5 Utilities to Format File Sizes in JavaScript
** 5 Utilities to Format File Sizes in JavaScript ** When working with file uploads, downloads, or managing files in your app, displaying file sizes in a human-readable format is essential. Instead of showing raw bytes, it’s much more user-friendly to display something like "5 KB" or "2 MB" rather than just "5120 bytes". This makes your app more polished and easier to use. Here are 5 utilities to help you format file sizes in JavaScript. These libraries and functions will make it easy to show file sizes in a friendly way. 1. file-size-pretty file-size-pretty is a simple, lightweight package to format file sizes into human-readable strings. It supports all common units (KB, MB, GB, etc.) and is very easy to use. Installation: npm install file-size-pretty Usage: const formatSize = require('file-size-pretty'); console.log(formatSize(1048576)); // "1 MB" This package is perfect for when you need something fast and easy without any frills. 2. filesize filesize is another excellent package for formatting file sizes. It provides options to show the size in various formats and allows you to customize the output. Installation: npm install filesize Usage: const filesize = require('filesize'); console.log(filesize(1048576)); // "1 MB" With filesize, you can easily format file sizes, and you also get a nice, customizable API for more control. 3. humanize-plus humanize-plus is a utility for turning numbers into more human-readable formats. It includes the humanize.filesize() method that converts bytes into a readable format. Installation: npm install humanize-plus Usage: const humanize = require('humanize-plus'); console.log(humanize.filesize(1048576)); // "1 MB" This one is a great choice if you need a general-purpose humanizing utility, with a simple API for file sizes. 4. pretty-bytes pretty-bytes is a minimal library that converts bytes into a readable string. It’s very lightweight and focuses on doing one thing — formatting file sizes in a simple way. Installation: npm install pretty-bytes Usage: const prettyBytes = require('pretty-bytes'); console.log(prettyBytes(1048576)); // "1 MB" If you’re looking for something ultra-lightweight, pretty-bytes is an excellent choice. 5. filesize.js filesize.js is a lightweight library for formatting file sizes. It’s known for being simple and fast, with great support for large numbers. Installation: npm install filesize.js Usage: const filesize = require('filesize'); console.log(filesize(1048576)); // "1 MB" With support for binary prefixes and large files, this is a great utility for serious projects that need precise formatting. Conclusion There are plenty of options when it comes to formatting file sizes in JavaScript. Whether you need something lightweight and simple like file-size-pretty or something more feature-rich like filesize, there’s a tool for every use case. Which one do you prefer? Feel free to drop a comment below and let me know if I missed any of your favorite utilities! Happy coding!
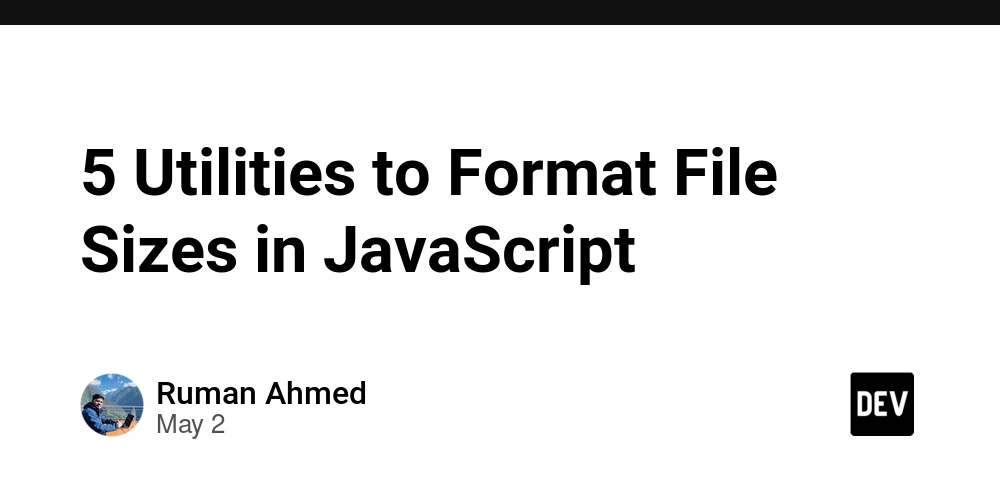
**
5 Utilities to Format File Sizes in JavaScript
**
When working with file uploads, downloads, or managing files in your app, displaying file sizes in a human-readable format is essential. Instead of showing raw bytes, it’s much more user-friendly to display something like "5 KB" or "2 MB" rather than just "5120 bytes". This makes your app more polished and easier to use.
Here are 5 utilities to help you format file sizes in JavaScript. These libraries and functions will make it easy to show file sizes in a friendly way.
1. file-size-pretty
file-size-pretty
is a simple, lightweight package to format file sizes into human-readable strings. It supports all common units (KB, MB, GB, etc.) and is very easy to use.
Installation:
npm install file-size-pretty
Usage:
const formatSize = require('file-size-pretty');
console.log(formatSize(1048576)); // "1 MB"
This package is perfect for when you need something fast and easy without any frills.
2. filesize
filesize
is another excellent package for formatting file sizes. It provides options to show the size in various formats and allows you to customize the output.
Installation:
npm install filesize
Usage:
const filesize = require('filesize');
console.log(filesize(1048576)); // "1 MB"
With filesize
, you can easily format file sizes, and you also get a nice, customizable API for more control.
3. humanize-plus
humanize-plus
is a utility for turning numbers into more human-readable formats. It includes the humanize.filesize()
method that converts bytes into a readable format.
Installation:
npm install humanize-plus
Usage:
const humanize = require('humanize-plus');
console.log(humanize.filesize(1048576)); // "1 MB"
This one is a great choice if you need a general-purpose humanizing utility, with a simple API for file sizes.
4. pretty-bytes
pretty-bytes
is a minimal library that converts bytes into a readable string. It’s very lightweight and focuses on doing one thing — formatting file sizes in a simple way.
Installation:
npm install pretty-bytes
Usage:
const prettyBytes = require('pretty-bytes');
console.log(prettyBytes(1048576)); // "1 MB"
If you’re looking for something ultra-lightweight, pretty-bytes
is an excellent choice.
5. filesize.js
filesize.js
is a lightweight library for formatting file sizes. It’s known for being simple and fast, with great support for large numbers.
Installation:
npm install filesize.js
Usage:
const filesize = require('filesize');
console.log(filesize(1048576)); // "1 MB"
With support for binary prefixes and large files, this is a great utility for serious projects that need precise formatting.
Conclusion
There are plenty of options when it comes to formatting file sizes in JavaScript. Whether you need something lightweight and simple like file-size-pretty
or something more feature-rich like filesize
, there’s a tool for every use case.
Which one do you prefer? Feel free to drop a comment below and let me know if I missed any of your favorite utilities!
Happy coding!