Say Goodbye to Color Guessing: Enable HSL Previews in Tailwind + VSCode
If you're working with Tailwind CSS and custom color variables in Visual Studio Code, you might have noticed an annoying limitation: VSCode doesn't display the handy color preview box when your HSL values aren't properly formatted. Today, we'll explore a simple but impactful solution to this problem. The Problem: Missing Color Previews in VSCode When defining custom CSS variables for colors in your Tailwind CSS project, you might write them like this: --primary: 0 0% 9%; While this is technically correct CSS (using the modern space-separated HSL syntax), VSCode doesn't recognize this format for its color preview functionality. The small color box that normally appears next to color values is conspicuously absent, making it harder to visualize your color scheme at a glance. The Solution: Wrapping HSL Values Properly The fix is remarkably simple - we just need to wrap these values in the hsl() function: --primary: hsl(0 0% 9%); This small change makes all the difference. VSCode now properly recognizes these as color values and displays the color preview box, while maintaining identical functionality in your Tailwind CSS setup. Why This Matters for Tailwind CSS Developers Improved Developer Experience: Instant visual feedback on your color variables speeds up development and reduces errors. Maintains Compatibility: This change doesn't affect how Tailwind CSS processes these variables - they work exactly the same way in your application. Future-Proofing: The hsl() function is standard CSS and works across all modern browsers. Implementation in Your Project To implement this across your project: Search for all instances of --[a-z-]+: \d+ %? \d+%? \d+%?; in your CSS files Replace them with --$1: hsl($2 $3 $4); (using your editor's regex replace functionality) Verify all colors still work as expected Here's how a developer might prompt an AI to perform this CSS color format conversion, along with before/after examples: Example AI Prompt: begin-ai-prompt I need to convert CSS color variables from space-separated HSL to the hsl() function format for better VSCode color previews. Task: Find all CSS custom properties (like --primary: 0 0% 9%;) and rewrite them using hsl(), like --primary: hsl(0 0% 9%);. Matching pattern: Regex: --([a-z-]+): (\d+) (%?)(\d+%?) (%?)(\d+%?); Replacement rule: --$1: hsl($2 $3$4 $5$6); Example Before: :root { --background: 0 0% 98%; } Expected After: :root { --background: hsl(0 0% 98%); } Please apply this change across the entire CSS file while keeping all other code intact. @layer base { :root { --background: 0 0% 98%; --foreground: 0 0% 3.9%; --primary: 0 0% 9%; --secondary: 0 0% 96.1%; --accent: 12 76% 61%; --border: 0 0% 89.8%; } .dark { --background: 0 0% 3.9%; --foreground: 0 0% 98%; --primary: 0 0% 98%; } } end-ai-prompt Full Before & After Example Tested and verified ont ChatGTP and DeepSeek Before Conversion (Original CSS) @layer base { :root { --background: 0 0% 98%; --foreground: 0 0% 3.9%; --primary: 0 0% 9%; --secondary: 0 0% 96.1%; --accent: 12 76% 61%; --border: 0 0% 89.8%; } .dark { --background: 0 0% 3.9%; --foreground: 0 0% 98%; --primary: 0 0% 98%; } } After Conversion (AI-Processed CSS) @layer base { :root { --background: hsl(0 0% 98%); --foreground: hsl(0 0% 3.9%); --primary: hsl(0 0% 9%); --secondary: hsl(0 0% 96.1%); --accent: hsl(12 76% 61%); --border: hsl(0 0% 89.8%); } .dark { --background: hsl(0 0% 3.9%); --foreground: hsl(0 0% 98%); --primary: hsl(0 0% 98%); } } Key Notes for the AI Prompt The regex ensures only CSS custom properties with HSL-like values are modified. The replacement preserves the original variable names (--background, --primary, etc.). The AI should not modify other CSS rules (like @layer, .dark, or non-color properties). The change is purely syntactic—functionality remains identical. Bonus Tip: VSCode HSL Preview For even better HSL support in VSCode, consider installing the CSS Variable AutoComplete extension, which provides enhanced color previews and autocomplete for CSS variables. Conclusion This tiny syntax adjustment - adding hsl() around your color variables - requires minimal effort but delivers immediate quality-of-life improvements for frontend developers working with Tailwind CSS. It's one of those small optimizations that, once you start using it, you'll wonder how you worked without it. Have you made similar small-but-impactful optimizations to your development workflow? Share your tips in the comments below!
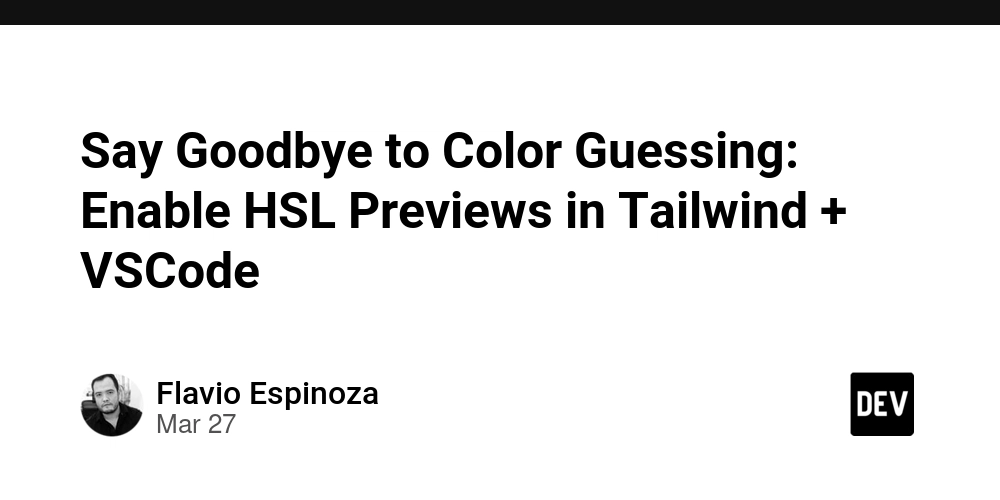
If you're working with Tailwind CSS
and custom color variables in Visual Studio Code, you might have noticed an annoying limitation: VSCode doesn't display the handy color preview box when your HSL values aren't properly formatted. Today, we'll explore a simple but impactful solution to this problem.
The Problem: Missing Color Previews in VSCode
When defining custom CSS variables for colors in your Tailwind CSS project, you might write them like this:
--primary: 0 0% 9%;
While this is technically correct CSS (using the modern space-separated HSL syntax), VSCode doesn't recognize this format for its color preview functionality. The small color box that normally appears next to color values is conspicuously absent, making it harder to visualize your color scheme at a glance.
The Solution: Wrapping HSL Values Properly
The fix is remarkably simple - we just need to wrap these values in the hsl()
function:
--primary: hsl(0 0% 9%);
This small change makes all the difference. VSCode now properly recognizes these as color values and displays the color preview box, while maintaining identical functionality in your Tailwind CSS setup.
Why This Matters for Tailwind CSS Developers
- Improved Developer Experience: Instant visual feedback on your color variables speeds up development and reduces errors.
- Maintains Compatibility: This change doesn't affect how Tailwind CSS processes these variables - they work exactly the same way in your application.
-
Future-Proofing: The
hsl()
function is standard CSS and works across all modern browsers.
Implementation in Your Project
To implement this across your project:
- Search for all instances of
--[a-z-]+: \d+ %? \d+%? \d+%?;
in your CSS files - Replace them with
--$1: hsl($2 $3 $4);
(using your editor's regex replace functionality) - Verify all colors still work as expected
Here's how a developer might prompt an AI to perform this CSS color format conversion, along with before/after examples:
Example AI Prompt:
begin-ai-prompt
I need to convert CSS color variables from space-separated HSL to the hsl()
function format for better VSCode color previews.
Task:
Find all CSS custom properties (like --primary: 0 0% 9%;
) and rewrite them using hsl()
, like --primary: hsl(0 0% 9%);
.
Matching pattern:
Regex: --([a-z-]+): (\d+) (%?)(\d+%?) (%?)(\d+%?);
Replacement rule:
--$1: hsl($2 $3$4 $5$6);
Example Before:
:root {
--background: 0 0% 98%;
}
Expected After:
:root {
--background: hsl(0 0% 98%);
}
Please apply this change across the entire CSS file while keeping all other code intact.
@layer base {
:root {
--background: 0 0% 98%;
--foreground: 0 0% 3.9%;
--primary: 0 0% 9%;
--secondary: 0 0% 96.1%;
--accent: 12 76% 61%;
--border: 0 0% 89.8%;
}
.dark {
--background: 0 0% 3.9%;
--foreground: 0 0% 98%;
--primary: 0 0% 98%;
}
}
end-ai-prompt
Full Before & After Example
Tested and verified ont ChatGTP and DeepSeek
Before Conversion (Original CSS)
@layer base {
:root {
--background: 0 0% 98%;
--foreground: 0 0% 3.9%;
--primary: 0 0% 9%;
--secondary: 0 0% 96.1%;
--accent: 12 76% 61%;
--border: 0 0% 89.8%;
}
.dark {
--background: 0 0% 3.9%;
--foreground: 0 0% 98%;
--primary: 0 0% 98%;
}
}
After Conversion (AI-Processed CSS)
@layer base {
:root {
--background: hsl(0 0% 98%);
--foreground: hsl(0 0% 3.9%);
--primary: hsl(0 0% 9%);
--secondary: hsl(0 0% 96.1%);
--accent: hsl(12 76% 61%);
--border: hsl(0 0% 89.8%);
}
.dark {
--background: hsl(0 0% 3.9%);
--foreground: hsl(0 0% 98%);
--primary: hsl(0 0% 98%);
}
}
Key Notes for the AI Prompt
- The regex ensures only CSS custom properties with HSL-like values are modified.
- The replacement preserves the original variable names (
--background
,--primary
, etc.). - The AI should not modify other CSS rules (like
@layer
,.dark
, or non-color properties). - The change is purely syntactic—functionality remains identical.
Bonus Tip: VSCode HSL Preview
For even better HSL support in VSCode, consider installing the CSS Variable AutoComplete extension, which provides enhanced color previews and autocomplete for CSS variables.
Conclusion
This tiny syntax adjustment - adding hsl()
around your color variables - requires minimal effort but delivers immediate quality-of-life improvements for frontend developers working with Tailwind CSS. It's one of those small optimizations that, once you start using it, you'll wonder how you worked without it.
Have you made similar small-but-impactful optimizations to your development workflow? Share your tips in the comments below!