Optimizing Multithreading Performance in Java: Best Practices and Techniques
Multithreading is a powerful feature in Java that enables applications to execute multiple tasks concurrently, thereby improving efficiency and responsiveness. However, if not implemented correctly, multithreading can introduce performance bottlenecks and synchronization issues. In this Java tutorial, we will explore the best practices and techniques for optimizing multithreading performance in Java, ensuring that applications run smoothly and efficiently. Understanding Multithreading in Java Java provides built-in support for multithreading through the Thread class and the Runnable interface. The core concept of multithreading involves creating multiple threads that can execute tasks simultaneously. While this increases the application's efficiency, it can also introduce complexity related to thread synchronization, resource sharing, and deadlocks. Optimizing multithreading performance in Java requires a structured approach that minimizes synchronization overhead, reduces contention, and leverages available computing resources effectively. Below are some essential techniques to achieve optimal performance. Best Practices for Multithreading Optimization 1. Minimize Synchronization Overhead Synchronization ensures that multiple threads do not simultaneously access shared resources, preventing data inconsistency. However, excessive synchronization can lead to performance degradation due to increased blocking time. To optimize synchronization: Use synchronized blocks selectively instead of synchronizing entire methods. Consider using ReentrantLock for better performance and flexibility. Employ volatile for simple flag updates instead of full synchronization. Use concurrent data structures from the java.util.concurrent package. 2. Avoid Thread Contention Thread contention occurs when multiple threads compete for shared resources, leading to performance bottlenecks. Reducing contention can enhance efficiency. Strategies include: Partitioning workloads to minimize resource contention. Using thread-local variables to keep data independent per thread. Implementing read-write locks (ReentrantReadWriteLock) where applicable. 3. Use Efficient Thread Pooling Creating new threads frequently can be costly in terms of memory and CPU usage. Using a thread pool efficiently manages a fixed number of worker threads that handle multiple tasks. Best practices include: Using ExecutorService to manage thread pools. Selecting appropriate thread pool sizes based on CPU cores and workload. Choosing fixed thread pools for consistent workloads and cached thread pools for bursty workloads. 4. Avoid Deadlocks Deadlocks occur when two or more threads are waiting indefinitely for each other's resources, causing the application to freeze. To prevent deadlocks: Always acquire locks in a consistent order. Avoid nested locks where possible. Use timeouts with lock acquisition (tryLock method). 5. Reduce Context Switching Frequent context switching can reduce the efficiency of a multithreaded application. Since switching between threads involves CPU overhead, reducing unnecessary switching enhances performance. To achieve this: Assign long-running tasks to dedicated threads. Group similar tasks to run within the same thread. Avoid excessive thread creation and destruction. 6. Leverage Non-Blocking Algorithms Using non-blocking algorithms and data structures can significantly improve multithreading performance. Java provides several non-blocking constructs such as: AtomicInteger, AtomicLong, and AtomicReference from java.util.concurrent.atomic. ConcurrentHashMap for better concurrency without blocking. 7. Utilize Parallel Streams and Fork/Join Framework Java 8 introduced parallel streams and the Fork/Join framework, which help distribute workloads across multiple threads efficiently. However, using them incorrectly can lead to suboptimal performance. Best practices include: Using parallel streams only for computationally intensive tasks. Avoiding parallel processing for small tasks as the overhead might outweigh the benefits. Using the ForkJoinPool for recursive task processing. 8. Profile and Monitor Thread Performance Optimizing multithreading performance in Java requires continuous profiling and monitoring. Tools like JVisualVM, JConsole, and Java Mission Control provide insights into thread behavior, helping detect bottlenecks. Regularly monitoring thread activity ensures: Identification of high CPU usage by specific threads. Detection of thread starvation and deadlocks. Proper balancing of workloads among available threads. Conclusion Optimizing multithreading performance in Java is essential for developing high-performance applications. By following best practices such as minimizing synchronization overhead, avoiding thread contention, leveraging thread pools, and using non-blocking a
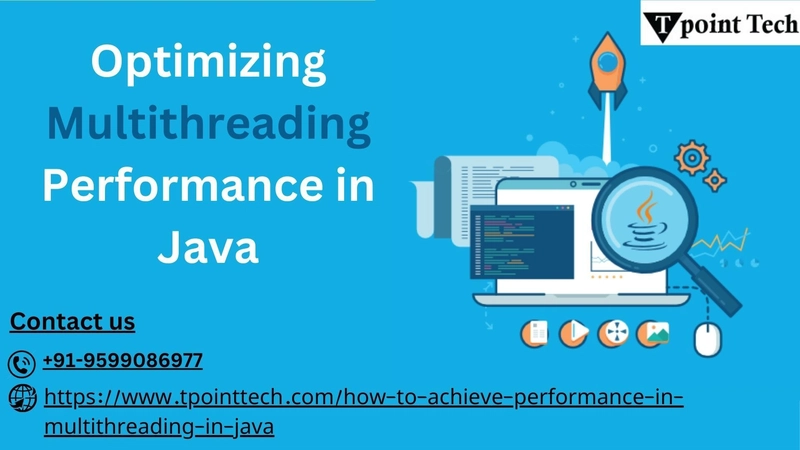
Multithreading is a powerful feature in Java that enables applications to execute multiple tasks concurrently, thereby improving efficiency and responsiveness. However, if not implemented correctly, multithreading can introduce performance bottlenecks and synchronization issues. In this Java tutorial, we will explore the best practices and techniques for optimizing multithreading performance in Java, ensuring that applications run smoothly and efficiently.
Understanding Multithreading in Java
Java provides built-in support for multithreading through the Thread class and the Runnable interface. The core concept of multithreading involves creating multiple threads that can execute tasks simultaneously. While this increases the application's efficiency, it can also introduce complexity related to thread synchronization, resource sharing, and deadlocks.
Optimizing multithreading performance in Java requires a structured approach that minimizes synchronization overhead, reduces contention, and leverages available computing resources effectively. Below are some essential techniques to achieve optimal performance.
Best Practices for Multithreading Optimization
1. Minimize Synchronization Overhead
Synchronization ensures that multiple threads do not simultaneously access shared resources, preventing data inconsistency. However, excessive synchronization can lead to performance degradation due to increased blocking time. To optimize synchronization:
- Use synchronized blocks selectively instead of synchronizing entire methods.
- Consider using ReentrantLock for better performance and flexibility.
- Employ volatile for simple flag updates instead of full synchronization.
- Use concurrent data structures from the java.util.concurrent package.
2. Avoid Thread Contention
Thread contention occurs when multiple threads compete for shared resources, leading to performance bottlenecks. Reducing contention can enhance efficiency. Strategies include:
- Partitioning workloads to minimize resource contention.
- Using thread-local variables to keep data independent per thread.
- Implementing read-write locks (ReentrantReadWriteLock) where applicable.
3. Use Efficient Thread Pooling
Creating new threads frequently can be costly in terms of memory and CPU usage. Using a thread pool efficiently manages a fixed number of worker threads that handle multiple tasks. Best practices include:
- Using ExecutorService to manage thread pools.
- Selecting appropriate thread pool sizes based on CPU cores and workload.
- Choosing fixed thread pools for consistent workloads and cached thread pools for bursty workloads.
4. Avoid Deadlocks
Deadlocks occur when two or more threads are waiting indefinitely for each other's resources, causing the application to freeze. To prevent deadlocks:
- Always acquire locks in a consistent order.
- Avoid nested locks where possible.
- Use timeouts with lock acquisition (tryLock method).
5. Reduce Context Switching
Frequent context switching can reduce the efficiency of a multithreaded application. Since switching between threads involves CPU overhead, reducing unnecessary switching enhances performance. To achieve this:
- Assign long-running tasks to dedicated threads.
- Group similar tasks to run within the same thread.
- Avoid excessive thread creation and destruction.
6. Leverage Non-Blocking Algorithms
Using non-blocking algorithms and data structures can significantly improve multithreading performance. Java provides several non-blocking constructs such as:
- AtomicInteger, AtomicLong, and AtomicReference from java.util.concurrent.atomic.
- ConcurrentHashMap for better concurrency without blocking.
7. Utilize Parallel Streams and Fork/Join Framework
Java 8 introduced parallel streams and the Fork/Join framework, which help distribute workloads across multiple threads efficiently. However, using them incorrectly can lead to suboptimal performance. Best practices include:
- Using parallel streams only for computationally intensive tasks.
- Avoiding parallel processing for small tasks as the overhead might outweigh the benefits.
- Using the ForkJoinPool for recursive task processing.
8. Profile and Monitor Thread Performance
Optimizing multithreading performance in Java requires continuous profiling and monitoring. Tools like JVisualVM, JConsole, and Java Mission Control provide insights into thread behavior, helping detect bottlenecks. Regularly monitoring thread activity ensures:
- Identification of high CPU usage by specific threads.
- Detection of thread starvation and deadlocks.
- Proper balancing of workloads among available threads.
Conclusion
Optimizing multithreading performance in Java is essential for developing high-performance applications. By following best practices such as minimizing synchronization overhead, avoiding thread contention, leveraging thread pools, and using non-blocking algorithms, developers can ensure efficient parallel execution. Additionally, profiling and monitoring thread behavior can help identify potential issues early, leading to smoother application performance.
Understanding and applying these techniques will enhance Java applications' scalability, responsiveness, and overall efficiency, making them well-suited for modern multi-core environments. Whether you are building a high-performance server application or a real-time processing system, these optimization strategies will help you achieve better multithreading performance in Java.