Polyfill for call in JavaScript
What is Function.prototype.call? The call method allows you to invoke a function with a specified this context and pass arguments individually. Here’s a quick example: How we can Implement custom call Setting Default Context: context = context || globalThis; If context is null or undefined, it defaults to globalThis. This ensures the function is called in the global context when no context is provided. Creating a Unique Key: const uniqueKey = Symbol("fn"); A Symbol is used to create a unique property key to avoid overwriting existing properties on the context object. Assigning the Function to the Context: context[uniqueKey] = this; The function (this refers to the function being called) is temporarily added as a property of the context object. Calling the Function: const result = context[uniqueKey](...args); The function is called with the provided arguments using the spread syntax. Cleaning Up: delete context[uniqueKey]; The temporary property is removed from the context object to prevent side effects. Returning the Result: return result; The result of the function call is returned.
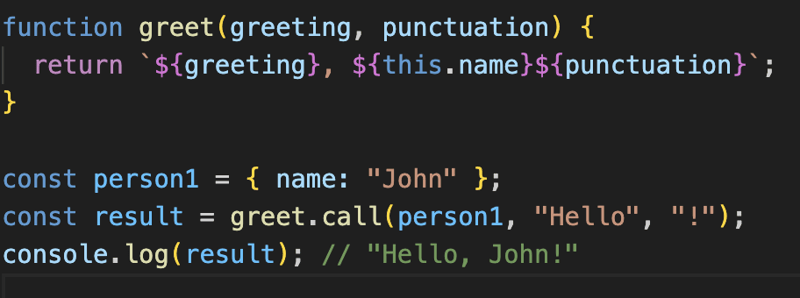
What is Function.prototype.call?
The call method allows you to invoke a function with a specified this context and pass arguments individually. Here’s a quick example:
How we can Implement custom call
Setting Default Context:
context = context || globalThis;
- If context is null or undefined, it defaults to globalThis. This ensures the function is called in the global context when no context is provided.
Creating a Unique Key:
const uniqueKey = Symbol("fn");
- A Symbol is used to create a unique property key to avoid overwriting existing properties on the context object.
Assigning the Function to the Context:
context[uniqueKey] = this;
- The function (this refers to the function being called) is temporarily added as a property of the context object.
Calling the Function:
const result = context[uniqueKey](...args);
- The function is called with the provided arguments using the spread syntax.
Cleaning Up:
delete context[uniqueKey];
- The temporary property is removed from the context object to prevent side effects.
Returning the Result:
return result;
- The result of the function call is returned.