Introduction to TensorFlow with real code examples
# Introduction to TensorFlow with Real Code Examples Hello, fellow developers! Today, we're going to embark on an exciting journey into the world of Machine Learning (ML) using one of the most popular open-source ML libraries - TensorFlow. This post will guide you through setting up your environment, understanding its core concepts, and providing real code examples to help you get started with this powerful tool. Prerequisites Before we dive in, let's ensure that you have the following prerequisites met: Familiarity with Python programming language Basic understanding of Machine Learning concepts A working development environment on your machine (MacOS/Linux/Windows) Installing TensorFlow Using pip You can install TensorFlow using pip, which is a package manager for Python. Here's the command you need to run in your terminal or command prompt: pip install tensorflow If you want to use GPU-accelerated computations, ensure that CUDA is installed on your machine and use the following command instead: pip install tensorflow-gpu Using Colab (Google's Jupyter notebook environment) If you don't want to set up TensorFlow locally, you can use Google Colab, which comes with a GPU by default. You can access it via this link. Understanding TensorFlow TensorFlow is an end-to-end open-source platform for ML. It consists of: TensorFlow Core: The primary library that provides the basic functionality of constructing and running computational graphs. TensorFlow Lite: A lightweight version for mobile and embedded devices. TensorFlow Hub: A repository of pre-trained ML models, tools, and utilities to help you easily integrate them into your projects. TensorBoard: A powerful visualization tool for exploring and understanding the structure and performance of your ML models. Creating Your First TensorFlow Program Let's create a simple neural network using TensorFlow to approximate the function y = x^2. Here's the code: import tensorflow as tf from tensorflow import keras # Define input and output shapes for our model x_input = tf.keras.Input(shape=(1,)) y_output = keras.layers.Dense(units=1)(x_input) # Create a model instance model = keras.Model(inputs=x_input, outputs=y_output) # Compile the model with Mean Squared Error loss function and Adam optimizer model.compile(optimizer='adam', loss='mean_squared_error') # Create a dataset data = tf.data.Dataset.from_tensor_slices((tf.random.uniform((1000, 1), maxval=100), tf.range(100))) data = data.shuffle(1000).batch(32) # Train the model for a specified number of epochs model.fit(data, epochs=50) This code creates a simple neural network with one input (x), one output (y), and one hidden layer (Dense layer). It then compiles the model with the Mean Squared Error loss function and Adam optimizer. After creating a dataset for training, it trains the model for 50 epochs. We hope this introduction to TensorFlow has been helpful! In future posts, we'll explore more advanced topics such as building convolutional neural networks, using TensorBoard, and integrating TensorFlow with other AI tools. Stay tuned! Happy coding! :)
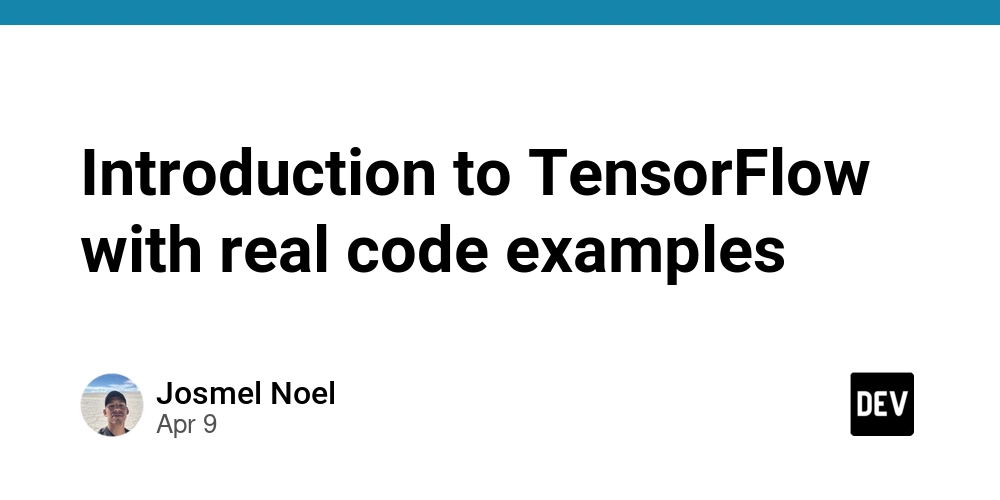
# Introduction to TensorFlow with Real Code Examples
Hello, fellow developers! Today, we're going to embark on an exciting journey into the world of Machine Learning (ML) using one of the most popular open-source ML libraries - TensorFlow. This post will guide you through setting up your environment, understanding its core concepts, and providing real code examples to help you get started with this powerful tool.
Prerequisites
Before we dive in, let's ensure that you have the following prerequisites met:
- Familiarity with Python programming language
- Basic understanding of Machine Learning concepts
- A working development environment on your machine (MacOS/Linux/Windows)
Installing TensorFlow
Using pip
You can install TensorFlow using pip, which is a package manager for Python. Here's the command you need to run in your terminal or command prompt:
pip install tensorflow
If you want to use GPU-accelerated computations, ensure that CUDA is installed on your machine and use the following command instead:
pip install tensorflow-gpu
Using Colab (Google's Jupyter notebook environment)
If you don't want to set up TensorFlow locally, you can use Google Colab, which comes with a GPU by default. You can access it via this link.
Understanding TensorFlow
TensorFlow is an end-to-end open-source platform for ML. It consists of:
- TensorFlow Core: The primary library that provides the basic functionality of constructing and running computational graphs.
- TensorFlow Lite: A lightweight version for mobile and embedded devices.
- TensorFlow Hub: A repository of pre-trained ML models, tools, and utilities to help you easily integrate them into your projects.
- TensorBoard: A powerful visualization tool for exploring and understanding the structure and performance of your ML models.
Creating Your First TensorFlow Program
Let's create a simple neural network using TensorFlow to approximate the function y = x^2
. Here's the code:
import tensorflow as tf
from tensorflow import keras
# Define input and output shapes for our model
x_input = tf.keras.Input(shape=(1,))
y_output = keras.layers.Dense(units=1)(x_input)
# Create a model instance
model = keras.Model(inputs=x_input, outputs=y_output)
# Compile the model with Mean Squared Error loss function and Adam optimizer
model.compile(optimizer='adam', loss='mean_squared_error')
# Create a dataset
data = tf.data.Dataset.from_tensor_slices((tf.random.uniform((1000, 1), maxval=100), tf.range(100)))
data = data.shuffle(1000).batch(32)
# Train the model for a specified number of epochs
model.fit(data, epochs=50)
This code creates a simple neural network with one input (x), one output (y), and one hidden layer (Dense layer). It then compiles the model with the Mean Squared Error loss function and Adam optimizer. After creating a dataset for training, it trains the model for 50 epochs.
We hope this introduction to TensorFlow has been helpful! In future posts, we'll explore more advanced topics such as building convolutional neural networks, using TensorBoard, and integrating TensorFlow with other AI tools. Stay tuned!
Happy coding! :)