Caching Strategies (Redis, Memcached)
Caching Strategies: Redis vs. Memcached Introduction: Caching is crucial for optimizing application performance by storing frequently accessed data in memory. Redis and Memcached are popular in-memory data stores often used for caching, but they differ in features and capabilities. This article compares their strengths and weaknesses. Prerequisites: Both Redis and Memcached require a basic understanding of networking and server administration. Familiarity with programming languages like Python or PHP is beneficial for implementing caching strategies. Advantages: Reduced Database Load: Caching significantly reduces the load on databases by serving data directly from memory, leading to faster response times. Improved Scalability: Caching can improve application scalability by handling a larger number of requests without overwhelming the backend systems. Enhanced User Experience: Faster response times result in a smoother and more responsive user experience. Disadvantages: Data Consistency: Maintaining data consistency between the cache and the database requires careful management and potential strategies like cache invalidation. Cache Eviction: Limited memory necessitates eviction policies (e.g., LRU, FIFO) which can lead to data loss if not managed carefully. Complexity: Implementing and managing caching strategies can add complexity to your application architecture. Features: Feature Redis Memcached Data Structures Strings, Lists, Sets, Hashes, Sorted Sets Key-Value pairs only Persistence Supports persistent storage Primarily in-memory Client Libraries Wide range of libraries Wide range of libraries Example (Python with Redis): import redis r = redis.Redis(host='localhost', port=6379, db=0) r.set('name', 'John Doe') name = r.get('name').decode('utf-8') print(name) # Output: John Doe Conclusion: Both Redis and Memcached are valuable tools for improving application performance. Memcached excels in its simplicity and speed for basic key-value storage, while Redis offers a richer set of data structures and persistence capabilities. The choice depends on the specific application requirements and complexity. Careful consideration of data consistency and eviction strategies is vital for successful caching implementation regardless of the chosen technology.
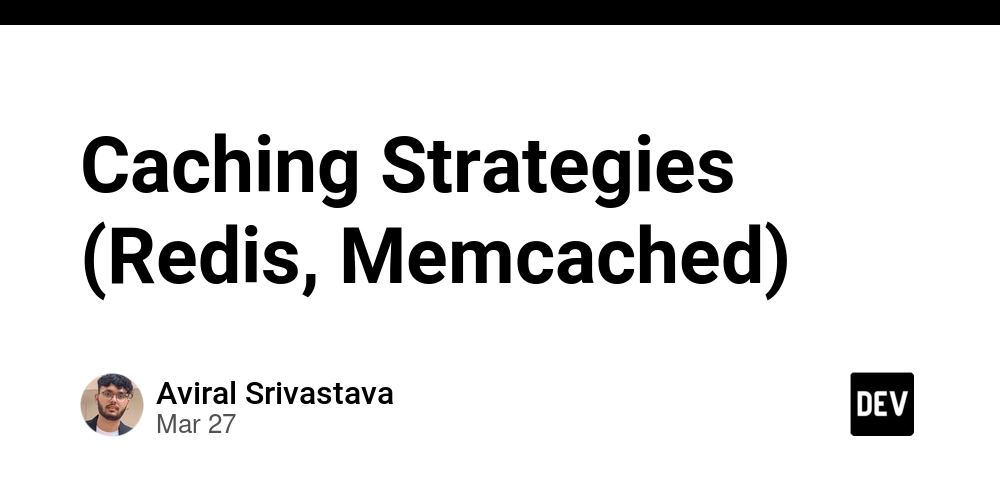
Caching Strategies: Redis vs. Memcached
Introduction:
Caching is crucial for optimizing application performance by storing frequently accessed data in memory. Redis and Memcached are popular in-memory data stores often used for caching, but they differ in features and capabilities. This article compares their strengths and weaknesses.
Prerequisites:
Both Redis and Memcached require a basic understanding of networking and server administration. Familiarity with programming languages like Python or PHP is beneficial for implementing caching strategies.
Advantages:
- Reduced Database Load: Caching significantly reduces the load on databases by serving data directly from memory, leading to faster response times.
- Improved Scalability: Caching can improve application scalability by handling a larger number of requests without overwhelming the backend systems.
- Enhanced User Experience: Faster response times result in a smoother and more responsive user experience.
Disadvantages:
- Data Consistency: Maintaining data consistency between the cache and the database requires careful management and potential strategies like cache invalidation.
- Cache Eviction: Limited memory necessitates eviction policies (e.g., LRU, FIFO) which can lead to data loss if not managed carefully.
- Complexity: Implementing and managing caching strategies can add complexity to your application architecture.
Features:
Feature | Redis | Memcached |
---|---|---|
Data Structures | Strings, Lists, Sets, Hashes, Sorted Sets | Key-Value pairs only |
Persistence | Supports persistent storage | Primarily in-memory |
Client Libraries | Wide range of libraries | Wide range of libraries |
Example (Python with Redis):
import redis
r = redis.Redis(host='localhost', port=6379, db=0)
r.set('name', 'John Doe')
name = r.get('name').decode('utf-8')
print(name) # Output: John Doe
Conclusion:
Both Redis and Memcached are valuable tools for improving application performance. Memcached excels in its simplicity and speed for basic key-value storage, while Redis offers a richer set of data structures and persistence capabilities. The choice depends on the specific application requirements and complexity. Careful consideration of data consistency and eviction strategies is vital for successful caching implementation regardless of the chosen technology.