Weekly Challenge: Sub circular
Weekly Challenge 316 Each week Mohammad S. Anwar sends out The Weekly Challenge, a chance for all of us to come up with solutions to two weekly tasks. My solutions are written in Python first, and then converted to Perl. It's a great way for us all to practice some coding. Challenge, My solutions Task 1: Circular Task You are given a list of words. Write a script to find out whether the last character of each word is the first character of the following word. My solution Both of this week's challenges are relatively straight forward, so don't require much explanation. For this task, I have an iterator called idx that starts at 1 (the second word) to one less than the number of words (the last word). For each value, I check if the first letter of this word is the same as the last letter of the previous word. If it isn't, I return False. If the loop is exhausted, I return True. There is no need to check if the last letter of the last word is the same as the first letter of the first word. def circular(words: list) -> bool: for idx in range(1, len(words)): if words[idx][0] != words[idx-1][-1]: return False return True The Perl solution also follows this logic, although is longer to get the first/last character of each word. sub main (@words) { foreach my $idx (1 .. $#words) { if (substr($words[$idx], 0, 1) ne substr($words[$idx-1], -1, 1)) { say 'false'; return; } } say 'true'; } Examples $ ./ch-1.py perl loves scala True $ ./ch-1.py love the programming False $ ./ch-1.py java awk kotlin node.js True Task 2: Subsequence Task You are given two string. Write a script to find out if one string is a subsequence of another. A subsequence of a string is a new string that is formed from the original string by deleting some (can be none) of the characters without disturbing the relative positions of the remaining characters. My solution Some times is just easier to whip up a quick regular expression to solve a challenge and this is one of those tasks. For this task, I escape all the characters in the first string. In Python this is done with map(re.escape, (l for l in str1)), while Perl uses map { quotemeta } split //, $str1. I then join these with the regular expression .*, which means zero or more of any character. I then use re.search to see if the first string is a subsequence of the second one. def subsequence(str1: str, str2: str) -> bool: r = '.*'.join(map(re.escape, (l for l in str1))) return bool(re.search(r, str2)) Examples $ ./ch-2.py uvw bcudvew True $ ./ch-2.py aec abcde False $ ./ch-2.py sip javascript True
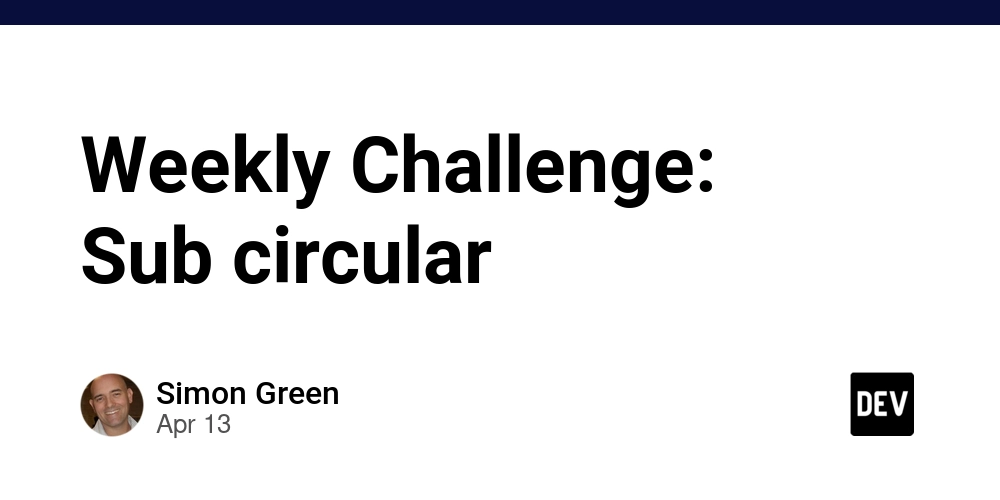
Weekly Challenge 316
Each week Mohammad S. Anwar sends out The Weekly Challenge, a chance for all of us to come up with solutions to two weekly tasks. My solutions are written in Python first, and then converted to Perl. It's a great way for us all to practice some coding.
Task 1: Circular
Task
You are given a list of words.
Write a script to find out whether the last character of each word is the first character of the following word.
My solution
Both of this week's challenges are relatively straight forward, so don't require much explanation. For this task, I have an iterator called idx
that starts at 1 (the second word) to one less than the number of words (the last word).
For each value, I check if the first letter of this word is the same as the last letter of the previous word. If it isn't, I return False
. If the loop is exhausted, I return True
.
There is no need to check if the last letter of the last word is the same as the first letter of the first word.
def circular(words: list) -> bool:
for idx in range(1, len(words)):
if words[idx][0] != words[idx-1][-1]:
return False
return True
The Perl solution also follows this logic, although is longer to get the first/last character of each word.
sub main (@words) {
foreach my $idx (1 .. $#words) {
if (substr($words[$idx], 0, 1) ne substr($words[$idx-1], -1, 1)) {
say 'false';
return;
}
}
say 'true';
}
Examples
$ ./ch-1.py perl loves scala
True
$ ./ch-1.py love the programming
False
$ ./ch-1.py java awk kotlin node.js
True
Task 2: Subsequence
Task
You are given two string. Write a script to find out if one string is a subsequence of another.
A subsequence of a string is a new string that is formed from the original string by deleting some (can be none) of the characters without disturbing the relative positions of the remaining characters.
My solution
Some times is just easier to whip up a quick regular expression to solve a challenge and this is one of those tasks. For this task, I escape all the characters in the first string. In Python this is done with map(re.escape, (l for l in str1))
, while Perl uses map { quotemeta } split //, $str1
.
I then join these with the regular expression .*
, which means zero or more of any character. I then use re.search
to see if the first string is a subsequence of the second one.
def subsequence(str1: str, str2: str) -> bool:
r = '.*'.join(map(re.escape, (l for l in str1)))
return bool(re.search(r, str2))
Examples
$ ./ch-2.py uvw bcudvew
True
$ ./ch-2.py aec abcde
False
$ ./ch-2.py sip javascript
True