ES Modules and CommonJS Modules Explained
JavaScript offers two main module systems—ES Modules (ESM) and CommonJS (CJS). Understanding their differences and usage helps you write clean, modular, and maintainable code. 1. CommonJS Modules (CJS) CommonJS is widely used in Node.js environments. It loads modules synchronously and uses module.exports and require(). Defining & Using CommonJS Modules math.js: module.exports.add = (a, b) => a + b; app.js: const math = require('./math'); console.log(math.add(2, 3)); // 5 Characteristics: Synchronous loading Widely adopted in Node.js Does not support tree shaking natively 2. ES Modules (ESM) Introduced in ES6, ESM provides native support for modules in JavaScript with static analysis and tree shaking capabilities. Defining & Using ES Modules math.js: export const add = (a, b) => a + b; app.js: import { add } from './math.js'; console.log(add(2, 3)); // 5 Characteristics: Asynchronous loading Static analysis and tree shaking Supported natively by modern browsers and Node.js (with .mjs extension or type="module") 3. Differences between ESM and CJS Feature CommonJS ES Modules Loading Synchronous Asynchronous Syntax require()/module.exports import/export Tree shaking Limited Supported Dynamic Imports Not native Native via import() Node.js support Native Native (since v14 fully stable) Browser support No (requires bundling) Yes (modern browsers) 4. Dynamic Imports ES Modules support dynamic imports, which can improve performance by loading modules on-demand: button.addEventListener('click', async () => { const module = await import('./math.js'); console.log(module.add(5, 10)); }); 5. Interoperability Between ESM and CJS When using both module types together: From CommonJS to ESM: // CommonJS module requiring an ESM module (Node.js) (async () => { const math = await import('./math.mjs'); console.log(math.add(2, 3)); })(); From ESM to CommonJS: // ES module importing CommonJS import { createRequire } from 'module'; const require = createRequire(import.meta.url); const fs = require('fs'); 6. When to Choose Which? CommonJS: Legacy projects Immediate compatibility with older Node.js versions ES Modules: New projects Modern JavaScript practices Front-end projects without transpilation or bundlers 7. Best Practices Prefer ESM in modern projects for better optimization and future-proofing. Clearly distinguish modules (.mjs for ESM in Node.js or specify "type": "module" in package.json). Use dynamic imports to enhance application performance. 8. Conclusion Both CommonJS and ES Modules have distinct strengths. Understanding their differences and interoperability allows you to make informed choices that best fit your project's needs. Have you transitioned from CommonJS to ES Modules? Share your experiences and tips below!
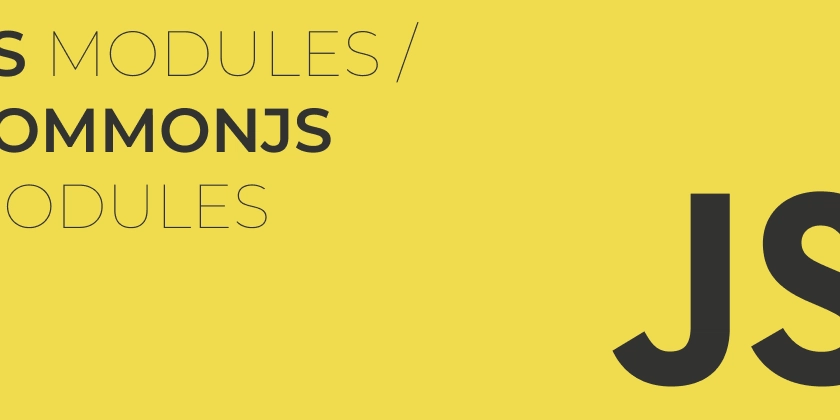
JavaScript offers two main module systems—ES Modules (ESM) and CommonJS (CJS). Understanding their differences and usage helps you write clean, modular, and maintainable code.
1. CommonJS Modules (CJS)
CommonJS is widely used in Node.js environments. It loads modules synchronously and uses module.exports
and require()
.
Defining & Using CommonJS Modules
math.js:
module.exports.add = (a, b) => a + b;
app.js:
const math = require('./math');
console.log(math.add(2, 3)); // 5
Characteristics:
- Synchronous loading
- Widely adopted in Node.js
- Does not support tree shaking natively
2. ES Modules (ESM)
Introduced in ES6, ESM provides native support for modules in JavaScript with static analysis and tree shaking capabilities.
Defining & Using ES Modules
math.js:
export const add = (a, b) => a + b;
app.js:
import { add } from './math.js';
console.log(add(2, 3)); // 5
Characteristics:
- Asynchronous loading
- Static analysis and tree shaking
- Supported natively by modern browsers and Node.js (with
.mjs
extension or type="module")
3. Differences between ESM and CJS
Feature | CommonJS | ES Modules |
---|---|---|
Loading | Synchronous | Asynchronous |
Syntax |
require() /module.exports
|
import /export
|
Tree shaking | Limited | Supported |
Dynamic Imports | Not native | Native via import()
|
Node.js support | Native | Native (since v14 fully stable) |
Browser support | No (requires bundling) | Yes (modern browsers) |
4. Dynamic Imports
ES Modules support dynamic imports, which can improve performance by loading modules on-demand:
button.addEventListener('click', async () => {
const module = await import('./math.js');
console.log(module.add(5, 10));
});
5. Interoperability Between ESM and CJS
When using both module types together:
- From CommonJS to ESM:
// CommonJS module requiring an ESM module (Node.js)
(async () => {
const math = await import('./math.mjs');
console.log(math.add(2, 3));
})();
- From ESM to CommonJS:
// ES module importing CommonJS
import { createRequire } from 'module';
const require = createRequire(import.meta.url);
const fs = require('fs');
6. When to Choose Which?
-
CommonJS:
- Legacy projects
- Immediate compatibility with older Node.js versions
-
ES Modules:
- New projects
- Modern JavaScript practices
- Front-end projects without transpilation or bundlers
7. Best Practices
- Prefer ESM in modern projects for better optimization and future-proofing.
- Clearly distinguish modules (
.mjs
for ESM in Node.js or specify"type": "module"
inpackage.json
). - Use dynamic imports to enhance application performance.
8. Conclusion
Both CommonJS and ES Modules have distinct strengths. Understanding their differences and interoperability allows you to make informed choices that best fit your project's needs.
Have you transitioned from CommonJS to ES Modules? Share your experiences and tips below!