React js-Axios,usestate,useeffect
What is Axios? Axios is a promise-based HTTP client for JavaScript that simplifies making HTTP requests from both the browser and Node.js environments. It supports all modern browsers and works well in React applications for interacting with APIs and handling HTTP requests. Key Features of Axios Promise-based API. Works in both NodeJS and browsers. Automatically transforms JSON data. Supports request and response interceptors. Allows easy handling of timeouts and cancellation of requests. supports making GET, POST, PUT, DELETE, and other HTTP requests. Setting Up Axios in a React Application Before we start making HTTP requests with Axios, you need to install it. If you’re starting a new React project, create one first using create-react-app (if you haven’t already). Step 1: Install Axios To install Axios, run the following command in your React project’s root directory: npm install axios Step 2: Import Axios into Your Component In your React component, import Axios at the top import axios from 'axios'; Step 3: Add Axios as Dependency Install Axios library using the command given below npm i axios Example: import axios from 'axios'; axios.get('https://api.example.com/data') .then(response => { console.log('Data received:', response.data); }) .catch(error => { console.error('Error occurred:', error); }); useState state could only be used in class components. But now, with hooks like useState, you can use state in functional components too. const [state, setState] = useState(initialState) state: It is the value of the current state. setState: It is the function that is used to update the state. initialState: It is the initial value of the state. How Does useState() Work? The useState() hook allows you to add state to functional components in React. It works by: Initialize State: When you call useState(initialValue), it creates a state variable and an updater function. const [count, setCount] = useState(0); State is Preserved Across Renders: React remembers the state value between re-renders of the component. Each time the component renders, React keeps the latest value of count. State Updates with the Updater Function: When you call setCount(newValue) React updates the state and it re-renders the component to reflect the new state value. setCount(count + 1)}>Increment Triggers Re-render: React will re-render only the component where useState was used—ensuring your UI updates automatically when the state changes. Why useState is Helpful: You can easily track changes in your component. No need for complex code like in class components. Counter using useState A common example of using useState is managing the state of a counter with actions to increment and decrement the value. import { useState } from 'react'; export default function Counter() { const [count, setCount] = useState(0); function handleClick() { setCount(count + 1); } return ( Click {count} me ); } What is useEffect? useEffect is a React hook that lets you handle side effects in functional components. Side effects are things like: Fetching data from an API Setting up a timer Updating the DOM directly Subscribing to events Why Use useEffect? In class components, side effects like data fetching were handled in lifecycle methods like componentDidMount and componentDidUpdate. import React, { useEffect } from 'react'; const SimpleEffect = () => { useEffect(() => { // This runs once when the component is loaded console.log("Component has loaded!"); }, []); // Empty array means it runs only once, when the component mounts return Hello, world!; }; export default SimpleEffect;
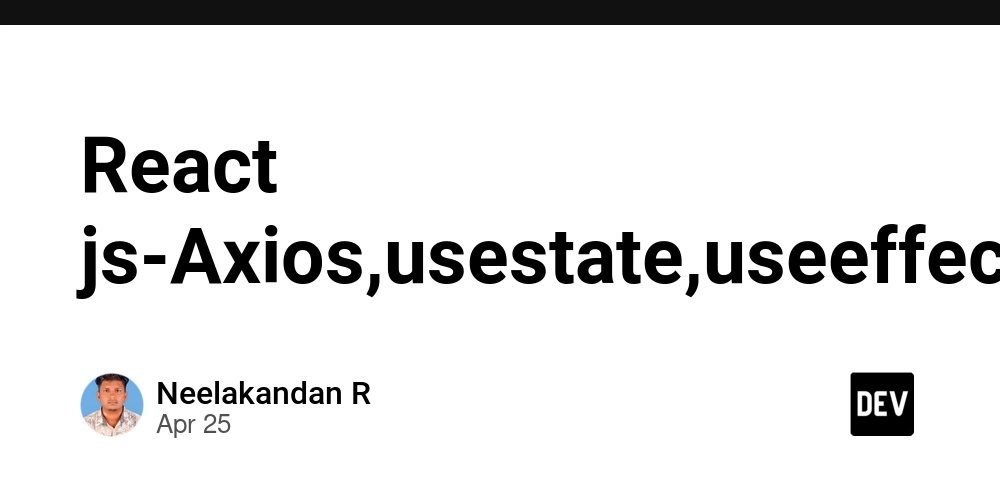
What is Axios?
Axios is a promise-based HTTP client for JavaScript that simplifies making HTTP requests from both the browser and Node.js environments. It supports all modern browsers and works well in React applications for interacting with APIs and handling HTTP requests.
Key Features of Axios
Promise-based API.
Works in both NodeJS and browsers.
Automatically transforms JSON data.
Supports request and response interceptors.
Allows easy handling of timeouts and cancellation of requests.
supports making GET, POST, PUT, DELETE, and other HTTP requests.
Setting Up Axios in a React Application
Before we start making HTTP requests with Axios, you need to install it. If you’re starting a new React project, create one first using create-react-app (if you haven’t already).
Step 1: Install Axios
To install Axios, run the following command in your React project’s root directory:
npm install axios
Step 2: Import Axios into Your Component
In your React component, import Axios at the top
import axios from 'axios';
Step 3: Add Axios as Dependency
Install Axios library using the command given below
npm i axios
Example:
import axios from 'axios';
axios.get('https://api.example.com/data')
.then(response => {
console.log('Data received:', response.data);
})
.catch(error => {
console.error('Error occurred:', error);
});
useState
state could only be used in class components. But now, with hooks like useState, you can use state in functional components too.
const [state, setState] = useState(initialState)
state: It is the value of the current state.
setState: It is the function that is used to update the state.
initialState: It is the initial value of the state.
How Does useState() Work?
The useState() hook allows you to add state to functional components in React. It works by:
- Initialize State: When you call useState(initialValue), it creates a state variable and an updater function.
const [count, setCount] = useState(0);
State is Preserved Across Renders: React remembers the state value between re-renders of the component. Each time the component renders, React keeps the latest value of count.
State Updates with the Updater Function: When you call setCount(newValue) React updates the state and it re-renders the component to reflect the new state value.
setCount(count + 1)}>Increment
- Triggers Re-render: React will re-render only the component where useState was used—ensuring your UI updates automatically when the state changes.
Why useState is Helpful:
You can easily track changes in your component.
No need for complex code like in class components.
Counter using useState
A common example of using useState is managing the state of a counter with actions to increment and decrement the value.
import { useState } from 'react';
export default function Counter() {
const [count, setCount] = useState(0);
function handleClick() {
setCount(count + 1);
}
return (
);
}
What is useEffect?
useEffect is a React hook that lets you handle side effects in functional components. Side effects are things like:
Fetching data from an API
Setting up a timer
Updating the DOM directly
Subscribing to events
Why Use useEffect?
In class components, side effects like data fetching were handled in lifecycle methods like componentDidMount and componentDidUpdate.
import React, { useEffect } from 'react';
const SimpleEffect = () => {
useEffect(() => {
// This runs once when the component is loaded
console.log("Component has loaded!");
}, []); // Empty array means it runs only once, when the component mounts
return Hello, world!
;
};
export default SimpleEffect;