How to Document Constants in Python Effectively?
In Python programming, it's common to define constants that are used throughout your code. These constants, stored in a file like constants.py, can help keep your code organized and make it easier to update settings or values in the future. However, when documenting functions that use these constants, developers often face the dilemma of whether to refer to the constants directly in the documentation or use their values instead. Why Use Constants in Python? Constants like PATH_DATASETS = "data/datasets/" and WIDTH = 416 serve as fixed values that can be reused across multiple functions in your program. This practice enhances code readability, maintainability, and minimizes the risk of introducing errors when changes are needed. The Problem with Documentation When you define a function that uses a constant as a default parameter, such as def main_train(..., width: int = constants.WIDTH, ...), the documentation generated by tools like Sphinx will refer to constants.WIDTH in the function signature. Hovering over the function in your IDE, such as VSCode, may show constants.WIDTH instead of its actual default value (in this case, 416). This could lead to confusion for someone reading the documentation, as they may not immediately recognize the value of constants.WIDTH. Therefore, it raises a significant question in the development process: Should you replace references to constants with their actual values in comments and documentation, or should you keep the references to the constants for maintainability? Best Practices for Documentation When preparing your documentation, consider the following approaches: Keep constants in the documentation Keeping the reference to constants.WIDTH in your documentation can be beneficial since it automatically updates when you change the constant value. This way, you're reducing the chance of having outdated documentation that doesn't reflect your code's logic. If someone wants to know what WIDTH represents, they can check its declaration in constants.py. Use Sphinx docstring convention Instead of saying, "The default is constants.WIDTH", clarify by stating: "The default width is defined in constants.py. Check this file for the actual value." This way, users are directed where to find the most updated information. Example of Python Code Here’s an example to illustrate this approach: # constants.py PATH_DATASETS = "data/datasets/" WIDTH = 416 # main.py from constants import WIDTH from pathlib import PurePath from typing import List def main_train(path_home: PurePath, path_dataset: PurePath, template: List[PurePath], width: int = WIDTH): """ Args: path_home (PurePath): The home path. path_dataset (PurePath): The dataset path. template (List[PurePath]): A list of template paths. width (int): Width of the network for processing images. Must be a multiple of 32. The default width is defined in `constants.py`. Check this file for the actual value. """ # Function implementation here Balancing Readability and Maintainability For projects where constants are heavily referenced, it's vital to strike a balance between documentation readability and maintainability. While using values may clarify the documentation, it can lead to more work in the long run if you have to update your documentation every time a constant changes. In general, it's advisable to keep constant references in your docstrings. Moreover, you can create a single source of truth by documenting their intended use and significance in the constants file itself. This could involve adding comments next to each constant explaining its purpose, thus aiding users in understanding the value behind the constant more fully. Frequently Asked Questions (FAQ) Q: Why is it important to use constants in Python? A: Using constants improves code maintainability and readability, reducing hardcoding and potential errors when changes are needed. Q: How can I improve function documentation in Sphinx? A: Focus on clarity by referencing constants and providing context within the documentation, guiding users to understand the logic without hardcoding values. Q: Should I avoid using default parameter values for constants? A: Not necessarily. Using constants as default values can be a good practice, as long as the documentation clarifies where they can be found and their significance. Keeping your documentation clear and your constants well-organized enhances code comprehension, making it easier for others (and yourself) to work with your code in the future!
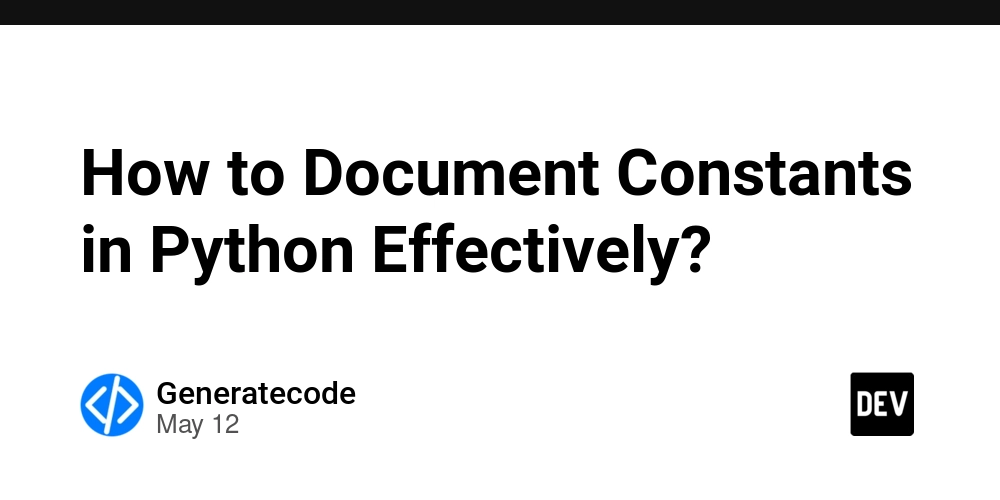
In Python programming, it's common to define constants that are used throughout your code. These constants, stored in a file like constants.py
, can help keep your code organized and make it easier to update settings or values in the future. However, when documenting functions that use these constants, developers often face the dilemma of whether to refer to the constants directly in the documentation or use their values instead.
Why Use Constants in Python?
Constants like PATH_DATASETS = "data/datasets/"
and WIDTH = 416
serve as fixed values that can be reused across multiple functions in your program. This practice enhances code readability, maintainability, and minimizes the risk of introducing errors when changes are needed.
The Problem with Documentation
When you define a function that uses a constant as a default parameter, such as def main_train(..., width: int = constants.WIDTH, ...)
, the documentation generated by tools like Sphinx will refer to constants.WIDTH
in the function signature. Hovering over the function in your IDE, such as VSCode, may show constants.WIDTH
instead of its actual default value (in this case, 416).
This could lead to confusion for someone reading the documentation, as they may not immediately recognize the value of constants.WIDTH
. Therefore, it raises a significant question in the development process: Should you replace references to constants with their actual values in comments and documentation, or should you keep the references to the constants for maintainability?
Best Practices for Documentation
When preparing your documentation, consider the following approaches:
-
Keep constants in the documentation
Keeping the reference toconstants.WIDTH
in your documentation can be beneficial since it automatically updates when you change the constant value. This way, you're reducing the chance of having outdated documentation that doesn't reflect your code's logic. If someone wants to know whatWIDTH
represents, they can check its declaration inconstants.py
. -
Use Sphinx docstring convention
Instead of saying, "The default isconstants.WIDTH
", clarify by stating: "The default width is defined inconstants.py
. Check this file for the actual value." This way, users are directed where to find the most updated information.
Example of Python Code
Here’s an example to illustrate this approach:
# constants.py
PATH_DATASETS = "data/datasets/"
WIDTH = 416
# main.py
from constants import WIDTH
from pathlib import PurePath
from typing import List
def main_train(path_home: PurePath, path_dataset: PurePath, template: List[PurePath], width: int = WIDTH):
"""
Args:
path_home (PurePath): The home path.
path_dataset (PurePath): The dataset path.
template (List[PurePath]): A list of template paths.
width (int): Width of the network for processing images. Must be a multiple of 32. The default width is defined in `constants.py`. Check this file for the actual value.
"""
# Function implementation here
Balancing Readability and Maintainability
For projects where constants are heavily referenced, it's vital to strike a balance between documentation readability and maintainability. While using values may clarify the documentation, it can lead to more work in the long run if you have to update your documentation every time a constant changes.
In general, it's advisable to keep constant references in your docstrings. Moreover, you can create a single source of truth by documenting their intended use and significance in the constants file itself. This could involve adding comments next to each constant explaining its purpose, thus aiding users in understanding the value behind the constant more fully.
Frequently Asked Questions (FAQ)
Q: Why is it important to use constants in Python?
A: Using constants improves code maintainability and readability, reducing hardcoding and potential errors when changes are needed.
Q: How can I improve function documentation in Sphinx?
A: Focus on clarity by referencing constants and providing context within the documentation, guiding users to understand the logic without hardcoding values.
Q: Should I avoid using default parameter values for constants?
A: Not necessarily. Using constants as default values can be a good practice, as long as the documentation clarifies where they can be found and their significance.
Keeping your documentation clear and your constants well-organized enhances code comprehension, making it easier for others (and yourself) to work with your code in the future!