(Developer Tutorial) How to View PDF Files in C#?
Viewing PDFs in a C# application is a common requirement, especially for document management, report generation, and business applications. While many libraries provide PDF handling, IronPDF stands out due to its ease of use, rendering accuracy, and ability to integrate seamlessly into .NET applications. In this article, we will explore how to create a C# PDF Viewer using IronPDF, covering installation, key features, and multiple code examples. Why Use IronPDF for PDF Viewing? IronPDF, a powerful .NET PDF library, provides robust PDF handling features, including: Accurate PDF Rendering: High-quality display of PDF content. Extracting Text & Images: Allows interaction with PDF data. Conversion to Images: Easily convert PDFs into image formats for viewing. Integration with WinForms & WPF: Embed PDF content in GUI applications. Search & Annotation Support: Enables text searching and adding annotations. Viewing and interacting with PDFs is a common requirement in modern applications, from document management systems to reporting tools. Whether you are building a desktop application (WinForms, WPF), a web-based solution (ASP.NET Core, Blazor), or even a console-based utility, having the ability to render, extract, and search PDFs enhances your application's usability. In this article, we will explore how to create a PDF Viewer in C# using the IronPDF library. We will cover: Rendering PDFs into images for easy viewing. Extracting text for search and accessibility. Displaying PDFs in a WinForms application using a WebBrowser. Searching for specific text inside a PDF. The same logic and techniques demonstrated here can be applied to other application types, such as ASP.NET web applications or WPF applications, with minor modifications. Setting Up the Project Before diving into the implementation, let's set up our environment. The examples below will primarily focus on WinForms and Console applications, but the same principles apply to other .NET applications. Step 1: Install IronPDF Install IronPDF via NuGet Package Manager: Install-Package IronPDF Once installed, you can use IronPDF in WinForms, WPF, ASP.NET, and Console applications. 1. Rendering a PDF as an Image for Displaying in Applications A common approach to viewing PDFs in C# applications is to convert each page into an image. This allows displaying the PDF in WinForms (PictureBox), WPF (Image Control), or even ASP.NET Web applications. Code Example (WinForms Application with PictureBox) The following example loads a PDF file, converts its first page into an image, and displays it in a WinForms application using a PictureBox. using IronPdf; using System; using System.Drawing; using System.Windows.Forms; // Write this code in designer cs file var pdf = new PdfDocument("SamplePDF.pdf"); String[] image = pdf.RasterizeToImageFiles(@"Images\*.png"); pdfPicBox.Image = Image.FromFile(image[0]); Code Explanation: Convert PDF to Images: Use pdf.RasterizeToImageFiles() to generate image files from a PDF, making it easier to display in applications. View in Windows Forms: Load the first image into a PictureBox using Image.FromFile(image[0]). Useful Scenarios: Helpful for previewing PDFs in UI applications, generating thumbnails, or displaying PDFs in environments where a PDF viewer is unavailable. Enhanced Compatibility: Works well in web, desktop, and mobile apps where direct PDF rendering isn't supported. Output 2. Extracting Text from a PDF for Search & Accessibility If you need to search for text, index documents, or convert PDFs into plain text, IronPDF provides built-in text extraction. Code Example (Console Application for Text Extraction) The following example reads a PDF file and extracts all text from it. This is useful for search engines, accessibility tools, or document parsing applications. using IronPDF; // Load the PDF document PdfDocument pdfDocument = new PdfDocument("TableSample.pdf"); // Extract all text from the PDF string extractedText = pdfDocument.ExtractAllText(); Console.WriteLine(extractedText); Code Explanation Loads a PDF file using PdfDocument(pdfPath). Extracts all text from the PDF using pdf.Text. Prints the extracted text to the console. Output: 3. Embedding a PDF Viewer in a WinForms Application A more advanced approach to PDF viewing is embedding the entire PDF document inside a WinForms application using the WebBrowser control. Code Example (WinForms PDF Viewer using WebBrowser) This example converts a PDF file to HTML and then displays it using the WebBrowser control in a WinForms application. var pdf = new PdfDocument("SamplePDF.pdf"); pdf.SaveAsHtml("pdfTohtml.html"); string filename= Path.GetFullPath("pdfTohtml.html"); pdfWebBrowser.Url = new Uri(filename);
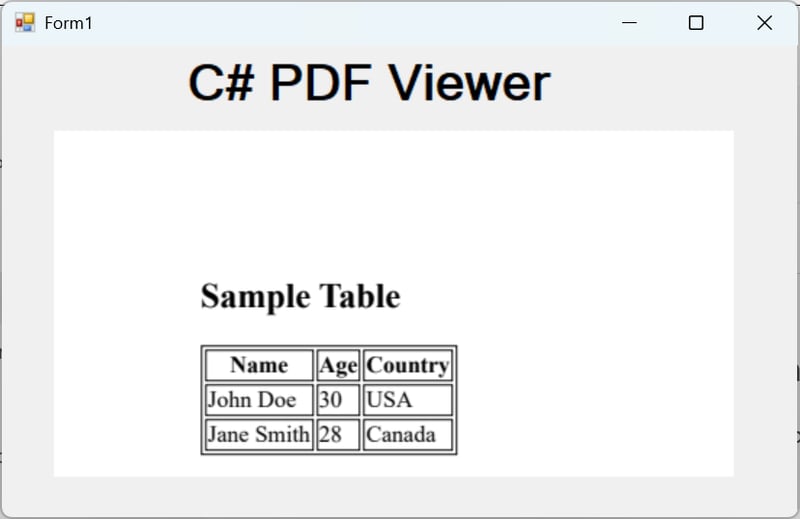
Viewing PDFs in a C# application is a common requirement, especially for document management, report generation, and business applications. While many libraries provide PDF handling, IronPDF stands out due to its ease of use, rendering accuracy, and ability to integrate seamlessly into .NET applications. In this article, we will explore how to create a C# PDF Viewer using IronPDF, covering installation, key features, and multiple code examples.
Why Use IronPDF for PDF Viewing?
IronPDF, a powerful .NET PDF library, provides robust PDF handling features, including:
- Accurate PDF Rendering: High-quality display of PDF content.
- Extracting Text & Images: Allows interaction with PDF data.
- Conversion to Images: Easily convert PDFs into image formats for viewing.
- Integration with WinForms & WPF: Embed PDF content in GUI applications.
- Search & Annotation Support: Enables text searching and adding annotations.
Viewing and interacting with PDFs is a common requirement in modern applications, from document management systems to reporting tools. Whether you are building a desktop application (WinForms, WPF), a web-based solution (ASP.NET Core, Blazor), or even a console-based utility, having the ability to render, extract, and search PDFs enhances your application's usability.
In this article, we will explore how to create a PDF Viewer in C# using the IronPDF library. We will cover:
- Rendering PDFs into images for easy viewing.
- Extracting text for search and accessibility.
- Displaying PDFs in a WinForms application using a WebBrowser.
- Searching for specific text inside a PDF.
The same logic and techniques demonstrated here can be applied to other application types, such as ASP.NET web applications or WPF applications, with minor modifications.
Setting Up the Project
Before diving into the implementation, let's set up our environment. The examples below will primarily focus on WinForms and Console applications, but the same principles apply to other .NET applications.
Step 1: Install IronPDF
Install IronPDF via NuGet Package Manager:
Install-Package IronPDF
Once installed, you can use IronPDF in WinForms, WPF, ASP.NET, and Console applications.
1. Rendering a PDF as an Image for Displaying in Applications
A common approach to viewing PDFs in C# applications is to convert each page into an image. This allows displaying the PDF in WinForms (PictureBox), WPF (Image Control), or even ASP.NET Web applications.
Code Example (WinForms Application with PictureBox)
The following example loads a PDF file, converts its first page into an image, and displays it in a WinForms application using a PictureBox.
using IronPdf;
using System;
using System.Drawing;
using System.Windows.Forms;
// Write this code in designer cs file
var pdf = new PdfDocument("SamplePDF.pdf");
String[] image = pdf.RasterizeToImageFiles(@"Images\*.png");
pdfPicBox.Image = Image.FromFile(image[0]);
Code Explanation:
-
Convert PDF to Images: Use
pdf.RasterizeToImageFiles()
to generate image files from a PDF, making it easier to display in applications. -
View in Windows Forms: Load the first image into a PictureBox using
Image.FromFile(image[0])
.
Useful Scenarios: Helpful for previewing PDFs in UI applications, generating thumbnails, or displaying PDFs in environments where a PDF viewer is unavailable.
Enhanced Compatibility: Works well in web, desktop, and mobile apps where direct PDF rendering isn't supported.
Output
2. Extracting Text from a PDF for Search & Accessibility
If you need to search for text, index documents, or convert PDFs into plain text, IronPDF provides built-in text extraction.
Code Example (Console Application for Text Extraction)
The following example reads a PDF file and extracts all text from it. This is useful for search engines, accessibility tools, or document parsing applications.
using IronPDF;
// Load the PDF document
PdfDocument pdfDocument = new PdfDocument("TableSample.pdf");
// Extract all text from the PDF
string extractedText = pdfDocument.ExtractAllText();
Console.WriteLine(extractedText);
Code Explanation
- Loads a PDF file using
PdfDocument(pdfPath)
. - Extracts all text from the PDF using pdf.Text.
- Prints the extracted text to the console.
3. Embedding a PDF Viewer in a WinForms Application
A more advanced approach to PDF viewing is embedding the entire PDF document inside a WinForms application using the WebBrowser control.
Code Example (WinForms PDF Viewer using WebBrowser)
This example converts a PDF file to HTML and then displays it using the WebBrowser control in a WinForms application.
var pdf = new PdfDocument("SamplePDF.pdf");
pdf.SaveAsHtml("pdfTohtml.html");
string filename= Path.GetFullPath("pdfTohtml.html");
pdfWebBrowser.Url = new Uri(filename);
Code Explanation
-
Loads the PDF File:
PdfDocument("SamplePDF.pdf")
opens the "SamplePDF.pdf" file for processing. -
Converts PDF to HTML:
pdf.SaveAsHtml("pdfTohtml.html")
saves the PDF as an HTML file, making it viewable in a web browser. -
Gets Absolute Path:
Path.GetFullPath("pdfTohtml.html")
ensures the file path is correctly resolved for browser navigation. -
Displays HTML in PDF Viewer:
pdfWebBrowser.Url = new Uri(filePath);
loads the converted HTML file into a web browser control for viewing.
IronPDF's PDF-to-HTML conversion feature allows seamless PDF viewing in web-based applications without requiring third-party PDF viewers. By converting PDFs to HTML, developers can embed PDFs into web forms, ASP.NET applications, and modern web pages, ensuring cross-platform compatibility. This approach enhances accessibility, responsiveness, and user experience, making PDFs viewable in any browser without additional plugins. It is especially useful for document previews, reporting systems, and secure web-based PDF viewing.
4. Searching for Text Inside a PDF
Adding a search functionality inside a PDF viewer allows users to quickly find relevant information.
Code Example (Searching for Text in a PDF)
The following example loads a PDF file, searches for a specific word or phrase, and prints whether it was found or not.
var pdf = new PdfDocument("SamplePDF.pdf");
string searchText = "John";
bool found = pdf.ExtractAllText().Contains(searchText);
Console.WriteLine(found ? "Text found in PDF!" : "Text not found.");
Code Explanation:
- Loads the PDF File:
PdfDocument("SamplePDF.pdf")
opens the "SamplePDF.pdf" for text extraction. - Defines Search Text: Stores "John" in searchText to check its presence in the PDF.
- Searches for Text: Extracts all text using
pdf.ExtractAllText()
and checks if "John" exists. - Displays Result: Prints
"Text found in PDF!"
if found; otherwise,"Text not found."
Conclusion
IronPDF provides a powerful and flexible way to view PDF files in C# applications without relying on a dedicated PDF viewer control. Whether you're rendering PDF pages as images, extracting text from PDF documents, or integrating PDFs into a Windows Forms application, IronPDF simplifies the process with its robust features. The same logic can be applied to multiple PDF files in web, desktop, or console applications, making it a versatile choice for .NET developers. You can try IronPDF with a free trial to explore its full capabilities before making a commitment.