The Complete TypeScript Tutorial
Are you a JavaScript developer looking to build more robust, scalable, and maintainable applications? Do you find yourself debugging frustrating runtime errors that could have been caught earlier? If so, it's time to explore TypeScript, a powerful superset of JavaScript that adds optional static typing. This typescript tutorial will give you a comprehensive overview of what TypeScript is, why it's become so popular, and the core concepts you need to understand to get started. For years, JavaScript's dynamic nature has been both a blessing and a curse. Its flexibility allows for rapid prototyping and development, but as projects grow in size and complexity, this dynamism can lead to unexpected bugs and make code harder to understand and refactor. This is where TypeScript shines. It provides a layer of type safety on top of JavaScript, allowing you to define the shape of your data and catch type-related errors during the development phase, before your code even runs in the browser or on the server. What Exactly is TypeScript? At its heart, TypeScript is simply JavaScript with extra features. It's a superset, meaning all valid JavaScript code is also valid TypeScript code. The key addition is the static type system. You can annotate your variables, function parameters, and return values with types (like string, number, boolean, array, object, etc.). The TypeScript compiler (tsc) then checks for type compatibility. If there's a mismatch, it throws a compilation error, alerting you to potential issues immediately. Think of it like adding a blueprint to your construction project. JavaScript lets you start building immediately, figuring things out as you go. TypeScript encourages you to define your structure upfront, making the build process smoother and the final structure more stable and predictable. Why Should You Learn TypeScript? The benefits of adopting TypeScript are numerous and significant, especially for larger projects or teams: Catch Errors Early: This is arguably the biggest win. Instead of encountering TypeErrors or undefined is not a function errors at runtime, the TypeScript compiler will flag these issues before you even test your code. This saves immense debugging time and effort. Improved Code Readability and Maintainability: Type annotations act as documentation. When you see user: User, you instantly know that the user variable is expected to conform to the User interface or type, which defines its properties. This makes code easier to understand, even for developers new to the project. Enhanced Tooling and Developer Experience: Modern IDEs (like VS Code, WebStorm) have fantastic TypeScript support. You get intelligent autocompletion, instant error highlighting, signature help, and robust refactoring capabilities based on type information. This drastically speeds up development and reduces mistakes. Better Collaboration: In team environments, explicit types serve as a clear contract between different parts of the codebase. Developers can understand how to use functions or objects created by others without having to read through extensive documentation or guess based on runtime behavior. Increased Scalability: As your application grows, managing complexity becomes challenging. TypeScript's structure helps keep your codebase organized and makes it easier to add new features or modify existing ones with confidence, knowing the compiler has your back. Access to Modern JavaScript Features: TypeScript often provides support for upcoming ECMAScript features (like decorators, class fields) before they are fully standardized or widely available in all JavaScript environments, allowing you to use them today. Getting Started with TypeScript: The Basics Starting with TypeScript is straightforward. You typically install it via npm: npm install -g typescript Once installed, you can write TypeScript code in files with a .ts extension. For example, app.ts. To convert your TypeScript code into plain JavaScript that browsers or Node.js can understand, you use the TypeScript compiler: tsc app.ts This command will generate an app.js file. For larger projects, you'll use a tsconfig.json file to configure the compiler options, specifying things like the target JavaScript version, where your source files are, and where the compiled output should go. Key TypeScript Concepts Understanding a few core concepts is crucial: • Basic Types: TypeScript supports primitive types like string, number, boolean, null, undefined, and symbol. It also has any (use sparingly!), unknown (a safer alternative to any), void (for functions that don't return a value), and never (for functions that never return, like those that throw errors). • Arrays and Tuples: You can type arrays (e.g., number[] or Array) and tuples (arrays with a fixed number of elements of specific types, e.g., [string, number]). • Type Annotations vs. Type Inference: While you can explicitly annotate everything (let name: string = "Alice";), TypeScript is often
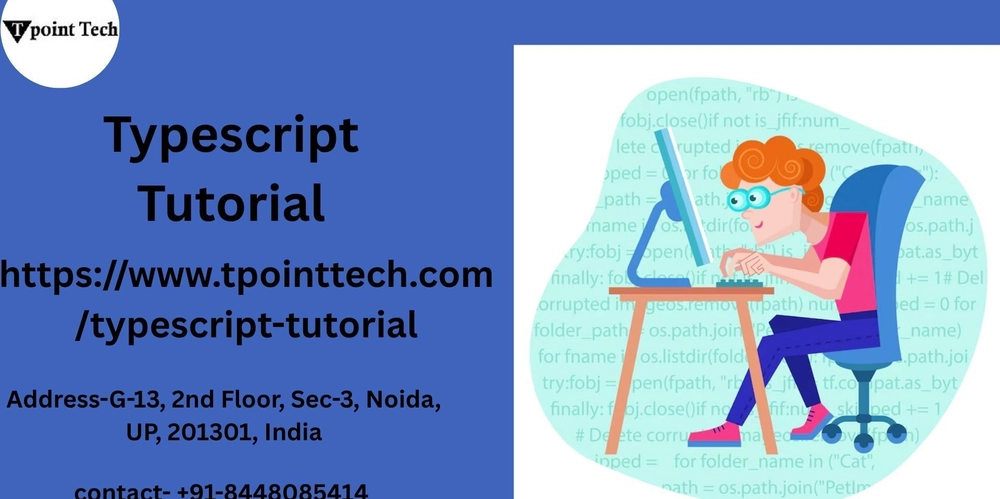
Are you a JavaScript developer looking to build more robust, scalable, and maintainable applications? Do you find yourself debugging frustrating runtime errors that could have been caught earlier? If so, it's time to explore TypeScript, a powerful superset of JavaScript that adds optional static typing. This typescript tutorial will give you a comprehensive overview of what TypeScript is, why it's become so popular, and the core concepts you need to understand to get started.
For years, JavaScript's dynamic nature has been both a blessing and a curse. Its flexibility allows for rapid prototyping and development, but as projects grow in size and complexity, this dynamism can lead to unexpected bugs and make code harder to understand and refactor. This is where TypeScript shines. It provides a layer of type safety on top of JavaScript, allowing you to define the shape of your data and catch type-related errors during the development phase, before your code even runs in the browser or on the server.
What Exactly is TypeScript?
At its heart, TypeScript is simply JavaScript with extra features. It's a superset, meaning all valid JavaScript code is also valid TypeScript code. The key addition is the static type system. You can annotate your variables, function parameters, and return values with types (like string, number, boolean, array, object, etc.). The TypeScript compiler (tsc) then checks for type compatibility. If there's a mismatch, it throws a compilation error, alerting you to potential issues immediately.
Think of it like adding a blueprint to your construction project. JavaScript lets you start building immediately, figuring things out as you go. TypeScript encourages you to define your structure upfront, making the build process smoother and the final structure more stable and predictable.
Why Should You Learn TypeScript?
The benefits of adopting TypeScript are numerous and significant, especially for larger projects or teams:
- Catch Errors Early: This is arguably the biggest win. Instead of encountering TypeErrors or undefined is not a function errors at runtime, the TypeScript compiler will flag these issues before you even test your code. This saves immense debugging time and effort.
- Improved Code Readability and Maintainability: Type annotations act as documentation. When you see user: User, you instantly know that the user variable is expected to conform to the User interface or type, which defines its properties. This makes code easier to understand, even for developers new to the project.
- Enhanced Tooling and Developer Experience: Modern IDEs (like VS Code, WebStorm) have fantastic TypeScript support. You get intelligent autocompletion, instant error highlighting, signature help, and robust refactoring capabilities based on type information. This drastically speeds up development and reduces mistakes.
- Better Collaboration: In team environments, explicit types serve as a clear contract between different parts of the codebase. Developers can understand how to use functions or objects created by others without having to read through extensive documentation or guess based on runtime behavior.
- Increased Scalability: As your application grows, managing complexity becomes challenging. TypeScript's structure helps keep your codebase organized and makes it easier to add new features or modify existing ones with confidence, knowing the compiler has your back.
- Access to Modern JavaScript Features: TypeScript often provides support for upcoming ECMAScript features (like decorators, class fields) before they are fully standardized or widely available in all JavaScript environments, allowing you to use them today. Getting Started with TypeScript: The Basics Starting with TypeScript is straightforward. You typically install it via npm: npm install -g typescript Once installed, you can write TypeScript code in files with a .ts extension. For example, app.ts. To convert your TypeScript code into plain JavaScript that browsers or Node.js can understand, you use the TypeScript compiler: tsc app.ts This command will generate an app.js file. For larger projects, you'll use a tsconfig.json file to configure the compiler options, specifying things like the target JavaScript version, where your source files are, and where the compiled output should go. Key TypeScript Concepts Understanding a few core concepts is crucial: • Basic Types: TypeScript supports primitive types like string, number, boolean, null, undefined, and symbol. It also has any (use sparingly!), unknown (a safer alternative to any), void (for functions that don't return a value), and never (for functions that never return, like those that throw errors). • Arrays and Tuples: You can type arrays (e.g., number[] or Array) and tuples (arrays with a fixed number of elements of specific types, e.g., [string, number]). • Type Annotations vs. Type Inference: While you can explicitly annotate everything (let name: string = "Alice";), TypeScript is often smart enough to figure out the type based on the assigned value (let age = 30; - TS infers age is a number). Type inference allows you to write less verbose code while still getting type safety. • Interfaces and Types: These are fundamental for defining the structure (or "shape") of objects. An interface or a type alias can describe what properties an object must have and what their types are. This is incredibly useful for working with data structures. • Classes: TypeScript adds classical object-oriented programming features, including classes, constructors, access modifiers (public, private, protected), and inheritance, providing a familiar structure for many developers. • Functions: You can specify the types of function parameters and the type of the value the function returns (function greet(name: string): string { return "Hello, " + name; }). This helps ensure you call functions correctly and handle their return values appropriately. • Union and Intersection Types: Union types (string | number) allow a variable to hold a value that is one of several types. Intersection types (TypeA & TypeB) create a new type that combines all properties from multiple types. These provide flexibility while maintaining type safety. • Generics: Generics allow you to write code that can work with different types without losing type information. This is powerful for creating reusable components like functions that operate on arrays of any type or data structures that hold elements of a specific type. TypeScript in the Real World TypeScript is not just for standalone projects. It integrates seamlessly with popular JavaScript frameworks and libraries. It's the default language for Angular, and widely used in the React and Vue ecosystems, often with type definitions available for most major libraries. This means you can bring the benefits of type safety to your frontend and backend (Node.js) development. Moving Forward This overview provides a foundation, but the world of TypeScript is vast. To truly harness its power, you'll need to practice, experiment, and dive deeper into specific features relevant to your projects. By following a comprehensive TypeScript tutorial](urlhttps://www.tpointtech.com/typescript-tutorial), you can quickly start leveraging its power. Embracing TypeScript is an investment that pays significant dividends in code quality, developer confidence, and long-term project maintainability. If you're serious about writing better JavaScript, taking the time to learn TypeScript is one of the best decisions you can make. Get started today and experience the difference type safety brings!