How to make Tool Tips on JavaFx?
Tooltipsare common components in web developments, in this case, I will show you how you could create tool tips in JavaFx using FxPopup, JavaFxadds tool tips to its collection of components, however, these have the drawback that they are not compatible with all types of components io.github.hugoquinn2 fxpopup 1.2.0 As a great advantage, all CSS styles are applied to the main scene, so following the following XML structure, you can apply your own styles. ToolTip (.tool-tip) ├── Label (.tool-tip-label) Social media buttons // Create Social media buttons Button youtube = new Button(); Button gitHub = new Button(); Button twitter = new Button(); Button whatsapp = new Button(); // Apply styles and icons youtube.getStyleClass().add("youtube-button"); gitHub.getStyleClass().add("github-button"); twitter.getStyleClass().add("twitter-button"); whatsapp.getStyleClass().add("whatsapp-button"); youtube.setGraphic(new FontIcon("anto-youtube")); gitHub.setGraphic(new FontIcon("anto-github")); twitter.setGraphic(new FontIcon("anto-twitter")); whatsapp.setGraphic(new FontIcon("anto-whats-app")); // Create tool tips for buttons ToolTip toolTipYoutube = new ToolTip("Youtube", youtube, HPos.LEFT); ToolTip toolTipGitHub = new ToolTip("GitHub", gitHub, VPos.TOP); ToolTip toolTipTwitter = new ToolTip("Twitter", twitter, VPos.BOTTOM); ToolTip toolTipWhatsapp = new ToolTip("Whatsapp", whatsapp, HPos.RIGHT); User Card // Create User Card ImageView userProfileImage = new ImageView("https://th.bing.com/th/id/OIP.gxCrcJ9wcnuS-hppF4l8ggHaHa?rs=1&pid=ImgDetMain"); // Cut user Image to a circle userProfileImage.setFitWidth(100); userProfileImage.setPreserveRatio(true); double w = userProfileImage.getFitWidth(); double h = userProfileImage.getFitHeight(); double size = Math.min(w, h); double x = (w - size) / 2; double y = (h - size) / 2; double radius = w / 2; userProfileImage.setViewport(new Rectangle2D(x, y, size, size)); userProfileImage.setClip(new Circle(radius, radius, radius)); // Make custom Tool Tip ToolTip toolTip = new ToolTip("Sophia Martinez", userProfileImage, HPos.RIGHT, 15); Label description = new Label("Human Resources Manager, People & Culture Department"); toolTip.getChildren().addAll(description); // Apply styles toolTip.setMaxWidth(180); toolTip.setMinWidth(180); toolTip.setAlignment(Pos.CENTER_LEFT); description.setWrapText(true); toolTip.getText().setStyle("-fx-font-weight: bold;");
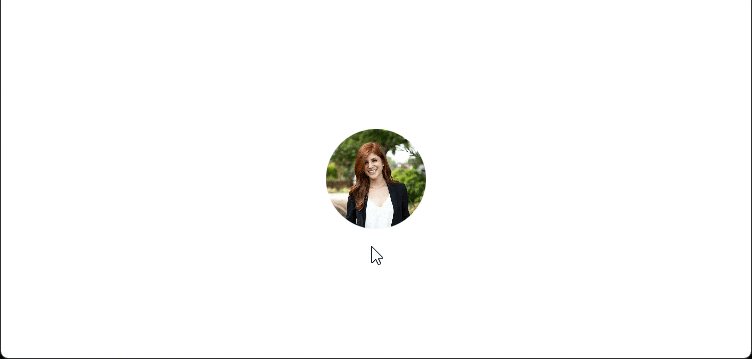
Tooltips
are common components in web developments, in this case, I will show you how you could create tool tips in JavaFx
using FxPopup
, JavaFx
adds tool tips to its collection of components, however, these have the drawback that they are not compatible with all types of components
io.github.hugoquinn2
fxpopup
1.2.0
As a great advantage, all CSS styles are applied to the main scene, so following the following XML structure, you can apply your own styles.
ToolTip (.tool-tip)
├── Label (.tool-tip-label)
Social media buttons
// Create Social media buttons
Button youtube = new Button();
Button gitHub = new Button();
Button twitter = new Button();
Button whatsapp = new Button();
// Apply styles and icons
youtube.getStyleClass().add("youtube-button");
gitHub.getStyleClass().add("github-button");
twitter.getStyleClass().add("twitter-button");
whatsapp.getStyleClass().add("whatsapp-button");
youtube.setGraphic(new FontIcon("anto-youtube"));
gitHub.setGraphic(new FontIcon("anto-github"));
twitter.setGraphic(new FontIcon("anto-twitter"));
whatsapp.setGraphic(new FontIcon("anto-whats-app"));
// Create tool tips for buttons
ToolTip toolTipYoutube = new ToolTip("Youtube", youtube, HPos.LEFT);
ToolTip toolTipGitHub = new ToolTip("GitHub", gitHub, VPos.TOP);
ToolTip toolTipTwitter = new ToolTip("Twitter", twitter, VPos.BOTTOM);
ToolTip toolTipWhatsapp = new ToolTip("Whatsapp", whatsapp, HPos.RIGHT);
User Card
// Create User Card
ImageView userProfileImage = new ImageView("https://th.bing.com/th/id/OIP.gxCrcJ9wcnuS-hppF4l8ggHaHa?rs=1&pid=ImgDetMain");
// Cut user Image to a circle
userProfileImage.setFitWidth(100);
userProfileImage.setPreserveRatio(true);
double w = userProfileImage.getFitWidth();
double h = userProfileImage.getFitHeight();
double size = Math.min(w, h);
double x = (w - size) / 2;
double y = (h - size) / 2;
double radius = w / 2;
userProfileImage.setViewport(new Rectangle2D(x, y, size, size));
userProfileImage.setClip(new Circle(radius, radius, radius));
// Make custom Tool Tip
ToolTip toolTip = new ToolTip("Sophia Martinez", userProfileImage, HPos.RIGHT, 15);
Label description = new Label("Human Resources Manager, People & Culture Department");
toolTip.getChildren().addAll(description);
// Apply styles
toolTip.setMaxWidth(180);
toolTip.setMinWidth(180);
toolTip.setAlignment(Pos.CENTER_LEFT);
description.setWrapText(true);
toolTip.getText().setStyle("-fx-font-weight: bold;");