Building Autonomous AI Agents with DeepSeek, LangChain, and AWS Lambda
Introduction: The Rise of Agentic Workflows Autonomous AI agents are reshaping how businesses approach decision-making, automation, and data-driven workflows. These agents, powered by Large Language Models (LLMs) like DeepSeek, are capable of processing complex tasks, retrieving real-time information, and executing decisions autonomously. As this technology becomes more widely accessible, the potential for AI-driven workflows has expanded, making it an exciting time to explore how to leverage these systems in real-world applications. In this blog, I’ll walk through how to build an agentic AI workflow using DeepSeek, LangChain, AWS Lambda, and AWS Step Functions, showcasing how these tools can be combined to build scalable, intelligent automation systems. Why DeepSeek for Autonomous AI Agents? DeepSeek is an open-source LLM optimized for various use cases that require long context lengths (16K tokens), which is ideal for multi-step reasoning, and advanced NLP capabilities for structured decision-making. It is efficient in AI-driven automation, making it a suitable option for building intelligent agents. DeepSeek’s flexibility and scalability make it a great choice for integrating into workflows that require dynamic information retrieval and autonomous decision-making. Step 1: Setting Up the Environment Before we start building, it’s important to get your environment properly set up. Here's how to get started: Install Required Packages You’ll need the following packages: pip install langchain boto3 sagemaker transformers fastapi uvicorn Initialize DeepSeek Model in SageMaker For running the model at scale, it’s best to use Amazon SageMaker: from transformers import AutoModelForCausalLM, AutoTokenizer import sagemaker model_name = "deepseek-ai/deepseek-llm-7b" tokenizer = AutoTokenizer.from_pretrained(model_name) model = AutoModelForCausalLM.from_pretrained(model_name) session = sagemaker.Session() role = sagemaker.get_execution_role() Step 2: Building an AI Agent with LangChain To create an intelligent agent, LangChain is an ideal framework. It simplifies the process of integrating LLMs with tools and workflows. from langchain.llms import HuggingFacePipeline from langchain.agents import initialize_agent, AgentType from langchain.memory import ConversationBufferMemory llm = HuggingFacePipeline.from_model(model_name) memory = ConversationBufferMemory(memory_key="chat_history") agent = initialize_agent( llm=llm, tools=[], # Tools will be added in later steps agent=AgentType.ZERO_SHOT_REACT_DESCRIPTION, memory=memory ) This setup creates an agent that can remember previous interactions and utilize DeepSeek for reasoning. Step 3: Enhancing the Agent with AWS-Powered Tools Adding AWS Lambda Integration You can extend the agent’s capabilities by integrating AWS Lambda. This allows the agent to invoke serverless functions that can interact with external APIs or perform specific tasks. Example: Stock Market Price Lookup Tool import boto3, json lambda_client = boto3.client("lambda") def stock_price_tool(query): response = lambda_client.invoke( FunctionName="GetStockPrice", Payload=json.dumps({"ticker": query}) ) return json.loads(response["Payload"].read())["price"] agent.add_tool(stock_price_tool, name="Stock Price Checker") This tool allows the agent to query real-time stock prices and respond accordingly. Step 4: Automating the Agent Workflow with AWS Step Functions AWS Step Functions are key for orchestrating complex workflows. They allow you to define a sequence of tasks in a state machine, ensuring that each step in the process is completed successfully. Example Workflow: Customer Support Automation You can define a workflow where customer queries are received, processed, and handled by an AI agent: Step Functions JSON Definition { "StartAt": "Receive Customer Query", "States": { "Receive Customer Query": { "Type": "Task", "Resource": "arn:aws:lambda:customer-service-bot", "Next": "Classify Intent" }, "Classify Intent": { "Type": "Task", "Resource": "arn:aws:lambda:classify-intent", "Next": "Query DeepSeek" }, "Query DeepSeek": { "Type": "Task", "Resource": "arn:aws:lambda:deepseek-response", "End": true } } } This example demonstrates how to automate customer support workflows by integrating AWS services like Lambda and Step Functions. Step 5: Deploying the Agent as a Serverless API To make the agent accessible, you can deploy it as a serverless API using FastAPI and AWS Lambda. This allows you to expose the agent's capabilities through an HTTP interface. from fastapi import FastAPI import requests app = FastAPI() @app.get("/query") def query_agent(text: str):
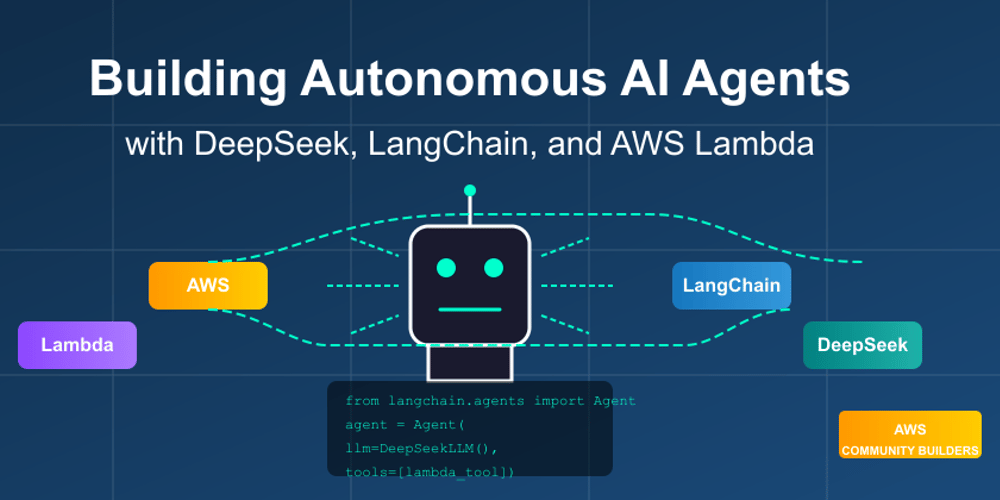
Introduction: The Rise of Agentic Workflows
Autonomous AI agents are reshaping how businesses approach decision-making, automation, and data-driven workflows. These agents, powered by Large Language Models (LLMs) like DeepSeek, are capable of processing complex tasks, retrieving real-time information, and executing decisions autonomously. As this technology becomes more widely accessible, the potential for AI-driven workflows has expanded, making it an exciting time to explore how to leverage these systems in real-world applications.
In this blog, I’ll walk through how to build an agentic AI workflow using DeepSeek, LangChain, AWS Lambda, and AWS Step Functions, showcasing how these tools can be combined to build scalable, intelligent automation systems.
Why DeepSeek for Autonomous AI Agents?
DeepSeek is an open-source LLM optimized for various use cases that require long context lengths (16K tokens), which is ideal for multi-step reasoning, and advanced NLP capabilities for structured decision-making. It is efficient in AI-driven automation, making it a suitable option for building intelligent agents.
DeepSeek’s flexibility and scalability make it a great choice for integrating into workflows that require dynamic information retrieval and autonomous decision-making.
Step 1: Setting Up the Environment
Before we start building, it’s important to get your environment properly set up. Here's how to get started:
Install Required Packages
You’ll need the following packages:
pip install langchain boto3 sagemaker transformers fastapi uvicorn
Initialize DeepSeek Model in SageMaker
For running the model at scale, it’s best to use Amazon SageMaker:
from transformers import AutoModelForCausalLM, AutoTokenizer
import sagemaker
model_name = "deepseek-ai/deepseek-llm-7b"
tokenizer = AutoTokenizer.from_pretrained(model_name)
model = AutoModelForCausalLM.from_pretrained(model_name)
session = sagemaker.Session()
role = sagemaker.get_execution_role()
Step 2: Building an AI Agent with LangChain
To create an intelligent agent, LangChain is an ideal framework. It simplifies the process of integrating LLMs with tools and workflows.
from langchain.llms import HuggingFacePipeline
from langchain.agents import initialize_agent, AgentType
from langchain.memory import ConversationBufferMemory
llm = HuggingFacePipeline.from_model(model_name)
memory = ConversationBufferMemory(memory_key="chat_history")
agent = initialize_agent(
llm=llm,
tools=[], # Tools will be added in later steps
agent=AgentType.ZERO_SHOT_REACT_DESCRIPTION,
memory=memory
)
This setup creates an agent that can remember previous interactions and utilize DeepSeek for reasoning.
Step 3: Enhancing the Agent with AWS-Powered Tools
Adding AWS Lambda Integration
You can extend the agent’s capabilities by integrating AWS Lambda. This allows the agent to invoke serverless functions that can interact with external APIs or perform specific tasks.
Example: Stock Market Price Lookup Tool
import boto3, json
lambda_client = boto3.client("lambda")
def stock_price_tool(query):
response = lambda_client.invoke(
FunctionName="GetStockPrice",
Payload=json.dumps({"ticker": query})
)
return json.loads(response["Payload"].read())["price"]
agent.add_tool(stock_price_tool, name="Stock Price Checker")
This tool allows the agent to query real-time stock prices and respond accordingly.
Step 4: Automating the Agent Workflow with AWS Step Functions
AWS Step Functions are key for orchestrating complex workflows. They allow you to define a sequence of tasks in a state machine, ensuring that each step in the process is completed successfully.
Example Workflow: Customer Support Automation
You can define a workflow where customer queries are received, processed, and handled by an AI agent:
Step Functions JSON Definition
{
"StartAt": "Receive Customer Query",
"States": {
"Receive Customer Query": {
"Type": "Task",
"Resource": "arn:aws:lambda:customer-service-bot",
"Next": "Classify Intent"
},
"Classify Intent": {
"Type": "Task",
"Resource": "arn:aws:lambda:classify-intent",
"Next": "Query DeepSeek"
},
"Query DeepSeek": {
"Type": "Task",
"Resource": "arn:aws:lambda:deepseek-response",
"End": true
}
}
}
This example demonstrates how to automate customer support workflows by integrating AWS services like Lambda and Step Functions.
Step 5: Deploying the Agent as a Serverless API
To make the agent accessible, you can deploy it as a serverless API using FastAPI and AWS Lambda. This allows you to expose the agent's capabilities through an HTTP interface.
from fastapi import FastAPI
import requests
app = FastAPI()
@app.get("/query")
def query_agent(text: str):
response = requests.post("https://sagemaker-endpoint-url", json={"inputs": text})
return response.json()
Deploying with AWS Lambda and API Gateway
- Package FastAPI as a Lambda function.
- Expose the API via AWS API Gateway.
- Enable inference requests from external services.
This setup allows external services or users to interact with your agent via a simple HTTP interface.
Step 6: Future Enhancements
As the AI landscape continues to evolve, there are several areas to explore further: