How to Overcome Tailwind CSS Limitations with UnoCSS
As front-end development grows more complex, developers face increasing challenges with CSS frameworks. While Tailwind CSS has been a go-to solution for many teams, it comes with certain limitations that can impact development efficiency and performance. This guide explores how UnoCSS addresses these challenges and provides practical solutions with real-world examples. The Challenge Many development teams encounter several common issues when working with Tailwind CSS: Slow build times in large projects Verbose class combinations for complex styling Limited flexibility in custom utility creation Heavy reliance on PostCSS and additional plugins Complex icon management requiring external libraries Let's explore how UnoCSS solves each of these challenges with practical examples and implementations. Solution 1: Optimizing Build Performance The Problem Consider a typical React project with 100+ components. With Tailwind CSS, each change triggers a full rebuild: # Typical Tailwind CSS setup npm install tailwindcss postcss autoprefixer npx tailwindcss init // tailwind.config.js - Requires scanning all files module.exports = { content: ["./src/**/*.{js,jsx,tsx,html}"], theme: { extend: {}, }, } The Solution UnoCSS eliminates this overhead with its on-demand architecture: npm install -D unocss // uno.config.ts - No file scanning required import { defineConfig } from 'unocss' export default defineConfig({ // Configuration is processed at build time theme: { colors: { brand: '#1a73e8', }, }, }) Real-world Impact: For a project with 100 components: Tailwind CSS rebuild: ~800ms UnoCSS rebuild: ~200ms Time saved per rebuild: 600ms In an 8-hour workday with 100 rebuilds: 1 minute saved Solution 2: Simplifying Complex Styling Patterns The Problem Style combinations in Tailwind CSS become unwieldy, especially with responsive and state variants: Complex Component The Solution UnoCSS's variant groups provide a cleaner, more maintainable approach: Complex Component Solution 3: Implementing Flexible Component Patterns The Problem Traditional component styling often leads to class name bloat: Click Me The Solution UnoCSS shortcuts provide a maintainable pattern: // uno.config.ts export default defineConfig({ shortcuts: { 'btn': 'px-4 py-2 rounded-lg transform transition-transform', 'btn-primary': 'btn bg-blue-500 hover:bg-blue-600 text-white font-medium hover:scale-105 active:scale-95', 'btn-secondary': 'btn bg-gray-200 hover:bg-gray-300 text-gray-800 font-medium hover:scale-105 active:scale-95' } }) Click Me Solution 4: Streamlining Icon Management The Problem Traditional icon implementation requires multiple dependencies: # Traditional approach npm install @heroicons/react import { SearchIcon } from '@heroicons/react/solid' function SearchBar() { return ( ) } The Solution UnoCSS provides built-in icon support: // uno.config.ts import { defineConfig } from 'unocss' import { presetIcons } from 'unocss' export default defineConfig({ presets: [ presetIcons({ scale: 1.2, warn: true, }), ], }) Solution 5: Dynamic Utility Generation The Problem Custom utilities in Tailwind CSS require plugin configuration: // tailwind.config.js module.exports = { plugins: [ plugin(function({ addUtilities }) { const newUtilities = { '.custom-rotate-15': { transform: 'rotate(15deg)', }, } addUtilities(newUtilities) }) ] } The Solution UnoCSS offers dynamic rules for flexible utility generation: // uno.config.ts export default defineConfig({ rules: [ [/^rotate-(\d+)$/, ([, d]) => ({ transform: `rotate(${d}deg)` })], [/^custom-(.+)$/, ([, name]) => { if (preset.includes(name)) { return preset[name] } }], ], }) Best Practices and Key Takeaways Performance Optimization * Use UnoCSS's on-demand generation for large projects * Leverage the Vite plugin for optimal build times Code Organization * Implement variant groups for complex style combinations * Use shortcuts for commonly repeated patterns * Organize related utilities using attributify mode Development Workflow * Take advantage of the inspector tool for debugging Use the CDN runtime for quick prototyping Implement dynamic rules for custom utility patterns Conclusion UnoCSS effectively addresses many limitations developers face with Tailwind CSS while maintaining familiar utility-first principles. By implementing these solutions, teams can achieve: Faster build times Cleaner, more maintainable code More flexible utility generat
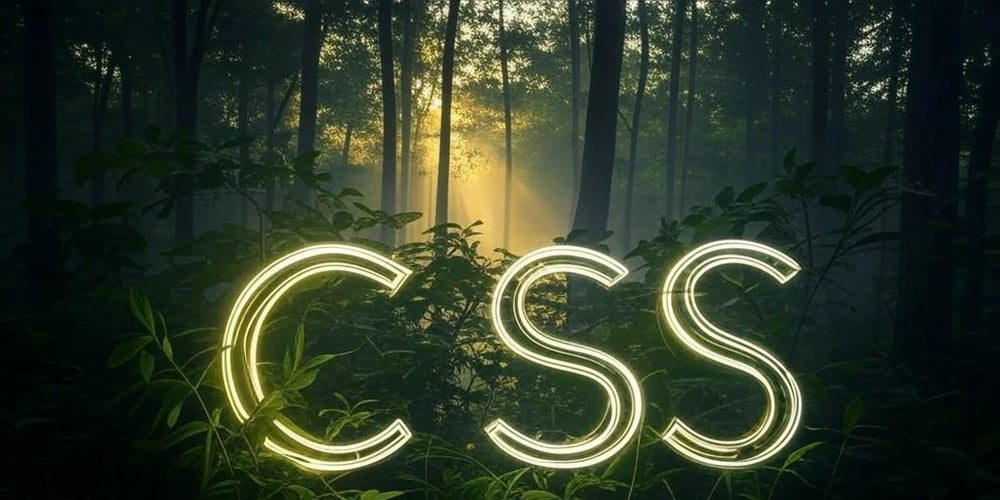
As front-end development grows more complex, developers face increasing challenges with CSS frameworks. While Tailwind CSS has been a go-to solution for many teams, it comes with certain limitations that can impact development efficiency and performance. This guide explores how UnoCSS addresses these challenges and provides practical solutions with real-world examples.
The Challenge
Many development teams encounter several common issues when working with Tailwind CSS:
Slow build times in large projects
Verbose class combinations for complex styling
Limited flexibility in custom utility creation
Heavy reliance on PostCSS and additional plugins
Complex icon management requiring external libraries
Let's explore how UnoCSS solves each of these challenges with practical examples and implementations.
Solution 1: Optimizing Build Performance
The Problem
Consider a typical React project with 100+ components. With Tailwind CSS, each change triggers a full rebuild:
# Typical Tailwind CSS setup
npm install tailwindcss postcss autoprefixer
npx tailwindcss init
// tailwind.config.js - Requires scanning all files
module.exports = {
content: ["./src/**/*.{js,jsx,tsx,html}"],
theme: {
extend: {},
},
}
The Solution
UnoCSS eliminates this overhead with its on-demand architecture:
npm install -D unocss
// uno.config.ts - No file scanning required
import { defineConfig } from 'unocss'
export default defineConfig({
// Configuration is processed at build time
theme: {
colors: {
brand: '#1a73e8',
},
},
})
Real-world Impact: For a project with 100 components:
Tailwind CSS rebuild: ~800ms
UnoCSS rebuild: ~200ms
Time saved per rebuild: 600ms
In an 8-hour workday with 100 rebuilds: 1 minute saved
Solution 2: Simplifying Complex Styling Patterns
The Problem
Style combinations in Tailwind CSS become unwieldy, especially with responsive and state variants:
class="
bg-white dark:bg-gray-800
hover:bg-gray-50 dark:hover:bg-gray-700
focus:ring-2 focus:ring-blue-500 dark:focus:ring-blue-400
active:bg-gray-100 dark:active:bg-gray-600
sm:px-6 md:px-8 lg:px-10
py-4
">
Complex Component
The Solution
UnoCSS's variant groups provide a cleaner, more maintainable approach:
class="
bg-white py-4
dark:(bg-gray-800)
hover:(bg-gray-50 dark:bg-gray-700)
focus:(ring-2 ring-blue-500 dark:ring-blue-400)
active:(bg-gray-100 dark:bg-gray-600)
sm:px-6 md:px-8 lg:px-10
">
Complex Component
Solution 3: Implementing Flexible Component Patterns
The Problem
Traditional component styling often leads to class name bloat:
The Solution
UnoCSS shortcuts provide a maintainable pattern:
// uno.config.ts
export default defineConfig({
shortcuts: {
'btn': 'px-4 py-2 rounded-lg transform transition-transform',
'btn-primary': 'btn bg-blue-500 hover:bg-blue-600 text-white font-medium hover:scale-105 active:scale-95',
'btn-secondary': 'btn bg-gray-200 hover:bg-gray-300 text-gray-800 font-medium hover:scale-105 active:scale-95'
}
})
Solution 4: Streamlining Icon Management
The Problem
Traditional icon implementation requires multiple dependencies:
# Traditional approach
npm install @heroicons/react
import { SearchIcon } from '@heroicons/react/solid'
function SearchBar() {
return (
<div className="relative">
<SearchIcon className="w-5 h-5 text-gray-400" />
<input type="text" className="pl-10" />
div>
)
}
The Solution
UnoCSS provides built-in icon support:
// uno.config.ts
import { defineConfig } from 'unocss'
import { presetIcons } from 'unocss'
export default defineConfig({
presets: [
presetIcons({
scale: 1.2,
warn: true,
}),
],
})
class="relative">
class="i-heroicons-search absolute left-3 top-1/2 -translate-y-1/2 text-gray-400"/>
type="text" class="pl-10"/>
Solution 5: Dynamic Utility Generation
The Problem
Custom utilities in Tailwind CSS require plugin configuration:
// tailwind.config.js
module.exports = {
plugins: [
plugin(function({ addUtilities }) {
const newUtilities = {
'.custom-rotate-15': {
transform: 'rotate(15deg)',
},
}
addUtilities(newUtilities)
})
]
}
The Solution
UnoCSS offers dynamic rules for flexible utility generation:
// uno.config.ts
export default defineConfig({
rules: [
[/^rotate-(\d+)$/, ([, d]) => ({ transform: `rotate(${d}deg)` })],
[/^custom-(.+)$/, ([, name]) => {
if (preset.includes(name)) {
return preset[name]
}
}],
],
})
Best Practices and Key Takeaways
- Performance Optimization
* Use UnoCSS's on-demand generation for large projects
* Leverage the Vite plugin for optimal build times
- Code Organization
* Implement variant groups for complex style combinations
* Use shortcuts for commonly repeated patterns
* Organize related utilities using attributify mode
- Development Workflow
* Take advantage of the inspector tool for debugging
Use the CDN runtime for quick prototyping
Implement dynamic rules for custom utility patterns
Conclusion
UnoCSS effectively addresses many limitations developers face with Tailwind CSS while maintaining familiar utility-first principles. By implementing these solutions, teams can achieve:
Faster build times
Cleaner, more maintainable code
More flexible utility generation
Simplified icon management
Better development experience
Remember that migrating to UnoCSS doesn't require a complete rewrite of your existing Tailwind CSS code. You can gradually adopt these solutions as your project grows and evolves.
For teams considering the switch, start with the performance optimizations and gradually implement other features based on your specific needs. The key is to identify which limitations impact your team the most and address them first using UnoCSS's powerful features.