Clean, Fast, and Efficient: 4 PHP Tips to Supercharge Your Code
Hey, PHP folks! Want cleaner, faster code without breaking a sweat? Here are four practical tips to sharpen your skills. I’ll keep it short but juicy, with examples and a peek at why they work—spoiler: some are turbo-charged by C optimizations. Let’s roll! Tip 1: Use empty() for Smarter Variable Checks The empty() function is your go-to for checking if a variable exists and has a meaningful value (not '', 0, null). It’s not just handy—it’s a language construct baked into PHP’s core, optimized in C for speed. Before: if (isset($var) && $var !== '' && $var !== 0) { echo "It’s got something!"; } After: if (!empty($var)) { echo "It’s got something!"; } Why it’s better: empty() combines multiple checks into one sleek call. Since it’s written in C, it’s faster than stacking isset() and comparisons. Plus, it’s easier to read—less clutter, more clarity. Tip 2: Lean on Built-in Functions PHP’s built-in functions, like str_replace() or array_sum(), aren’t just convenient—they’re coded in C, making them lightning-fast compared to DIY solutions. Before: $str = "Hello, world!"; $search = "world"; $replace = "PHP"; $result = ""; for ($i = 0; $i $num * $num, $numbers); Why it’s better: array_map() is slicker and faster, thanks to PHP’s array-handling optimizations. It cuts out loop overhead and keeps your code concise—perfect for big datasets or just showing off your modern PHP chops. Tip 4: Cache Smarter with Static Variables Got a function doing heavy lifting, like a database call? Use static variables to cache results inside the function. It’s a simple trick that leverages PHP’s memory management to skip redundant work. Before: function getUserData($id) { // Imagine a slow DB query here return ['id' => $id, 'name' => 'User ' . $id]; } $user1 = getUserData(1); $user2 = getUserData(1); // Repeats the work After: function getUserData($id) { static $cache = []; if (!isset($cache[$id])) { $cache[$id] = ['id' => $id, 'name' => 'User ' . $id]; } return $cache[$id]; } $user1 = getUserData(1); $user2 = getUserData(1); // Pulls from cache Why it’s better: Static vars stick around between calls, storing results in memory. This avoids re-running expensive operations, making your app snappier—especially in loops or high-traffic scenarios. Wrap-Up There you go—four PHP tips to make your code faster, cleaner, and smarter: empty() for checks, built-ins for speed, array functions for style, and static caching for efficiency. Many of these tap into C-level optimizations baked into PHP, so you’re getting performance boosts for free. Try them out, tweak them, and let me know your own tricks in the comments. Happy coding!
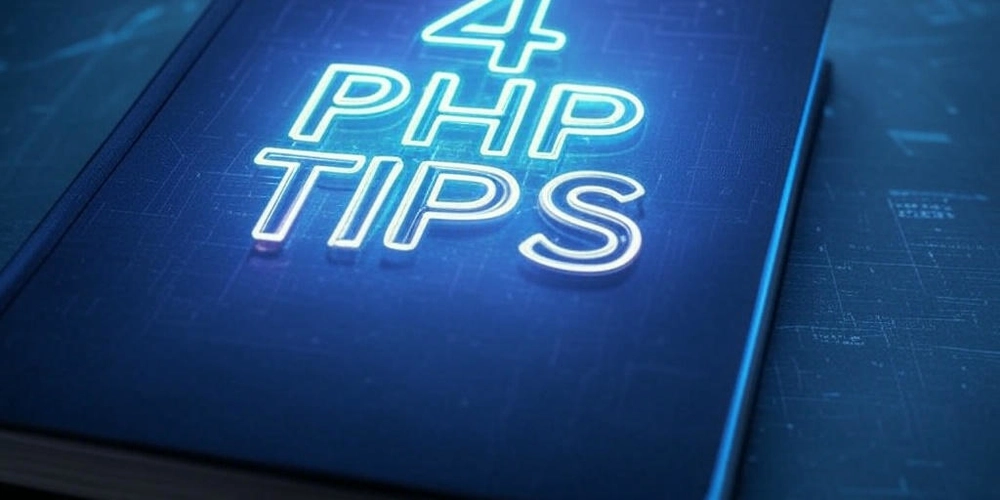
Hey, PHP folks! Want cleaner, faster code without breaking a sweat? Here are four practical tips to sharpen your skills. I’ll keep it short but juicy, with examples and a peek at why they work—spoiler: some are turbo-charged by C optimizations. Let’s roll!
Tip 1: Use empty()
for Smarter Variable Checks
The empty()
function is your go-to for checking if a variable exists and has a meaningful value (not ''
, 0
, null
). It’s not just handy—it’s a language construct baked into PHP’s core, optimized in C for speed.
Before:
if (isset($var) && $var !== '' && $var !== 0) {
echo "It’s got something!";
}
After:
if (!empty($var)) {
echo "It’s got something!";
}
Why it’s better:
empty()
combines multiple checks into one sleek call. Since it’s written in C, it’s faster than stacking isset()
and comparisons. Plus, it’s easier to read—less clutter, more clarity.
Tip 2: Lean on Built-in Functions
PHP’s built-in functions, like str_replace()
or array_sum()
, aren’t just convenient—they’re coded in C, making them lightning-fast compared to DIY solutions.
Before:
$str = "Hello, world!";
$search = "world";
$replace = "PHP";
$result = "";
for ($i = 0; $i < strlen($str); $i++) {
if (substr($str, $i, strlen($search)) == $search) {
$result .= $replace;
$i += strlen($search) - 1;
} else {
$result .= $str[$i];
}
}
After:
$str = "Hello, world!";
$result = str_replace("world", "PHP", $str);
Why it’s better:
Built-ins like str_replace()
are optimized at the C level, so they outpace manual loops by a mile. They’re also battle-tested, reducing bugs and boosting readability. Why reinvent the wheel when PHP’s got a turbo version?
Tip 3: Swap Loops for Array Functions
Need to process arrays? Skip the foreach
grind and use array_map()
or array_filter()
. These functions are designed for arrays and come with internal optimizations that traditional loops can’t match.
Before:
$numbers = [1, 2, 3, 4];
$squares = [];
foreach ($numbers as $num) {
$squares[] = $num * $num;
}
After:
$numbers = [1, 2, 3, 4];
$squares = array_map(fn($num) => $num * $num, $numbers);
Why it’s better:
array_map()
is slicker and faster, thanks to PHP’s array-handling optimizations. It cuts out loop overhead and keeps your code concise—perfect for big datasets or just showing off your modern PHP chops.
Tip 4: Cache Smarter with Static Variables
Got a function doing heavy lifting, like a database call? Use static variables to cache results inside the function. It’s a simple trick that leverages PHP’s memory management to skip redundant work.
Before:
function getUserData($id) {
// Imagine a slow DB query here
return ['id' => $id, 'name' => 'User ' . $id];
}
$user1 = getUserData(1);
$user2 = getUserData(1); // Repeats the work
After:
function getUserData($id) {
static $cache = [];
if (!isset($cache[$id])) {
$cache[$id] = ['id' => $id, 'name' => 'User ' . $id];
}
return $cache[$id];
}
$user1 = getUserData(1);
$user2 = getUserData(1); // Pulls from cache
Why it’s better:
Static vars stick around between calls, storing results in memory. This avoids re-running expensive operations, making your app snappier—especially in loops or high-traffic scenarios.
Wrap-Up
There you go—four PHP tips to make your code faster, cleaner, and smarter: empty()
for checks, built-ins for speed, array functions for style, and static caching for efficiency. Many of these tap into C-level optimizations baked into PHP, so you’re getting performance boosts for free. Try them out, tweak them, and let me know your own tricks in the comments. Happy coding!