Tutorial 9: Handling User Input with Text Fields and Alerts
In this tutorial, we will create a simple iOS app that handles user input using a text field and shows alerts based on the user’s input. We will also use a timer to create a time-based challenge for the user. 1. Setup Your Project Open Xcode and create a new iOS project. Choose the Single View App template, and ensure you select Swift as the programming language. This tutorial will guide you on how to add the necessary UI elements and code. 2. Adding UI Elements In the storyboard file, drag and drop the following elements: UITextField: For entering the secret code. UIButton: For submitting the code. UILabel: To display the current level. UILabel: To show the remaining time on the timer. Connect these elements to your ViewController.swift file using @IBOutlet. 3. The Code Here’s the code you’ll need to add to ViewController.swift to handle the logic for this app. import UIKit class ViewController: UIViewController { // Outlets for UI elements @IBOutlet weak var codeTextField: UITextField! @IBOutlet weak var submitButton: UIButton! @IBOutlet weak var levelLabel: UILabel! @IBOutlet weak var timerLabel: UILabel! // Define the secret codes for each level let codes = ["1234", "5678", "91011", "121314"] // Define the current level and the timer var currentLevel = 0 var timer: Timer? var remainingTime = 10 var totalAttempts = 0 override func viewDidLoad() { super.viewDidLoad() startNewLevel() } // Action for the submit button @IBAction func submitCodeButtonTapped(_ sender: UIButton) { // Get the user input from the text field if let enteredCode = codeTextField.text, !enteredCode.isEmpty { totalAttempts += 1 checkCode(enteredCode) } else { showErrorAlert(message: "Please enter a code to proceed.") } } // Function to start a new level func startNewLevel() { // Reset the timer and update the UI for the new level levelLabel.text = "Level: \(currentLevel + 1)" remainingTime = 10 timerLabel.text = "Timer: \(remainingTime) sec" // Start a countdown timer startTimer() } // Timer functionality func startTimer() { // Invalidating any existing timer timer?.invalidate() // Create a new timer that updates every second timer = Timer.scheduledTimer(timeInterval: 1, target: self, selector: #selector(updateTimer), userInfo: nil, repeats: true) } @objc func updateTimer() { // Decrease remaining time by 1 second remainingTime -= 1 // Update the timer label timerLabel.text = "Timer: \(remainingTime) sec" // If time runs out, end the level with failure if remainingTime
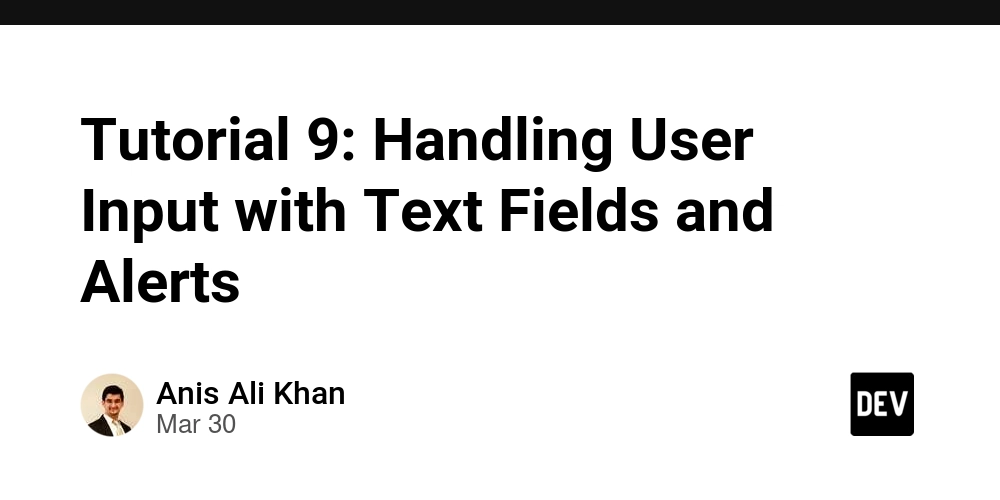
In this tutorial, we will create a simple iOS app that handles user input using a text field and shows alerts based on the user’s input. We will also use a timer to create a time-based challenge for the user.
1. Setup Your Project
Open Xcode and create a new iOS project. Choose the Single View App
template, and ensure you select Swift
as the programming language. This tutorial will guide you on how to add the necessary UI elements and code.
2. Adding UI Elements
In the storyboard file, drag and drop the following elements:
-
UITextField
: For entering the secret code. -
UIButton
: For submitting the code. -
UILabel
: To display the current level. -
UILabel
: To show the remaining time on the timer.
Connect these elements to your ViewController.swift
file using @IBOutlet
.
3. The Code
Here’s the code you’ll need to add to ViewController.swift
to handle the logic for this app.
import UIKit
class ViewController: UIViewController {
// Outlets for UI elements
@IBOutlet weak var codeTextField: UITextField!
@IBOutlet weak var submitButton: UIButton!
@IBOutlet weak var levelLabel: UILabel!
@IBOutlet weak var timerLabel: UILabel!
// Define the secret codes for each level
let codes = ["1234", "5678", "91011", "121314"]
// Define the current level and the timer
var currentLevel = 0
var timer: Timer?
var remainingTime = 10
var totalAttempts = 0
override func viewDidLoad() {
super.viewDidLoad()
startNewLevel()
}
// Action for the submit button
@IBAction func submitCodeButtonTapped(_ sender: UIButton) {
// Get the user input from the text field
if let enteredCode = codeTextField.text, !enteredCode.isEmpty {
totalAttempts += 1
checkCode(enteredCode)
} else {
showErrorAlert(message: "Please enter a code to proceed.")
}
}
// Function to start a new level
func startNewLevel() {
// Reset the timer and update the UI for the new level
levelLabel.text = "Level: \(currentLevel + 1)"
remainingTime = 10
timerLabel.text = "Timer: \(remainingTime) sec"
// Start a countdown timer
startTimer()
}
// Timer functionality
func startTimer() {
// Invalidating any existing timer
timer?.invalidate()
// Create a new timer that updates every second
timer = Timer.scheduledTimer(timeInterval: 1, target: self, selector: #selector(updateTimer), userInfo: nil, repeats: true)
}
@objc func updateTimer() {
// Decrease remaining time by 1 second
remainingTime -= 1
// Update the timer label
timerLabel.text = "Timer: \(remainingTime) sec"
// If time runs out, end the level with failure
if remainingTime <= 0 {
timer?.invalidate()
showFailureAlert(message: "Time's up! Mission failed.")
}
}
// Function to check the entered code
func checkCode(_ enteredCode: String) {
// Check if the entered code is correct
if enteredCode == codes[currentLevel] {
showSuccessAlert(message: "Correct! Proceed to the next level.")
} else {
showFailureAlert(message: "Incorrect code. Try again!")
}
}
// Show success alert
func showSuccessAlert(message: String) {
let alertController = UIAlertController(title: "Success!", message: message, preferredStyle: .alert)
alertController.addAction(UIAlertAction(title: "Next Level", style: .default, handler: { _ in
self.currentLevel += 1
if self.currentLevel < self.codes.count {
self.startNewLevel()
} else {
self.showMissionCompleteAlert()
}
}))
self.present(alertController, animated: true, completion: nil)
}
// Show failure alert
func showFailureAlert(message: String) {
let alertController = UIAlertController(title: "Failure", message: message, preferredStyle: .alert)
alertController.addAction(UIAlertAction(title: "Retry", style: .default, handler: nil))
self.present(alertController, animated: true, completion: nil)
}
// Show error alert (e.g., empty text field)
func showErrorAlert(message: String) {
let alertController = UIAlertController(title: "Error", message: message, preferredStyle: .alert)
alertController.addAction(UIAlertAction(title: "Okay", style: .default, handler: nil))
self.present(alertController, animated: true, completion: nil)
}
// Show mission complete alert
func showMissionCompleteAlert() {
let alertController = UIAlertController(title: "Mission Complete!", message: "You've successfully completed all levels.", preferredStyle: .alert)
alertController.addAction(UIAlertAction(title: "Play Again", style: .default, handler: { _ in
self.resetGame()
}))
self.present(alertController, animated: true, completion: nil)
}
// Reset game after completing all levels
func resetGame() {
currentLevel = 0
totalAttempts = 0
startNewLevel()
}
}
4. Testing the App
Once you have completed the code, run the app in the simulator. Enter a code into the text field, tap the submit button, and see how the alerts and timer interact with the user’s input.
5. Conclusion
In this tutorial, we’ve built an app that challenges users to enter secret codes under a time limit. With user input handling, alerts, and timers, this app demonstrates key features that can be adapted to many types of iOS applications.
Happy coding!