JavaScript Tail Call Optimization
Comprehensive Guide to JavaScript Tail Call Optimization Introduction Tail Call Optimization (TCO) is an advanced feature in some programming languages where the implementation optimizes recursive function calls to prevent stack overflow. For JavaScript, TCO is a complex and nuanced topic, deeply rooted in the language's execution model, historical context, and its standards, particularly ECMAScript 2015 (ES6). This article aims to provide an in-depth exploration of JavaScript Tail Call Optimization, offering historical insights, code examples, edge cases, alternative approaches, real-world use cases, performance considerations, and advanced debugging techniques. Historical and Technical Context The Evolution of JavaScript JavaScript was originally designed as a lightweight scripting language for client-side web development. Its design has evolved significantly since its inception in 1995. With the introduction of ECMAScript standards, particularly ECMAScript 3 in 1999 and later ECMAScript 2015, the language began to mirror more functional programming paradigms where recursive functions are more prevalent. TCO was proposed to enhance performance in heavily recursive algorithms. While languages like Scheme and Haskell have implemented TCO to great effect, JavaScript’s adoption has been cautious due to concerns about compatibility and performance semantics on various engines. Understanding Tail Calls A tail call occurs when a function calls another function as its last operation before returning a value. With TCO, the JavaScript engine can optimize the call by reusing the current function's stack frame, thereby preventing stack overflow errors in deeply recursive functions. Here is a simple example to understand the concept: function tailCallExample(x, y) { if (y === 0) return x; return tailCallExample(y, x % y); // Tail Call } In this example, tailCallExample is a tail-recursive function that computes the greatest common divisor (GCD) of two numbers. The ES6 Specification ECMAScript 2015 made provisions for TCO, and according to the specification, the JavaScript engine may choose to optimize tail calls. Despite the specification, TCO has not been widely adopted in major JavaScript engines, such as V8 (Chrome, Node.js), SpiderMonkey (Firefox), and JavaScriptCore (Safari). This hesitance is primarily due to performance considerations, potential side effects, and existing code reliant on stack usage. Code Examples of Tail Call Optimization Basic Tail Recursive Function Here is how TCO can be applied in a cleaner manner through a simple countdown function: function countdown(n) { if (n console.log(result)); // Outputs: 120 Real-World Use Cases in Industry Applications Data Processing: Recursive data structures like trees (e.g., XML/JSON parsers) can benefit from TCO for efficient traversal without deep stacks. Game Development: Recursive algorithms are often used for AI. Implementing TCO in game loop calculations can enhance performance where recursion depths may significantly increase. Web Frameworks: Some modern JS frameworks leverage functional programming, where recursion is common (e.g., Redux for state management). Performance Considerations and Optimization Strategies The performance of recursive functions, especially those that can leverage TCO, can be influenced by multiple factors: Engine Implementation: The lack of widespread support (particularly in V8) means that for many developers, TCO CPU savings won’t materialize naturally. Stack Depth: Consider defaults—JavaScript engines typically limit stack sizes; when writing code intended for TCO, carefully consider maximum recursion depths. Memory Usage: TCO can save memory consumption when repeated calls yield the same operations, reducing total stack memory. Profiling Techniques Utilizing profiling tools in environments may help visibly monitor stack memory usage. Chrome's DevTools provide options under the Performance tab to visualize stack traces and their depths. Potential Pitfalls and Advanced Debugging Techniques Stack Overflows: Understanding what scenarios bypass TCO is important to avoid pitfalls. Browser Compatibility: Testing across multiple browsers (especially for TCO support) is crucial, given JavaScript’s historically fragmentary support. Debugging Recursive Functions: Recursion can lead to confusion during debugging; verbose logging or utilizing breakpoints judiciously can uncover problematic areas in code. Conclusion JavaScript Tail Call Optimization presents a fascinating, although complex aspect of the language. While its adoption remains limited in practical applications, the implications of TCO can reshape how we handle recursion in JavaScript—should it become more mainstream among engine implementers. As a senior developer, assessing TC
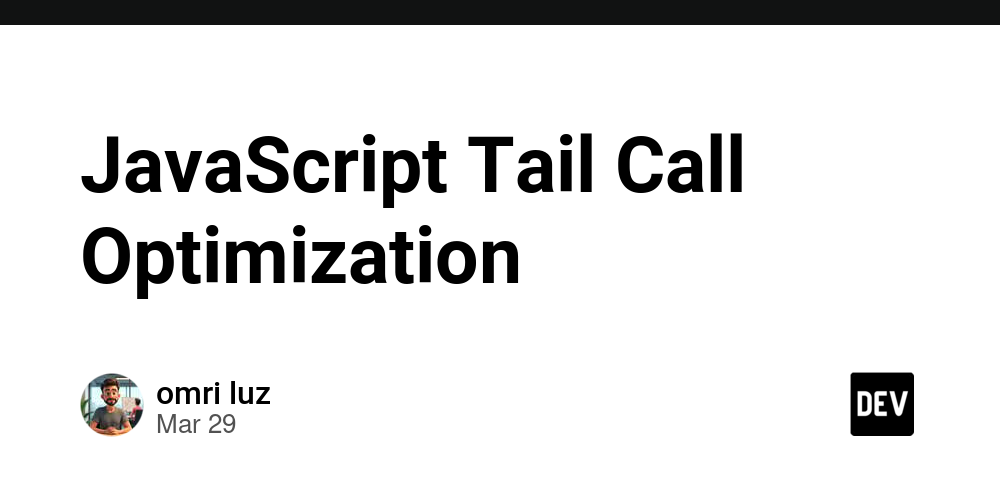
Comprehensive Guide to JavaScript Tail Call Optimization
Introduction
Tail Call Optimization (TCO) is an advanced feature in some programming languages where the implementation optimizes recursive function calls to prevent stack overflow. For JavaScript, TCO is a complex and nuanced topic, deeply rooted in the language's execution model, historical context, and its standards, particularly ECMAScript 2015 (ES6). This article aims to provide an in-depth exploration of JavaScript Tail Call Optimization, offering historical insights, code examples, edge cases, alternative approaches, real-world use cases, performance considerations, and advanced debugging techniques.
Historical and Technical Context
The Evolution of JavaScript
JavaScript was originally designed as a lightweight scripting language for client-side web development. Its design has evolved significantly since its inception in 1995. With the introduction of ECMAScript standards, particularly ECMAScript 3 in 1999 and later ECMAScript 2015, the language began to mirror more functional programming paradigms where recursive functions are more prevalent.
TCO was proposed to enhance performance in heavily recursive algorithms. While languages like Scheme and Haskell have implemented TCO to great effect, JavaScript’s adoption has been cautious due to concerns about compatibility and performance semantics on various engines.
Understanding Tail Calls
A tail call occurs when a function calls another function as its last operation before returning a value. With TCO, the JavaScript engine can optimize the call by reusing the current function's stack frame, thereby preventing stack overflow errors in deeply recursive functions.
Here is a simple example to understand the concept:
function tailCallExample(x, y) {
if (y === 0) return x;
return tailCallExample(y, x % y); // Tail Call
}
In this example, tailCallExample
is a tail-recursive function that computes the greatest common divisor (GCD) of two numbers.
The ES6 Specification
ECMAScript 2015 made provisions for TCO, and according to the specification, the JavaScript engine may choose to optimize tail calls. Despite the specification, TCO has not been widely adopted in major JavaScript engines, such as V8 (Chrome, Node.js), SpiderMonkey (Firefox), and JavaScriptCore (Safari). This hesitance is primarily due to performance considerations, potential side effects, and existing code reliant on stack usage.
Code Examples of Tail Call Optimization
Basic Tail Recursive Function
Here is how TCO can be applied in a cleaner manner through a simple countdown function:
function countdown(n) {
if (n <= 0) {
console.log("Done!");
return;
}
console.log(n);
return countdown(n - 1); // Tail Call
}
countdown(5);
In this basic countdown example, if TCO were applied, it could prevent stack growth with large values of n
.
Advanced Example: Calculating Factorials
Let’s extend our example to calculate factorials, demonstrating how TCO can be leveraged in practice.
function factorialTailRecursive(n, accumulator = 1) {
if (n <= 1) return accumulator;
return factorialTailRecursive(n - 1, n * accumulator); // Tail Call
}
console.log(factorialTailRecursive(5)); // Outputs: 120
Here, the accumulator
parameter helps maintain the computed result of the factorial while allowing the main calculation (n - 1
) to be the last step—making it a tail call.
Fibonacci Sequence
Calculating Fibonacci using TCO can also yield better performance:
function fibonacciTailRecursive(n, a = 0, b = 1) {
if (n === 0) return a;
if (n === 1) return b;
return fibonacciTailRecursive(n - 1, b, a + b); // Tail Call
}
console.log(fibonacciTailRecursive(10)); // Outputs: 55
Illustrating Edge Cases
Despite its advantages, TCO in JavaScript has several edge cases to consider:
- Non-Tail Calls: Any other function call made before returning will negate TCO.
function example(n) {
if (n === 0) return 1;
return example(n - 1) + 1; // NOT a tail call
}
This call can lead to stack overflow for large n
.
- Inlining and Side Effects: If the recursive call is assigned to another variable or if external operations (like console.log) precede the call, TCO cannot occur.
Comparison with Alternative Approaches
While TCO can help manage recursion effectively, we can also explore other methods for handling recursive problems without growing the stack:
- Iterative Solutions: Converting recursive functions into loops prevents excessive stack usage:
function iterativeFactorial(n) {
let result = 1;
for (let i = n; i > 1; i--) {
result *= i;
}
return result;
}
- Function Continuations: Using callbacks or higher-order functions for managing state can replace recursion:
function factorialContinuation(n, cont) {
if (n <= 1) return cont(1);
return factorialContinuation(n - 1, (result) => cont(n * result)); // Continuation-passing style
}
factorialContinuation(5, (result) => console.log(result)); // Outputs: 120
Real-World Use Cases in Industry Applications
- Data Processing: Recursive data structures like trees (e.g., XML/JSON parsers) can benefit from TCO for efficient traversal without deep stacks.
- Game Development: Recursive algorithms are often used for AI. Implementing TCO in game loop calculations can enhance performance where recursion depths may significantly increase.
- Web Frameworks: Some modern JS frameworks leverage functional programming, where recursion is common (e.g., Redux for state management).
Performance Considerations and Optimization Strategies
The performance of recursive functions, especially those that can leverage TCO, can be influenced by multiple factors:
- Engine Implementation: The lack of widespread support (particularly in V8) means that for many developers, TCO CPU savings won’t materialize naturally.
- Stack Depth: Consider defaults—JavaScript engines typically limit stack sizes; when writing code intended for TCO, carefully consider maximum recursion depths.
- Memory Usage: TCO can save memory consumption when repeated calls yield the same operations, reducing total stack memory.
Profiling Techniques
Utilizing profiling tools in environments may help visibly monitor stack memory usage. Chrome's DevTools provide options under the Performance tab to visualize stack traces and their depths.
Potential Pitfalls and Advanced Debugging Techniques
- Stack Overflows: Understanding what scenarios bypass TCO is important to avoid pitfalls.
- Browser Compatibility: Testing across multiple browsers (especially for TCO support) is crucial, given JavaScript’s historically fragmentary support.
- Debugging Recursive Functions: Recursion can lead to confusion during debugging; verbose logging or utilizing breakpoints judiciously can uncover problematic areas in code.
Conclusion
JavaScript Tail Call Optimization presents a fascinating, although complex aspect of the language. While its adoption remains limited in practical applications, the implications of TCO can reshape how we handle recursion in JavaScript—should it become more mainstream among engine implementers. As a senior developer, assessing TCO's practical applications, limits, debugging intricacies, and performance optimization strategies will enable crafting more efficient JavaScript code.
References and Further Reading
- MDN Web Docs: Tail Call Optimization
- ECMAScript 2015 Language Specification
- V8 JavaScript Engine Documentation
- Functional Programming in JavaScript by Luis Atencio
Through this guide, we hope to empower developers to harness the power of Tail Call Optimization in JavaScript, broadening their toolkit for crafting efficient and scalable applications.