Secure Client-Side Encryption and Decryption with JavaScript: A Practical Guide
In today's digital landscape, securing sensitive data is paramount. Client-side encryption, performed directly within the user's browser, offers a powerful method to protect information before it's transmitted or stored. This article explores how to implement robust encryption and decryption using JavaScript, and highlights the utility of tools like Randzy's Encrypt Online and Decrypt online tool. Understanding Client-Side Encryption Client-side encryption involves transforming plaintext data into ciphertext directly within the user's browser, using JavaScript. This ensures that sensitive information remains protected even if the communication channel or storage is compromised. Key advantages include: Enhanced Privacy: Data is encrypted before leaving the user's device, minimizing exposure to potential eavesdroppers. Reduced Server Load: Encryption and decryption operations are offloaded to the client, reducing server-side processing demands. Improved Security: Even if a server is breached, encrypted data remains protected without the decryption key. Implementing Encryption and Decryption with JavaScript JavaScript's Web Crypto API provides a standardized interface for performing cryptographic operations. Common encryption algorithms include AES (Advanced Encryption Standard). Here's a simplified example demonstrating AES-CBC encryption and decryption: async function encrypt(plaintext, key, iv) { const encodedPlaintext = new TextEncoder().encode(plaintext); const cryptoKey = await crypto.subtle.importKey( "raw", key, { name: "AES-CBC" }, false, ["encrypt"] ); const ciphertext = await crypto.subtle.encrypt( { name: "AES-CBC", iv: iv }, cryptoKey, encodedPlaintext ); return ciphertext; } async function decrypt(ciphertext, key, iv) { const cryptoKey = await crypto.subtle.importKey( "raw", key, { name: "AES-CBC" }, false, ["decrypt"] ); const plaintext = await crypto.subtle.decrypt( { name: "AES-CBC", iv: iv }, cryptoKey, ciphertext ); return new TextDecoder().decode(plaintext); } // Example usage (key and iv must be securely generated and managed) // ... (key and iv generation and storage code) ... // Example of key and iv generation. async function generateKeyAndIv(){ const key = await crypto.getRandomValues(new Uint8Array(32)); const iv = await crypto.getRandomValues(new Uint8Array(16)); return {key:key, iv:iv}; } // Example of converting the key and iv to array buffers. function toArrayBuffer(arr){ return arr.buffer; } // example of usage. async function runExample(){ const {key, iv} = await generateKeyAndIv(); const plaintext = "This is a secret message."; const ciphertext = await encrypt(plaintext, toArrayBuffer(key), toArrayBuffer(iv)); const decryptedText = await decrypt(ciphertext, toArrayBuffer(key), toArrayBuffer(iv)); console.log("Plaintext:", plaintext); console.log("Ciphertext:", ciphertext); console.log("Decrypted Text:", decryptedText); } runExample(); Randzy's Encryption and Decryption: A User-Friendly Solution For those seeking a straightforward and accessible encryption/decryption tool, Randzy's Encryption and Decryption offers a convenient online solution. This tool simplifies the encryption and decryption process, enabling users to secure their data without requiring extensive technical expertise. Randzy's Encrypt/Decrypt performs all cryptographic operations client-side, ensuring that sensitive information remains within the user's browser. Key Features of Randzy's Encrypt/Decrypt: Ease of Use: An intuitive interface simplifies encryption and decryption. Client-Side Processing: Ensures data privacy by performing all operations locally. Multiple Algorithm Support: Providing users with options for their security needs. Secure Key Management: Randzy's encrypt/decrypt uses user input to generate keys, and does not store them. Best Practices for Client-Side Encryption Secure Key Generation and Management: Keys must be generated using cryptographically secure methods and stored securely. Use Strong Algorithms: Opt for robust encryption algorithms like AES-256. Implement Proper Initialization Vectors (IVs): IVs should be unique and unpredictable. Consider Data Integrity: Use authenticated encryption modes like AES-GCM to prevent tampering. Client-side encryption empowers users to protect sensitive data directly within their browsers. Tools like String Encryption Online provide accessible solutions for secure data handling. By adhering to best practices and leveraging these technologies, you can enhance the security and privacy of your online interactions.
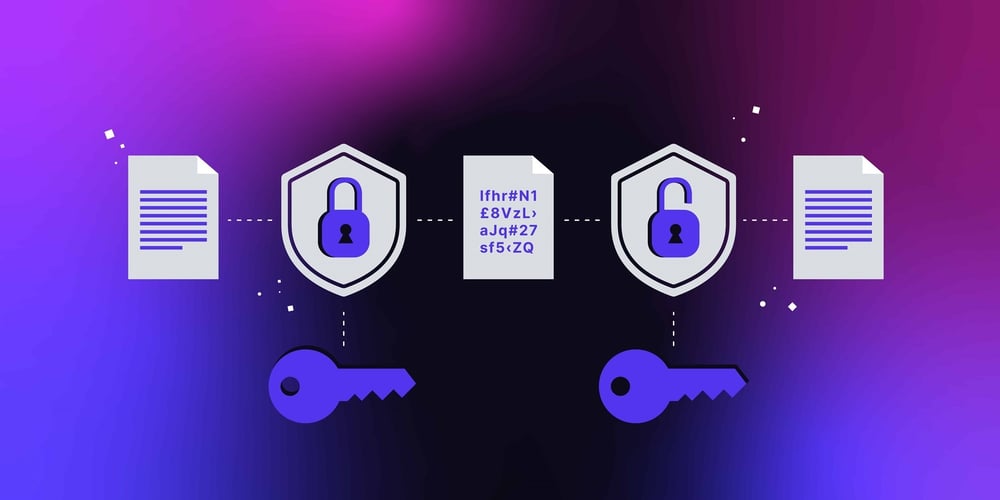
In today's digital landscape, securing sensitive data is paramount. Client-side encryption, performed directly within the user's browser, offers a powerful method to protect information before it's transmitted or stored. This article explores how to implement robust encryption and decryption using JavaScript, and highlights the utility of tools like Randzy's Encrypt Online and Decrypt online tool.
Understanding Client-Side Encryption
Client-side encryption involves transforming plaintext data into ciphertext directly within the user's browser, using JavaScript. This ensures that sensitive information remains protected even if the communication channel or storage is compromised. Key advantages include:
Enhanced Privacy: Data is encrypted before leaving the user's device, minimizing exposure to potential eavesdroppers.
Reduced Server Load: Encryption and decryption operations are offloaded to the client, reducing server-side processing demands.
Improved Security: Even if a server is breached, encrypted data remains protected without the decryption key.
Implementing Encryption and Decryption with JavaScript
JavaScript's Web Crypto API provides a standardized interface for performing cryptographic operations. Common encryption algorithms include AES (Advanced Encryption Standard).
Here's a simplified example demonstrating AES-CBC encryption and decryption:
async function encrypt(plaintext, key, iv) {
const encodedPlaintext = new TextEncoder().encode(plaintext);
const cryptoKey = await crypto.subtle.importKey(
"raw",
key,
{ name: "AES-CBC" },
false,
["encrypt"]
);
const ciphertext = await crypto.subtle.encrypt(
{ name: "AES-CBC", iv: iv },
cryptoKey,
encodedPlaintext
);
return ciphertext;
}
async function decrypt(ciphertext, key, iv) {
const cryptoKey = await crypto.subtle.importKey(
"raw",
key,
{ name: "AES-CBC" },
false,
["decrypt"]
);
const plaintext = await crypto.subtle.decrypt(
{ name: "AES-CBC", iv: iv },
cryptoKey,
ciphertext
);
return new TextDecoder().decode(plaintext);
}
// Example usage (key and iv must be securely generated and managed)
// ... (key and iv generation and storage code) ...
// Example of key and iv generation.
async function generateKeyAndIv(){
const key = await crypto.getRandomValues(new Uint8Array(32));
const iv = await crypto.getRandomValues(new Uint8Array(16));
return {key:key, iv:iv};
}
// Example of converting the key and iv to array buffers.
function toArrayBuffer(arr){
return arr.buffer;
}
// example of usage.
async function runExample(){
const {key, iv} = await generateKeyAndIv();
const plaintext = "This is a secret message.";
const ciphertext = await encrypt(plaintext, toArrayBuffer(key), toArrayBuffer(iv));
const decryptedText = await decrypt(ciphertext, toArrayBuffer(key), toArrayBuffer(iv));
console.log("Plaintext:", plaintext);
console.log("Ciphertext:", ciphertext);
console.log("Decrypted Text:", decryptedText);
}
runExample();
Randzy's Encryption and Decryption: A User-Friendly Solution
For those seeking a straightforward and accessible encryption/decryption tool, Randzy's Encryption and Decryption offers a convenient online solution.
This tool simplifies the encryption and decryption process, enabling users to secure their data without requiring extensive technical expertise. Randzy's Encrypt/Decrypt performs all cryptographic operations client-side, ensuring that sensitive information remains within the user's browser.
Key Features of Randzy's Encrypt/Decrypt:
Ease of Use: An intuitive interface simplifies encryption and decryption.
Client-Side Processing: Ensures data privacy by performing all operations locally.
Multiple Algorithm Support: Providing users with options for their security needs.
Secure Key Management: Randzy's encrypt/decrypt uses user input to generate keys, and does not store them.
Best Practices for Client-Side Encryption
Secure Key Generation and Management: Keys must be generated using cryptographically secure methods and stored securely.
Use Strong Algorithms: Opt for robust encryption algorithms like AES-256.
Implement Proper Initialization Vectors (IVs): IVs should be unique and unpredictable.
Consider Data Integrity: Use authenticated encryption modes like AES-GCM to prevent tampering.
Client-side encryption empowers users to protect sensitive data directly within their browsers. Tools like String Encryption Online provide accessible solutions for secure data handling. By adhering to best practices and leveraging these technologies, you can enhance the security and privacy of your online interactions.