How to Show AppBar on Scroll in Flutter?
Introduction Are you looking to implement a feature in Flutter where the AppBar is initially hidden and only appears when the user starts scrolling? This is a common UI requirement, especially for apps with rich content. In this guide, we'll walk you through the steps to achieve this using the SliverAppBar widget in Flutter. Understanding SliverAppBar The SliverAppBar is a part of the Sliver family in Flutter, which allows for scrolling effects that are not possible with the standard AppBar. It can expand, collapse, and be pinned at the top of the screen easily, which makes it suitable for complex scrollable layouts. The feature you want—starting with no AppBar and having it appear on scroll—can be effectively implemented with SliverAppBar. Why Use SliverAppBar? Using SliverAppBar is beneficial because it integrates with the CustomScrollView to create smooth scrolling effects. By leveraging this widget: You can create a header that scrolls away but remains accessible later. It provides customizable options for how the AppBar behaves during scroll. This gives you much more control compared to the traditional AppBar. Step-by-Step Implementation Let’s break down the implementation into simple steps. Step 1: Setting Up Your Flutter Project First, ensure that you have a Flutter project set up. You can create a new Flutter application using the command: flutter create my_app Navigate to your project folder: cd my_app Step 2: Update Your pubspec.yaml Make sure your Flutter SDK is up to date and include any necessary dependencies. For basic functionality, the Flutter SDK should suffice. Step 3: Implementing the SliverAppBar Here's how to create your main widget with SliverAppBar. Open the lib/main.dart file and replace its content with the following: import 'package:flutter/material.dart'; void main() { runApp(MyApp()); } class MyApp extends StatelessWidget { @override Widget build(BuildContext context) { return MaterialApp( home: HomePage(), ); } } class HomePage extends StatelessWidget { @override Widget build(BuildContext context) { return Scaffold( body: CustomScrollView( slivers: [ SliverAppBar( expandedHeight: 200.0, flexibleSpace: FlexibleSpaceBar( title: Text('My AppBar'), background: Image.network( 'https://example.com/your-image.jpg', fit: BoxFit.cover, ), ), pinned: true, floating: false, ), SliverList( delegate: SliverChildBuilderDelegate( (BuildContext context, int index) { return ListTile( title: Text('Item $index'), ); }, childCount: 50, ), ), ], ), ); } } Explanation of the Code CustomScrollView: This widget allows for a custom scroll behavior. It can contain one or more Sliver widgets. SliverAppBar: This is initialized with expandedHeight to define its size and includes a FlexibleSpaceBar to create an engaging AppBar. FlexibleSpaceBar: This widget allows you to manipulate the AppBar's content with scrolling. Here, we add a title and an example image. SliverList: This is used to display a scrollable list of items. Here, we are just generating a list for demonstration. Step 4: Customize Behavior The SliverAppBar's pinned: true property ensures that the AppBar will remain visible when scrolling ends. If set to false, it will fully disappear and reappear as you scroll back. To achieve exactly what you requested—starting with no AppBar and showing the AppBar on scroll—you can consider setting the initial visibility state programmatically, but typically this functionality needs more advanced state management, possibly with other widgets. Conclusion Implementing a SliverAppBar in Flutter is a robust way to control app headers based on scroll actions. By following the steps outlined above, you can create an engaging and visually appealing user experience. Try customizing the background image and the number of items in the list to suit your app's aesthetics and needs. Frequently Asked Questions (FAQs) How does SliverAppBar improve user experience? The SliverAppBar allows for dynamic UI functionality, adapting to user scroll behavior for better engagement. Can I use SliverAppBar with other scrollable widgets? Yes, SliverAppBar can be paired with various Sliver widgets like SliverGrid, SliverList, and others to create complex layouts. What if I need more control over AppBar visibility? You can implement additional state management solutions, like Provider, to control AppBar visibility more intricately based on other user inputs. Is it possible to animate AppBar appearance? While SliverAppBar handles some of this for you, you can manually customize animations using Flutter's animation libraries for advanced effec
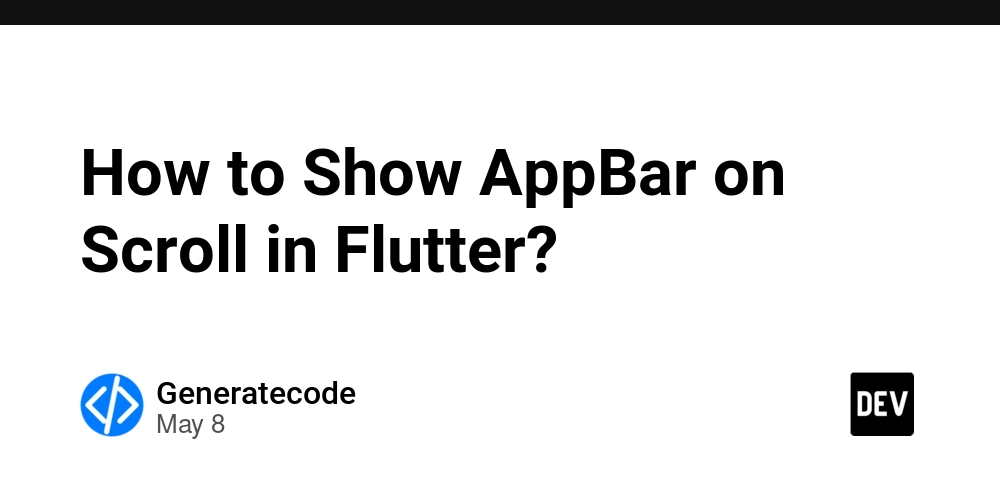
Introduction
Are you looking to implement a feature in Flutter where the AppBar is initially hidden and only appears when the user starts scrolling? This is a common UI requirement, especially for apps with rich content. In this guide, we'll walk you through the steps to achieve this using the SliverAppBar
widget in Flutter.
Understanding SliverAppBar
The SliverAppBar
is a part of the Sliver
family in Flutter, which allows for scrolling effects that are not possible with the standard AppBar. It can expand, collapse, and be pinned at the top of the screen easily, which makes it suitable for complex scrollable layouts. The feature you want—starting with no AppBar and having it appear on scroll—can be effectively implemented with SliverAppBar
.
Why Use SliverAppBar?
Using SliverAppBar
is beneficial because it integrates with the CustomScrollView
to create smooth scrolling effects. By leveraging this widget:
- You can create a header that scrolls away but remains accessible later.
- It provides customizable options for how the AppBar behaves during scroll.
This gives you much more control compared to the traditional AppBar.
Step-by-Step Implementation
Let’s break down the implementation into simple steps.
Step 1: Setting Up Your Flutter Project
First, ensure that you have a Flutter project set up. You can create a new Flutter application using the command:
flutter create my_app
Navigate to your project folder:
cd my_app
Step 2: Update Your pubspec.yaml
Make sure your Flutter SDK is up to date and include any necessary dependencies. For basic functionality, the Flutter SDK should suffice.
Step 3: Implementing the SliverAppBar
Here's how to create your main widget with SliverAppBar
. Open the lib/main.dart
file and replace its content with the following:
import 'package:flutter/material.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: HomePage(),
);
}
}
class HomePage extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Scaffold(
body: CustomScrollView(
slivers: [
SliverAppBar(
expandedHeight: 200.0,
flexibleSpace: FlexibleSpaceBar(
title: Text('My AppBar'),
background: Image.network(
'https://example.com/your-image.jpg',
fit: BoxFit.cover,
),
),
pinned: true,
floating: false,
),
SliverList(
delegate: SliverChildBuilderDelegate(
(BuildContext context, int index) {
return ListTile(
title: Text('Item $index'),
);
},
childCount: 50,
),
),
],
),
);
}
}
Explanation of the Code
-
CustomScrollView: This widget allows for a custom scroll behavior. It can contain one or more
Sliver
widgets. -
SliverAppBar: This is initialized with
expandedHeight
to define its size and includes aFlexibleSpaceBar
to create an engaging AppBar. - FlexibleSpaceBar: This widget allows you to manipulate the AppBar's content with scrolling. Here, we add a title and an example image.
- SliverList: This is used to display a scrollable list of items. Here, we are just generating a list for demonstration.
Step 4: Customize Behavior
The SliverAppBar
's pinned: true
property ensures that the AppBar will remain visible when scrolling ends. If set to false
, it will fully disappear and reappear as you scroll back.
To achieve exactly what you requested—starting with no AppBar and showing the AppBar on scroll—you can consider setting the initial visibility state programmatically, but typically this functionality needs more advanced state management, possibly with other widgets.
Conclusion
Implementing a SliverAppBar
in Flutter is a robust way to control app headers based on scroll actions. By following the steps outlined above, you can create an engaging and visually appealing user experience. Try customizing the background image and the number of items in the list to suit your app's aesthetics and needs.
Frequently Asked Questions (FAQs)
How does SliverAppBar improve user experience?
The SliverAppBar
allows for dynamic UI functionality, adapting to user scroll behavior for better engagement.
Can I use SliverAppBar with other scrollable widgets?
Yes, SliverAppBar
can be paired with various Sliver
widgets like SliverGrid
, SliverList
, and others to create complex layouts.
What if I need more control over AppBar visibility?
You can implement additional state management solutions, like Provider, to control AppBar visibility more intricately based on other user inputs.
Is it possible to animate AppBar appearance?
While SliverAppBar
handles some of this for you, you can manually customize animations using Flutter's animation libraries for advanced effects.
Now you have a solid foundation to implement your desired AppBar behavior in Flutter with ease!