The Adventures of Blink S3e3: Branching into the Git Multiverse
Hey friends, welcome back to the Adventures of Blink! This week's adventure continues our deep-dive into the world of source control. Last week we talked about how to commit our code and save our changes in our git repository, but this doesn't answer a fundamental question: how do we experiment in our code? Youtube, if you'd rather watch than read Experimentation can be messy If I already have my entire project solved and I'm just writing the code, what we learned last week is pretty much all I need. As long as I can commit, my code will be safe in git. But what if I don't know for sure how I'm going to solve my problem? It would be helpful to be able to experiment... and the result of an experiment could be anything, from committing my newly-found solution all the way to throwing everything out and starting over. Further - if I'm not the only programmer working on this problem, we may need to commit several changes from several people simultaneously to try out a potential solution to a problem, only to have to remove it all if it doesn't work. This is going to get icky, fast. What we need is a copy If I want to conduct a risky experiment, it would be great to have the ability to work in a copy of my code, and then accept that copy only if the experiment succeeds. Git calls this concept a branch. With branches, we can keep multiple flavors of our codebase in the same repository, and we can even put them together at a later point if we want. Seeing where we are The first command we need to learn is git branch. This will allow us to see the 'lay of the land' - it shows us what branches are in the repository and which one we have checked out currently. When you first create a git repository, it creates a default branch (usually called 'main'). From that point on, you'll have 'main' checked out until you manually initiate a switch to another branch. However, you'll need to create another branch to move to, because no other branches exist in your repository yet! Creating a branch There are a couple of ways to create a new branch, but the easiest one in my opinion is git checkout -b . This is nice because checkout will cause you to switch to your new branch when it's created, and that's almost always what you want to do next! Switching between branches I kinda gave it away in the last one, but switching to a branch is accomplished by git checkout . The only time this won't work is when you have new files added but not yet committed, you'll be prompted to stash those. Merging branches If you want to merge a branch into another, it's a very easy command - git merge . But this comes with rules: You have to have the destination branch checked out. If you're merging "feature1" into your "main", you should have main checked out Merges only bring things that are committed. If you've written code but haven't committed it, it's not yet attached to a branch, so it will go nowhere. Always check git status to be sure you're not leaving edits behind! Cleaning up after yourself Merging a branch does not eliminate the branch - it's still out there, kept separate, available if you need it. But at some point you'll want to delete the branch because it's just clutter... so you'll do this: git branch -D . This will delete the branch altogether. BE CAREFUL! If you haven't merged it into another branch already, you'll lose EVERYTHING on this branch when you delete it! Merge Conflicts Once you have branches, you have the potential for synchronization issues. Eventually you'll have a branch that gets out of sync with main... or with another branch... and you'll need to be able to bring them back together. The cool part: git will actually help you with this! When you attempt to merge something and create a conflict, git locates the conflicting changes and marks them for you... then you can easily resolve the merge and make a new commit with your resolution. Side note: Release Managers It's common in large organizations to have a "Releases" team or something similar... folks who oversee the deployment of the code. They're not typically the folks who created the code - they just handle releases. This is a bad idea, for reasons that I hope are obvious to you here. Think about it: if something goes wrong with this code, who's going to be able to resolve the problem fastest? The person who wrote it, or a release manager who was handed this big change and told to deploy it? Avoiding Conflict Even though merge conflicts can be resolved relatively easily, it would still be better to not have them. What are some ways we can prevent conflicts from arising? Good modular hygiene: Git only detects conflicts if they're within the same file. So if we've done a good job of making our code modular, separating concerns and making it so everything isn't mashed together in a giant ball
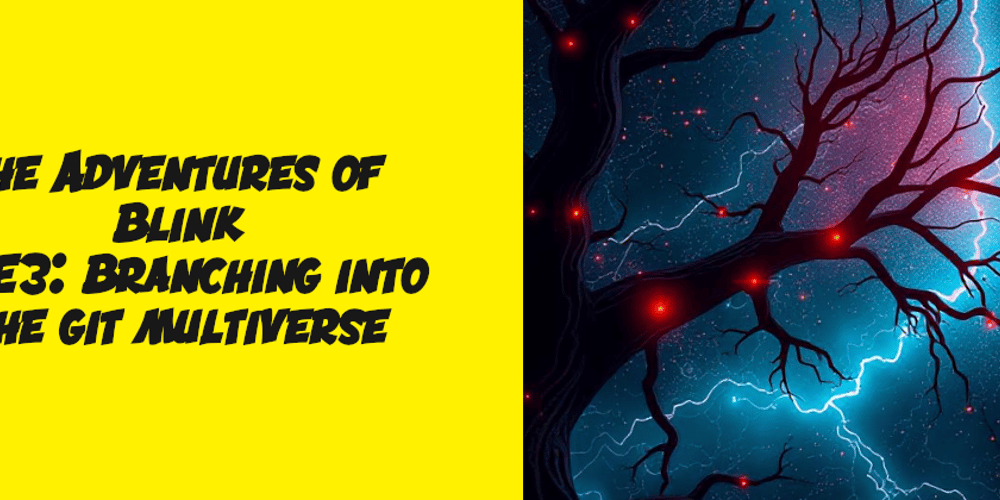
Hey friends, welcome back to the Adventures of Blink! This week's adventure continues our deep-dive into the world of source control. Last week we talked about how to commit our code and save our changes in our git repository, but this doesn't answer a fundamental question: how do we experiment in our code?
Youtube, if you'd rather watch than read
Experimentation can be messy
If I already have my entire project solved and I'm just writing the code, what we learned last week is pretty much all I need. As long as I can commit, my code will be safe in git. But what if I don't know for sure how I'm going to solve my problem? It would be helpful to be able to experiment... and the result of an experiment could be anything, from committing my newly-found solution all the way to throwing everything out and starting over. Further - if I'm not the only programmer working on this problem, we may need to commit several changes from several people simultaneously to try out a potential solution to a problem, only to have to remove it all if it doesn't work. This is going to get icky, fast.
What we need is a copy
If I want to conduct a risky experiment, it would be great to have the ability to work in a copy of my code, and then accept that copy only if the experiment succeeds. Git calls this concept a branch. With branches, we can keep multiple flavors of our codebase in the same repository, and we can even put them together at a later point if we want.
Seeing where we are
The first command we need to learn is git branch
. This will allow us to see the 'lay of the land' - it shows us what branches are in the repository and which one we have checked out currently.
When you first create a git repository, it creates a default branch (usually called 'main'). From that point on, you'll have 'main' checked out until you manually initiate a switch to another branch. However, you'll need to create another branch to move to, because no other branches exist in your repository yet!
Creating a branch
There are a couple of ways to create a new branch, but the easiest one in my opinion is git checkout -b
. This is nice because checkout will cause you to switch to your new branch when it's created, and that's almost always what you want to do next!
Switching between branches
I kinda gave it away in the last one, but switching to a branch is accomplished by git checkout
. The only time this won't work is when you have new files added but not yet committed, you'll be prompted to stash those.
Merging branches
If you want to merge a branch into another, it's a very easy command - git merge
. But this comes with rules:
- You have to have the destination branch checked out. If you're merging "feature1" into your "main", you should have main checked out
- Merges only bring things that are committed. If you've written code but haven't committed it, it's not yet attached to a branch, so it will go nowhere. Always check
git status
to be sure you're not leaving edits behind!
Cleaning up after yourself
Merging a branch does not eliminate the branch - it's still out there, kept separate, available if you need it. But at some point you'll want to delete the branch because it's just clutter... so you'll do this: git branch -D
. This will delete the branch altogether. BE CAREFUL! If you haven't merged it into another branch already, you'll lose EVERYTHING on this branch when you delete it!
Merge Conflicts
Once you have branches, you have the potential for synchronization issues. Eventually you'll have a branch that gets out of sync with main... or with another branch... and you'll need to be able to bring them back together.
The cool part: git will actually help you with this! When you attempt to merge something and create a conflict, git locates the conflicting changes and marks them for you... then you can easily resolve the merge and make a new commit with your resolution.
Side note: Release Managers
It's common in large organizations to have a "Releases" team or something similar... folks who oversee the deployment of the code. They're not typically the folks who created the code - they just handle releases.
This is a bad idea, for reasons that I hope are obvious to you here. Think about it: if something goes wrong with this code, who's going to be able to resolve the problem fastest? The person who wrote it, or a release manager who was handed this big change and told to deploy it?
Avoiding Conflict
Even though merge conflicts can be resolved relatively easily, it would still be better to not have them. What are some ways we can prevent conflicts from arising?
Good modular hygiene: Git only detects conflicts if they're within the same file. So if we've done a good job of making our code modular, separating concerns and making it so everything isn't mashed together in a giant ball of spaghetti-code, we'll avoid lots of conflicts because you can work on module X while I work on module Y and we shouldn't have to be in the same files very much.
Small, frequent commits: The longer your branch exists, the more likely someone will make a change somewhere else that conflicts with it. Don't let your branch live for a long time - create it for a single purpose, do that small amount of work, and merge it as quickly as you can. Then make a new branch for the next work item. This won't eliminate all chance of conflict, but it definitely tilts the table in your favor. We've covered this in a previous adventure... it's called Continuous Integration.
Wrapping up
That brings this Adventure of Blink to a conclusion - I hope you're enjoying learning alongside me! Make sure you follow / subscribe so you'll be alerted to new episodes... tune in next week as we join our local git with GitHub and GitLab! See you soon!