What is Unit Testing? A Comprehensive Guide
Unit testing is a fundamental software testing practice that helps developers validate the correctness of individual components of an application. By isolating and testing each unit separately, developers can catch bugs early, improve code quality, and facilitate smooth integration. Learn more about unit testing. What is Unit Testing? Unit testing involves testing small, isolated units of code, typically functions or methods, to ensure they work as expected. It is commonly automated and performed using frameworks like JUnit (Java), PyTest (Python), and Mocha (JavaScript). Discover test-driven development techniques. Why is Unit Testing Important? Unit testing provides several key benefits: Early Bug Detection – Identifies issues at the development stage. Code Maintainability – Ensures new changes don’t break existing functionality. Simplified Debugging – Makes it easier to pinpoint the root cause of failures. Better Refactoring & Scaling – Helps teams confidently modify and extend applications. Learn more about software testing methodologies. How to Write a Unit Test? A simple unit test follows these steps: Arrange – Set up the necessary data and state. Act – Execute the function or method. Assert – Verify that the result matches the expected outcome. Example: Unit Test in Python using PyTest import pytest from calculator import add def test_addition(): assert add(2, 3) == 5 Run the test using: pytest test_calculator.py Explore best practices for Python testing. Unit Testing vs. Other Testing Methods Feature Unit Testing Integration Testing System Testing Scope Individual components Modules working together Full application Speed Fast Moderate Slow Dependency None External APIs & Databases Complete System Use Case Code correctness Module interactions User experience validation Unit testing forms the foundation of a robust testing strategy, ensuring each component functions correctly before integration. Read more about automation in testing. Best Practices for Unit Testing ✅ Keep Tests Independent – Avoid dependencies on external systems. ✅ Use Meaningful Assertions – Clearly define expected results. ✅ Mock External Dependencies – Use libraries like unittest.mock or Mockito. ✅ Automate Unit Tests – Integrate with CI/CD pipelines. ✅ Maintain Good Test Coverage – Aim for at least 80% code coverage. Learn how Keploy automates test case generation. Top Unit Testing Frameworks Enhance unit testing with these tools: JUnit – Java unit testing framework. PyTest – Python’s powerful testing framework. Mocha – JavaScript test runner. Keploy – AI-driven tool that automates test case generation and API mocking. Explore Keploy for unit testing. Conclusion Unit testing is a crucial practice for maintaining code reliability and stability. By following best practices and leveraging the right tools like Keploy, developers can streamline testing and improve software quality. Learn more about unit testing here.
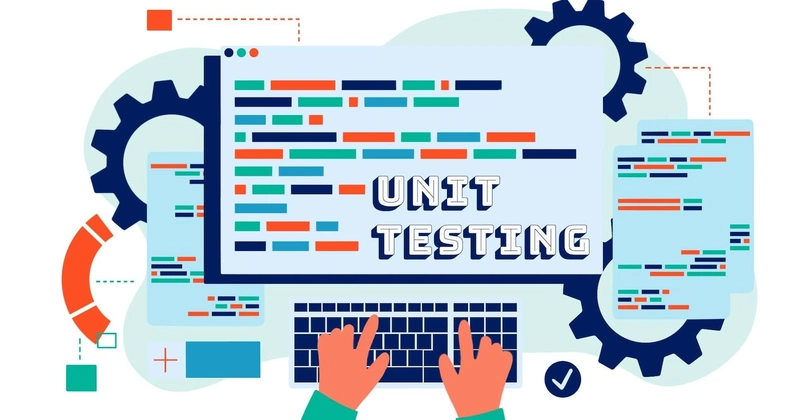
Unit testing is a fundamental software testing practice that helps developers validate the correctness of individual components of an application. By isolating and testing each unit separately, developers can catch bugs early, improve code quality, and facilitate smooth integration. Learn more about unit testing.
What is Unit Testing?
Unit testing involves testing small, isolated units of code, typically functions or methods, to ensure they work as expected. It is commonly automated and performed using frameworks like JUnit (Java), PyTest (Python), and Mocha (JavaScript). Discover test-driven development techniques.
Why is Unit Testing Important?
Unit testing provides several key benefits:
- Early Bug Detection – Identifies issues at the development stage.
- Code Maintainability – Ensures new changes don’t break existing functionality.
- Simplified Debugging – Makes it easier to pinpoint the root cause of failures.
- Better Refactoring & Scaling – Helps teams confidently modify and extend applications.
Learn more about software testing methodologies.
How to Write a Unit Test?
A simple unit test follows these steps:
- Arrange – Set up the necessary data and state.
- Act – Execute the function or method.
- Assert – Verify that the result matches the expected outcome.
Example: Unit Test in Python using PyTest
import pytest
from calculator import add
def test_addition():
assert add(2, 3) == 5
Run the test using:
pytest test_calculator.py
Explore best practices for Python testing.
Unit Testing vs. Other Testing Methods
Feature | Unit Testing | Integration Testing | System Testing |
---|---|---|---|
Scope | Individual components | Modules working together | Full application |
Speed | Fast | Moderate | Slow |
Dependency | None | External APIs & Databases | Complete System |
Use Case | Code correctness | Module interactions | User experience validation |
Unit testing forms the foundation of a robust testing strategy, ensuring each component functions correctly before integration. Read more about automation in testing.
Best Practices for Unit Testing
✅ Keep Tests Independent – Avoid dependencies on external systems. ✅ Use Meaningful Assertions – Clearly define expected results. ✅ Mock External Dependencies – Use libraries like unittest.mock or Mockito. ✅ Automate Unit Tests – Integrate with CI/CD pipelines. ✅ Maintain Good Test Coverage – Aim for at least 80% code coverage.
Learn how Keploy automates test case generation.
Top Unit Testing Frameworks
Enhance unit testing with these tools:
- JUnit – Java unit testing framework.
- PyTest – Python’s powerful testing framework.
- Mocha – JavaScript test runner.
- Keploy – AI-driven tool that automates test case generation and API mocking. Explore Keploy for unit testing.
Conclusion
Unit testing is a crucial practice for maintaining code reliability and stability. By following best practices and leveraging the right tools like Keploy, developers can streamline testing and improve software quality. Learn more about unit testing here.