Building C# Web and Console Applications: A Hands-On Guide with a Guessing Game
Introduction C# is a versatile programming language widely used for developing various types of applications, including web and console applications. The .NET framework and .NET Core provide robust environments for building scalable, high-performance applications. This guide outlines the steps for creating a C# web application using ASP.NET Core and a C# console application using .NET. As part of this tutorial, we will build a simple Guessing Game for both console and web environments. This will demonstrate how to implement user interaction, logic processing, and UI handling in different application types. 1. Creating a C# Console Application A console application is a simple, lightweight program that runs in a command-line interface. It is typically used for utilities, automation scripts, or testing. Prerequisites Install .NET SDK from Microsoft Use an IDE such as Visual Studio, Visual Studio Code, or JetBrains Rider Steps to Create a Console Application Step 1: Open Command Prompt or Terminal Run the following command to create a new console application: dotnet new console -n MyConsoleApp Step 2: Navigate to the Project Directory cd MyConsoleApp Step 3: Open and Modify Program.cs We will implement a simple guessing game where the user must guess a randomly generated number. using System; class GuessingGame { static void Main() { Random random = new Random(); int numberToGuess = random.Next(1, 101); int userGuess = 0; int attempts = 0; Console.WriteLine("Welcome to the Guessing Game!"); Console.WriteLine("Try to guess the number between 1 and 100."); while (userGuess != numberToGuess) { Console.Write("Enter your guess: "); string input = Console.ReadLine(); // Validate input if (!int.TryParse(input, out userGuess) || userGuess 100) { Console.WriteLine("Invalid input. Please enter a number between 1 and 100."); continue; } attempts++; if (userGuess numberToGuess) Console.WriteLine("Too high! Try again."); else Console.WriteLine($"Congratulations! You guessed it right in {attempts} attempts."); } } } Step 4: Build and Run the Application Before running the modified code, build the application using: dotnet build Then run the application: dotnet run 2 Creating a C# Web Application using ASP.NET Core A web application is a more complex system that runs on a web server and interacts with users through a web browser. Prerequisites Install the latest .NET SDK Install Visual Studio (with ASP.NET and Web Development workload) Basic knowledge of HTML, CSS, and JavaScript Steps to Create a Web Application Step 1: Open Visual Studio and create a new project. dotnet new webapp -n MyWebApp Step 2: Navigate to the Project Directory cd MyWebApp Step 3: Open Program.cs and Modify It We will implement a simple web-based guessing game using ASP.NET Core Razor Pages. using Microsoft.AspNetCore.Builder; using Microsoft.AspNetCore.Http; using Microsoft.Extensions.Hosting; using System; var builder = WebApplication.CreateBuilder(args); var app = builder.Build(); app.UseHttpsRedirection(); // Generate a random number to guess int numberToGuess = new Random().Next(1, 101); int attempts = 0; // Serve the game page and process guesses app.Map("/", async context => { string resultMessage = ""; // This will hold feedback messages // Only process form data if it's a POST request if (context.Request.Method == "POST") { var form = await context.Request.ReadFormAsync(); string guessInput = form["userGuess"]; if (!string.IsNullOrEmpty(guessInput) && int.TryParse(guessInput, out int userGuess)) { attempts++; if (userGuess 100) resultMessage = " Invalid input! Please enter a number between 1 and 100."; else if (userGuess numberToGuess) resultMessage = " Too high! Try again."; else { resultMessage = $" Congratulations! You guessed it right in {attempts} attempts."; numberToGuess = new Random().Next(1, 101); // Reset the game attempts = 0; } } } // HTML structure string html = $@" Guessing Game Welcome to the Web Guessing Game! Guess a number between 1 and 100. Guess {resultMessage} "; context.Response.ContentType = "text/html"; // Ensure correct content type await context.Response.WriteAsync(html); }); a
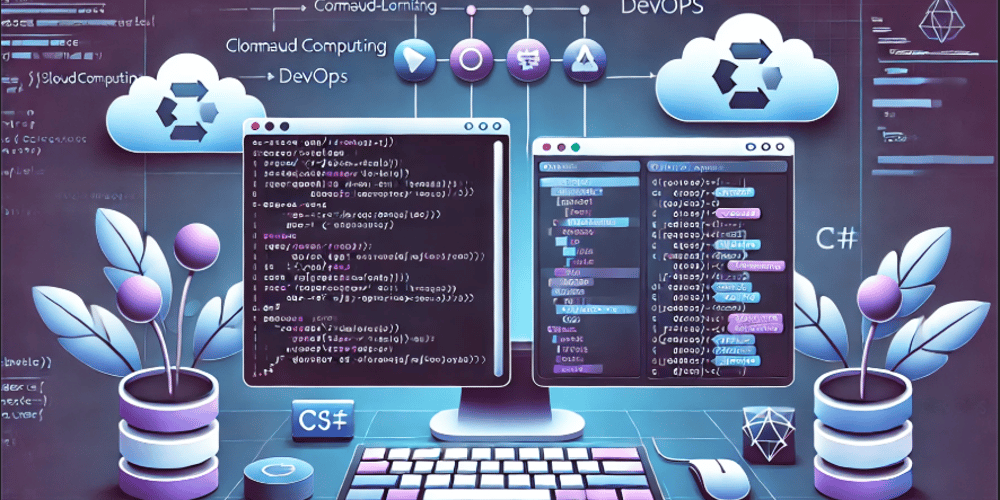
Introduction
C# is a versatile programming language widely used for developing various types of applications, including web and console applications. The .NET framework and .NET Core provide robust environments for building scalable, high-performance applications.
This guide outlines the steps for creating a C# web application using ASP.NET Core and a C# console application using .NET.
As part of this tutorial, we will build a simple Guessing Game for both console and web environments. This will demonstrate how to implement user interaction, logic processing, and UI handling in different application types.
1. Creating a C# Console Application
A console application is a simple, lightweight program that runs in a command-line interface. It is typically used for utilities, automation scripts, or testing.
Prerequisites
- Install .NET SDK from Microsoft
- Use an IDE such as Visual Studio, Visual Studio Code, or JetBrains Rider
Steps to Create a Console Application
Step 1: Open Command Prompt or Terminal
Run the following command to create a new console application:
dotnet new console -n MyConsoleApp
Step 2: Navigate to the Project Directory
cd MyConsoleApp
Step 3: Open and Modify Program.cs
We will implement a simple guessing game where the user must guess a randomly generated number.
using System;
class GuessingGame
{
static void Main()
{
Random random = new Random();
int numberToGuess = random.Next(1, 101);
int userGuess = 0;
int attempts = 0;
Console.WriteLine("Welcome to the Guessing Game!");
Console.WriteLine("Try to guess the number between 1 and 100.");
while (userGuess != numberToGuess)
{
Console.Write("Enter your guess: ");
string input = Console.ReadLine();
// Validate input
if (!int.TryParse(input, out userGuess) || userGuess < 1 || userGuess > 100)
{
Console.WriteLine("Invalid input. Please enter a number between 1 and 100.");
continue;
}
attempts++;
if (userGuess < numberToGuess)
Console.WriteLine("Too low! Try again.");
else if (userGuess > numberToGuess)
Console.WriteLine("Too high! Try again.");
else
Console.WriteLine($"Congratulations! You guessed it right in {attempts} attempts.");
}
}
}
Step 4: Build and Run the Application
Before running the modified code, build the application using:
dotnet build
Then run the application:
dotnet run
2 Creating a C# Web Application using ASP.NET Core
A web application is a more complex system that runs on a web server and interacts with users through a web browser.
Prerequisites
- Install the latest .NET SDK
- Install Visual Studio (with ASP.NET and Web Development workload)
- Basic knowledge of HTML, CSS, and JavaScript
Steps to Create a Web Application
Step 1: Open Visual Studio and create a new project.
dotnet new webapp -n MyWebApp
Step 2: Navigate to the Project Directory
cd MyWebApp
Step 3: Open Program.cs
and Modify It
We will implement a simple web-based guessing game using ASP.NET Core Razor Pages.
using Microsoft.AspNetCore.Builder;
using Microsoft.AspNetCore.Http;
using Microsoft.Extensions.Hosting;
using System;
var builder = WebApplication.CreateBuilder(args);
var app = builder.Build();
app.UseHttpsRedirection();
// Generate a random number to guess
int numberToGuess = new Random().Next(1, 101);
int attempts = 0;
// Serve the game page and process guesses
app.Map("/", async context =>
{
string resultMessage = ""; // This will hold feedback messages
// Only process form data if it's a POST request
if (context.Request.Method == "POST")
{
var form = await context.Request.ReadFormAsync();
string guessInput = form["userGuess"];
if (!string.IsNullOrEmpty(guessInput) && int.TryParse(guessInput, out int userGuess))
{
attempts++;
if (userGuess < 1 || userGuess > 100)
resultMessage = " Invalid input! Please enter a number between 1 and 100."
;
else if (userGuess < numberToGuess)
resultMessage = " Too low! Try again."
;
else if (userGuess > numberToGuess)
resultMessage = " Too high! Try again."
;
else
{
resultMessage = $" Congratulations! You guessed it right in
{attempts} attempts.";
numberToGuess = new Random().Next(1, 101); // Reset the game
attempts = 0;
}
}
}
// HTML structure
string html = $@"
Guessing Game
Welcome to the Web Guessing Game!
Guess a number between 1 and 100.
{resultMessage}
";
context.Response.ContentType = "text/html"; // Ensure correct content type
await context.Response.WriteAsync(html);
});
app.Run();
Step 4: Build and Run the Application
Before running the modified code, build the application using:
dotnet build
Then run the application:
dotnet run
After running, the application will be accessible at https://localhost:5001 (or as indicated in the console output).
3 Differences Between Console and Web Applications
Feature | Console Application | Web Application |
---|---|---|
UI | Command-line | Web-based |
User Interaction | Text input/output | Browser-based interface |
Use Case | Automation, testing, scripts | Web services, websites |
Framework | .NET Console | ASP.NET Core |
4 Explanation of Commands Used
Command | Description |
---|---|
dotnet new console -n MyConsoleApp |
Creates a new console application project named MyConsoleApp . |
dotnet new webapp -n MyWebApp |
Creates a new web application project named MyWebApp . |
cd MyConsoleApp / cd MyWebApp
|
Changes the directory to the specified project folder. |
dotnet build |
Compiles the project, checking for errors and generating necessary binaries. |
dotnet run |
Runs the application after it has been built. |
This guide has provided a comprehensive, hands-on approach to building both C# console and web applications, highlighting their unique strengths and use cases.
Through the Guessing Game project, we explored how applications handle user interaction, logic processing, and UI design across different environments.
- Console applications are lightweight, efficient, and ideal for automation, scripting, and quick prototyping.
- Web applications offer a more interactive, browser-based experience, making them suitable for dynamic and scalable solutions.
By harnessing the robust capabilities of .NET, developers can create high-performance applications that cater to a wide range of business and technical needs. Whether building simple command-line tools or full-fledged web applications, mastering these concepts will provide a strong foundation for future development endeavors.
Keep learning, keep building!