file into the index.js file just to connect styles to the project. Create _fonts.scss file inside the styles folder, here we will import our fonts into the project with the @font-face rule. Into the @font-face rule, we need to add the "font-family" name and src file of our font. @font-face { font-family: 'Roboto-Bold'; src: url('../fonts/Roboto-Bold.ttf'); } @font-face { font-family: 'Roboto-Light'; src: url('../fonts/Roboto-Light.ttf'); } @font-face { font-family: 'Roboto-Medium'; src: url('../fonts/Roboto-Medium.ttf'); } @font-face { font-family: 'Roboto-Regular'; src: url('../fonts/Roboto-Regular.ttf'); } Okay, we added fonts to our app and now they are available for each component we need. Let's move on. 2. Configuring SCSS Reset for Your New React Project Reset styles are a set of CSS (or SCSS in our case) rules designed to remove browser default styling, ensuring a consistent starting point across different browsers. They reset margins, padding, and other properties to a standard baseline, preventing unexpected layout variations. By using reset styles, we can start with a clean slate and build our designs without interference from browser defaults. We will use simple reset styles to reset base rules. For that purpose let's create a new _reset.scss file inside the styles folder and import that file into main styles.scss file. Now we can add reset rules into _reset.scss file: html, body, div, span, applet, object, iframe, h1, h2, h3, h4, h5, h6, p, blockquote, pre, a, abbr, acronym, address, big, cite, code, del, dfn, em, img, ins, kbd, q, s, samp, small, strike, strong, sub, sup, tt, var, b, u, i, center, dl, dt, dd, ol, ul, li, fieldset, form, label, legend, table, caption, tbody, tfoot, thead, tr, th, td, article, aside, canvas, details, embed, figure, figcaption, footer, header, hgroup, menu, nav, output, ruby, section, summary, time, mark, audio, video { margin: 0; padding: 0; border: 0; font-size: 100%; font: inherit; vertical-align: baseline; } article, aside, details, figcaption, figure, footer, header, hgroup, menu, nav, section { display: block; } body { line-height: 1; } ol, ul { list-style: none; } blockquote, q { quotes: none; } blockquote:before, blockquote:after, q:before, q:after { content: ''; content: none; } table { border-collapse: collapse; border-spacing: 0; } Great, finally we can start coding and create our first layout and page in our project. 3. Building and Structuring the Main Layout Page in Our CMS A layout component in React is a reusable component that defines the overall structure and layout of a page or section of the application. It typically contains placeholders or slots where other components can be rendered dynamically. Using a dedicated layout component helps maintain consistency and separation of concerns throughout the application. In other words, we have CMS pages where the header and sidebar will be the same (structure, data, UI) on each page,
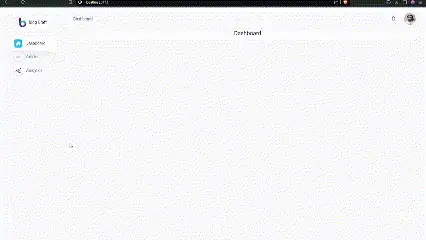
In our previous post, we completed the initial setup of our project, including the configuration of all necessary packages, and now, we're diving into the next phase of our application development journey. This entails creating the first route for our login and signup pages and configuring the entire routing process. Additionally, we'll establish some fundamental app configurations such as resetting styles, setting up fonts, and configuring the main layout. Let's outline the plan of action for this crucial stage of our project.
- Setting Up Fonts in Our React Application
- Configuring SCSS Reset for Our New React Project
- Building and Structuring the Main Layout Page in Our CMS
Now we have a plan that we need to work on, and it is not small. Let's make a new cup of coffee and back to work.
1. Setting Up Fonts in Our React Application
It's one of the preparation steps, that need to be done.
I will use the Google Fonts service, we can use CDN links but I like to have those fonts directly in a project that is why I will download a list of fonts. I want to use the neutral font "Roboto", I think this type of font will fantastically represent our app with its functionality. I will download them and take only a few of them: regular, bold, light, and medium types.
Btw, if you want to get more about implementing fonts into React projects you can check another tutorial: "How to Add Custom Fonts to Your React App?"
Okay, create an assets folder in our src project folder, The assets folder will store styles, fonts, icons, and some images. Inside the assets folder create a new fonts folder and copy into this folder our fonts.
Next, create a styles folder inside the assets and index.scss file which will store styles for the whole app. SCSS allows to import of other style files, and it's convenient because you can separate different modules. Also, we need to import our main styles.scss file into the index.js file just to connect styles to the project.
Create _fonts.scss file inside the styles folder, here we will import our fonts into the project with the @font-face rule. Into the @font-face rule, we need to add the "font-family" name and src file of our font.
@font-face {
font-family: 'Roboto-Bold';
src: url('../fonts/Roboto-Bold.ttf');
}
@font-face {
font-family: 'Roboto-Light';
src: url('../fonts/Roboto-Light.ttf');
}
@font-face {
font-family: 'Roboto-Medium';
src: url('../fonts/Roboto-Medium.ttf');
}
@font-face {
font-family: 'Roboto-Regular';
src: url('../fonts/Roboto-Regular.ttf');
}
Okay, we added fonts to our app and now they are available for each component we need. Let's move on.
2. Configuring SCSS Reset for Your New React Project
Reset styles are a set of CSS (or SCSS in our case) rules designed to remove browser default styling, ensuring a consistent starting point across different browsers. They reset margins, padding, and other properties to a standard baseline, preventing unexpected layout variations. By using reset styles, we can start with a clean slate and build our designs without interference from browser defaults.
We will use simple reset styles to reset base rules. For that purpose let's create a new _reset.scss file inside the styles folder and import that file into main styles.scss file. Now we can add reset rules into _reset.scss file:
html, body, div, span, applet, object, iframe,
h1, h2, h3, h4, h5, h6, p, blockquote, pre,
a, abbr, acronym, address, big, cite, code,
del, dfn, em, img, ins, kbd, q, s, samp,
small, strike, strong, sub, sup, tt, var,
b, u, i, center,
dl, dt, dd, ol, ul, li,
fieldset, form, label, legend,
table, caption, tbody, tfoot, thead, tr, th, td,
article, aside, canvas, details, embed,
figure, figcaption, footer, header, hgroup,
menu, nav, output, ruby, section, summary,
time, mark, audio, video {
margin: 0;
padding: 0;
border: 0;
font-size: 100%;
font: inherit;
vertical-align: baseline;
}
article, aside, details, figcaption, figure,
footer, header, hgroup, menu, nav, section {
display: block;
}
body {
line-height: 1;
}
ol, ul {
list-style: none;
}
blockquote, q {
quotes: none;
}
blockquote:before, blockquote:after,
q:before, q:after {
content: '';
content: none;
}
table {
border-collapse: collapse;
border-spacing: 0;
}
Great, finally we can start coding and create our first layout and page in our project.
3. Building and Structuring the Main Layout Page in Our CMS
A layout component in React is a reusable component that defines the overall structure and layout of a page or section of the application. It typically contains placeholders or slots where other components can be rendered dynamically. Using a dedicated layout component helps maintain consistency and separation of concerns throughout the application. In other words, we have CMS pages where the header and sidebar will be the same (structure, data, UI) on each page, so we can create a simple layout that will always render the same navigation, and update only the main page. The layout will be like a component between rendered routes and the App.jsx main component.
Let's create a layouts folder in the src directory, we will have two layouts "main", and an additional "authentication" layout for our "sign in" and "sign up" pages, because they will not contain a header or sidebar.
Inside the "main" folder create MainLayout.jsx component and MainLayout.styles.scss file for component styles, also let's create "header" and "footer" folders, with the same file structure over here in the "main" folder so that all "Main Layout" components stay in the same place. Great, let's write some code.
Open the App.jsx file and import "Router", "Routes", and "Route" from the "react-router-dom", also we need to import the "MainLayout" component and "Dashboard" (dashboard will be our first route, create "views" folder and "dashboard" folder with typical component structure. For now, the Dashboard will be an empty React component that will show only the "Dashboard" header).
"Router" will be our parent wrapper, then "MainLayout" and then our "Routes" with the first "Dashboard" route. So that router will pass its routes as a child to the "MainLayout" and the layout component will render the child component inside.
import React from 'react';
import { BrowserRouter as Router, Routes, Route } from 'react-router-dom';
import MainLayout from './layouts/main/MainLayout';
import Dashboard from './views/dashboard/Dashboard';
const App = () => {
return (
<Router>
<MainLayout>
<Routes>
<Route path="/" element={<Dashboard />} />
</Routes>
</MainLayout>
</Router>
);
};
export default App;
Now, the "MainLayout" component. The component should use children as props, children will render our routes, so we need to import the header and sidebar, wrap them into some tags, and add some styles to separate pages on three sections: content, header, and sidebar.
import React from 'react';
import './mainLayout.styles.scss';
import Header from './header/header.component';
import Sidebar from './sidebar/sidebar.component';
const MainLayout = ({ children }) => {
return (
<div className='mainLayout'>
<Sidebar />
<main className='mainLayout__container'>
<Header />
<div className='mainLayout__container--content'>
{children}
</div>
</main>
</div>
);
};
export default MainLayout;
After all these manipulations, we simply need to craft "Header" and "Sidebar". Let's start with "Header", which will simply show the route name on the left side and a notification with a profile menu on the right side. So, we need to open the "header.component" file and add some tags and styles.
import React, { useEffect, useState } from "react";
import { useLocation } from "react-router-dom";
import './header.styles.scss';
import NotificationsNoneIcon from '@mui/icons-material/NotificationsNone';
const Header = () => {
const location = useLocation();
const [pageTitle, setPageTitle] = useState("");
const formatPageTitle = (path) => {
switch (path) {
case "/":
return "Dashboard";
default:
path = path.slice(1);
path = path.charAt(0).toUpperCase() + path.slice(1);
return path;
}
};
useEffect(() => {
setPageTitle(formatPageTitle(location.pathname));
}, [location]);
return (
<header className="header">
<div className="header__container">
<div className="header__left">
<div className="header__left--title">
<h1>{pageTitle}</h1>
</div>
</div>
<div className="header__right">
<div className="header__right--notification">
<NotificationsNoneIcon />
</div>
<div className="header__right--profile">
<img
src="https://images.pexels.com/photos/220453/pexels-photo-220453.jpeg?auto=compress&cs=tinysrgb&w=1260&h=750&dpr=1"
alt="profile"
/>
</div>
</div>
</div>
</header>
);
};
export default Header;
Sidebar - will contain a logo and a list of routes with icons. Also, we will use "useLocation" hook to get the path and dynamicaly set the class name to each link. I think this will be enough for now.
import React from "react";
import { NavLink, useLocation } from "react-router-dom";
import './sidebar.styles.scss';
import HomeIcon from '@mui/icons-material/Home';
import ListIcon from '@mui/icons-material/List';
import QueryStatsIcon from '@mui/icons-material/QueryStats';
const Sidebar = () => {
const location = useLocation();
const isActive = (path) => {
return location.pathname === path;
};
return (
<aside className="sidebar">
<div className="sidebar__logo">
<div className="sidebar__logo--icon">
<img src="../../../../logo.png" alt="logo" />
</div>
<h1 className="sidebar__title">Blog Craft</h1>
</div>
<hr className="sidebar__divider"/>
<ul className="sidebar__list">
<NavLink to="/" className={`${isActive('/') ? 'sidebar--item-active' : ''}`}>
<li className="sidebar__list--item">
<div className="sidebar__list--item-icon">
<HomeIcon />
</div>
<div className="sidebar__list--item-text">
Dashboard
</div>
</li>
</NavLink>
</ul>
</aside>
);
};
export default Sidebar;
That's it, we have a fully workable layout with the header, and the sidebar.
In this article, we've covered key aspects of building a React CMS, focusing on fonts, SCSS resets, and layout implementation. We began by setting up custom fonts, ensuring our application has a consistent and professional typography. Next, we implemented SCSS reset styles to create a clean slate for our designs across different browsers. Finally, we built a reusable layout component, complete with a header and sidebar, which will serve as the foundation for our CMS pages.
By following these steps, we've established a solid structure for our React CMS, setting the stage for further development. With our fonts, styles, and layout in place, we're now ready to move forward with creating specific features and functionalities for our content management system.
If you need a source code for this tutorial you can get it here.
Found this post useful? ☕ A coffee-sized contribution goes a long way in keeping me inspired! Thank you)
Next step: "Structuring the Server: Node.js, MongoDB, and User Models for Our CMS"