How to Fix 'Undefined Mixin' Error in Dart Stylesheets
When working with Dart and stylesheets, particularly those using SCSS/Sass, you might encounter a common issue: the 'Undefined mixin' error. This problem often arises when importing mixins and functions from Bootstrap or any other library. In this article, we'll explore why this error happens and provide a comprehensive solution to fix it. Understanding the Undefined Mixin Error The 'Undefined mixin' error in SCSS usually indicates that the compiler cannot find a specific mixin or function that you are trying to use in your stylesheet. In your case, you're trying to utilize a media-breakpoint-down(md) mixin that is part of Bootstrap's SCSS library. The correct order of imports is crucial since Sass relies on the definitions being available in the scope when they're used. Why the Error Occurs Importing Stylesheets Your first stylesheet imports several Bootstrap SCSS components: @import 'node_modules/bootstrap/scss/functions'; @import 'node_modules/bootstrap/scss/variables'; @import 'node_modules/bootstrap/scss/mixins'; By importing the functions, variables, and mixins in that precise order, you ensure that each component is loaded correctly before it's used. However, when you try to include this stylesheet into a second stylesheet, the compiler might not recognize the mixins without the correct path or import directives. Differences in Stylesheet Importing When you import stylesheet1 into stylesheet2 using the following code: @import './stylesheet1'; It’s important to note that SCSS does not share the imported mixins globally from one file to another unless explicitly stated. Thus, you need to ensure that the context of the imports is maintained. Step-by-Step Solution To resolve this issue, follow these steps: Step 1: Use @use Instead of @import Instead of using @import, which has been deprecated in favor of @use, change your import to: In stylesheet1.scss, keep your imports: @use 'node_modules/bootstrap/scss/functions'; @use 'node_modules/bootstrap/scss/variables'; @use 'node_modules/bootstrap/scss/mixins'; Then in stylesheet2.scss, import it like so: @use './stylesheet1'; Step 2: Access Mixin Correctly Once you've switched to @use, you'll need to use the mixins with the namespace. For instance, if you need to use the media-breakpoint-down mixin, you should do it as follows: @include stylesheet1.mixin.media-breakpoint-down(md) { width: 100%; margin: 0 10px; } Step 3: Verify Your File Structure Ensure that your file structure correctly matches your import paths. This should help maintain the context of your imports. Here is an example file structure: project/ │ ├── stylesheets/ │ ├── stylesheet1.scss │ └── stylesheet2.scss │ └── node_modules/ └── bootstrap/ By keeping your files organized and following this import methodology, you should avoid the 'Undefined mixin' error. Frequently Asked Questions What is the difference between @import and @use in SCSS? @import is the older way of including stylesheets, while @use is the new approach that encapsulates styles and prevents namespace conflicts. How can I debug SCSS errors? You can enable verbose error reporting by running the Sass compiler with a flag like --verbose to get more detailed error messages which can assist in debugging issues. Can I use mixins without importing them again in another stylesheet? No, you would need to either import the necessary files again or use the @use command, which allows for better encapsulation and avoids conflicts. By applying these suggestions, you should successfully eliminate the 'Undefined mixin' error from your Dart stylesheets, leading to a smoother development experience.
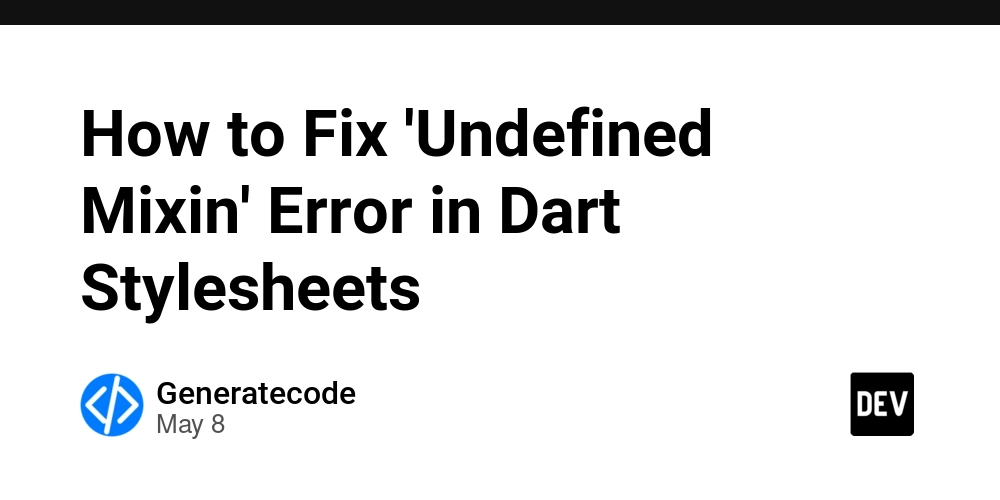
When working with Dart and stylesheets, particularly those using SCSS/Sass, you might encounter a common issue: the 'Undefined mixin' error. This problem often arises when importing mixins and functions from Bootstrap or any other library. In this article, we'll explore why this error happens and provide a comprehensive solution to fix it.
Understanding the Undefined Mixin Error
The 'Undefined mixin' error in SCSS usually indicates that the compiler cannot find a specific mixin or function that you are trying to use in your stylesheet. In your case, you're trying to utilize a media-breakpoint-down(md)
mixin that is part of Bootstrap's SCSS library. The correct order of imports is crucial since Sass relies on the definitions being available in the scope when they're used.
Why the Error Occurs
Importing Stylesheets
Your first stylesheet imports several Bootstrap SCSS components:
@import 'node_modules/bootstrap/scss/functions';
@import 'node_modules/bootstrap/scss/variables';
@import 'node_modules/bootstrap/scss/mixins';
By importing the functions, variables, and mixins in that precise order, you ensure that each component is loaded correctly before it's used. However, when you try to include this stylesheet into a second stylesheet, the compiler might not recognize the mixins without the correct path or import directives.
Differences in Stylesheet Importing
When you import stylesheet1
into stylesheet2
using the following code:
@import './stylesheet1';
It’s important to note that SCSS does not share the imported mixins globally from one file to another unless explicitly stated. Thus, you need to ensure that the context of the imports is maintained.
Step-by-Step Solution
To resolve this issue, follow these steps:
Step 1: Use @use
Instead of @import
Instead of using @import
, which has been deprecated in favor of @use
, change your import to:
In stylesheet1.scss
, keep your imports:
@use 'node_modules/bootstrap/scss/functions';
@use 'node_modules/bootstrap/scss/variables';
@use 'node_modules/bootstrap/scss/mixins';
Then in stylesheet2.scss
, import it like so:
@use './stylesheet1';
Step 2: Access Mixin Correctly
Once you've switched to @use
, you'll need to use the mixins with the namespace. For instance, if you need to use the media-breakpoint-down
mixin, you should do it as follows:
@include stylesheet1.mixin.media-breakpoint-down(md) {
width: 100%;
margin: 0 10px;
}
Step 3: Verify Your File Structure
Ensure that your file structure correctly matches your import paths. This should help maintain the context of your imports. Here is an example file structure:
project/
│
├── stylesheets/
│ ├── stylesheet1.scss
│ └── stylesheet2.scss
│
└── node_modules/
└── bootstrap/
By keeping your files organized and following this import methodology, you should avoid the 'Undefined mixin' error.
Frequently Asked Questions
What is the difference between @import
and @use
in SCSS?
@import
is the older way of including stylesheets, while @use
is the new approach that encapsulates styles and prevents namespace conflicts.
How can I debug SCSS errors?
You can enable verbose error reporting by running the Sass compiler with a flag like --verbose
to get more detailed error messages which can assist in debugging issues.
Can I use mixins without importing them again in another stylesheet?
No, you would need to either import the necessary files again or use the @use
command, which allows for better encapsulation and avoids conflicts.
By applying these suggestions, you should successfully eliminate the 'Undefined mixin' error from your Dart stylesheets, leading to a smoother development experience.