Synchronous vs. Asynchronous Communication in Programming
Programming often involves handling tasks that take time—such as reading files, making network requests, or processing large datasets. How these tasks are managed can significantly impact performance and user experience. This article explores synchronous and asynchronous communication in programming, with a deep dive into a practical use case: fetching data from an API. 1. Synchronous Communication Definition Synchronous (sync) operations execute sequentially, meaning the program waits for each task to complete before moving to the next. This is a blocking approach—execution halts until the current operation finishes. How It Works The program calls a function and waits for its return value. No other operations run until the current one completes. Simple to reason about but can lead to performance bottlenecks. Example: Synchronous API Call (Pseudocode) function fetchUserData() { const response = makeNetworkRequestSync("https://api.example.com/users"); // Blocks here console.log("Data received:", response); console.log("This runs only after the request finishes."); } fetchUserData(); Problem: If the network request takes 5 seconds, the entire program freezes for that duration. 2. Asynchronous Communication Definition Asynchronous (async) operations allow tasks to run independently without blocking the main program flow. Instead of waiting, the program continues execution and gets notified when the task completes. How It Works Uses callbacks, promises, or async/await to handle delayed results. Non-blocking: Other code runs while waiting for the async task. More efficient but requires careful error handling. Example: Asynchronous API Call (JavaScript) Using Callbacks (Older Approach) function fetchUserData(callback) { makeNetworkRequestAsync("https://api.example.com/users", (response) => { callback(response); }); console.log("Request sent, but the program continues..."); } fetchUserData((data) => { console.log("Data received:", data); }); Behavior: The console.log runs immediately, and the callback executes later when the data arrives. Using Promises (Modern Approach) function fetchUserData() { return fetch("https://api.example.com/users") // Returns a Promise .then(response => response.json()); } fetchUserData() .then(data => console.log("Data received:", data)) .catch(error => console.error("Error:", error)); console.log("Request sent, but the program continues..."); Behavior: The fetch runs in the background, and .then() processes the result later. Using Async/Await (Cleaner Syntax) async function fetchUserData() { try { const response = await fetch("https://api.example.com/users"); const data = await response.json(); console.log("Data received:", data); } catch (error) { console.error("Error:", error); } } fetchUserData(); console.log("Request sent, but the program continues..."); Behavior: await pauses the function (not the whole program) until the request completes. 3. Deep Dive: Fetching Data from an API (Real-World Use Case) Scenario A web app needs to fetch user data from a REST API and display it. Synchronous Approach (Problematic) // ❌ Synchronous fetch (hypothetical, as JS doesn't support sync HTTP requests by default) const data = synchronousFetch("https://api.example.com/users"); console.log(data); renderUserData(data); // UI freezes until data loads Issues: The entire UI becomes unresponsive. If the API is slow, the app appears "frozen." Asynchronous Approach (Optimal) // ✅ Asynchronous fetch (using async/await) async function loadUserData() { try { const response = await fetch("https://api.example.com/users"); const data = await response.json(); renderUserData(data); // Updates UI when ready } catch (error) { showError(error); } } loadUserData(); showLoadingSpinner(); // UI remains interactive Advantages: The UI stays responsive while waiting. Errors can be handled gracefully. Better user experience. 4. When to Use Sync vs. Async Use Case Synchronous Asynchronous Simple scripts (no delays) ✅ Good ❌ Overkill Network requests (APIs) ❌ Bad (blocks) ✅ Best File I/O (reading/writing) ❌ Slow ✅ Efficient UI interactions (web apps) ❌ Freezes UI ✅ Required 5. Key Takeaways Synchronous = Simple but blocking (bad for performance). Asynchronous = Efficient but requires callbacks/promises. Real-world apps (web, mobile, servers) rely heavily on async operations. Modern JavaScript (async/await, Promises) makes async code cleaner. By understanding these concepts, you can write faster, non-blocking applications that provide a smoother
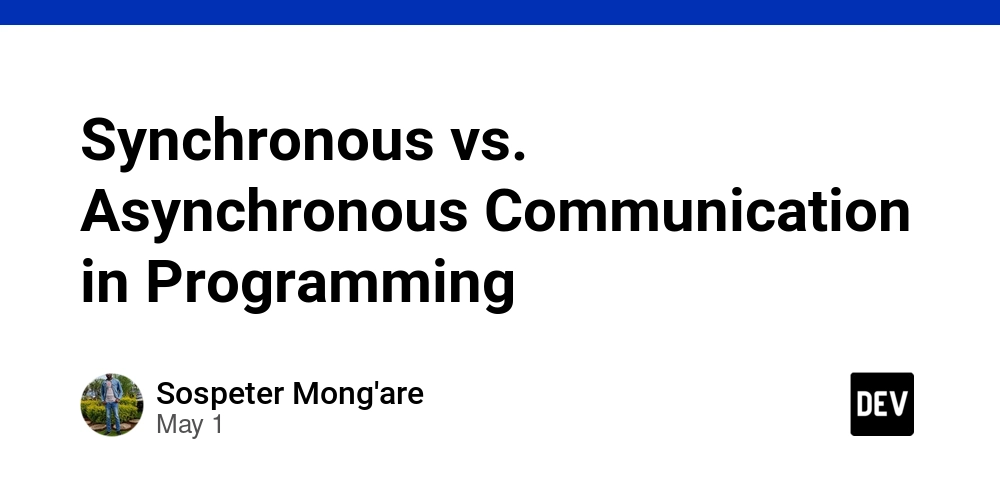
Programming often involves handling tasks that take time—such as reading files, making network requests, or processing large datasets. How these tasks are managed can significantly impact performance and user experience. This article explores synchronous and asynchronous communication in programming, with a deep dive into a practical use case: fetching data from an API.
1. Synchronous Communication
Definition
Synchronous (sync) operations execute sequentially, meaning the program waits for each task to complete before moving to the next. This is a blocking approach—execution halts until the current operation finishes.
How It Works
- The program calls a function and waits for its return value.
- No other operations run until the current one completes.
- Simple to reason about but can lead to performance bottlenecks.
Example: Synchronous API Call (Pseudocode)
function fetchUserData() {
const response = makeNetworkRequestSync("https://api.example.com/users"); // Blocks here
console.log("Data received:", response);
console.log("This runs only after the request finishes.");
}
fetchUserData();
Problem: If the network request takes 5 seconds, the entire program freezes for that duration.
2. Asynchronous Communication
Definition
Asynchronous (async) operations allow tasks to run independently without blocking the main program flow. Instead of waiting, the program continues execution and gets notified when the task completes.
How It Works
- Uses callbacks, promises, or async/await to handle delayed results.
- Non-blocking: Other code runs while waiting for the async task.
- More efficient but requires careful error handling.
Example: Asynchronous API Call (JavaScript)
Using Callbacks (Older Approach)
function fetchUserData(callback) {
makeNetworkRequestAsync("https://api.example.com/users", (response) => {
callback(response);
});
console.log("Request sent, but the program continues...");
}
fetchUserData((data) => {
console.log("Data received:", data);
});
Behavior: The console.log
runs immediately, and the callback executes later when the data arrives.
Using Promises (Modern Approach)
function fetchUserData() {
return fetch("https://api.example.com/users") // Returns a Promise
.then(response => response.json());
}
fetchUserData()
.then(data => console.log("Data received:", data))
.catch(error => console.error("Error:", error));
console.log("Request sent, but the program continues...");
Behavior: The fetch
runs in the background, and .then()
processes the result later.
Using Async/Await (Cleaner Syntax)
async function fetchUserData() {
try {
const response = await fetch("https://api.example.com/users");
const data = await response.json();
console.log("Data received:", data);
} catch (error) {
console.error("Error:", error);
}
}
fetchUserData();
console.log("Request sent, but the program continues...");
Behavior: await
pauses the function (not the whole program) until the request completes.
3. Deep Dive: Fetching Data from an API (Real-World Use Case)
Scenario
A web app needs to fetch user data from a REST API and display it.
Synchronous Approach (Problematic)
// ❌ Synchronous fetch (hypothetical, as JS doesn't support sync HTTP requests by default)
const data = synchronousFetch("https://api.example.com/users");
console.log(data);
renderUserData(data); // UI freezes until data loads
Issues:
- The entire UI becomes unresponsive.
- If the API is slow, the app appears "frozen."
Asynchronous Approach (Optimal)
// ✅ Asynchronous fetch (using async/await)
async function loadUserData() {
try {
const response = await fetch("https://api.example.com/users");
const data = await response.json();
renderUserData(data); // Updates UI when ready
} catch (error) {
showError(error);
}
}
loadUserData();
showLoadingSpinner(); // UI remains interactive
Advantages:
- The UI stays responsive while waiting.
- Errors can be handled gracefully.
- Better user experience.
4. When to Use Sync vs. Async
Use Case | Synchronous | Asynchronous |
---|---|---|
Simple scripts (no delays) | ✅ Good | ❌ Overkill |
Network requests (APIs) | ❌ Bad (blocks) | ✅ Best |
File I/O (reading/writing) | ❌ Slow | ✅ Efficient |
UI interactions (web apps) | ❌ Freezes UI | ✅ Required |
5. Key Takeaways
- Synchronous = Simple but blocking (bad for performance).
- Asynchronous = Efficient but requires callbacks/promises.
- Real-world apps (web, mobile, servers) rely heavily on async operations.
-
Modern JavaScript (
async/await
,Promises
) makes async code cleaner.
By understanding these concepts, you can write faster, non-blocking applications that provide a smoother user experience.
Would you like a deeper look into threading vs. event loops in async programming?