How to Handle TypeScript's 'never' Type and Unreachable Code
Introduction In TypeScript, handling unreachable code correctly is crucial for maintaining a robust and error-free application. When you define a function that returns never, it implies that the function will not successfully complete. However, when using such functions, TypeScript may sometimes generate errors, especially when combined with conditional statements. This article addresses how to effectively manage the never type in TypeScript, particularly when dealing with unreachable code. Understanding the Problem The scenario presented involves an if-else construct where the last else condition uses the assert.unreachable function. Since the return type of assert.unreachable is never, you would expect TypeScript to understand that the flow of execution cannot proceed beyond this point. However, TypeScript raises an error: Variable 'result' is used before being assigned. This confusion arises because TypeScript aims for exhaustive checks in your control flow analysis. Hence, let’s first go over the usage of the never type and how these errors can emerge in these scenarios. Example of the Issue Here’s your existing code snippet for context: if (someCondition) { result = $(document.createElement("div")); } else if (anotherCondition) { result = $(document.createElement("div")); } else { assert.unreachable("Unknown label in buildHTML."); } result.attr("data-childNumber", childNumber.toString()); The Problematic Function The unreachable function can be defined as: export function unreachable(message?: string): never { if (message === undefined) message = "Unreachable code reached."; else message = "Unreachable code reached: " + message; raiseTheAlarm(message); } Here, TypeScript raises another error: A function returning 'never' cannot have a reachable end point. This error occurs because TypeScript detects a potential continuation of program logic after calling raiseTheAlarm. Solutions and Best Practices To handle these issues more elegantly, we can consider the following solutions and best practices: 1. Explicitly Using throw or return While it may seem inelegant to use throw null; or add a return statement after raiseTheAlarm, it is a valid way to signal the TypeScript compiler about unreachable code paths. Here's how you can revise your unreachable function: export function unreachable(message?: string): never { if (message === undefined) message = "Unreachable code reached."; else message = "Unreachable code reached: " + message; raiseTheAlarm(message); throw new Error(message); // or return; } By returning or throwing an error, you explicitly indicate that execution should not proceed beyond this point. 2. Using Type Assertions If you're confident of your logic and want to express this type correctness to the TypeScript compiler, you can use type assertions when assigning to result: const result = (function() { if (someCondition) { return $(document.createElement("div")); } else if (anotherCondition) { return $(document.createElement("div")); } else { assert.unreachable("Unknown label in buildHTML."); } })() as JQuery; Here, this constructs a self-executing function that creates result only after your conditional checks are thoroughly evaluated. This ensures result is assigned before any usage. 3. Employing Custom Errors for Clarity You can also define custom error classes to throw when unreachable code is hit. This adds clarity and allows for better error handling: class UnreachableError extends Error { constructor(message: string) { super(message); this.name = 'UnreachableError'; } } export function unreachable(message?: string): never { throw new UnreachableError(message || "Unreachable code reached."); } Using a specialized error class elevates your error-handling strategy considerably. Conclusion In TypeScript, correctly managing the never type is essential for writing robust applications. By understanding how TypeScript evaluates control flow and using throw, return, or type assertions effectively, you can resolve issues surrounding unreachable code without compromising code elegance. Employing custom error classes can improve clarity and handling. With these strategies in hand, you'll be able to harness TypeScript's rigorous type system more effectively. Frequently Asked Questions What is the never type in TypeScript? The never type in TypeScript indicates that a function does not return a value because it either throws an error or goes into an infinite loop. Why does TypeScript raise an error for unreachable code? TypeScript performs flow analysis to ensure all potential paths are covered. If it detects a point in the code that logically cannot be reached, it raises an error for clarity and type safety. Can I ignore TypeScript errors regarding unreachable code? While you technically can suppress TypeScript's error checks, it is not advisable since i
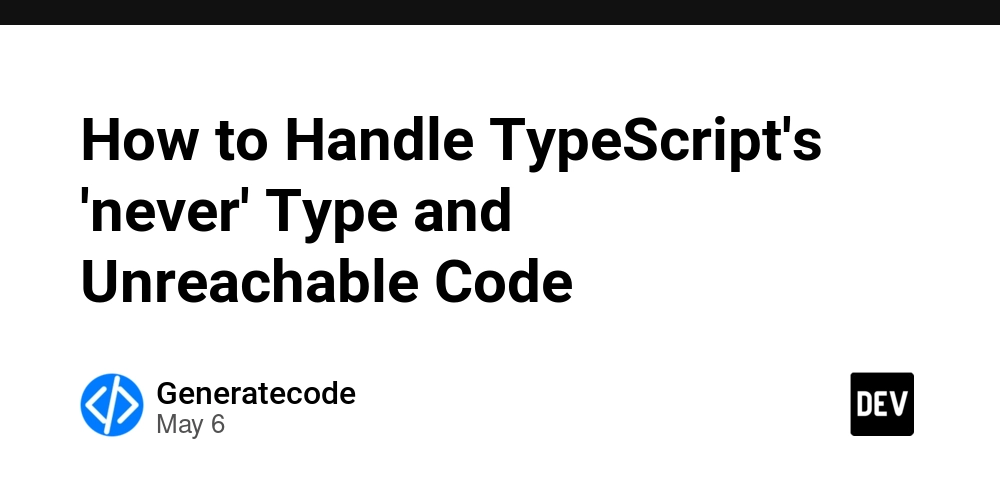
Introduction
In TypeScript, handling unreachable code correctly is crucial for maintaining a robust and error-free application. When you define a function that returns never
, it implies that the function will not successfully complete. However, when using such functions, TypeScript may sometimes generate errors, especially when combined with conditional statements. This article addresses how to effectively manage the never
type in TypeScript, particularly when dealing with unreachable code.
Understanding the Problem
The scenario presented involves an if-else
construct where the last else
condition uses the assert.unreachable
function. Since the return type of assert.unreachable
is never
, you would expect TypeScript to understand that the flow of execution cannot proceed beyond this point. However, TypeScript raises an error: Variable 'result' is used before being assigned
.
This confusion arises because TypeScript aims for exhaustive checks in your control flow analysis. Hence, let’s first go over the usage of the never
type and how these errors can emerge in these scenarios.
Example of the Issue
Here’s your existing code snippet for context:
if (someCondition) {
result = $(document.createElement("div"));
} else if (anotherCondition) {
result = $(document.createElement("div"));
} else {
assert.unreachable("Unknown label in buildHTML.");
}
result.attr("data-childNumber", childNumber.toString());
The Problematic Function
The unreachable
function can be defined as:
export function unreachable(message?: string): never {
if (message === undefined) message = "Unreachable code reached.";
else message = "Unreachable code reached: " + message;
raiseTheAlarm(message);
}
Here, TypeScript raises another error: A function returning 'never' cannot have a reachable end point.
This error occurs because TypeScript detects a potential continuation of program logic after calling raiseTheAlarm
.
Solutions and Best Practices
To handle these issues more elegantly, we can consider the following solutions and best practices:
1. Explicitly Using throw
or return
While it may seem inelegant to use throw null;
or add a return
statement after raiseTheAlarm
, it is a valid way to signal the TypeScript compiler about unreachable code paths. Here's how you can revise your unreachable
function:
export function unreachable(message?: string): never {
if (message === undefined) message = "Unreachable code reached.";
else message = "Unreachable code reached: " + message;
raiseTheAlarm(message);
throw new Error(message); // or return;
}
By returning or throwing an error, you explicitly indicate that execution should not proceed beyond this point.
2. Using Type Assertions
If you're confident of your logic and want to express this type correctness to the TypeScript compiler, you can use type assertions when assigning to result
:
const result = (function() {
if (someCondition) {
return $(document.createElement("div"));
} else if (anotherCondition) {
return $(document.createElement("div"));
} else {
assert.unreachable("Unknown label in buildHTML.");
}
})() as JQuery;
Here, this constructs a self-executing function that creates result
only after your conditional checks are thoroughly evaluated. This ensures result
is assigned before any usage.
3. Employing Custom Errors for Clarity
You can also define custom error classes to throw when unreachable code is hit. This adds clarity and allows for better error handling:
class UnreachableError extends Error {
constructor(message: string) {
super(message);
this.name = 'UnreachableError';
}
}
export function unreachable(message?: string): never {
throw new UnreachableError(message || "Unreachable code reached.");
}
Using a specialized error class elevates your error-handling strategy considerably.
Conclusion
In TypeScript, correctly managing the never
type is essential for writing robust applications. By understanding how TypeScript evaluates control flow and using throw
, return
, or type assertions effectively, you can resolve issues surrounding unreachable code without compromising code elegance. Employing custom error classes can improve clarity and handling. With these strategies in hand, you'll be able to harness TypeScript's rigorous type system more effectively.
Frequently Asked Questions
What is the never
type in TypeScript?
The never
type in TypeScript indicates that a function does not return a value because it either throws an error or goes into an infinite loop.
Why does TypeScript raise an error for unreachable code?
TypeScript performs flow analysis to ensure all potential paths are covered. If it detects a point in the code that logically cannot be reached, it raises an error for clarity and type safety.
Can I ignore TypeScript errors regarding unreachable code?
While you technically can suppress TypeScript's error checks, it is not advisable since it can lead to hidden bugs and logic error risks.